A Servo control library that works on any output pin, not just the PWM pins
Fork of UniServ by
UniServ.cpp
00001 /* UniServ Servo Library 00002 * Copyright (c) 2010 Matt Parsons 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 00024 /** Default metoda za servo motor C90 00025 * @code 00026 * #include "mbed.h" 00027 * #include "UniServ.h" 00028 * 00029 * t.start(); 00030 * sMotor.write_us(2000); 00031 * t.stop(); 00032 * pc.printf("%f\n\r", t.read()); 00033 * wait_ms(1000); 00034 * t.reset(); 00035 * t.start(); 00036 * sMotor.write_us(0); 00037 * t.stop(); 00038 * pc.printf("%f\n\r", t.read()); 00039 * wait_ms(1000); 00040 * t.reset(); 00041 * t.start(); 00042 * sMotor.write_us(3000); 00043 * t.stop(); 00044 * pc.printf("%f\n\r", t.read()); 00045 * wait_ms(1000); 00046 * t.reset(); 00047 * t.start(); 00048 * sMotor.write_us(1000); 00049 * t.stop(); 00050 * pc.printf("%f\n\r", t.read()); 00051 * wait_ms(1000); 00052 * sMotor.Disable(); 00053 * 00054 * Dodao metodu Disable() zbog toga da se oslobodi 00055 * cpu dok se ne izvrši slijedeća operacija 00056 * @endcode 00057 */ 00058 00059 #include "mbed.h" 00060 #include "UniServ.h" 00061 00062 UniServ::UniServ(PinName pin) : ServPin(pin){ 00063 Period=20000; 00064 Position=1500; 00065 ServMin=400; 00066 ServMax=2600; 00067 00068 Pulse.attach_us(this,&UniServ::SigStart,Period); 00069 } 00070 00071 void UniServ::SigStart(){ 00072 ServPin=1; 00073 PulseEnd.attach_us(this,&UniServ::SigStop,Position); 00074 } 00075 00076 void UniServ::SigStop(){ 00077 ServPin=0; 00078 } 00079 00080 void UniServ::write_us(int PosIn){ 00081 Position=PosIn; 00082 if(PosIn<ServMin){Position=ServMin;} 00083 if(PosIn>ServMax){Position=ServMax;} 00084 } 00085 00086 int UniServ::read_us(){ 00087 return Position; 00088 } 00089 void UniServ::Disable() { 00090 Pulse.detach(); 00091 } 00092 00093 00094
Generated on Wed Jul 27 2022 02:30:08 by
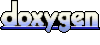