
Smartage application
Dependencies: BufferedSerial SX1276GenericLib USBDeviceHT mbed Crypto X_NUCLEO_IKS01A2
Fork of STM32L0_LoRa by
utils.cpp
00001 #include "main.h" 00002 00003 time_t cvt_date(char const *date, char const *time); 00004 00005 BufferedSerial *ser; 00006 #ifdef FEATURE_USBSERIAL 00007 USBSerialBuffered *usb; 00008 #endif 00009 bool _useDprintf; 00010 00011 void InitSerial(int timeout, DigitalOut *led) 00012 { 00013 _useDprintf = true; 00014 bool uartActive; 00015 { 00016 { 00017 // need to turn rx low to avoid floating signal 00018 DigitalOut rx(USBRX); 00019 rx = 0; 00020 } 00021 DigitalIn uartRX(USBRX); 00022 uartActive = uartRX.read(); 00023 } 00024 #ifdef FEATURE_USBSERIAL 00025 if (!uartActive) { 00026 usb = new USBSerialBuffered(); 00027 Timer t; 00028 t.start(); 00029 while(!usb->connected()) { 00030 if (led) 00031 *led = !*led; 00032 wait_ms(100); 00033 if (timeout) { 00034 if (t.read_ms() >= timeout) 00035 return; 00036 } 00037 } 00038 return; 00039 } else { 00040 #else 00041 { 00042 #endif 00043 ser = new BufferedSerial(USBTX, USBRX); 00044 ser->baud(230400); 00045 ser->format(8); 00046 } 00047 time_t t = cvt_date(__DATE__, __TIME__); 00048 if (t > time(NULL)) { 00049 set_time(t); 00050 } 00051 00052 } 00053 00054 void printTimeStamp() 00055 { 00056 static LowPowerTimer *timer; 00057 if (!timer) { 00058 timer = new LowPowerTimer(); 00059 timer->start(); 00060 } 00061 time_t seconds = time(NULL); 00062 struct tm *tm = localtime(&seconds); 00063 int usecs = timer->read_us(); 00064 if (usecs < 0) { 00065 usecs = 0; 00066 timer->stop(); 00067 timer->reset(); 00068 timer->start(); 00069 } 00070 int msecs = usecs % 1000000; 00071 00072 rprintf("%02d:%02d:%02d.%06d ", tm->tm_hour, tm->tm_min, tm->tm_sec, msecs); 00073 } 00074 00075 void dprintf(const char *format, ...) 00076 { 00077 std::va_list arg; 00078 00079 va_start(arg, format); 00080 VAprintf(true, true, _useDprintf, format, arg); 00081 va_end(arg); 00082 } 00083 00084 void rprintf(const char *format, ...) 00085 { 00086 std::va_list arg; 00087 00088 va_start(arg, format); 00089 VAprintf(false, false, _useDprintf, format, arg); 00090 va_end(arg); 00091 } 00092 00093 void VAprintf(bool timstamp, bool newline, bool printEnabled, const char *format, va_list arg) 00094 { 00095 if (!printEnabled) 00096 return; 00097 00098 if (timstamp) 00099 printTimeStamp(); 00100 #ifdef FEATURE_USBSERIAL 00101 if (usb) { 00102 usb->vprintf_irqsafe(format, arg); 00103 if (newline) 00104 usb->printf_irqsafe("\r\n"); 00105 #else 00106 if (0) { 00107 #endif 00108 } else if (ser) { 00109 // serial jas 00110 int r = 0; 00111 r = vsnprintf(NULL, 0, format, arg); 00112 if (r < 82) { 00113 char buffer[82+1]; 00114 00115 vsnprintf(buffer, sizeof(buffer), format, arg); 00116 r = ser->write(buffer, r); 00117 } else { 00118 char *buffer = new char[r+1]; 00119 if (buffer) { 00120 vsnprintf(buffer, r+1, format, arg); 00121 r = ser->write(buffer, r); 00122 delete[] buffer; 00123 } else { 00124 error("%s %d cannot alloc memory (%d bytes)!\r\n", __FILE__, __LINE__, r+1); 00125 r = 0; 00126 } 00127 } 00128 if (newline) 00129 ser->write("\r\n", 2); 00130 } 00131 } 00132 00133 00134 void dump(const char *title, const void *data, int len, bool dwords) 00135 { 00136 dprintf("dump(\"%s\", 0x%x, %d bytes)", title, data, len); 00137 00138 int i, j, cnt; 00139 unsigned char *u; 00140 const int width = 16; 00141 const int seppos = 7; 00142 00143 cnt = 0; 00144 u = (unsigned char *)data; 00145 while (len > 0) { 00146 rprintf("%08x: ", (unsigned int)data + cnt); 00147 if (dwords) { 00148 unsigned int *ip = ( unsigned int *)u; 00149 rprintf(" 0x%08x\r\n", *ip); 00150 u+= 4; 00151 len -= 4; 00152 cnt += 4; 00153 continue; 00154 } 00155 cnt += width; 00156 j = len < width ? len : width; 00157 for (i = 0; i < j; i++) { 00158 rprintf("%2.2x ", *(u + i)); 00159 if (i == seppos) 00160 rprintf(" "); 00161 } 00162 rprintf(" "); 00163 if (j < width) { 00164 i = width - j; 00165 if (i > seppos + 1) 00166 rprintf(" "); 00167 while (i--) { 00168 rprintf("%s", " "); 00169 } 00170 } 00171 for (i = 0; i < j; i++) { 00172 int c = *(u + i); 00173 if (c >= ' ' && c <= '~') 00174 rprintf("%c", c); 00175 else 00176 rprintf("."); 00177 if (i == seppos) 00178 rprintf(" "); 00179 } 00180 len -= width; 00181 u += width; 00182 rprintf("\r\n"); 00183 } 00184 rprintf("--\r\n"); 00185 } 00186 00187 /* 00188 * Convert compile time to system time 00189 */ 00190 time_t 00191 cvt_date(char const *date, char const *time) 00192 { 00193 char s_month[5]; 00194 int year; 00195 struct tm t; 00196 static const char month_names[] = "JanFebMarAprMayJunJulAugSepOctNovDec"; 00197 sscanf(date, "%s %d %d", s_month, &t.tm_mday, &year); 00198 sscanf(time, "%2d %*c %2d %*c %2d", &t.tm_hour, &t.tm_min, &t.tm_sec); 00199 // Find where is s_month in month_names. Deduce month value. 00200 t.tm_mon = (strstr(month_names, s_month) - month_names) / 3; 00201 t.tm_year = year - 1900; 00202 return (int)mktime(&t); 00203 }
Generated on Thu Jul 14 2022 21:27:01 by
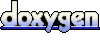