
Smartage application
Dependencies: BufferedSerial SX1276GenericLib USBDeviceHT mbed Crypto X_NUCLEO_IKS01A2
Fork of STM32L0_LoRa by
smartage.cpp
00001 #include "mbed.h" 00002 #include "PinMap.h" 00003 #include "smartage.h" 00004 #include "sx1276-mbed-hal.h" 00005 #include "main.h" 00006 #include "Crypto.h" 00007 00008 #ifdef FEATURE_LORA 00009 00010 /* Set this flag to '1' to display debug messages on the console */ 00011 #define DEBUG_MESSAGE 1 00012 00013 #define USE_MODEM_LORA 1 00014 #define RF_FREQUENCY RF_FREQUENCY_868_1 // Hz 00015 #define TX_OUTPUT_POWER 14 // 14 dBm 00016 00017 #if USE_MODEM_LORA == 1 00018 00019 #define LORA_BANDWIDTH 125000 // LoRa default, details in SX1276::BandwidthMap 00020 #define LORA_SPREADING_FACTOR LORA_SF7 00021 #define LORA_CODINGRATE LORA_ERROR_CODING_RATE_4_5 00022 00023 #define LORA_PREAMBLE_LENGTH 8 // Same for Tx and Rx 00024 #define LORA_SYMBOL_TIMEOUT 5 // Symbols 00025 #define LORA_FIX_LENGTH_PAYLOAD_ON false 00026 #define LORA_FHSS_ENABLED false 00027 #define LORA_NB_SYMB_HOP 4 00028 #define LORA_IQ_INVERSION_ON false 00029 #define LORA_CRC_ENABLED true 00030 00031 #else 00032 #error "Please define a modem in the compiler options." 00033 #endif 00034 00035 #define WHILE_QUANTITY 5 00036 #define RX_TIMEOUT_VALUE 3500 // in ms 00037 00038 //#define BUFFER_SIZE 32 // Define the payload size here 00039 #define BUFFER_SIZE 64 // Define the payload size here 00040 00041 /* 00042 * Global variables declarations 00043 */ 00044 typedef enum 00045 { 00046 LOWPOWER = 0, 00047 IDLE, 00048 RX, 00049 TX, 00050 TX_TIMEOUT, 00051 DO_TX, 00052 CAD, 00053 CAD_DONE 00054 } AppStates_t; 00055 00056 volatile AppStates_t State = LOWPOWER; 00057 00058 /*! 00059 * Radio events function pointer 00060 */ 00061 static RadioEvents_t RadioEvents; 00062 00063 /* 00064 * Global variables declarations 00065 */ 00066 SX1276Generic *Radio; 00067 00068 uint16_t BufferSize = BUFFER_SIZE; 00069 uint8_t *Buffer; 00070 unsigned char myKEY[16] = {0x10, 0x11, 0x12, 0x13, 0x14, 0x15, 0x16, 0x17, 0x18, 0x19, 0x1A, 0x1B, 0x1C, 0x1D, 0x1E, 0x1F,}; 00071 unsigned char myIV[16] = { 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08, 0x09, 0x0A, 0x0B, 0x0C, 0x0D, 0x0E, 0x0F, } ; 00072 const uint8_t ack[] = { 0xff, 0xff, 0x00, 0x00, 'A', 'C', 'K', '!'}; 00073 00074 DigitalOut *led3; 00075 void print_stuff(){ 00076 dprintf("Smartage Application" ); 00077 dprintf("Freqency: %.1f", (double)RF_FREQUENCY/1000000.0); 00078 dprintf("TXPower: %d dBm", TX_OUTPUT_POWER); 00079 #if USE_MODEM_LORA == 1 00080 dprintf("Bandwidth: %d Hz", LORA_BANDWIDTH); 00081 dprintf("Spreading factor: SF%d", LORA_SPREADING_FACTOR); 00082 #elif USE_MODEM_FSK == 1 00083 dprintf("Bandwidth: %d kHz", FSK_BANDWIDTH); 00084 dprintf("Baudrate: %d", FSK_DATARATE); 00085 #endif 00086 } 00087 00088 void SendAndBack(uint8_t* str, uint8_t* empty_distance, uint8_t* temperature, bool tilt_status) 00089 { 00090 #if defined(TARGET_DISCO_L072CZ_LRWAN1) 00091 DigitalOut *led = new DigitalOut(LED4); // RX red 00092 led3 = new DigitalOut(LED3); // TX blue 00093 #else 00094 DigitalOut *led = new DigitalOut(LED1); 00095 led3 = led; 00096 #endif 00097 00098 Buffer = new uint8_t[BUFFER_SIZE]; 00099 *led3 = 1; 00100 00101 #ifdef B_L072Z_LRWAN1_LORA 00102 Radio = new SX1276Generic(NULL, MURATA_SX1276, 00103 LORA_SPI_MOSI, LORA_SPI_MISO, LORA_SPI_SCLK, LORA_CS, LORA_RESET, 00104 LORA_DIO0, LORA_DIO1, LORA_DIO2, LORA_DIO3, LORA_DIO4, LORA_DIO5, 00105 LORA_ANT_RX, LORA_ANT_TX, LORA_ANT_BOOST, LORA_TCXO); 00106 #endif 00107 uint8_t i; 00108 // Initialize Radio driver 00109 RadioEvents.TxDone = OnTxDone; 00110 RadioEvents.RxDone = OnRxDone; 00111 RadioEvents.RxError = OnRxError; 00112 RadioEvents.TxTimeout = OnTxTimeout; 00113 RadioEvents.RxTimeout = OnRxTimeout; 00114 if (Radio->Init( &RadioEvents ) == false) { 00115 while(1) { 00116 dprintf("Radio could not be detected!"); 00117 wait( 1 ); 00118 } 00119 } 00120 00121 00122 switch(Radio->DetectBoardType()) { 00123 case MURATA_SX1276: 00124 if (DEBUG_MESSAGE) 00125 dprintf(" > Board Type: MURATA_SX1276_STM32L0 <"); 00126 break; 00127 default: 00128 dprintf(" > Board Type: unknown <"); 00129 } 00130 00131 Radio->SetChannel(RF_FREQUENCY ); 00132 00133 #if USE_MODEM_LORA == 1 00134 00135 if (LORA_FHSS_ENABLED) 00136 dprintf(" > LORA FHSS Mode <"); 00137 if (!LORA_FHSS_ENABLED) 00138 dprintf(" > LORA Mode <"); 00139 00140 Radio->SetTxConfig( MODEM_LORA, TX_OUTPUT_POWER, 0, LORA_BANDWIDTH, 00141 LORA_SPREADING_FACTOR, LORA_CODINGRATE, 00142 LORA_PREAMBLE_LENGTH, LORA_FIX_LENGTH_PAYLOAD_ON, 00143 LORA_CRC_ENABLED, LORA_FHSS_ENABLED, LORA_NB_SYMB_HOP, 00144 LORA_IQ_INVERSION_ON, 2000 ); 00145 00146 Radio->SetRxConfig( MODEM_LORA, LORA_BANDWIDTH, LORA_SPREADING_FACTOR, 00147 LORA_CODINGRATE, 0, LORA_PREAMBLE_LENGTH, 00148 LORA_SYMBOL_TIMEOUT, LORA_FIX_LENGTH_PAYLOAD_ON, 0, 00149 LORA_CRC_ENABLED, LORA_FHSS_ENABLED, LORA_NB_SYMB_HOP, 00150 LORA_IQ_INVERSION_ON, true ); 00151 00152 #else 00153 00154 #error "Please define a modem in the compiler options." 00155 00156 #endif 00157 00158 if (DEBUG_MESSAGE) 00159 dprintf("Sending the message ... "); 00160 00161 00162 Radio->Rx( RX_TIMEOUT_VALUE ); //CHIAMERà il timeout rx da cui poi chiamo DO_TX case. 00163 00164 int trasmission_routine = 0; 00165 AES myAES(AES_128, myKEY, myIV); 00166 while (trasmission_routine<=WHILE_QUANTITY){ 00167 switch( State ) 00168 { 00169 case RX: 00170 //SE RICEVO HO GIà STAMPATO IL MESSAGGIO QUINDI DEVO SOLO USCIRE 00171 *led3 = 0; 00172 00173 if( BufferSize > 0 ) 00174 { 00175 //setto trasmission_routine a un valore maggiore di quello necessario per rimanere nel ciclo 00176 trasmission_routine = WHILE_QUANTITY+1; 00177 } 00178 State = LOWPOWER; 00179 break; 00180 case DO_TX: 00181 *led3 = 1; 00182 00183 // We fill the buffer with numbers for the payload 00184 Buffer[4]='G'; 00185 Buffer[5]='C'; 00186 Buffer[6]='-'; 00187 Buffer[7]='1'; 00188 i += 4; 00189 // Then it follows the distance 00190 memcpy(Buffer, str, sizeof(str)); 00191 i += 4; 00192 // Then it follows the empty distance 00193 memcpy(Buffer+8, empty_distance, sizeof(empty_distance)); 00194 i+= 4; 00195 // Then temperature ... 00196 memcpy(Buffer+12, temperature, sizeof(temperature)); 00197 i +=4; 00198 00199 //Finally, tilt status 00200 if (tilt_status){ 00201 Buffer[16] = 'T'; 00202 Buffer[17] = 'I'; 00203 Buffer[18] = 'L'; 00204 Buffer[19] = 'T'; 00205 00206 } 00207 else{ 00208 Buffer[16] = 'F'; 00209 Buffer[17] = 'I'; 00210 Buffer[18] = 'N'; 00211 Buffer[19] = 'E'; 00212 00213 } 00214 i += 4; 00215 00216 for( i; i < BufferSize; i++ ) 00217 { 00218 Buffer[i] = i - sizeof(str)+4; 00219 } 00220 dump("Check: ", Buffer, BufferSize); 00221 myAES.encrypt(Buffer, Buffer, BufferSize); 00222 dump("Crypto: ", Buffer, BufferSize); 00223 wait_ms( 10 ); 00224 Radio->Send( Buffer, BufferSize ); 00225 trasmission_routine += 1; 00226 State = LOWPOWER; 00227 break; 00228 case TX: 00229 Radio->Rx( RX_TIMEOUT_VALUE ); 00230 State = LOWPOWER; 00231 break; 00232 case TX_TIMEOUT: 00233 Radio->Rx( RX_TIMEOUT_VALUE ); 00234 State = LOWPOWER; 00235 break; 00236 case LOWPOWER: 00237 sleep(); 00238 break; 00239 default: 00240 State = LOWPOWER; 00241 break; 00242 } 00243 } 00244 dprintf("> Finished!"); 00245 //wait for a bit - in seconds. 00246 wait(10.0f); 00247 //destroy led led3 e Buffer e radio 00248 delete(led); 00249 delete(led3); 00250 delete(Buffer); 00251 delete(Radio); 00252 } 00253 00254 void OnTxDone(void *radio, void *userThisPtr, void *userData) 00255 { 00256 Radio->Sleep( ); 00257 State = TX; 00258 if (DEBUG_MESSAGE) 00259 dprintf("> OnTxDone"); 00260 } 00261 00262 void OnRxDone(void *radio, void *userThisPtr, void *userData, uint8_t *payload, uint16_t size, int16_t rssi, int8_t snr) 00263 { 00264 if(memcmp(payload, ack, 8) == 0) { 00265 Radio->Sleep( ); 00266 BufferSize = size; 00267 memcpy( Buffer, payload, BufferSize ); 00268 State = RX; 00269 if (DEBUG_MESSAGE) 00270 dprintf("> OnRxDone: RssiValue=%d dBm, SnrValue=%d", rssi, snr); 00271 //dump("Data:", payload, size); 00272 } 00273 } 00274 00275 void OnTxTimeout(void *radio, void *userThisPtr, void *userData) 00276 { 00277 *led3 = 0; 00278 Radio->Sleep( ); 00279 State = TX_TIMEOUT; 00280 if(DEBUG_MESSAGE) 00281 dprintf("> OnTxTimeout"); 00282 } 00283 00284 void OnRxTimeout(void *radio, void *userThisPtr, void *userData) 00285 { 00286 *led3 = 0; 00287 Radio->Sleep( ); 00288 Buffer[BufferSize-1] = 0; 00289 State = DO_TX; 00290 Radio->Rx( RX_TIMEOUT_VALUE ); 00291 if (DEBUG_MESSAGE) 00292 dprintf("> OnRxTimeout"); 00293 } 00294 00295 void OnRxError(void *radio, void *userThisPtr, void *userData) 00296 { 00297 Radio->Sleep( ); 00298 State = DO_TX; 00299 if (DEBUG_MESSAGE) 00300 dprintf("> OnRxError"); 00301 } 00302 00303 #endif
Generated on Thu Jul 14 2022 21:27:01 by
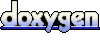