
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
wifi_scan.cpp
00001 /* 00002 * Copyright (c) 2017, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 #include "mbed.h" 00019 #include "greentea-client/test_env.h" 00020 #include "unity.h" 00021 #include "utest.h" 00022 #include "wifi_tests.h" 00023 #include <stdbool.h> 00024 00025 using namespace utest::v1; 00026 00027 void wifi_scan(void) 00028 { 00029 WiFiInterface *wifi = get_interface(); 00030 00031 WiFiAccessPoint ap[MBED_CONF_APP_MAX_SCAN_SIZE]; 00032 00033 int size = wifi->scan(ap, MBED_CONF_APP_MAX_SCAN_SIZE); 00034 TEST_ASSERT(size >= 2); 00035 00036 bool secure_found = false; 00037 bool unsecure_found = false; 00038 00039 char secure_bssid[6]; 00040 char unsecure_bssid[6]; 00041 const char *coversion_string = "%hhx:%hhx:%hhx:%hhx:%hhx:%hhx"; 00042 TEST_ASSERT_EQUAL_INT_MESSAGE(6, sscanf(MBED_CONF_APP_AP_MAC_SECURE, coversion_string, &secure_bssid[0], &secure_bssid[1], &secure_bssid[2], &secure_bssid[3], &secure_bssid[4], &secure_bssid[5]), "Failed to convert ap-mac-secure from mbed_app.json"); 00043 TEST_ASSERT_EQUAL_INT_MESSAGE(6, sscanf(MBED_CONF_APP_AP_MAC_UNSECURE, coversion_string, &unsecure_bssid[0], &unsecure_bssid[1], &unsecure_bssid[2], &unsecure_bssid[3], &unsecure_bssid[4], &unsecure_bssid[5]), "Failed to convert ap-mac-unsecure from mbed_app.json"); 00044 00045 for (int i=0; i<size; i++) { 00046 const char *ssid = ap[i].get_ssid(); 00047 const uint8_t *bssid = ap[i].get_bssid(); 00048 nsapi_security_t security = ap[i].get_security(); 00049 int8_t rssi = ap[i].get_rssi(); 00050 uint8_t ch = ap[i].get_channel(); 00051 TEST_ASSERT_NOT_EQUAL(0, strlen(ssid)); 00052 TEST_ASSERT_INT8_WITHIN(-35, -65, rssi); 00053 if (strcmp(MBED_CONF_APP_WIFI_SECURE_SSID, ssid) == 0) { 00054 secure_found = true; 00055 TEST_ASSERT_EQUAL_INT(NSAPI_SECURITY_WPA2 , security); 00056 TEST_ASSERT_EQUAL_INT(MBED_CONF_APP_WIFI_CH_SECURE, ch); 00057 } 00058 if (strcmp(MBED_CONF_APP_WIFI_UNSECURE_SSID, ssid) == 0) { 00059 unsecure_found = true; 00060 TEST_ASSERT_EQUAL_INT(NSAPI_SECURITY_NONE , security); 00061 TEST_ASSERT_EQUAL_INT(MBED_CONF_APP_WIFI_CH_UNSECURE, ch); 00062 } 00063 } 00064 TEST_ASSERT_TRUE(secure_found); 00065 TEST_ASSERT_TRUE(unsecure_found); 00066 } 00067
Generated on Sun Jul 17 2022 08:25:33 by
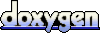