
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
whiteboard_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2011-2017 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 #ifndef WHITEBOARD_API_H_ 00016 #define WHITEBOARD_API_H_ 00017 00018 #include "net_interface.h" 00019 00020 /** 00021 * \file whiteboard_api.h 00022 * \brief An API for DAD, intercepting messages from backhaul network and keeping track of routes inside the PAN. 00023 */ 00024 00025 #ifdef __cplusplus 00026 extern "C" { 00027 #endif 00028 00029 /** 00030 * \struct whiteboard_entry_t 00031 * \brief A structure describing a whiteboarding entry. 00032 */ 00033 typedef struct whiteboard_entry_t { 00034 uint8_t address[16]; /**< Registered IP address. */ 00035 uint_least24_t ttl; /**< Whiteboard entry TTL (seconds). */ 00036 uint8_t eui64[8]; /**< EUI-64 of node that IP address is registered to. */ 00037 int8_t interface_index; /**< Interface id */ 00038 } whiteboard_entry_t; 00039 00040 /** 00041 * \brief Read whiteboard entries 00042 * \param cur NULL to read first entry, or get next entry after cur. 00043 * \return A pointer to whiteboard_entry_t structure. 00044 */ 00045 extern whiteboard_entry_t *whiteboard_get(whiteboard_entry_t *cur); 00046 00047 /** 00048 * @brief Whiteboard_set_device_hard_limit Sets the absolut limit of child devices this device can handle. 00049 * This is very useful in situations, where 1 GW drops from network and causes it's children 00050 * to join other GW networks. This might cause other GWs to run out of memory. 00051 * @param limit Absolute maximum amount of devices allowed to join. Default value=0 means unlimited (as long as there is memory) 00052 */ 00053 extern void whiteboard_set_device_hard_limit(uint16_t limit); 00054 00055 #ifdef __cplusplus 00056 } 00057 #endif 00058 #endif /* WHITEBOARD_API_H_ */
Generated on Sun Jul 17 2022 08:25:33 by
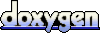