
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
vmpu_exports.h
00001 /* 00002 * Copyright (c) 2013-2016, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #ifndef __UVISOR_API_VMPU_EXPORTS_H__ 00018 #define __UVISOR_API_VMPU_EXPORTS_H__ 00019 00020 #include "api/inc/uvisor_exports.h" 00021 #include <stdint.h> 00022 00023 /* The maximum box namespace length is 37 so that it is exactly big enough for 00024 * a human-readable hex string GUID (as formatted by RFC 4122) followed by a 00025 * terminating NULL. */ 00026 #define UVISOR_MAX_BOX_NAMESPACE_LENGTH 37 00027 00028 /** Invalid box id for use in marking objects with invalid ownership. */ 00029 #define UVISOR_BOX_ID_INVALID ((uint8_t) -1) 00030 00031 /* supervisor user access modes */ 00032 #define UVISOR_TACL_UEXECUTE 0x0001UL 00033 #define UVISOR_TACL_UWRITE 0x0002UL 00034 #define UVISOR_TACL_UREAD 0x0004UL 00035 #define UVISOR_TACL_UACL (UVISOR_TACL_UREAD |\ 00036 UVISOR_TACL_UWRITE |\ 00037 UVISOR_TACL_UEXECUTE) 00038 00039 /* supervisor access modes */ 00040 #define UVISOR_TACL_SEXECUTE 0x0008UL 00041 #define UVISOR_TACL_SWRITE 0x0010UL 00042 #define UVISOR_TACL_SREAD 0x0020UL 00043 #define UVISOR_TACL_SACL (UVISOR_TACL_SREAD |\ 00044 UVISOR_TACL_SWRITE |\ 00045 UVISOR_TACL_SEXECUTE) 00046 00047 #define UVISOR_TACL_EXECUTE (UVISOR_TACL_UEXECUTE |\ 00048 UVISOR_TACL_SEXECUTE) 00049 00050 /* all possible access control flags */ 00051 #define UVISOR_TACL_ACCESS (UVISOR_TACL_UACL |\ 00052 UVISOR_TACL_SACL) 00053 00054 /* various modes */ 00055 #define UVISOR_TACL_STACK 0x0040UL 00056 #define UVISOR_TACL_SIZE_ROUND_UP 0x0080UL 00057 #define UVISOR_TACL_SIZE_ROUND_DOWN 0x0100UL 00058 #define UVISOR_TACL_PERIPHERAL 0x0200UL 00059 #define UVISOR_TACL_SHARED 0x0400UL 00060 #define UVISOR_TACL_USER 0x0800UL 00061 #define UVISOR_TACL_IRQ 0x1000UL 00062 00063 #if defined(UVISOR_PRESENT) && UVISOR_PRESENT == 1 00064 00065 #endif /* defined(UVISOR_PRESENT) && UVISOR_PRESENT == 1 */ 00066 00067 #define UVISOR_TACLDEF_SECURE_BSS (UVISOR_TACL_UREAD |\ 00068 UVISOR_TACL_UWRITE |\ 00069 UVISOR_TACL_SREAD |\ 00070 UVISOR_TACL_SWRITE |\ 00071 UVISOR_TACL_SIZE_ROUND_UP) 00072 00073 #define UVISOR_TACLDEF_SECURE_CONST (UVISOR_TACL_UREAD |\ 00074 UVISOR_TACL_SREAD |\ 00075 UVISOR_TACL_SIZE_ROUND_UP) 00076 00077 #define UVISOR_TACLDEF_DATA UVISOR_TACLDEF_SECURE_BSS 00078 00079 #define UVISOR_TACLDEF_PERIPH (UVISOR_TACL_PERIPHERAL |\ 00080 UVISOR_TACL_UREAD |\ 00081 UVISOR_TACL_UWRITE |\ 00082 UVISOR_TACL_SREAD |\ 00083 UVISOR_TACL_SWRITE |\ 00084 UVISOR_TACL_SIZE_ROUND_UP) 00085 00086 #define UVISOR_TACLDEF_STACK (UVISOR_TACL_STACK |\ 00087 UVISOR_TACL_UREAD |\ 00088 UVISOR_TACL_UWRITE |\ 00089 UVISOR_TACL_SREAD |\ 00090 UVISOR_TACL_SWRITE) 00091 00092 #define UVISOR_PAD32(x) (32 - (sizeof(x) & ~0x1FUL)) 00093 #define UVISOR_BOX_MAGIC 0x42CFB66FUL 00094 #define UVISOR_BOX_VERSION 100 00095 #define UVISOR_STACK_BAND_SIZE 128 00096 #define UVISOR_MEM_SIZE_ROUND(x) UVISOR_REGION_ROUND_UP(x) 00097 00098 #define UVISOR_MIN_STACK_SIZE 1024 00099 #define UVISOR_MIN_STACK(x) (((x) < UVISOR_MIN_STACK_SIZE) ? UVISOR_MIN_STACK_SIZE : (x)) 00100 00101 #if defined(UVISOR_PRESENT) && UVISOR_PRESENT == 1 00102 00103 /** Round an address down to the closest 32-byte boundary. 00104 * @param address[in] The address to round. 00105 */ 00106 #define UVISOR_ROUND32_DOWN(address) ((address) & ~0x1FUL) 00107 00108 /** Round an address up to the closest 32-byte boundary. 00109 * @param address[in] The address to round. 00110 */ 00111 #define UVISOR_ROUND32_UP(address) UVISOR_ROUND32_DOWN((address) + 31UL) 00112 00113 00114 #if defined(ARCH_MPU_ARMv7M) 00115 #define UVISOR_REGION_ROUND_DOWN(x) ((x) & ~((1UL << UVISOR_REGION_BITS(x)) - 1)) 00116 #define UVISOR_REGION_ROUND_UP(x) (1UL << UVISOR_REGION_BITS(x)) 00117 #define UVISOR_STACK_SIZE_ROUND(x) UVISOR_REGION_ROUND_UP(x) 00118 #elif defined(ARCH_MPU_ARMv8M) || defined(ARCH_MPU_KINETIS) 00119 #define UVISOR_REGION_ROUND_DOWN(x) UVISOR_ROUND32_DOWN(x) 00120 #define UVISOR_REGION_ROUND_UP(x) UVISOR_ROUND32_UP(x) 00121 #define UVISOR_STACK_SIZE_ROUND(x) UVISOR_REGION_ROUND_UP((x) + (UVISOR_STACK_BAND_SIZE * 2)) 00122 #else 00123 #error "Unknown MPU architecture. uvisor: Check your Makefile. uvisor-lib: Check if uVisor is supported" 00124 #endif 00125 00126 #else /* defined(UVISOR_PRESENT) && UVISOR_PRESENT == 1 */ 00127 00128 #define UVISOR_REGION_ROUND_DOWN(x) (x) 00129 #define UVISOR_REGION_ROUND_UP(x) (x) 00130 #define UVISOR_STACK_SIZE_ROUND(x) (x) 00131 00132 #endif 00133 00134 #ifndef UVISOR_BOX_STACK_SIZE 00135 #define UVISOR_BOX_STACK_SIZE UVISOR_MIN_STACK_SIZE 00136 #endif/*UVISOR_BOX_STACK*/ 00137 00138 /* NOPs added for write buffering synchronization (2 are for dsb. 16bits) */ 00139 #define UVISOR_NOP_CNT (2 + 3) 00140 #define UVISOR_NOP_GROUP \ 00141 "dsb\n" \ 00142 "nop\n" \ 00143 "nop\n" \ 00144 "nop\n" 00145 00146 typedef uint32_t UvisorBoxAcl; 00147 00148 typedef struct { 00149 void * param1; 00150 uint32_t param2; 00151 UvisorBoxAcl acl; 00152 } UVISOR_PACKED UvisorBoxAclItem; 00153 00154 /* This struct contains all the BSS sections that uVisor allocates for a secure 00155 * box. It can be used to keep the sizes of the sections or their pointers. */ 00156 typedef struct uvisor_bss_sections_t { 00157 uint32_t index; 00158 uint32_t context; 00159 uint32_t newlib_reent; 00160 uint32_t rpc; 00161 uint32_t ipc; 00162 uint32_t heap; 00163 } UVISOR_PACKED UvisorBssSections; 00164 00165 /* The number of per-box BSS sections. */ 00166 #define UVISOR_BSS_SECTIONS_COUNT (sizeof(UvisorBssSections) / sizeof(uint32_t)) 00167 00168 /* Compile-time per-box configuration table 00169 * Each box has one of this table in flash. Every other data structure that this 00170 * table might point to must be in flash as well. The uVisor core must check the 00171 * sanity of the table before trusting its fields. */ 00172 typedef struct { 00173 const uint32_t magic; 00174 const uint32_t version; 00175 00176 /* The UvisorBssSections struct is union-ed with a size_t array to allow for 00177 * loops to scan the sizes of all the BSS sections and allocate the 00178 * necessary space for each of them. */ 00179 union { 00180 size_t sizes[UVISOR_BSS_SECTIONS_COUNT]; 00181 UvisorBssSections size_of; 00182 } const bss; 00183 00184 /* Contains the size of the secure box static stack. */ 00185 /* Note: This does not include guards. */ 00186 /* Note: It is kept separately from the BSS sections as it's implementation 00187 * specific where the stack sits with respect to the BSS. */ 00188 const uint32_t stack_size; 00189 00190 /* Opaque-to-uVisor data that potentially contains uvisor-lib-specific or 00191 * OS-specific per-box configuration */ 00192 const void * const lib_config; 00193 00194 const char * const box_namespace; 00195 const UvisorBoxAclItem * const acl_list; 00196 const uint32_t acl_count; 00197 } UVISOR_PACKED UvisorBoxConfig; 00198 00199 /* Enumeration-time per-box index table 00200 * Each box has one of this table in SRAM. The index tables are initialized at 00201 * box enumeration time and are then managed by the secure boxes themselves. */ 00202 /* Note: Each box is able to read and write its own version of this table. Do 00203 * not trust these pointers in the uVisor core. */ 00204 typedef struct { 00205 /* The UvisorSramPointers struct is union-ed with a void * array to allow 00206 * for loops to scan the pointers to all the SRAM sections and access them 00207 * individually. */ 00208 union { 00209 void * pointers[UVISOR_BSS_SECTIONS_COUNT]; 00210 UvisorBssSections address_of; 00211 } bss; 00212 00213 /* Size of the box heap */ 00214 uint32_t box_heap_size; 00215 /* Pointer to the currently active heap. 00216 * This is set to `NULL` by uVisor, signalling to the user lib that the 00217 * box heap needs to be initialized before use! */ 00218 void * active_heap; 00219 00220 /* Box ID */ 00221 int box_id_self; 00222 00223 /* Pointer to the box config */ 00224 const UvisorBoxConfig * config; 00225 } UVISOR_PACKED UvisorBoxIndex; 00226 00227 /* 00228 * only use this macro for rounding const values during compile time: 00229 * for variables please use uvisor_region_bits(x) instead 00230 */ 00231 #define UVISOR_REGION_BITS(x) (((x)<=32UL)?5:(((x)<=64UL)?\ 00232 6:(((x)<=128UL)?7:(((x)<=256UL)?8:(((x)<=512UL)?9:(((x)<=1024UL)?\ 00233 10:(((x)<=2048UL)?11:(((x)<=4096UL)?12:(((x)<=8192UL)?\ 00234 13:(((x)<=16384UL)?14:(((x)<=32768UL)?15:(((x)<=65536UL)?\ 00235 16:(((x)<=131072UL)?17:(((x)<=262144UL)?18:(((x)<=524288UL)?\ 00236 19:(((x)<=1048576UL)?20:(((x)<=2097152UL)?21:(((x)<=4194304UL)?\ 00237 22:(((x)<=8388608UL)?23:(((x)<=16777216UL)?24:(((x)<=33554432UL)?\ 00238 25:(((x)<=67108864UL)?26:(((x)<=134217728UL)?27:(((x)<=268435456UL)?\ 00239 28:(((x)<=536870912UL)?29:(((x)<=1073741824UL)?30:(((x)<=2147483648UL)?\ 00240 31:32))))))))))))))))))))))))))) 00241 00242 #if defined(UVISOR_PRESENT) && UVISOR_PRESENT == 1 00243 static UVISOR_FORCEINLINE int vmpu_bits(uint32_t size) 00244 { 00245 /* If size is 0, the result of __builtin_clz is undefined */ 00246 return (0 == size) ? 0: 32 - __builtin_clz(size); 00247 } 00248 #endif /* defined(UVISOR_PRESENT) && UVISOR_PRESENT == 1 */ 00249 00250 #endif /* __UVISOR_API_VMPU_EXPORTS_H__ */
Generated on Sun Jul 17 2022 08:25:33 by
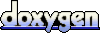