
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
uvisor_spinlock_exports.h
00001 /* 00002 * Copyright (c) 2016, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #ifndef __UVISOR_API_UVISOR_SPINLOCK_H__ 00018 #define __UVISOR_API_UVISOR_SPINLOCK_H__ 00019 00020 #include "api/inc/uvisor_exports.h" 00021 #include <stdbool.h> 00022 00023 typedef struct { 00024 bool acquired; 00025 } UvisorSpinlock; 00026 00027 /* This function is safe to call from interrupt context. */ 00028 UVISOR_EXTERN void uvisor_spin_init(UvisorSpinlock * spinlock); 00029 00030 /* Attempt to spin lock once. Return true if the lock was obtained, false if 00031 * otherwise. This function is safe to call from interrupt context. */ 00032 UVISOR_EXTERN bool uvisor_spin_trylock(UvisorSpinlock * spinlock); 00033 00034 /* Spin in a tight loop until the lock is obtained. This function is safe to 00035 * call from interrupt context, but probably not wise. */ 00036 UVISOR_EXTERN void uvisor_spin_lock(UvisorSpinlock * spinlock); 00037 00038 /* Unlock the spin lock. This function is safe to call from interrupt context. 00039 * */ 00040 UVISOR_EXTERN void uvisor_spin_unlock(UvisorSpinlock * spinlock); 00041 00042 #endif /* __UVISOR_API_UVISOR_SPINLOCK_H__ */
Generated on Sun Jul 17 2022 08:25:33 by
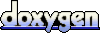