
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
utest_types.cpp
00001 /**************************************************************************** 00002 * Copyright (c) 2015, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 **************************************************************************** 00017 */ 00018 00019 #include "utest/utest_types.h" 00020 00021 const char* utest::v1::stringify(utest::v1::failure_reason_t reason) 00022 { 00023 const char *string; 00024 switch(reason & ~REASON_IGNORE) 00025 { 00026 case REASON_NONE: 00027 string = "Ignored: No Failure"; 00028 break; 00029 case REASON_CASES: 00030 string = "Ignored: Test Cases Failed"; 00031 break; 00032 case REASON_EMPTY_CASE: 00033 string = "Ignored: Test Case is Empty"; 00034 break; 00035 case REASON_TIMEOUT: 00036 string = "Ignored: Timed Out"; 00037 break; 00038 case REASON_ASSERTION: 00039 string = "Ignored: Assertion Failed"; 00040 break; 00041 case REASON_TEST_SETUP: 00042 string = "Ignored: Test Setup Failed"; 00043 break; 00044 case REASON_TEST_TEARDOWN: 00045 string = "Ignored: Test Teardown Failed"; 00046 break; 00047 case REASON_CASE_SETUP: 00048 string = "Ignored: Case Setup Failed"; 00049 break; 00050 case REASON_CASE_HANDLER: 00051 string = "Ignored: Case Handler Failed"; 00052 break; 00053 case REASON_CASE_TEARDOWN: 00054 string = "Ignored: Case Teardown Failed"; 00055 break; 00056 case REASON_CASE_INDEX: 00057 string = "Ignored: Case Index Invalid"; 00058 break; 00059 case REASON_SCHEDULER: 00060 string = "Ignored: Scheduling Asynchronous Callback Failed"; 00061 break; 00062 default: 00063 case REASON_UNKNOWN: 00064 string = "Ignored: Unknown Failure"; 00065 break; 00066 } 00067 if (!(reason & REASON_IGNORE)) string += 9; 00068 return string; 00069 } 00070 00071 const char* utest::v1::stringify(utest::v1::failure_t failure) 00072 { 00073 return stringify(failure.reason); 00074 } 00075 00076 const char* utest::v1::stringify(utest::v1::location_t location) 00077 { 00078 const char *string; 00079 switch(location) 00080 { 00081 case LOCATION_TEST_SETUP: 00082 string = "Test Setup Handler"; 00083 break; 00084 case LOCATION_TEST_TEARDOWN: 00085 string = "Test Teardown Handler"; 00086 break; 00087 case LOCATION_CASE_SETUP: 00088 string = "Case Setup Handler"; 00089 break; 00090 case LOCATION_CASE_HANDLER: 00091 string = "Case Handler"; 00092 break; 00093 case LOCATION_CASE_TEARDOWN: 00094 string = "Case Teardown Handler"; 00095 break; 00096 default: 00097 case LOCATION_UNKNOWN: 00098 string = "Unknown Location"; 00099 break; 00100 } 00101 return string; 00102 } 00103 00104 const char* utest::v1::stringify(utest::v1::status_t status) 00105 { 00106 switch(status) 00107 { 00108 case STATUS_CONTINUE: 00109 return "Continue"; 00110 case STATUS_IGNORE: 00111 return "Ignore"; 00112 case STATUS_ABORT: 00113 return "Abort"; 00114 } 00115 return "Unknown Status"; 00116 } 00117 00118 00119 const utest::v1::base_control_t utest::v1::CaseNext = { REPEAT_NONE, TIMEOUT_NONE }; 00120 00121 const utest::v1::base_control_t utest::v1::CaseNoRepeat = { REPEAT_NONE, TIMEOUT_UNDECLR }; 00122 00123 const utest::v1::base_control_t utest::v1::CaseRepeatAll = { REPEAT_ALL, TIMEOUT_UNDECLR }; 00124 00125 const utest::v1::base_control_t utest::v1::CaseRepeatHandler = { REPEAT_HANDLER, TIMEOUT_UNDECLR }; 00126 00127 const utest::v1::base_control_t utest::v1::CaseNoTimeout = { REPEAT_UNDECLR, TIMEOUT_NONE }; 00128 00129 const utest::v1::base_control_t utest::v1::CaseAwait = { REPEAT_UNDECLR, TIMEOUT_FOREVER }; 00130 00131 // equal to CaeReapeatAll 00132 const utest::v1::base_control_t utest::v1::CaseRepeat = { REPEAT_ALL, TIMEOUT_UNDECLR }; 00133 00134 // equal to CaseRepeatHandler 00135 const utest::v1::base_control_t utest::v1::CaseRepeatHandlerOnly = { REPEAT_HANDLER, TIMEOUT_UNDECLR };
Generated on Sun Jul 17 2022 08:25:33 by
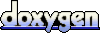