
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
utest_stack_trace.cpp
00001 /**************************************************************************** 00002 * Copyright (c) 2016, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #ifdef UTEST_STACK_TRACE 00018 00019 #include "greentea-client/test_env.h" 00020 #include "mbed.h" 00021 #include "utest/utest.h" 00022 #include "unity/unity.h" 00023 #include "utest/utest_stack_trace.h" 00024 #include "utest/utest_serial.h" 00025 00026 using namespace utest::v1; 00027 00028 std::string utest_trace[UTEST_MAX_BACKTRACE]; 00029 00030 static unsigned trace_index = 0; 00031 static unsigned total_calls = 0; 00032 00033 void utest_trace_initialise() 00034 { 00035 total_calls = 0; 00036 trace_index = 0; 00037 for(unsigned i = 0; i < UTEST_MAX_BACKTRACE; i++) { 00038 utest_trace[i].clear(); 00039 } 00040 } 00041 00042 void utest_add_to_trace(char *func_name) 00043 { 00044 utest_trace[trace_index] = std::string(func_name); 00045 trace_index = (trace_index + 1 == UTEST_MAX_BACKTRACE) ? 0 : trace_index + 1; 00046 total_calls ++; 00047 } 00048 00049 void utest_dump_trace() 00050 { 00051 unsigned current = (trace_index == 0) ? UTEST_MAX_BACKTRACE - 1 : trace_index - 1; 00052 00053 utest_printf("==================================================================\n"); 00054 utest_printf("Utest back trace: Total calls logged = %u.\n", total_calls); 00055 utest_printf("==================================================================\n"); 00056 while (current != trace_index) { 00057 00058 utest_printf("%u > %s\n", current, utest_trace[current].c_str()); 00059 current = (current == 0) ? UTEST_MAX_BACKTRACE - 1 : current - 1; 00060 } 00061 utest_printf("==================================================================\n"); 00062 } 00063 00064 #endif
Generated on Sun Jul 17 2022 08:25:33 by
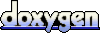