
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
utest_harness.h
00001 /**************************************************************************** 00002 * Copyright (c) 2015, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 **************************************************************************** 00017 */ 00018 00019 #ifndef UTEST_HARNESS_H 00020 #define UTEST_HARNESS_H 00021 00022 #include <stdint.h> 00023 #include <stdbool.h> 00024 #include <stdio.h> 00025 00026 #include "utest/utest_types.h" 00027 #include "utest/utest_case.h" 00028 #include "utest/utest_default_handlers.h" 00029 #include "utest/utest_specification.h" 00030 #include "utest/utest_scheduler.h" 00031 00032 00033 namespace utest { 00034 /** \addtogroup frameworks */ 00035 /** @{*/ 00036 namespace v1 { 00037 00038 /** Test Harness. 00039 * 00040 * This class runs a test specification for you and calls all required handlers. 00041 * The harness executes the test specification in an asynchronous fashion, therefore 00042 * `run()` returns immediately. 00043 * 00044 * By default, this harness uses the MINAR scheduler for asynchronous callbacks. 00045 * If you wamt to provide your own custom scheduler, set `config.utest.use_custom_scheduler` to `true` 00046 * inside your yotta config and set a custom scheduler implementation using the `set_scheduler()` function. 00047 * You must set the scheduler before running a specification. 00048 * 00049 * @note In case of an test abort, the harness will busy-wait and never finish. 00050 */ 00051 class Harness 00052 { 00053 public: 00054 /// Runs a test specification 00055 /// @retval `true` if the specification can be run 00056 /// @retval `false` if another specification is currently running 00057 static bool run(const Specification& specification); 00058 00059 /// @cond 00060 __deprecated_message("Start case selection is done by returning the index from the test setup handler!") 00061 static bool run(const Specification& specification, size_t start_case); 00062 /// @endcond 00063 00064 /// @returns `true` if a test specification is being executed, `false` otherwise 00065 static bool is_busy (); 00066 00067 /// Sets the scheduler to be used. 00068 /// @return `true` if scheduler is properly specified (all functions non-null). 00069 static bool set_scheduler(utest_v1_scheduler_t scheduler); 00070 00071 /** Call this function in the asynchronous callback that you have been waiting for. 00072 * 00073 * You can only validate a callback once, calling this function when no callback is expected 00074 * has no side effects. 00075 * After callback validation, the next test case is scheduled. 00076 * 00077 * You may specify additional test case attributes with this callback. 00078 * So for example, you may delay the decision to repeat an asynchronous test case until the callback 00079 * needs to be validated. 00080 * 00081 * However, be aware, that only the repeat attributes can be modified and the usual arbitration rules apply. 00082 * The modified case attributes are only valid until the case handler returns updated attributes. 00083 * 00084 * @param control the test case attribute to be added to the existing attributes. 00085 */ 00086 static void validate_callback(const control_t control = control_t()); 00087 00088 /// Raising a failure causes the failure to be counted and the failure handler to be called. 00089 /// Further action then depends on its return state. 00090 static void raise_failure(const failure_reason_t reason); 00091 00092 protected: 00093 static void run_next_case(); 00094 static void handle_timeout(); 00095 static void schedule_next_case(); 00096 private: 00097 static void notify_testcases(); 00098 }; 00099 00100 } // namespace v1 00101 } // namespace utest 00102 00103 #endif // UTEST_HARNESS_H 00104 00105 /** @}*/
Generated on Sun Jul 17 2022 08:25:33 by
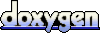