
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
utest_case.h
00001 /**************************************************************************** 00002 * Copyright (c) 2015, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 **************************************************************************** 00017 */ 00018 00019 #ifndef UTEST_CASES_H 00020 #define UTEST_CASES_H 00021 00022 #include <stdint.h> 00023 #include <stdbool.h> 00024 #include <stdio.h> 00025 #include "utest/utest_types.h" 00026 #include "utest/utest_default_handlers.h" 00027 00028 00029 namespace utest { 00030 /** \addtogroup frameworks */ 00031 /** @{*/ 00032 namespace v1 { 00033 /** 00034 * POD data structure of the Case class. 00035 * Unlike the Case class it can be used as a POD and be put in ROM. 00036 * 00037 * @warning Initialization of handlers with either default_handler or 00038 * ignore_handler helpers will prevent the object to be a POD. Prefer usage 00039 * of NULL in favor of ignore_handler or \verbatim <handler_type>(1) \endverbatim for default 00040 * handler. 00041 * 00042 * @see Case. 00043 */ 00044 struct case_t 00045 { 00046 /** 00047 * Textual description of the test case 00048 */ 00049 const char *description; 00050 00051 /** 00052 * Primitive test case handler 00053 * This is called only if the case setup succeeded. It is followed by 00054 * the test case teardown handler. 00055 */ 00056 const case_handler_t handler; 00057 00058 /** 00059 * @see case_control_handler_t 00060 */ 00061 const case_control_handler_t control_handler ; 00062 00063 /** 00064 * @see case_call_count_handler_t 00065 */ 00066 const case_call_count_handler_t repeat_count_handler ; 00067 00068 /** 00069 * Handler called before the execution of the case handler. It sets up 00070 * the case environment. 00071 */ 00072 const case_setup_handler_t setup_handler; 00073 00074 /** 00075 * Handler called after the execution of the case handler. It cleans up 00076 * the case environment. 00077 */ 00078 const case_teardown_handler_t teardown_handler; 00079 00080 /** 00081 * Handler called whenever a faillure occur; at any stage of the case 00082 * execution (including setup and teardown). 00083 */ 00084 const case_failure_handler_t failure_handler; 00085 }; 00086 00087 /** Test case wrapper class. 00088 * 00089 * This class contains the description of the test case and all handlers 00090 * for setting up, running the test case, tearing down and handling failures. 00091 * 00092 * By default you only need to provide a description and a test case handler. 00093 * You may override the setup, teardown and failure handlers, but you do not have to. 00094 * If you do not override these handler, the specified default handlers will be called. 00095 * 00096 * These constructors are overloaded to allow you a comfortable declaration of all your 00097 * callbacks. 00098 * The order is always: 00099 * - description (required) 00100 * - setup handler (optional) 00101 * - test case handler (required) 00102 * - teardown handler (optional) 00103 * - failure handler (optional) 00104 * 00105 * @note While you can specify an empty test case (ie. use `ignore_handler` for all callbacks), 00106 * the harness will abort the test unconditionally. 00107 */ 00108 class Case : private case_t 00109 { 00110 public: 00111 // overloads for case_handler_t 00112 Case(const char *description, 00113 const case_setup_handler_t setup_handler, 00114 const case_handler_t case_handler, 00115 const case_teardown_handler_t teardown_handler = default_handler, 00116 const case_failure_handler_t failure_handler = default_handler); 00117 00118 Case(const char *description, 00119 const case_handler_t case_handler, 00120 const case_failure_handler_t failure_handler = default_handler); 00121 00122 Case(const char *description, 00123 const case_handler_t case_handler, 00124 const case_teardown_handler_t teardown_handler, 00125 const case_failure_handler_t failure_handler = default_handler); 00126 00127 // overloads for case_control_handler_t 00128 Case(const char *description, 00129 const case_setup_handler_t setup_handler, 00130 const case_control_handler_t case_handler, 00131 const case_teardown_handler_t teardown_handler = default_handler, 00132 const case_failure_handler_t failure_handler = default_handler); 00133 00134 Case(const char *description, 00135 const case_control_handler_t case_handler, 00136 const case_failure_handler_t failure_handler = default_handler); 00137 00138 Case(const char *description, 00139 const case_control_handler_t case_handler, 00140 const case_teardown_handler_t teardown_handler, 00141 const case_failure_handler_t failure_handler = default_handler); 00142 00143 // overloads for case_call_count_handler_t 00144 Case(const char *description, 00145 const case_setup_handler_t setup_handler, 00146 const case_call_count_handler_t case_handler, 00147 const case_teardown_handler_t teardown_handler = default_handler, 00148 const case_failure_handler_t failure_handler = default_handler); 00149 00150 Case(const char *description, 00151 const case_call_count_handler_t case_handler, 00152 const case_failure_handler_t failure_handler = default_handler); 00153 00154 Case(const char *description, 00155 const case_call_count_handler_t case_handler, 00156 const case_teardown_handler_t teardown_handler, 00157 const case_failure_handler_t failure_handler = default_handler); 00158 00159 00160 /// @returns the textual description of the test case 00161 const char* get_description () const; 00162 00163 /// @returns `true` if setup, test and teardown handlers are set to `ignore_handler` 00164 bool is_empty () const; 00165 00166 private: 00167 // IMPORTANT: No data members shall be declared inside this class. 00168 // Data members shall be declared in case_t to preserve the layout 00169 // and the compatibility between the two types. 00170 00171 friend class Harness; 00172 friend class Specification; 00173 }; 00174 00175 } // namespace v1 00176 } // namespace utest 00177 00178 #endif // UTEST_CASES_H 00179 00180 /** @}*/
Generated on Sun Jul 17 2022 08:25:33 by
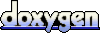