
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
udpecho_client_auto.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2013 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 00018 import sys 00019 import socket 00020 from sys import stdout 00021 from SocketServer import BaseRequestHandler, UDPServer 00022 00023 class UDPEchoClient_Handler(BaseRequestHandler): 00024 def handle(self): 00025 """ One handle per connection 00026 """ 00027 data, socket = self.request 00028 socket.sendto(data, self.client_address) 00029 if '{{end}}' in data: 00030 print 00031 print data 00032 else: 00033 sys.stdout.write('.') 00034 stdout.flush() 00035 00036 class UDPEchoClientTest(): 00037 00038 def send_server_ip_port(self, selftest, ip_address, port_no): 00039 c = selftest.mbed.serial_readline() # 'UDPCllient waiting for server IP and port...' 00040 if c is None: 00041 selftest.print_result(selftest.RESULT_IO_SERIAL) 00042 return 00043 selftest.notify(c.strip()) 00044 00045 selftest.notify("HOST: Sending server IP Address to target...") 00046 connection_str = ip_address + ":" + str(port_no) + "\n" 00047 selftest.mbed.serial_write(connection_str) 00048 00049 c = selftest.mbed.serial_readline() # 'UDPCllient waiting for server IP and port...' 00050 if c is None: 00051 self.print_result(selftest.RESULT_IO_SERIAL) 00052 return 00053 selftest.notify(c.strip()) 00054 return selftest.RESULT_PASSIVE 00055 00056 def test(self, selftest): 00057 # We need to discover SERVEP_IP and set up SERVER_PORT 00058 # Note: Port 7 is Echo Protocol: 00059 # 00060 # Port number rationale: 00061 # 00062 # The Echo Protocol is a service in the Internet Protocol Suite defined 00063 # in RFC 862. It was originally proposed for testing and measurement 00064 # of round-trip times[citation needed] in IP networks. 00065 # 00066 # A host may connect to a server that supports the Echo Protocol using 00067 # the Transmission Control Protocol (TCP) or the User Datagram Protocol 00068 # (UDP) on the well-known port number 7. The server sends back an 00069 # identical copy of the data it received. 00070 SERVER_IP = str(socket.gethostbyname(socket.getfqdn())) 00071 SERVER_PORT = 7 00072 00073 # Returning none will suppress host test from printing success code 00074 server = UDPServer((SERVER_IP, SERVER_PORT), UDPEchoClient_Handler) 00075 print "HOST: Listening for UDP connections..." 00076 self.send_server_ip_port(selftest, SERVER_IP, SERVER_PORT) 00077 server.serve_forever()
Generated on Sun Jul 17 2022 08:25:32 by
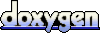