
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
thread_tasklet.h
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __INCLUDE_THREAD_TASKLET__ 00018 #define __INCLUDE_THREAD_TASKLET__ 00019 #include "ns_types.h" 00020 #include "eventOS_event.h" 00021 #include "mbed-mesh-api/mesh_interface_types.h" 00022 00023 #ifdef __cplusplus 00024 extern "C" { 00025 #endif 00026 00027 /** 00028 * Type of the network status callback. 00029 */ 00030 typedef void (*mesh_interface_cb)(mesh_connection_status_t mesh_status); 00031 00032 /* 00033 * \brief Read own global IP address 00034 * 00035 * \param address where own IP address will be written 00036 * \param len length of provided address buffer 00037 * 00038 * \return 0 on success 00039 * \return -1 if address reading fails 00040 */ 00041 int8_t thread_tasklet_get_ip_address(char *address, int8_t len); 00042 00043 /* 00044 * \brief Connect to mesh network 00045 * 00046 * \param callback to be called when network state changes 00047 * \param nwk_interface_id to use for networking 00048 * 00049 * \return >= 0 on success 00050 * \return -1 if callback function is used in another tasklet 00051 * \return -2 if memory allocation fails 00052 * \return -3 if network is already connected 00053 */ 00054 int8_t thread_tasklet_connect(mesh_interface_cb callback, int8_t nwk_interface_id); 00055 00056 /* 00057 * \brief Initialize mesh system. 00058 * Memory pool, timers, traces and support are initialized. 00059 */ 00060 void thread_tasklet_init(void); 00061 00062 /* 00063 * \brief Create network interface. 00064 * 00065 * \param device_id registered physical device 00066 * \return interface ID that can be used to communication with this interface 00067 */ 00068 int8_t thread_tasklet_network_init(int8_t device_id); 00069 00070 /* 00071 * \brief Set device configuration for thread network 00072 * \param eui64 mac address of the registered rf device 00073 * \param pskd private shared key 00074 */ 00075 void thread_tasklet_device_config_set(uint8_t *eui64, char *pskd); 00076 00077 /* 00078 * \brief Disconnect network interface. 00079 * 00080 * \param send_cb send possible network status change event if set to true. 00081 * \return >= 0 if disconnected successfully. 00082 * \return < 0 in case of errors 00083 */ 00084 int8_t thread_tasklet_disconnect(bool send_cb); 00085 00086 /* 00087 * \brief Set device data polling rate 00088 * 00089 * \param timeout timeout between data polls 00090 * \return 0 on success 00091 * \return < 0 in case of errors 00092 */ 00093 int8_t thread_tasklet_data_poll_rate_set(uint32_t timeout); 00094 00095 #ifdef __cplusplus 00096 } 00097 #endif 00098 #endif /* __INCLUDE_THREAD_TASKLET__ */
Generated on Sun Jul 17 2022 08:25:32 by
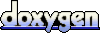