
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
thread_tasklet.c
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include <string.h> //memset 00018 #include "eventOS_event_timer.h" 00019 #include "common_functions.h" 00020 #include "net_interface.h" 00021 #include "ip6string.h" //ip6tos 00022 #include "nsdynmemLIB.h" 00023 #include "thread_management_if.h" 00024 #include "net_polling_api.h" 00025 #include "include/thread_tasklet.h" 00026 #include "include/mesh_system.h" 00027 #include <mbed_assert.h> 00028 #include "ns_event_loop.h" 00029 00030 // For tracing we need to define flag, have include and define group 00031 #define HAVE_DEBUG 1 00032 #include "ns_trace.h" 00033 #define TRACE_GROUP "m6Thread" 00034 00035 #include "mac_api.h" 00036 #include "sw_mac.h" 00037 00038 #define DETAILED_TRACES 00039 #ifdef DETAILED_TRACES 00040 #define TRACE_DETAIL tr_debug 00041 #else 00042 #define TRACE_DETAIL(...) 00043 #endif 00044 00045 #define INTERFACE_NAME "6L-THREAD" 00046 00047 // Tasklet timer events 00048 #define TIMER_EVENT_START_BOOTSTRAP 1 00049 00050 #define INVALID_INTERFACE_ID (-1) 00051 00052 /* 00053 * Thread tasklet states. 00054 */ 00055 typedef enum { 00056 TASKLET_STATE_CREATED = 0, 00057 TASKLET_STATE_INITIALIZED, 00058 TASKLET_STATE_BOOTSTRAP_STARTED, 00059 TASKLET_STATE_BOOTSTRAP_FAILED, 00060 TASKLET_STATE_BOOTSTRAP_READY 00061 } tasklet_state_t; 00062 00063 /* 00064 * Mesh tasklet data structure. 00065 */ 00066 typedef struct { 00067 void (*mesh_api_cb)(mesh_connection_status_t nwk_status); 00068 channel_list_s channel_list; 00069 tasklet_state_t tasklet_state; 00070 int8_t tasklet; 00071 00072 net_6lowpan_mode_e operating_mode; 00073 int8_t nwk_if_id; 00074 link_configuration_s link_config; 00075 00076 /** Default network ID*/ 00077 uint8_t networkid[16]; 00078 uint8_t extented_panid[8]; 00079 } thread_tasklet_data_str_t; 00080 00081 00082 /* Tasklet data */ 00083 static thread_tasklet_data_str_t *thread_tasklet_data_ptr = NULL; 00084 static device_configuration_s device_configuration; 00085 00086 /* private function prototypes */ 00087 void thread_tasklet_main(arm_event_s *event); 00088 void thread_tasklet_network_state_changed(mesh_connection_status_t status); 00089 void thread_tasklet_parse_network_event(arm_event_s *event); 00090 void thread_tasklet_configure_and_connect_to_network(void); 00091 #define TRACE_THREAD_TASKLET 00092 #ifndef TRACE_THREAD_TASKLET 00093 #define thread_tasklet_trace_bootstrap_info() ((void) 0) 00094 #else 00095 void thread_tasklet_trace_bootstrap_info(void); 00096 #endif 00097 00098 /* 00099 * \brief A function which will be eventually called by NanoStack OS when ever the OS has an event to deliver. 00100 * @param event, describes the sender, receiver and event type. 00101 * 00102 * NOTE: Interrupts requested by HW are possible during this function! 00103 */ 00104 void thread_tasklet_main(arm_event_s *event) 00105 { 00106 arm_library_event_type_e event_type; 00107 event_type = (arm_library_event_type_e) event->event_type; 00108 00109 switch (event_type) { 00110 case ARM_LIB_NWK_INTERFACE_EVENT: 00111 /* This event is delivered every and each time when there is new 00112 * information of network connectivity. 00113 */ 00114 thread_tasklet_parse_network_event(event); 00115 break; 00116 00117 case ARM_LIB_TASKLET_INIT_EVENT: 00118 /* Event with type EV_INIT is an initializer event of NanoStack OS. 00119 * The event is delivered when the NanoStack OS is running fine. 00120 * This event should be delivered ONLY ONCE. 00121 */ 00122 mesh_system_send_connect_event(thread_tasklet_data_ptr->tasklet); 00123 break; 00124 00125 case ARM_LIB_SYSTEM_TIMER_EVENT: 00126 eventOS_event_timer_cancel(event->event_id, 00127 thread_tasklet_data_ptr->tasklet); 00128 00129 if (event->event_id == TIMER_EVENT_START_BOOTSTRAP) { 00130 tr_debug("Restart bootstrap"); 00131 arm_nwk_interface_up(thread_tasklet_data_ptr->nwk_if_id); 00132 } 00133 break; 00134 00135 case APPLICATION_EVENT: 00136 if (event->event_id == APPL_EVENT_CONNECT) { 00137 thread_tasklet_configure_and_connect_to_network(); 00138 } 00139 break; 00140 00141 default: 00142 break; 00143 } // switch(event_type) 00144 } 00145 00146 /** 00147 * \brief Network state event handler. 00148 * \param event show network start response or current network state. 00149 * 00150 * - ARM_NWK_BOOTSTRAP_READY: Save NVK persistent data to NVM and Net role 00151 * - ARM_NWK_NWK_SCAN_FAIL: Link Layer Active Scan Fail, Stack is Already at Idle state 00152 * - ARM_NWK_IP_ADDRESS_ALLOCATION_FAIL: No ND Router at current Channel Stack is Already at Idle state 00153 * - ARM_NWK_NWK_CONNECTION_DOWN: Connection to Access point is lost wait for Scan Result 00154 * - ARM_NWK_NWK_PARENT_POLL_FAIL: Host should run net start without any PAN-id filter and all channels 00155 * - ARM_NWK_AUHTENTICATION_FAIL: Pana Authentication fail, Stack is Already at Idle state 00156 */ 00157 void thread_tasklet_parse_network_event(arm_event_s *event) 00158 { 00159 arm_nwk_interface_status_type_e status = (arm_nwk_interface_status_type_e) event->event_data; 00160 tr_debug("app_parse_network_event() %d", status); 00161 switch (status) { 00162 case ARM_NWK_BOOTSTRAP_READY: 00163 /* Network is ready and node is connected to Access Point */ 00164 if (thread_tasklet_data_ptr->tasklet_state != TASKLET_STATE_BOOTSTRAP_READY) { 00165 tr_info("Thread bootstrap ready"); 00166 thread_tasklet_data_ptr->tasklet_state = TASKLET_STATE_BOOTSTRAP_READY; 00167 thread_tasklet_trace_bootstrap_info(); 00168 thread_tasklet_network_state_changed(MESH_CONNECTED); 00169 } 00170 break; 00171 case ARM_NWK_NWK_SCAN_FAIL: 00172 /* Link Layer Active Scan Fail, Stack is Already at Idle state */ 00173 tr_debug("Link Layer Scan Fail: No Beacons"); 00174 thread_tasklet_data_ptr->tasklet_state = TASKLET_STATE_BOOTSTRAP_FAILED; 00175 break; 00176 case ARM_NWK_IP_ADDRESS_ALLOCATION_FAIL: 00177 /* No ND Router at current Channel Stack is Already at Idle state */ 00178 tr_debug("ND Scan/ GP REG fail"); 00179 thread_tasklet_data_ptr->tasklet_state = TASKLET_STATE_BOOTSTRAP_FAILED; 00180 break; 00181 case ARM_NWK_NWK_CONNECTION_DOWN: 00182 /* Connection to Access point is lost wait for Scan Result */ 00183 tr_debug("ND/RPL scan new network"); 00184 thread_tasklet_data_ptr->tasklet_state = TASKLET_STATE_BOOTSTRAP_FAILED; 00185 break; 00186 case ARM_NWK_NWK_PARENT_POLL_FAIL: 00187 thread_tasklet_data_ptr->tasklet_state = TASKLET_STATE_BOOTSTRAP_FAILED; 00188 break; 00189 case ARM_NWK_AUHTENTICATION_FAIL: 00190 tr_debug("Network authentication fail"); 00191 thread_tasklet_data_ptr->tasklet_state = TASKLET_STATE_BOOTSTRAP_FAILED; 00192 break; 00193 default: 00194 tr_warn("Unknown event %d", status); 00195 break; 00196 } 00197 00198 if (thread_tasklet_data_ptr->tasklet_state != TASKLET_STATE_BOOTSTRAP_READY) { 00199 // Set 5s timer for a new network scan 00200 eventOS_event_timer_request(TIMER_EVENT_START_BOOTSTRAP, 00201 ARM_LIB_SYSTEM_TIMER_EVENT, 00202 thread_tasklet_data_ptr->tasklet, 00203 5000); 00204 } 00205 } 00206 00207 void read_link_configuration() { 00208 00209 thread_tasklet_data_ptr->link_config.panId = MBED_CONF_MBED_MESH_API_THREAD_CONFIG_PANID; 00210 TRACE_DETAIL("PANID %x", thread_tasklet_data_ptr->link_config.panId); 00211 00212 thread_tasklet_data_ptr->link_config.rfChannel = MBED_CONF_MBED_MESH_API_THREAD_CONFIG_CHANNEL; 00213 TRACE_DETAIL("channel: %d", thread_tasklet_data_ptr->link_config.rfChannel); 00214 00215 // Mesh prefix 00216 const uint8_t mesh_local_prefix[] = MBED_CONF_MBED_MESH_API_THREAD_CONFIG_ML_PREFIX; 00217 MBED_ASSERT(sizeof(mesh_local_prefix) == 8); 00218 00219 memcpy(thread_tasklet_data_ptr->link_config.mesh_local_ula_prefix, mesh_local_prefix, 8); 00220 TRACE_DETAIL("Mesh prefix: %s", trace_array(mesh_local_prefix, 8)); 00221 00222 // Master Key 00223 const uint8_t master_key[] = MBED_CONF_MBED_MESH_API_THREAD_MASTER_KEY; 00224 MBED_ASSERT(sizeof(master_key) == 16); 00225 memcpy(thread_tasklet_data_ptr->link_config.master_key, master_key, 16); 00226 00227 // PSKc 00228 const uint8_t PSKc[] = MBED_CONF_MBED_MESH_API_THREAD_CONFIG_PSKC; 00229 MBED_ASSERT(sizeof(PSKc) == 16); 00230 memcpy(thread_tasklet_data_ptr->link_config.PSKc, PSKc, 16); 00231 00232 thread_tasklet_data_ptr->link_config.key_rotation = 3600; 00233 thread_tasklet_data_ptr->link_config.key_sequence = 0; 00234 00235 thread_tasklet_data_ptr->link_config.securityPolicy = MBED_CONF_MBED_MESH_API_THREAD_SECURITY_POLICY; 00236 00237 // network name 00238 MBED_ASSERT(strlen(MBED_CONF_MBED_MESH_API_THREAD_CONFIG_NETWORK_NAME) > 0 && strlen(MBED_CONF_MBED_MESH_API_THREAD_CONFIG_NETWORK_NAME) < 17); 00239 memcpy(thread_tasklet_data_ptr->link_config.name, MBED_CONF_MBED_MESH_API_THREAD_CONFIG_NETWORK_NAME, strlen(MBED_CONF_MBED_MESH_API_THREAD_CONFIG_NETWORK_NAME)); 00240 00241 thread_tasklet_data_ptr->link_config.timestamp = MBED_CONF_MBED_MESH_API_THREAD_CONFIG_COMMISSIONING_DATASET_TIMESTAMP; 00242 00243 // extended pan-id 00244 const uint8_t extented_panid[] = MBED_CONF_MBED_MESH_API_THREAD_CONFIG_EXTENDED_PANID; 00245 MBED_ASSERT(sizeof(extented_panid) == 8); 00246 memcpy(thread_tasklet_data_ptr->link_config.extented_pan_id, extented_panid, sizeof(extented_panid)); 00247 } 00248 00249 /* 00250 * \brief Configure mesh network 00251 * 00252 */ 00253 void thread_tasklet_configure_and_connect_to_network(void) 00254 { 00255 int8_t status; 00256 link_configuration_s* temp_link_config=NULL; 00257 00258 00259 if (MBED_CONF_MBED_MESH_API_THREAD_DEVICE_TYPE == MESH_DEVICE_TYPE_THREAD_MINIMAL_END_DEVICE) { 00260 thread_tasklet_data_ptr->operating_mode = NET_6LOWPAN_HOST; 00261 } 00262 else if (MBED_CONF_MBED_MESH_API_THREAD_DEVICE_TYPE == MESH_DEVICE_TYPE_THREAD_SLEEPY_END_DEVICE) { 00263 thread_tasklet_data_ptr->operating_mode = NET_6LOWPAN_SLEEPY_HOST; 00264 } else { 00265 thread_tasklet_data_ptr->operating_mode = NET_6LOWPAN_ROUTER; 00266 } 00267 00268 arm_nwk_interface_configure_6lowpan_bootstrap_set( 00269 thread_tasklet_data_ptr->nwk_if_id, 00270 thread_tasklet_data_ptr->operating_mode, 00271 NET_6LOWPAN_THREAD); 00272 00273 thread_tasklet_data_ptr->channel_list.channel_page = (channel_page_e)MBED_CONF_MBED_MESH_API_THREAD_CONFIG_CHANNEL_PAGE; 00274 thread_tasklet_data_ptr->channel_list.channel_mask[0] = MBED_CONF_MBED_MESH_API_THREAD_CONFIG_CHANNEL_MASK; 00275 00276 TRACE_DETAIL("channel page: %d", thread_tasklet_data_ptr->channel_list.channel_page); 00277 TRACE_DETAIL("channel mask: %d", (int)thread_tasklet_data_ptr->channel_list.channel_mask[0]); 00278 00279 // PSKd 00280 const char PSKd[] = MBED_CONF_MBED_MESH_API_THREAD_PSKD; 00281 MBED_ASSERT(sizeof(PSKd) > 5 && sizeof(PSKd) < 33); 00282 00283 char *dyn_buf = ns_dyn_mem_alloc(sizeof(PSKd)); 00284 strcpy(dyn_buf, PSKd); 00285 ns_dyn_mem_free(device_configuration.PSKd_ptr); 00286 device_configuration.PSKd_ptr = (uint8_t*)dyn_buf; 00287 device_configuration.PSKd_len = sizeof(PSKd) - 1; 00288 00289 if (true == MBED_CONF_MBED_MESH_API_THREAD_USE_STATIC_LINK_CONFIG) { 00290 read_link_configuration(); 00291 temp_link_config = &thread_tasklet_data_ptr->link_config; 00292 } 00293 00294 thread_management_node_init(thread_tasklet_data_ptr->nwk_if_id, 00295 &thread_tasklet_data_ptr->channel_list, 00296 &device_configuration, 00297 temp_link_config); 00298 00299 status = arm_nwk_interface_up(thread_tasklet_data_ptr->nwk_if_id); 00300 00301 if (status >= 0) { 00302 thread_tasklet_data_ptr->tasklet_state = TASKLET_STATE_BOOTSTRAP_STARTED; 00303 tr_info("Start Thread bootstrap (%s mode)", thread_tasklet_data_ptr->operating_mode == NET_6LOWPAN_SLEEPY_HOST ? "SED" : "Router"); 00304 } else { 00305 thread_tasklet_data_ptr->tasklet_state = TASKLET_STATE_BOOTSTRAP_FAILED; 00306 tr_err("Bootstrap start failed, %d", status); 00307 thread_tasklet_network_state_changed(MESH_BOOTSTRAP_START_FAILED); 00308 } 00309 } 00310 00311 /* 00312 * Inform application about network state change 00313 */ 00314 void thread_tasklet_network_state_changed(mesh_connection_status_t status) 00315 { 00316 if (thread_tasklet_data_ptr->mesh_api_cb) { 00317 (thread_tasklet_data_ptr->mesh_api_cb)(status); 00318 } 00319 } 00320 00321 /* 00322 * Trace bootstrap information. 00323 */ 00324 #ifdef TRACE_THREAD_TASKLET 00325 void thread_tasklet_trace_bootstrap_info() 00326 { 00327 link_layer_address_s app_link_address_info; 00328 uint8_t temp_ipv6[16]; 00329 if (arm_net_address_get(thread_tasklet_data_ptr->nwk_if_id, 00330 ADDR_IPV6_GP, temp_ipv6) == 0) { 00331 tr_debug("GP IPv6: %s", trace_ipv6(temp_ipv6)); 00332 } 00333 00334 if (arm_nwk_mac_address_read(thread_tasklet_data_ptr->nwk_if_id, 00335 &app_link_address_info) != 0) { 00336 tr_error("MAC Address read fail\n"); 00337 } else { 00338 uint8_t temp[2]; 00339 common_write_16_bit(app_link_address_info.mac_short,temp); 00340 tr_debug("MAC 16-bit: %s", trace_array(temp, 2)); 00341 common_write_16_bit(app_link_address_info.PANId, temp); 00342 tr_debug("PAN ID: %s", trace_array(temp, 2)); 00343 tr_debug("MAC 64-bit: %s", trace_array(app_link_address_info.mac_long, 8)); 00344 tr_debug("IID (Based on MAC 64-bit address): %s", trace_array(app_link_address_info.iid_eui64, 8)); 00345 } 00346 } 00347 #endif /* #define TRACE_THREAD_TASKLET */ 00348 00349 int8_t thread_tasklet_get_ip_address(char *address, int8_t len) 00350 { 00351 uint8_t binary_ipv6[16]; 00352 00353 if ((len >= 40) && (0 == arm_net_address_get( 00354 thread_tasklet_data_ptr->nwk_if_id, ADDR_IPV6_GP, binary_ipv6))) { 00355 ip6tos(binary_ipv6, address); 00356 //tr_debug("IP address: %s", address); 00357 return 0; 00358 } else { 00359 return -1; 00360 } 00361 } 00362 00363 int8_t thread_tasklet_connect(mesh_interface_cb callback, int8_t nwk_interface_id) 00364 { 00365 int8_t re_connecting = true; 00366 int8_t tasklet = thread_tasklet_data_ptr->tasklet; 00367 00368 if (thread_tasklet_data_ptr->nwk_if_id != INVALID_INTERFACE_ID) { 00369 return -3; // already connected to network 00370 } 00371 00372 if (thread_tasklet_data_ptr->tasklet_state == TASKLET_STATE_CREATED) { 00373 re_connecting = false; 00374 } 00375 00376 memset(thread_tasklet_data_ptr, 0, sizeof(thread_tasklet_data_str_t)); 00377 thread_tasklet_data_ptr->mesh_api_cb = callback; 00378 thread_tasklet_data_ptr->nwk_if_id = nwk_interface_id; 00379 thread_tasklet_data_ptr->tasklet_state = TASKLET_STATE_INITIALIZED; 00380 00381 if (re_connecting == false) { 00382 thread_tasklet_data_ptr->tasklet = eventOS_event_handler_create(&thread_tasklet_main, 00383 ARM_LIB_TASKLET_INIT_EVENT); 00384 if (thread_tasklet_data_ptr->tasklet < 0) { 00385 // -1 handler already used by other tasklet 00386 // -2 memory allocation failure 00387 return thread_tasklet_data_ptr->tasklet; 00388 } 00389 ns_event_loop_thread_start(); 00390 } else { 00391 thread_tasklet_data_ptr->tasklet = tasklet; 00392 mesh_system_send_connect_event(thread_tasklet_data_ptr->tasklet); 00393 } 00394 00395 return thread_tasklet_data_ptr->tasklet; 00396 } 00397 00398 int8_t thread_tasklet_disconnect(bool send_cb) 00399 { 00400 int8_t status = -1; 00401 // check that module is initialized 00402 if (thread_tasklet_data_ptr != NULL) { 00403 if (thread_tasklet_data_ptr->nwk_if_id != INVALID_INTERFACE_ID) { 00404 status = arm_nwk_interface_down(thread_tasklet_data_ptr->nwk_if_id); 00405 thread_tasklet_data_ptr->nwk_if_id = INVALID_INTERFACE_ID; 00406 if (send_cb == true) { 00407 thread_tasklet_network_state_changed(MESH_DISCONNECTED); 00408 } 00409 } 00410 00411 // Clear callback, it will be set again in next connect 00412 thread_tasklet_data_ptr->mesh_api_cb = NULL; 00413 } 00414 return status; 00415 } 00416 00417 void thread_tasklet_init(void) 00418 { 00419 if (thread_tasklet_data_ptr == NULL) { 00420 thread_tasklet_data_ptr = ns_dyn_mem_alloc(sizeof(thread_tasklet_data_str_t)); 00421 memset(thread_tasklet_data_ptr, 0, sizeof(thread_tasklet_data_str_t)); 00422 thread_tasklet_data_ptr->tasklet_state = TASKLET_STATE_CREATED; 00423 thread_tasklet_data_ptr->nwk_if_id = INVALID_INTERFACE_ID; 00424 } 00425 } 00426 00427 int8_t thread_tasklet_network_init(int8_t device_id) 00428 { 00429 // TODO, read interface name from configuration 00430 mac_description_storage_size_t storage_sizes; 00431 storage_sizes.device_decription_table_size = 32; 00432 storage_sizes.key_description_table_size = 6; 00433 storage_sizes.key_lookup_size = 1; 00434 storage_sizes.key_usage_size = 3; 00435 mac_api_t *api = ns_sw_mac_create(device_id, &storage_sizes); 00436 return arm_nwk_interface_lowpan_init(api, INTERFACE_NAME); 00437 } 00438 00439 void thread_tasklet_device_config_set(uint8_t *eui64, char *pskd) 00440 { 00441 (void) pskd; // this parameter is delivered via mbed configuration 00442 memcpy(device_configuration.eui64, eui64, 8); 00443 } 00444 00445 int8_t thread_tasklet_data_poll_rate_set(uint32_t timeout) 00446 { 00447 int8_t status = -1; 00448 if (thread_tasklet_data_ptr) { 00449 if (timeout != 0) { 00450 status = arm_nwk_host_mode_set(thread_tasklet_data_ptr->nwk_if_id, NET_HOST_SLOW_POLL_MODE, timeout); 00451 } else { 00452 status = arm_nwk_host_mode_set(thread_tasklet_data_ptr->nwk_if_id, NET_HOST_RX_ON_IDLE, timeout); 00453 } 00454 } 00455 00456 return status; 00457 } 00458
Generated on Sun Jul 17 2022 08:25:32 by
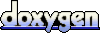