
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
thread_meshcop_lib.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2015-2017 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** 00016 * \file thread_meshcop_lib.h 00017 * \brief Public API to handle the Thread management framework message parsing and building. 00018 * 00019 */ 00020 00021 #ifndef THREAD_MESHCOP_LIB_H_ 00022 #define THREAD_MESHCOP_LIB_H_ 00023 00024 #include "ns_types.h" 00025 00026 /** 00027 * TLV Types used in thread network 00028 */ 00029 /** 00030 * Network configuration TLV 00031 */ 00032 /** Channel TLV. */ 00033 #define MESHCOP_TLV_CHANNEL 0 00034 /** PANID TLV. */ 00035 #define MESHCOP_TLV_PANID 1 00036 /** Extended PANID TLV. */ 00037 #define MESHCOP_TLV_XPANID 2 00038 /** Network Name TLV. */ 00039 #define MESHCOP_TLV_NETWORK_NAME 3 00040 /** PSKc TLV. */ 00041 #define MESHCOP_TLV_PSKC 4 00042 /** Network Master key TLV. */ 00043 #define MESHCOP_TLV_NETWORK_MASTER_KEY 5 00044 /** Network Key Sequence TLV. */ 00045 #define MESHCOP_TLV_NETWORK_KEY_SEQUENCE 6 00046 /** Mesh Local Ula prefix TLV. */ 00047 #define MESHCOP_TLV_NETWORK_MESH_LOCAL_ULA 7 00048 00049 /** 00050 * Network management TLV 00051 */ 00052 /** Steering data TLV. */ 00053 #define MESHCOP_TLV_STEERING_DATA 8 00054 /** Border router locator TLV. */ 00055 #define MESHCOP_TLV_BORDER_ROUTER_LOCATOR 9 00056 /** Commissioner ID TLV. */ 00057 #define MESHCOP_TLV_COMMISSIONER_ID 10 00058 /** Commissioner session ID TLV. */ 00059 #define MESHCOP_TLV_COMMISSIONER_SESSION_ID 11 00060 /** Security Policy TLV. */ 00061 #define MESHCOP_TLV_SECURITY_POLICY 12 00062 /** GET TLV. */ 00063 #define MESHCOP_TLV_GET 13 00064 /** Active Timestamp TLV. */ 00065 #define MESHCOP_TLV_ACTIVE_TIME_STAMP 14 00066 /** Commissioner UDP port TLV. */ 00067 #define MESHCOP_TLV_COMMISSIONER_UDP_PORT 15 00068 /** state TLV. */ 00069 #define MESHCOP_TLV_STATE 16 00070 /** Joiner UDP port TLV. */ 00071 #define MESHCOP_TLV_JOINER_UDP_PORT 18 00072 00073 /** 00074 * Network management TLV specific bit defines 00075 */ 00076 #define MESHCOP_TLV_ACTIVE_TIME_STAMP_U_BIT 0x01 00077 00078 /** 00079 * Relay message TLV 00080 */ 00081 /** Joiner Encapsulation TLV. */ 00082 #define MESHCOP_TLV_JOINER_ENCAPSULATION 17 00083 /** Joiner UDP port TLV. */ 00084 #define MESHCOP_TLV_JOINER_UDP_PORT 18 00085 /** Joiner IID TLV. */ 00086 #define MESHCOP_TLV_JOINER_IID 19 00087 /** Joiner Router locator TLV. */ 00088 #define MESHCOP_TLV_JOINER_ROUTER_LOCATOR 20 00089 /** Key Exchange Key TLV. */ 00090 #define MESHCOP_TLV_JOINER_ROUTER_KEK 21 00091 00092 /** 00093 * Application provisioning TLVs 00094 */ 00095 /** Provisioning URL TLV. */ 00096 #define MESHCOP_TLV_PROVISIONING_URL 32 00097 /** Vendr Name TLV. */ 00098 #define MESHCOP_TLV_VENDOR_NAME 33 00099 /** Vendor Model TLV. */ 00100 #define MESHCOP_TLV_VENDOR_MODEL 34 00101 /** Vendor SW version TLV. */ 00102 #define MESHCOP_TLV_VENDOR_SW_VERSION 35 00103 /** Vendor Data TLV. */ 00104 #define MESHCOP_TLV_VENDOR_DATA 36 00105 /** Vendor Stack Version TLV. */ 00106 #define MESHCOP_TLV_VENDOR_STACK_VERSION 37 00107 00108 /** 00109 * Tunneling 00110 */ 00111 /** UDP encapsulation TLV. */ 00112 #define MESHCOP_TLV_UDP_ENCAPSULATION 48 00113 /** IPv6 address TLV. */ 00114 #define MESHCOP_TLV_IPV6_ADDRESS 49 00115 /** TMF Forwarding Port TLV. */ 00116 #define MESHCOP_TLV_TMF_FORWARDING_PORT 50 00117 00118 /** 00119 * Thread management interface TLVs 00120 */ 00121 /** Pending Timestamp TLV. */ 00122 #define MESHCOP_TLV_PENDING_TIMESTAMP 51 00123 /** Delay timer for pending configuration TLV. */ 00124 #define MESHCOP_TLV_DELAY_TIMER 52 00125 /** Channel mask TLV. */ 00126 #define MESHCOP_TLV_CHANNEL_MASK 53 00127 /** Count TLV. */ 00128 #define MESHCOP_TLV_COUNT 54 00129 /** Period TLV. */ 00130 #define MESHCOP_TLV_PERIOD 55 00131 /** Duration TLV. */ 00132 #define MESHCOP_TLV_DURATION 56 00133 /** Energy List TLV. */ 00134 #define MESHCOP_TLV_ENERGY_LIST 57 00135 00136 /** Discovery Request TLV. */ 00137 #define MESHCOP_TLV_DISCOVERY_REQUEST 128 00138 /** Discovery response TLV. */ 00139 #define MESHCOP_TLV_DISCOVERY_RESPONSE 129 00140 00141 /** @defgroup Thread 1.2 experimental */ 00142 00143 /** Timeout TLV. */ 00144 #define MESHCOP_TLV_TIMEOUT 58 00145 00146 /** Domain prefix TLV TLV.*/ 00147 #define MESHCOP_TLV_DOMAIN_PREFIX 60 00148 00149 /** 00150 * Write array TLV. 00151 * 00152 * \param ptr pointer for array where to write the TLV. 00153 * \param type Type of TLV. 00154 * \param length length of the data that is written in TLV. 00155 * \param data array for TLV value. 00156 * 00157 * \return pointer value for writing the next TLV. 00158 */ 00159 uint8_t *thread_meshcop_tlv_data_write(uint8_t *ptr, const uint8_t type, const uint16_t length, const uint8_t *data); 00160 00161 /** 00162 * Write header. 00163 * 00164 * \param ptr pointer for array where to write the TLV. 00165 * \param type Type of TLV. 00166 * \param length length of the data that is written in TLV. 00167 * 00168 * \return pointer value for writing the remaining bytes of network data. 00169 */ 00170 uint8_t *thread_meshcop_tlv_data_write_header(uint8_t *ptr, uint8_t type, uint16_t length); 00171 00172 /** 00173 * Write 1 byte length TLV. 00174 * 00175 * \param ptr pointer for array where to write the TLV. 00176 * \param type Type of TLV. 00177 * \param data value. 00178 * 00179 * \return pointer value for writing the next TLV. 00180 */ 00181 uint8_t *thread_meshcop_tlv_data_write_uint8(uint8_t *ptr, const uint8_t type, const uint8_t data); 00182 00183 /** 00184 * Write 2 byte length TLV. 00185 * 00186 * \param ptr pointer for array where to write the TLV. 00187 * \param type Type of TLV. 00188 * \param data value. 00189 * 00190 * \return pointer value for writing the next TLV. 00191 */ 00192 uint8_t *thread_meshcop_tlv_data_write_uint16(uint8_t *ptr, const uint8_t type, const uint16_t data); 00193 00194 /** 00195 * Write 4 byte length TLV. 00196 * 00197 * \param ptr pointer for array where to write the TLV. 00198 * \param type Type of TLV. 00199 * \param data value. 00200 * 00201 * \return pointer value for writing the next TLV. 00202 */ 00203 uint8_t *thread_meshcop_tlv_data_write_uint32(uint8_t *ptr, const uint8_t type, const uint32_t data); 00204 00205 /** 00206 * Write 8 byte length TLV. 00207 * 00208 * \param ptr pointer for array where to write the TLV. 00209 * \param type Type of TLV. 00210 * \param data value. 00211 * 00212 * \return pointer value for writing the next TLV. 00213 */ 00214 uint8_t *thread_meshcop_tlv_data_write_uint64(uint8_t *ptr, const uint8_t type, const uint64_t data); 00215 00216 /** 00217 * Check if TLV exists in the message. 00218 * 00219 * \param ptr Message buffer. 00220 * \param length Length of the message buffer to validate message. 00221 * \param type Type of TLV searched. 00222 * 00223 * \return true if TLV is found. 00224 * \return false if TLV does not exist. 00225 */ 00226 bool thread_meshcop_tlv_exist(const uint8_t *ptr, const uint16_t length, const uint8_t type); 00227 00228 /** 00229 * Find TLV from message. 00230 * 00231 * \param ptr Message buffer. 00232 * \param length Length of the message buffer to validate message. 00233 * \param type Type of TLV searched. 00234 * \param result_ptr Pointer value is given as result if length is positive. Can be NULL which only searches for the length. 00235 * 00236 * \return The length of the TLV data found 00237 * \return 0 if TLV is empty or no TLV found. 00238 * \return negative value indicates corrupted message. 00239 */ 00240 uint16_t thread_meshcop_tlv_find(const uint8_t *ptr, const uint16_t length, const uint8_t type, uint8_t **result_ptr); 00241 00242 /** 00243 * Get length of the TLV. 00244 * 00245 * \param ptr Message buffer. 00246 * \param length Length of the message buffer to validate message. 00247 * 00248 * \return The length of the TLV data found 00249 * \return 0 if TLV is empty. 00250 * \return negative value indicates corrupted message or no TLV present. 00251 */ 00252 int16_t thread_meshcop_tlv_length(const uint8_t *ptr, uint16_t length); 00253 00254 /** 00255 * Get length of the TLV including the type and length field. 00256 * 00257 * \param ptr Message buffer. 00258 * \param length Length of the message buffer to validate message. 00259 * 00260 * \return The length of the TLV data found 00261 * \return 0 if TLV is empty. 00262 * \return negative value indicates corrupted message or no TLV present. 00263 */ 00264 int16_t thread_meshcop_tlv_length_required(const uint8_t *ptr, uint16_t length); 00265 00266 /** 00267 * Go through TLV list in order. 00268 * 00269 * \param ptr Message buffer. 00270 * \param length Length of the message buffer to validate message. Length value modified to remaining value or 0 when no more TLVs present 00271 * 00272 * \return ptr to the next TLV 00273 * \return NULL if no TLV found. 00274 */ 00275 const uint8_t *thread_meshcop_tlv_get_next(const uint8_t *ptr, uint16_t *length); 00276 00277 /** 00278 * Validate that required TLVs are present in the buffer. 00279 * 00280 * \param ptr TLV message buffer. 00281 * \param length Length of the TLV message buffer. 00282 * \param required_tlv_ptr buffer where the IDs of TLVs that are must be present 00283 * \param required_tlv_len Amount of TLVs 00284 * 00285 * \return amount of TLVs present in the buffer. 00286 */ 00287 bool thread_meshcop_tlv_list_present(const uint8_t *ptr, uint16_t length, const uint8_t *required_tlv_ptr, uint8_t required_tlv_len); 00288 00289 /** 00290 * Get list of TLVS included in the buffer. 00291 * 00292 * \param ptr TLV message buffer. 00293 * \param length Length of the TLV message buffer. 00294 * \param result_ptr [OUT] buffer where the IDs of TLVs are written. can be NULL when counting amount of TLVs 00295 * \param result_len [OUT] Amount of TLVs written in buffer 00296 * 00297 * \return amount of TLVs present in the buffer. 00298 */ 00299 uint16_t thread_meshcop_tlv_list_generate(const uint8_t *ptr, uint16_t length,uint8_t *result_ptr, uint16_t *result_len); 00300 00301 /** 00302 * Remove TLV from list of TLVs. 00303 * 00304 * \param tlv_ptr pointer to TLV List buffer. 00305 * \param tlv_len Length of the TLV list buffer. 00306 * \param tlv_type TLV that is removed from the List 00307 * 00308 * \return amount of TLVs present in the buffer. 00309 */ 00310 uint16_t thread_meshcop_tlv_list_remove(uint8_t *tlv_ptr, uint16_t tlv_len, uint8_t tlv_type); 00311 00312 /** 00313 * Check if specific type is available in list of TLVs. 00314 * 00315 * \param list_ptr Pointer to TLV list buffer. 00316 * \param list_len Length of the TLV list buffer. 00317 * \param tlv_type TLV type to be find from the list 00318 * 00319 * \return true if type exists, false if type does not exist. 00320 */ 00321 bool thread_meshcop_tlv_list_type_available(const uint8_t *list_ptr, uint16_t list_len, uint8_t tlv_type); 00322 00323 /** 00324 * Find next TLV from message. 00325 * 00326 * \param tlv_ba TLV message buffer. 00327 * \param tlv_ba_length Length of the TLV message buffer. 00328 * \param tlv_id ID of the TLV to be searched. 00329 * \param found_tlv [IN] Pointer value is given as result if length is > 0. Can be NULL which only searches for the length. 00330 * \ [OUT] Pointer to previous TLV found 00331 * 00332 * \return The length of the TLV data found and found_tlv updated to point beginning of value field. 0 if TLV is not found. 00333 */ 00334 uint16_t thread_meshcop_tlv_find_next(uint8_t* tlv_ba, uint16_t tlv_ba_length, uint8_t tlv_id, uint8_t** found_tlv); 00335 00336 /** 00337 * Read 1 byte length TLV. 00338 * 00339 * \param ptr pointer TLV message array. 00340 * \param length length of TLV message array. 00341 * \param type Type of TLV. 00342 * \param data_ptr pointer to variable where the value is read. 00343 * 00344 * \return pointer length of TLV 1 if success. 0 means TLV not found or does not have any data. 00345 * \return Any other value indicates that TLV is not as expected. 00346 */ 00347 uint8_t thread_meshcop_tlv_data_get_uint8(const uint8_t *ptr, const uint16_t length, const uint8_t type, uint8_t *data_ptr); 00348 00349 /** 00350 * Read 2 byte length TLV. 00351 * 00352 * \param ptr pointer TLV message array. 00353 * \param length length of TLV message array. 00354 * \param type Type of TLV. 00355 * \param data_ptr pointer to variable where the value is read. 00356 * 00357 * \return pointer length of TLV 2 if success. 0 means TLV not found or does not have any data. 00358 * \return Any other value indicates that TLV is not as expected. 00359 */ 00360 uint8_t thread_meshcop_tlv_data_get_uint16(const uint8_t *ptr, const uint16_t length, const uint8_t type, uint16_t *data_ptr); 00361 00362 /** 00363 * Read 4 byte length TLV. 00364 * 00365 * \param ptr pointer TLV message array. 00366 * \param length length of TLV message array. 00367 * \param type Type of TLV. 00368 * \param data_ptr pointer to variable where the value is read. 00369 * 00370 * \return pointer length of TLV 4 if success. 0 means TLV not found or does not have any data. 00371 * \return Any other value indicates that TLV is not as expected. 00372 */ 00373 uint8_t thread_meshcop_tlv_data_get_uint32(const uint8_t *ptr, const uint16_t length, const uint8_t type, uint32_t *data_ptr); 00374 00375 /** 00376 * Read 8 byte length TLV. 00377 * 00378 * \param ptr pointer TLV message array. 00379 * \param length length of TLV message array. 00380 * \param type Type of TLV. 00381 * \param data_ptr pointer to variable where the value is read. 00382 * 00383 * \return pointer length of TLV 4 if success. 0 means TLV not found or does not have any data. 00384 * \return Any other value indicates that TLV is not as expected. 00385 */ 00386 uint8_t thread_meshcop_tlv_data_get_uint64(const uint8_t *ptr, const uint16_t length, const uint8_t type, uint64_t *data_ptr); 00387 00388 #endif /* THREAD_MESHCOP_LIB_H_ */
Generated on Sun Jul 17 2022 08:25:32 by
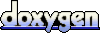