
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
thread_management_if.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2014-2016 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** 00016 * \file thread_management_if.h 00017 * \brief Thread management interface. 00018 * 00019 * This interface is used for configuring Thread devices. 00020 * After creating the Thread interface, you can use this interface to configure the Thread device 00021 * behaviour. When you are done with the configurations, you need to call interface up to enable a Thread node. 00022 * 00023 */ 00024 00025 #ifndef THREAD_MANAGEMENT_IF_H_ 00026 #define THREAD_MANAGEMENT_IF_H_ 00027 00028 #include "ns_types.h" 00029 #include "net_interface.h" /* Declaration for channel_list_s. */ 00030 00031 #ifdef __cplusplus 00032 extern "C" { 00033 #endif 00034 00035 /* 00036 * Current protocol version of the Thread implementation. 00037 */ 00038 #define THREAD_BEACON_PROTOCOL_ID 3 /**< Beacon Protocol ID */ 00039 #define THREAD_BEACON_PROTOCOL_VERSION 1 /**< Beacon Protocol version */ 00040 00041 /** 00042 * Thread network configuration. 00043 * 00044 * You can use this structure in start-up in case of a static configuration. 00045 * This data can also be read after joining the Thread network. 00046 * If this data is not provided to the stack, the device starts the commissioning process to join the Thread network. 00047 * 00048 * If the data is provided, all fields must be initialised to 0. 00049 * 00050 * If XPANID and MASTER KEY are provided, the device starts out-of-band commissioning. The values must be initialised to other than 0. 00051 * If mesh_local_eid is initialised to 0 it is randomized at start-up. 00052 * If extended_random_mac is initialised to 0 it is randomized at start-up. 00053 * 00054 * If timestamp values are set to 0 it triggers a network configuration update when joining the network. 00055 * 00056 * */ 00057 typedef struct link_configuration { 00058 uint8_t name[16]; /**< Name of the Thread network*/ 00059 uint8_t master_key[16]; /**< Master key of the thread network*/ 00060 uint8_t PSKc[16]; /**< PSKc value that is calculated from commissioning credentials credentials,XPANID and network name*/ 00061 uint8_t mesh_local_ula_prefix[8]; /**< Mesh local ula prefix*/ 00062 uint8_t mesh_local_eid[8]; /**< Mesh local extented id*/ 00063 uint8_t extented_pan_id[8]; /**< Extended pan id*/ 00064 uint8_t extended_random_mac[8]; /**< Extended random mac which is generated during commissioning*/ 00065 uint8_t channel_mask[8]; /**< channel page and mask only supported is page 0*/ 00066 uint8_t channel_page;/**< channel page supported pages 0*/ 00067 char *PSKc_ptr; /**< Commissioning credentials. TODO! think if we need the actual credentials*/ 00068 uint8_t PSKc_len; /**< Length of PSKc */ 00069 uint16_t key_rotation; /**< Key rotation time in hours*/ 00070 uint32_t key_sequence; /**< Key sequence counter */ 00071 uint16_t panId; /**< network id*/ 00072 uint8_t Protocol_id; /**< current protocol id*/ 00073 uint8_t version; /**< current protocol version*/ 00074 uint16_t rfChannel; /**< current rf channel*/ 00075 uint8_t securityPolicy; /**< Commission Security Policy*/ 00076 uint64_t timestamp;/**< commissioning data set timestamp. [48 bit timestamp seconds]-[15 bit timestamp ticks]-[U bit] */ 00077 } link_configuration_s; 00078 00079 /** 00080 * Security policy options. Default for all is '1'; 00081 */ 00082 #define SECURITY_POLICY_ALL_SECURITY 0xff 00083 #define SECURITY_POLICY_OUT_OF_BAND_COMMISSIONING_ALLOWED 0x80 /**< Obtaining the Master Key for out-of-band commissioning is enabled when this is set. */ 00084 #define SECURITY_POLICY_NATIVE_COMMISSIONING_ALLOWED 0x40 /**< Native Commissioning using PSKc is allowed when this is set. */ 00085 #define SECURITY_POLICY_ALL_ROUTERS_JOIN_ALLOWED 0x20 /**< Thread 1.x Routers are enabled when this is set. */ 00086 #define SECURITY_POLICY_EXTERNAL_COMMISSIONER_ALLOWED 0x10 /**< This indicates that external Commissioner authentication is allowed using PSKc. */ 00087 #define SECURITY_POLICY_BEACON_PAYLOAD_ENABLED 0x08 /**< Thread 1.x Beacons are enabled when this is set. */ 00088 00089 /* 00090 * Mandatory device information 00091 * 00092 * This information is required if commissioning is enabled for this device. 00093 */ 00094 typedef struct { 00095 uint8_t eui64[8];/**< eui64 of the device. This field is used to identify device when joining to network Mandatory*/ 00096 uint8_t *PSKd_ptr;/**< Device credentials used to authenticate device to commissioner Mandatory length 6-32*/ 00097 uint8_t PSKd_len;/**< Length of PSKd_ptr*/ 00098 char *provisioning_uri_ptr;/**< Provisioning url max 64 bytes*/ 00099 char *vendor_name_ptr;/**< Vendor name optional max 32 bytes*/ 00100 char *vendor_model_ptr;/**< Vendor model optional max 32 bytes*/ 00101 char *vendor_sw_version_ptr;/**< Vendor SW version optional max 16 bytes*/ 00102 uint8_t vendor_stack_version[6];/**< Vendor stack version optional all 0 indicates not set*/ 00103 uint8_t *vendor_data_ptr;/**< optional Array max 64 bytes*/ 00104 uint8_t vendor_data_len;/**< optional Array length max 64 bytes*/ 00105 } device_configuration_s; 00106 00107 00108 /** 00109 * Initialize Thread stack to node mode. 00110 * 00111 * If static configuration is given and new one is updated by commissioner 00112 * it will override current setup. it is safe to always give this as 00113 * default configuration. 00114 * 00115 * \param interface_id Network interface ID. 00116 * \param channel_list A pointer to channel list. Can be NULL if all channels accepted. 00117 * \param device_configuration A pointer to device configuration. 00118 * \param static_configuration A pointer to static configuration. Can be NULL. 00119 * 00120 * \return 0, Init OK. 00121 * \return <0 Init fail. 00122 */ 00123 int thread_management_node_init( 00124 int8_t interface_id, 00125 channel_list_s *channel_list, 00126 device_configuration_s *device_configuration, 00127 link_configuration_s *static_configuration); 00128 00129 /** 00130 * Thread device type. 00131 * 00132 * REED - Router enabled End device. Device can become router or end device depending on network conditions. 00133 * FED - Full End Device. Device creates links and makes address queries but does not become router. 00134 * MED - Minimal End Device. Device communicates through parent. With radio on 00135 * SED - Sleepy End Device. Device communicates through parent. Uses data poll to sleep. 00136 */ 00137 typedef enum { 00138 THREAD_DEVICE_REED = 1, 00139 THREAD_DEVICE_FED, 00140 THREAD_DEVICE_MED, 00141 THREAD_DEVICE_SED, 00142 } thread_device_type_e; 00143 00144 /** 00145 * Change thread device type. 00146 * 00147 * This function modifies the thread device mode. Default values are given in 00148 * function arm_nwk_interface_configure_6lowpan_bootstrap_set(). 00149 * 00150 * If this function is called when interface is up re-attach is made. 00151 * 00152 * \param interface_id Network interface ID. 00153 * \param device_type Device type of current bootstrap. 00154 * 00155 * \return 0, Set OK. 00156 * \return <0 Set fail. 00157 */ 00158 int thread_management_device_type_set(int8_t interface_id, thread_device_type_e device_type); 00159 00160 /** 00161 * Get Thread network settings. 00162 * 00163 * Configuration is a pointer to the static configuration and only valid in current context. 00164 * 00165 * \param interface_id Network interface ID. 00166 * 00167 * \return Pointer to link configuration. 00168 * \return NULL Failure. 00169 */ 00170 link_configuration_s *thread_management_configuration_get(int8_t interface_id); 00171 00172 /** Store Thread network link configuration settings to NVM. 00173 * 00174 * Storing is asynchronous operation and this method makes a request to store link 00175 * configuration settings. Operation will be completed in the background. 00176 * Once settings has been stored the Thread network will be restarted with new 00177 * configuration settings. 00178 * 00179 * /param interface Id of network interface. -1 if interface_id is not available. 00180 * /param link_config Pointer to a structure containing link configuration parameters 00181 * 00182 * /return 0 if store request has been delivered successfully to lower layer. 00183 * /return -1 if storing failed (request not delivered to lower layer) 00184 * /return -2 if store request delivered to lower layer but given interface_id was not valid. 00185 */ 00186 int thread_management_link_configuration_store(int8_t interface_id, link_configuration_s *link_config); 00187 00188 /** Delete Thread network link configuration settings. 00189 * 00190 * Deletion is asynchronous operation and this method makes a request to delete link 00191 * configuration settings. Operation will be completed in the background. 00192 * Once settings has been deleted the Thread network will be restarted with default settings. 00193 * 00194 * /param interface Id of network interface. -1 can be used if interface_id is not available. 00195 * 00196 * /return 0 if delete request has been delivered successfully to lower layer. 00197 * /return -1 if delete failed (request not delivered to lower layer) 00198 * /return -2 if delete request delivered to lower layer but given interface_id was not valid. 00199 */ 00200 int thread_management_link_configuration_delete(int8_t interface_id); 00201 00202 /** 00203 * Get Thread device settings. 00204 * 00205 * Configuration is a pointer to the static device configuration and only valid in current context. 00206 * 00207 * \param interface_id Network interface ID. 00208 * 00209 * \return Pointer to Device configuration. 00210 * \return NULL Failure. 00211 */ 00212 device_configuration_s *thread_management_device_configuration_get(int8_t interface_id); 00213 00214 /** 00215 * Thread router max child count set. 00216 * 00217 * This function is used to limit the number of children allowed for parent. 00218 * 00219 * \param interface_id Network interface ID. 00220 * \param maxChildCount Min accepted value is 0 and max 32. 00221 * 00222 * \return 0, Set OK. 00223 * \return <0 Set Fail. 00224 */ 00225 int thread_management_max_child_count( 00226 int8_t interface_id, 00227 uint8_t maxChildCount); 00228 00229 /** 00230 * Set Thread device link timeout. 00231 * 00232 * \param interface_id Network interface ID. 00233 * \param link_timeout New timeout value in seconds. 00234 * 00235 * \return 0, Set OK. 00236 * \return <0 Set Fail. 00237 */ 00238 int8_t thread_management_set_link_timeout(int8_t interface_id, uint32_t link_timeout); 00239 00240 /** 00241 * Get link timeout from Thread device. 00242 * 00243 * \param interface_id Network interface ID. 00244 * \param link_timeout [out] A pointer to the location for writing the timeout. 00245 * 00246 * \return 0, Get OK 00247 * \return <0 Get Fail 00248 */ 00249 int8_t thread_management_get_link_timeout(int8_t interface_id, uint32_t *link_timeout); 00250 00251 /** 00252 * Set Thread request full network data. 00253 * 00254 * \param interface_id Network interface ID. 00255 * \param full_nwk_data Whether or not to request full network data. 00256 * 00257 * \return 0, Set OK. 00258 * \return <0 Set Fail. 00259 */ 00260 int8_t thread_management_set_request_full_nwk_data(int8_t interface_id, bool full_nwk_data); 00261 00262 /** 00263 * Get Thread request full network data. 00264 * 00265 * \param interface_id Network interface ID. 00266 * \param link_timeout [out] A pointer to the location for writing the flag value. 00267 * \param full_nwk_data Request full network data 00268 * 00269 * \return 0, Get OK. 00270 * \return <0 Get Fail. 00271 */ 00272 int8_t thread_management_get_request_full_nwk_data(int8_t interface_id, bool *full_nwk_data); 00273 00274 /** 00275 * Additional Thread device settings. Changing these can cause non-compliance with Thread. 00276 * 00277 */ 00278 00279 /** 00280 * Diagnostics functions. 00281 */ 00282 00283 /** 00284 * Get leader mesh local 16 address. 00285 * 00286 * \param interface_id Network interface ID. 00287 * \param address_ptr A pointer to the location of address after copying. 00288 * 00289 * \return 0, Read OK. 00290 * \return <0 Read fail. 00291 */ 00292 int thread_management_get_leader_address(int8_t interface_id, uint8_t *address_ptr); 00293 00294 /** 00295 * Get leader anycast address. 00296 * 00297 * Address should be used when contacting Leader without need to know the actual routing details. 00298 * This address will remain valid even after leader changes. 00299 * 00300 * \param interface_id Network interface ID. 00301 * \param address_ptr A pointer to the location of address after copying. 00302 * 00303 * \return 0, Read OK. 00304 * \return <0 Read fail. Not connected to Thread network. 00305 */ 00306 int thread_management_get_leader_aloc(int8_t interface_id, uint8_t *address_ptr); 00307 00308 /** 00309 * Get parent link local 16 address. 00310 * 00311 * \param interface_id Network interface ID. 00312 * \param address_ptr A pointer to the location of address after copying. 00313 * 00314 * \return 0, Read OK. 00315 * \return <0 Read fail. 00316 */ 00317 int thread_management_get_parent_address(int8_t interface_id, uint8_t *address_ptr); 00318 00319 /** 00320 * Get own mesh local 64 address. 00321 * 00322 * \param interface_id Network interface ID. 00323 * \param address_ptr A pointer to the location of address after copying. 00324 * 00325 * \return 0, Read OK. 00326 * \return <0 Read fail. 00327 */ 00328 int thread_management_get_ml64_address(int8_t interface_id, uint8_t *address_ptr); 00329 00330 /** 00331 * Get own mesh local 16 address. 00332 * 00333 * \param interface_id Network interface ID. 00334 * \param address_ptr A pointer to the location of address after copying. 00335 * 00336 * \return 0, Read OK. 00337 * \return <0 Read fail. 00338 */ 00339 int thread_management_get_ml16_address(int8_t interface_id, uint8_t *address_ptr); 00340 00341 /** 00342 * Get commissioner address. 00343 * 00344 * This function returns the commissioner address where you can continue provisioning traffic. 00345 * If the commissioner is not present this function returns a failure. 00346 * 00347 * \param interface_id Network interface ID. 00348 * \param address_ptr A pointer to the location of address after copying. 00349 * \param port_ptr A pointer to the location of port after copying. 00350 * 00351 * \return 0, Read OK. 00352 * \return <0 Read fail. 00353 */ 00354 int thread_management_get_commissioner_address(int8_t interface_id, uint8_t *address_ptr, uint16_t *port_ptr); 00355 00356 00357 #ifdef __cplusplus 00358 } 00359 #endif 00360 00361 #endif /* THREAD_MANAGEMENT_IF_H_ */
Generated on Sun Jul 17 2022 08:25:32 by
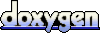