
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
thread_diagcop_lib.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2015-2016 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 00016 #ifndef NANOSTACK_SOURCE_6LOWPAN_THREAD_THREAD_DIAGCOP_LIB_H_ 00017 #define NANOSTACK_SOURCE_6LOWPAN_THREAD_THREAD_DIAGCOP_LIB_H_ 00018 00019 #include "ns_types.h" 00020 #include "thread_meshcop_lib.h" // this uses the same parser functions than meshcop but different message types 00021 00022 /** 00023 * \file thread_diagcop_lib.h 00024 * \brief CoAP diagnostic TLV. 00025 * Note that these TLV types overlap with meshcop 00026 * and must not be used in same messages. 00027 */ 00028 00029 #define DIAGCOP_TLV_EXTENDED_MAC_ADDRESS 0 /**< Can not reset*/ 00030 #define DIAGCOP_TLV_ADDRESS16 1 /**< Can not reset*/ 00031 #define DIAGCOP_TLV_MODE 2 /**< Can not reset*/ 00032 #define DIAGCOP_TLV_TIMEOUT 3 /**< Can not reset*/ 00033 #define DIAGCOP_TLV_CONNECTIVITY 4 /**< Can not reset*/ 00034 #define DIAGCOP_TLV_ROUTE64 5 /**< Can not reset*/ 00035 #define DIAGCOP_TLV_LEADER_DATA 6 /**< Can not reset*/ 00036 #define DIAGCOP_TLV_NETWORK_DATA 7 /**< Can not reset*/ 00037 #define DIAGCOP_TLV_IPV6_ADDRESS_LIST 8 /**< Can not reset*/ 00038 #define DIAGCOP_TLV_MAC_COUNTERS 9 /**< Can reset*/ 00039 #define DIAGCOP_TLV_BATTERY_LEVEL 14 /**< Can reset*/ 00040 #define DIAGCOP_TLV_SUPPLY_VOLTAGE 15 /**< Can not reset*/ 00041 #define DIAGCOP_TLV_CHILD_TABLE 16 /**< Can not reset*/ 00042 #define DIAGCOP_TLV_CHANNEL_PAGES 17 /**< Can not reset*/ 00043 #define DIAGCOP_TLV_TYPE_LIST 18 /**< List type*/ 00044 00045 /** 00046 * \brief Write array TLV. 00047 * 00048 * \param ptr A pointer to array in which to write the TLV. 00049 * \param type The type of TLV. 00050 * \param length The length of the data that is written in TLV. 00051 * \param data The array for TLV value. 00052 * \return pointer The value for writing the next TLV. 00053 */ 00054 #define thread_diagcop_tlv_data_write(ptr,type,length,data) thread_meshcop_tlv_data_write(ptr,type,length,data) 00055 00056 /** 00057 * \brief Write 1 byte length TLV. 00058 * 00059 * \param ptr A pointer to array in which to write the TLV. 00060 * \param type The type of TLV. 00061 * \param data value. 00062 * \return pointer value for writing the next TLV. 00063 */ 00064 #define thread_diagcop_tlv_data_write_uint8(ptr,type,data) thread_meshcop_tlv_data_write_uint8(ptr,type,data) 00065 00066 /** 00067 * \brief Write 2 byte length TLV. 00068 * 00069 * \param ptr pointer for array where to write the TLV. 00070 * \param type Type of TLV. 00071 * \param data value. 00072 * \return pointer value for writing the next TLV. 00073 */ 00074 #define thread_diagcop_tlv_data_write_uint16(ptr,type,data) thread_meshcop_tlv_data_write_uint16(ptr,type,data) 00075 00076 /** 00077 * \brief Write 4 byte length TLV. 00078 * 00079 * \param ptr pointer for array where to write the TLV. 00080 * \param type Type of TLV. 00081 * \param data value. 00082 * \return pointer value for writing the next TLV. 00083 */ 00084 #define thread_diagcop_tlv_data_write_uint32(ptr,type,data) thread_meshcop_tlv_data_write_uint32(ptr,type,data) 00085 00086 /** 00087 * \brief Write 8 byte length TLV. 00088 * 00089 * \param ptr pointer for array where to write the TLV. 00090 * \param type Type of TLV. 00091 * \param data value. 00092 * \return pointer value for writing the next TLV. 00093 */ 00094 #define thread_diagcop_tlv_data_write_uint64(ptr,type,data) thread_meshcop_tlv_data_write_uint64(ptr,type,data) 00095 00096 /** 00097 * \brief Find TLV from message. 00098 * 00099 * \param ptr Message buffer. 00100 * \param length Length of the message buffer to validate message. 00101 * \param type Type of TLV searched. 00102 * \param result Pointer value is given as result if length is positive. Can be NULL which only searches for the length. 00103 * \return The length of the TLV data found 00104 * \return 0 if TLV is empty or no TLV found. 00105 * \return negative value indicates corrupted message. 00106 */ 00107 #define thread_diagcop_tlv_find(ptr,length,type,result) thread_meshcop_tlv_find(ptr,length,type,result) 00108 00109 /** 00110 * \brief Read 1 byte length TLV. 00111 * 00112 * \param ptr pointer TLV message array. 00113 * \param length length of TLV message array. 00114 * \param type Type of TLV. 00115 * \param data pointer to variable where the value is read. 00116 * \return pointer length of TLV 1 if success. 0 means TLV not found or does not have any data. 00117 * Any other value indicates that TLV is not as expected. 00118 */ 00119 #define thread_diagcop_tlv_data_get_uint8(ptr,length,type,data) thread_meshcop_tlv_data_get_uint8(ptr,length,type,data) 00120 00121 /** 00122 * \brief Read 2 byte length TLV. 00123 * 00124 * \param ptr pointer TLV message array. 00125 * \param length length of TLV message array. 00126 * \param type Type of TLV. 00127 * \param data pointer to variable where the value is read. 00128 * \return pointer length of TLV 2 if success. 0 means TLV not found or does not have any data. 00129 * Any other value indicates that TLV is not as expected. 00130 */ 00131 #define thread_diagcop_tlv_data_get_uint16(ptr,length,type,data) thread_meshcop_tlv_data_get_uint16(ptr,length,type,data) 00132 00133 /** 00134 * \brief Read 4 byte length TLV. 00135 * 00136 * \param ptr pointer TLV message array. 00137 * \param length length of TLV message array. 00138 * \param type Type of TLV. 00139 * \param data pointer to variable where the value is read. 00140 * \return pointer length of TLV 4 if success. 0 means TLV not found or does not have any data. 00141 * Any other value indicates that TLV is not as expected. 00142 */ 00143 #define thread_diagcop_tlv_data_get_uint32(ptr,length,type,data) thread_meshcop_tlv_data_get_uint32(ptr,length,type,data) 00144 00145 /** 00146 * \brief Read 8 byte length TLV. 00147 * 00148 * \param ptr pointer TLV message array. 00149 * \param length length of TLV message array. 00150 * \param type Type of TLV. 00151 * \param data pointer to variable where the value is read. 00152 * \return pointer length of TLV 4 if success. 0 means TLV not found or does not have any data. 00153 * Any other value indicates that TLV is not as expected. 00154 */ 00155 #define thread_diagcop_tlv_data_get_uint64(ptr,length,type,data) thread_meshcop_tlv_data_get_uint64(ptr,length,type,data) 00156 00157 00158 #endif /* NANOSTACK_SOURCE_6LOWPAN_THREAD_THREAD_DIAGCOP_LIB_H_ */
Generated on Sun Jul 17 2022 08:25:32 by
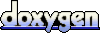