
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
thread_dhcpv6_server.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2014-2016 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** 00016 * \file thread_dhcpv6_server.h 00017 * \brief Thread specific DHCP server. 00018 * 00019 * \warning This api will be removed as there is no Thread specific modifications anymore. 00020 */ 00021 00022 #ifndef THREAD_DHCPV6_SERVER_H_ 00023 #define THREAD_DHCPV6_SERVER_H_ 00024 00025 #include "ns_types.h" 00026 00027 /** 00028 * Set DHCPV6 server for Thread GP data purpose 00029 * 00030 * \param interface_id Network Interface 00031 * \param prefix_ptr pointer DHCPv6 Server Given Prefix 00032 * \param max_client_cnt Max number of clients 00033 * \param stableData True if data is stable 00034 * 00035 * \return 0, Set OK 00036 * \return <0 Set Not OK 00037 */ 00038 int thread_dhcpv6_server_add(int8_t interface_id, uint8_t *prefix_ptr, uint16_t max_client_cnt, bool stableData); 00039 00040 /** 00041 * Modify dhcp address valid lifetime values default value is 1 day (86400) 00042 * 00043 * \param interface_id Network Interface 00044 * \param prefix_ptr pointer DHCPv6 Server Given Prefix (size must be 16 bytes) 00045 * \param valid_lifetime New valid life value given to new address valid values are (120 - 0xffffffff) 00046 * 00047 * \return 0, ADD OK 00048 * \return <0 Add Not OK 00049 */ 00050 int thread_dhcpv6_server_set_lifetime(int8_t interface_id, uint8_t *prefix_ptr, uint32_t valid_lifetime); 00051 00052 /** 00053 * Modify dhcp maximum client count. 00054 * 00055 * \param interface_id Network Interface 00056 * \param prefix_ptr pointer DHCPv6 Server Given Prefix (size must be 16 bytes) 00057 * \param max_client_count maximum amount of clients valid values (1 - 0xffffffff) 00058 * 00059 * \return 0, ADD OK 00060 * \return <0 Add Not OK 00061 */ 00062 int thread_dhcpv6_server_set_max_client(int8_t interface_id, uint8_t *prefix_ptr, uint32_t max_client_count); 00063 00064 /** 00065 * Modify dhcp anonymous mode. 00066 * 00067 * \param interface_id Network Interface 00068 * \param prefix_ptr pointer DHCPv6 Server Given Prefix (size must be 16 bytes) 00069 * \param anonymous true == addressing mode is anonymous, false == addressing mode uses mac address after prefix. 00070 * 00071 * \return 0, ADD OK 00072 * \return <0 Add Not OK 00073 */ 00074 int thread_dhcpv6_server_set_anonymous_addressing(int8_t interface_id, uint8_t *prefix_ptr, bool anonymous); 00075 00076 /** 00077 * Stop DHCPV6 server for Thread GP data purpose 00078 * 00079 * \param interface_id Network Interface 00080 * \param prefix_ptr pointer DHCPv6 Server Given Prefix 00081 * 00082 * \return 0, Stop OK 00083 * \return <0 Stop Not OK 00084 */ 00085 int thread_dhcpv6_server_delete(int8_t interface_id, uint8_t *prefix_ptr); 00086 00087 #endif /* THREAD_DHCPV6_SERVER_H_ */
Generated on Sun Jul 17 2022 08:25:32 by
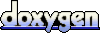