
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
thread_commissioning_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2015-2016 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** 00016 * \file thread_commissioning_api.h 00017 * \brief Thread commissioning API. 00018 * 00019 * This is a public API used for creating a commissioning device. You can create an on-mesh commissioner 00020 * and a native commissioner. 00021 */ 00022 00023 #ifndef THREAD_COMMISSIONING_API_H_ 00024 #define THREAD_COMMISSIONING_API_H_ 00025 00026 #ifdef __cplusplus 00027 extern "C" { 00028 #endif 00029 00030 #include "ns_types.h" 00031 00032 #define TRACE_GROUP_THREAD_COMMISSIONING_API "TCoA" /**< trace group definition */ 00033 00034 /** 00035 * /enum commissioning_state_e 00036 * /brief Commissioning states. 00037 */ 00038 typedef enum { 00039 COMMISSIONING_STATE_ACCEPT, 00040 COMMISSIONING_STATE_PENDING, 00041 COMMISSIONING_STATE_REJECT, 00042 COMMISSIONING_STATE_NO_NETWORK 00043 } commissioning_state_e; 00044 00045 /** \brief Commissioning petition response callback. 00046 * 00047 * \param interface_id Network interface ID. The request comes from this ID. 00048 * \param commissioner_session_id Commissioner session ID. 00049 * \param state State of the commissioning. 00050 * 00051 * \return 0 success, other values failure. 00052 */ 00053 typedef int (thread_commissioning_status_cb)(int8_t interface_id, uint16_t commissioner_session_id, commissioning_state_e state); 00054 00055 00056 /** \brief Register the commissioner interface. 00057 * 00058 * If the network interface is up, the commissioner functionality is started within the Thread network. 00059 * If there is no interface, the network needs to be scanned first. When the network is found you can add an insecure commissioner, 00060 * attach to it and start using a different communication method with the border router. 00061 * 00062 * \param interface_id Interface ID where the request was made. 00063 * \param PSKc Pre-shared key between the commissioner and the Thread network. 00064 * 00065 * \return 0 success, other values failure. 00066 */ 00067 int thread_commissioning_register(int8_t interface_id, uint8_t PSKc[16]); 00068 00069 /** \brief Unregister the commissioner interface. 00070 * 00071 * This cleans up all the commissioner data from the device and disconnects it from the Thread network if an insecure commissioner was used. 00072 * 00073 * \param interface_id Network interface ID. The request comes from this ID. 00074 * 00075 * \return 0 success, other values failure. 00076 */ 00077 int thread_commissioning_unregister(int8_t interface_id); 00078 00079 /** \brief Start the commissioning petition. 00080 * 00081 * If the commissioner is insecure, you need to scan the networks and select the Thread network where you want to be a commissioner. 00082 * 00083 * \param interface_id Network interface ID. The request comes from this ID. 00084 * \param commissioner_id_ptr NUL terminated commissioner ID string. 00085 * \param status_cb_ptr A callback function indicating the result of the operation. Can be NULL if no result code needed. 00086 * \return 0 Indicates success. 00087 * 00088 * \return -1 The client needs to scan the network to become an insecure commissioner. 00089 * \return Any other value indicates other failures. 00090 */ 00091 int thread_commissioning_petition_start(int8_t interface_id, char *commissioner_id_ptr, thread_commissioning_status_cb *status_cb_ptr); 00092 00093 /** \brief Send petition keep alive. 00094 * 00095 * This function must be called in 40 second intervals. TODO rethink if this should be automatic. 00096 * 00097 * \param interface_id Network interface ID. The request comes from this ID. 00098 * \param state Commissioning state. 00099 * 00100 * \return 0 success, other values failure. 00101 */ 00102 int thread_commissioning_petition_keep_alive(int8_t interface_id, commissioning_state_e state); 00103 00104 /** \brief Callback received when a new device is completing the joining process. 00105 * 00106 * The message may include the following meshcop TLV fields: 00107 * * State TLV 00108 * * Vendor Name TLV 00109 * * Vendor Model TLV 00110 * * Vendor SW Version TLV 00111 * * Vendor Data TLV 00112 * * Vendor Stack 00113 * * Version TLV 00114 * * Provisioning URL TLV 00115 * 00116 * \param interface_id Network interface ID. The request comes from this ID. 00117 * \param EUI64 The client identifier. 00118 * \param message_ptr A message including the meshcop TLV set. This message can be parsed using thread_meshcop_lib.h. 00119 * \param message_len The length of the message. 00120 * 00121 * \return 0 Device accepted. 00122 * \return Any other value, device rejected. 00123 */ 00124 typedef int (thread_commissioning_joiner_finalisation_cb)(int8_t interface_id, uint8_t EUI64[8], uint8_t *message_ptr, uint16_t message_len); 00125 00126 /** \brief Add a device to commission to the Thread network. 00127 * 00128 * \param interface_id Network interface ID. The request comes from this ID. 00129 * \param short_eui64 A boolean value indicating that short EUI version is used for bloom filter generation. 00130 * \param EUI64 A pointer to EUI64 buffer. 00131 * \param PSKd_ptr A pointer to PSKd buffer. 00132 * \param PSKd_len PSKd string length, current validity check is 1-32 bytes. 00133 * \param joining_device_cb_ptr A callback function indicating the result of the operation. Can be NULL if no result code needed. 00134 * 00135 * \return 0 success, other values failure 00136 */ 00137 int thread_commissioning_device_add(int8_t interface_id, bool short_eui64, uint8_t EUI64[8], uint8_t *PSKd_ptr, uint8_t PSKd_len, thread_commissioning_joiner_finalisation_cb *joining_device_cb_ptr); 00138 00139 /** \brief Delete a device to commission to the Thread network. 00140 * 00141 * \param interface_id Network interface ID. The request comes from this ID. 00142 * \param EUI64 A pointer to EUI64 buffer. 00143 * 00144 * \return 0 success, other values failure. 00145 */ 00146 int thread_commissioning_device_delete(int8_t interface_id, uint8_t EUI64[8]); 00147 00148 /** \brief Get next added device details. 00149 * 00150 * \param ptr A pointer for internal looping. First, use NULL pointer, after that use return pointer. 00151 * \param interface_id Network interface ID. The request comes from this ID. 00152 * \param short_eui64 A boolean value indicating that short EUI version is used for bloom filter generation. Can be NULL when no result wanted. 00153 * \param EUI64 A pointer to EUI64 buffer. Can be NULL when no result wanted. 00154 * \param PSKd A pointer to PSKd buffer. Can be NULL when no result wanted. 00155 * \param PSKd_len Length of data in PSKd. 00156 * 00157 * \return >NULL for next iteration. 00158 * \return NULL when end of list. 00159 */ 00160 void *thread_commission_device_get_next(void *ptr, int8_t interface_id, bool *short_eui64, uint8_t EUI64[8], uint8_t PSKd[32], uint8_t *PSKd_len); 00161 00162 /** Interfaces needed for native commissioner. 00163 * current design: 00164 * - The application configures the interface to scan available Thread networks to join 00165 * - Time passes and the user wants to start scanning for native commissioner networks. 00166 * - The application configures the interface to begin native commissioner interface scans. 00167 * - The application selects a network to connect to. 00168 * - The stack connects to that network -> interface UP event sent. 00169 * - The application starts using Commissioning API to send COMM_PET.req message triggering a DTLS handshake. 00170 * - Commission API queries the leader address and native info and uses the one that works. 00171 * 00172 * 00173 */ 00174 00175 typedef struct thread_commissioning_link_configuration { 00176 uint8_t name[16]; /**< Name of the Thread network. utf8 string nul terminated if shorter than 16. */ 00177 uint8_t extented_pan_id[8]; /**< Extended PAN ID. */ 00178 uint16_t panId; /**< Network ID. */ 00179 uint8_t Protocol_id; /**< Current protocol ID. */ 00180 uint8_t version; /**< Current protocol version. */ 00181 uint8_t rfChannel; /**< Current RF channel. */ 00182 } thread_commissioning_link_configuration_s; 00183 00184 /** \brief Native commissioner network scan result callback. 00185 * 00186 * This callback is called when networks that allow native commissioner to join are found. 00187 * Pointers are valid during this call. 00188 * 00189 * \param interface_id Interface ID of this Thread instance. 00190 * \param count Count of link configurations 00191 * \param link_ptr Poiner to Commissioning link configuration 00192 * 00193 */ 00194 typedef void thread_commissioning_native_select_cb(int8_t interface_id, uint8_t count, thread_commissioning_link_configuration_s *link_ptr ); 00195 00196 /** \brief Native commissioner network scan start. 00197 * 00198 * Starts the network scan mode to find networks where the device can become a native commissioner. 00199 * This stops the normal Thread joining process and informs the application of available networks. 00200 * 00201 * \param interface_id Interface ID of this Thread instance. 00202 * \param cb_ptr Callback function 00203 * 00204 * \return 0 success, other values failure. 00205 */ 00206 int thread_commissioning_native_commissioner_start(int8_t interface_id, thread_commissioning_native_select_cb *cb_ptr); 00207 00208 /** \brief Native commissioner network scan stop. 00209 * 00210 * Stops the network scan mode and continues the normal joining process. 00211 * 00212 * \param interface_id Interface ID of this Thread instance. 00213 * 00214 * \return 0 success, other values failure. 00215 */ 00216 int thread_commissioning_native_commissioner_stop(int8_t interface_id); 00217 00218 /** \brief Native commissioner connect. 00219 * 00220 * Connects to a specific Thread network to become an active native commissioner. 00221 * 00222 * This function can be called in any time. When the network scan is completed, the available native commissioner networks are listed 00223 * using the callback. 00224 * 00225 * If the connection fails, network scan keeps looking for a new network. After a successful connection, the interface up event is sent. 00226 * TODO do we need backup timers or blacklist if PSKc fails. who is responsible for triggering new scans? 00227 * 00228 * Matching of thread network is made using Network name, Xpanid, panid, TODO channel?? or not? gives channel flexibility 00229 * 00230 * \param interface_id Interface ID of this Thread instance. 00231 * \param link_ptr Pointer to Commissioning link configuration. 00232 * 00233 * \return 0 success, other values failure. 00234 */ 00235 int thread_commissioning_native_commissioner_connect(int8_t interface_id, thread_commissioning_link_configuration_s *link_ptr); 00236 00237 /** 00238 *\brief Get the address of the native commissioner parent and the commissioning port for the connection. 00239 * 00240 * \param interface_id Network interface ID. 00241 * \param address_ptr A pointer to address buffer (16 bytes) for the commission messages. 00242 * \param port Return the port for the commissioner. 00243 * 00244 * \return 0, address OK. 00245 * \return <0 fail. 00246 */ 00247 int thread_commissioning_native_commissioner_get_connection_info(int8_t interface_id, uint8_t *address_ptr, uint16_t *port); 00248 00249 /** 00250 * \brief Get the management instance ID from the commissioner interface. 00251 * 00252 * \param interface_id Network interface ID. 00253 * 00254 * \return > 0 Instance ID. 00255 * \return <= 0 fail. 00256 */ 00257 int8_t thread_commissioning_get_management_id(int8_t interface_id); 00258 00259 #ifdef __cplusplus 00260 } 00261 #endif 00262 00263 #endif /* THREAD_COMMISSIONING_API_H_ */
Generated on Sun Jul 17 2022 08:25:32 by
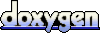