
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
test_randlib.c
00001 /* 00002 * Copyright (c) 2016 ARM. All rights reserved. 00003 */ 00004 #include "test_randlib.h" 00005 #include <string.h> 00006 #include <inttypes.h> 00007 #include "randLIB.h" 00008 00009 bool test_randLIB_seed_random() 00010 { 00011 randLIB_reset(); 00012 randLIB_seed_random(); 00013 return true; 00014 } 00015 00016 bool test_randLIB_get_8bit() 00017 { 00018 randLIB_reset(); 00019 randLIB_seed_random(); 00020 uint8_t test = randLIB_get_8bit(); 00021 if( test == 0 ) { 00022 test = randLIB_get_8bit(); 00023 if( test == 0 ) { 00024 return false; 00025 } 00026 } 00027 return true; 00028 } 00029 00030 bool test_randLIB_get_16bit() 00031 { 00032 randLIB_reset(); 00033 randLIB_seed_random(); 00034 uint16_t test = randLIB_get_16bit(); 00035 if( test == 0 ) { 00036 test = randLIB_get_16bit(); 00037 if( test == 0 ) { 00038 return false; 00039 } 00040 } 00041 return true; 00042 } 00043 00044 bool test_randLIB_get_32bit() 00045 { 00046 randLIB_reset(); 00047 randLIB_seed_random(); 00048 uint32_t test = randLIB_get_32bit(); 00049 if( test == 0 ) { 00050 test = randLIB_get_32bit(); 00051 if( test == 0 ) { 00052 return false; 00053 } 00054 } 00055 return true; 00056 } 00057 00058 static bool test_output(uint32_t seed, bool seed_inc, const uint64_t expected[8]) 00059 { 00060 random_stub_set_seed(seed, seed_inc); 00061 randLIB_reset(); 00062 randLIB_seed_random(); 00063 for (int i = 0; i < 8; i++) { 00064 if (randLIB_get_64bit() != expected[i]) { 00065 return false; 00066 } 00067 } 00068 00069 return true; 00070 } 00071 00072 bool test_randLIB_get_64bit() 00073 { 00074 /* Initial 8 xoroshiro128+ values with initial seed of 00075 * (0x0000000100000002, 0x00000003000000004). 00076 */ 00077 static const uint64_t expected1234[] = { 00078 UINT64_C(0x0000000400000006), 00079 UINT64_C(0x0100806200818026), 00080 UINT64_C(0x2a30826271904706), 00081 UINT64_C(0x918d7ea50109290d), 00082 UINT64_C(0x5dcbd701c1e1c64c), 00083 UINT64_C(0xaa129b152055f299), 00084 UINT64_C(0x4c95c2b1e1038a4d), 00085 UINT64_C(0x6479e7a3a75d865a) 00086 }; 00087 00088 if (!test_output(1, true, expected1234)) { 00089 goto fail; 00090 } 00091 00092 /* If passed all zero seeds, seeding should detect this 00093 * and use splitmix64 to create the actual seed 00094 * (0xe220a8397b1dcdaf, 0x6e789e6aa1b965f4), 00095 * and produce this output: 00096 */ 00097 static const uint64_t expected0000[] = { 00098 UINT64_C(0x509946a41cd733a3), 00099 UINT64_C(0x00885667b1934bfa), 00100 UINT64_C(0x1061f9ad258fd5d5), 00101 UINT64_C(0x3f8be44897a4317c), 00102 UINT64_C(0x60da683bea50e6ab), 00103 UINT64_C(0xd6b52f5379de1de0), 00104 UINT64_C(0x2608bc9fedc5b750), 00105 UINT64_C(0xb9fac9c7ec9de02a) 00106 }; 00107 00108 if (!test_output(0, false, expected0000)) { 00109 goto fail; 00110 } 00111 00112 /* If passed all "4" seeds, seeding should detect this 00113 * and use splitmix64 to create the actual seed 00114 * (0x03910b0eab9b37e1, 0x0b309ab53d42b2a2), 00115 * and produce this output: 00116 */ 00117 static const uint64_t expected4444[] = { 00118 UINT64_C(0x0ec1a5c3e8ddea83), 00119 UINT64_C(0x09e710b8faf5a491), 00120 UINT64_C(0xd4102776f79448b4), 00121 UINT64_C(0x5d61988b60091900), 00122 UINT64_C(0xf6c8a72a9c72cb4b), 00123 UINT64_C(0xb06923e0cf0f2fb1), 00124 UINT64_C(0x24bbed475153f573), 00125 UINT64_C(0xfff0b4bd08c5581f), 00126 }; 00127 00128 if (!test_output(4, false, expected4444)) { 00129 goto fail; 00130 } 00131 00132 /* Last test used constant seed of 4, which is the default */ 00133 return true; 00134 00135 fail: 00136 /* Put back the default seed of 4 (other tests might rely on it) */ 00137 random_stub_set_seed(4, false); 00138 return false; 00139 } 00140 00141 bool test_randLIB_get_n_bytes_random() 00142 { 00143 randLIB_reset(); 00144 randLIB_seed_random(); 00145 00146 uint8_t dat[5]; 00147 void *ret = randLIB_get_n_bytes_random(dat, 5); 00148 if(ret != dat){ 00149 return false; 00150 } 00151 00152 uint8_t dat2[5]; 00153 randLIB_get_n_bytes_random(dat2, 5); 00154 if (memcmp(dat, dat2, 5) == 0) { 00155 return false; 00156 } 00157 00158 return true; 00159 } 00160 00161 bool test_randLIB_get_random_in_range() 00162 { 00163 randLIB_reset(); 00164 randLIB_seed_random(); 00165 00166 uint16_t ret = randLIB_get_random_in_range(2, 2); 00167 if( ret != 2 ){ 00168 return false; 00169 } 00170 00171 ret = randLIB_get_random_in_range(2, 3); 00172 if( ret != 2 && ret != 3){ 00173 return false; 00174 } 00175 00176 ret = randLIB_get_random_in_range(0, 0xFFFF); 00177 00178 return true; 00179 } 00180 00181 bool test_randLIB_randomise_base() 00182 { 00183 randLIB_reset(); 00184 randLIB_seed_random(); 00185 00186 uint32_t ret = randLIB_randomise_base(0,0,0); 00187 if( ret ){ 00188 return false; 00189 } 00190 ret = randLIB_randomise_base(0xffff0000,0x8888,0x8888); 00191 if( ret != 0xffffffff ){ 00192 return false; 00193 } 00194 return true; 00195 }
Generated on Sun Jul 17 2022 08:25:32 by
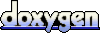