
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
test_coap_service_api.c
00001 /* 00002 * Copyright (c) 2015-2017 ARM Limited. All Rights Reserved. 00003 */ 00004 #include "test_coap_service_api.h" 00005 #include <string.h> 00006 #include "coap_service_api.h" 00007 #include "nsdynmemLIB_stub.h" 00008 #include "coap_connection_handler_stub.h" 00009 #include "coap_message_handler_stub.h" 00010 #include "eventOS_event_stub.h" 00011 #include "eventOS_event.h" 00012 #include "net_interface.h" 00013 00014 int sec_done_cb(int8_t service_id, uint8_t address[static 16], uint8_t keyblock[static 40]){ 00015 return 2; 00016 } 00017 00018 int sec_start_cb(int8_t service_id, uint8_t address[static 16], uint16_t port, uint8_t* pw, uint8_t *pw_len) 00019 { 00020 return 2; 00021 } 00022 00023 int request_recv_cb(int8_t service_id, uint8_t source_address[static 16], uint16_t source_port, sn_coap_hdr_s *request_ptr) 00024 { 00025 return 2; 00026 } 00027 00028 int virtual_sock_send_cb(int8_t service_id, uint8_t destination_addr_ptr[static 16], uint16_t port, const uint8_t *data_ptr, uint16_t data_len) 00029 { 00030 return 2; 00031 } 00032 00033 bool test_coap_service_initialize() 00034 { 00035 if( -1 != coap_service_initialize(1, 2, 0, NULL, NULL )) 00036 return false; 00037 00038 00039 nsdynmemlib_stub.returnCounter = 1; 00040 thread_conn_handler_stub.handler_obj = NULL; 00041 if( -1 != coap_service_initialize(1, 2, 0, NULL, NULL )) 00042 return false; 00043 00044 thread_conn_handler_stub.handler_obj = (coap_conn_handler_t*)malloc(sizeof(coap_conn_handler_t)); 00045 memset(thread_conn_handler_stub.handler_obj, 0, sizeof(coap_conn_handler_t)); 00046 coap_message_handler_stub.coap_ptr = NULL; 00047 00048 nsdynmemlib_stub.returnCounter = 1; 00049 thread_conn_handler_stub.int_value = -1; 00050 if( -1 != coap_service_initialize(1, 2, 0, NULL, NULL )) 00051 return false; 00052 00053 thread_conn_handler_stub.handler_obj = (coap_conn_handler_t*)malloc(sizeof(coap_conn_handler_t)); 00054 memset(thread_conn_handler_stub.handler_obj, 0, sizeof(coap_conn_handler_t)); 00055 nsdynmemlib_stub.returnCounter = 1; 00056 thread_conn_handler_stub.int_value = 0; 00057 00058 if( 1 != coap_service_initialize(1, 2, 0, NULL, NULL )) 00059 return false; 00060 00061 nsdynmemlib_stub.returnCounter = 1; 00062 if( 2 != coap_service_initialize(3, 4, 0, NULL, NULL )) 00063 return false; 00064 00065 coap_service_delete(2); 00066 coap_service_delete(1); 00067 00068 free( thread_conn_handler_stub.handler_obj ); 00069 thread_conn_handler_stub.handler_obj = NULL; 00070 return true; 00071 } 00072 00073 bool test_coap_service_delete() 00074 { 00075 coap_service_delete(1); 00076 00077 thread_conn_handler_stub.handler_obj = (coap_conn_handler_t*)malloc(sizeof(coap_conn_handler_t)); 00078 memset(thread_conn_handler_stub.handler_obj, 0, sizeof(coap_conn_handler_t)); 00079 nsdynmemlib_stub.returnCounter = 1; 00080 coap_message_handler_stub.coap_ptr = NULL; 00081 00082 if( 1 != coap_service_initialize(1, 2, 0, NULL, NULL )) 00083 return false; 00084 00085 coap_service_delete(1); 00086 00087 free( thread_conn_handler_stub.handler_obj ); 00088 thread_conn_handler_stub.handler_obj = NULL; 00089 00090 return true; 00091 } 00092 00093 bool test_coap_service_virtual_socket_recv() 00094 { 00095 uint8_t buf[16]; 00096 if( -1 != coap_service_virtual_socket_recv(1, &buf, 10, NULL, 0) ) 00097 return false; 00098 00099 thread_conn_handler_stub.handler_obj = (coap_conn_handler_t*)malloc(sizeof(coap_conn_handler_t)); 00100 memset(thread_conn_handler_stub.handler_obj, 0, sizeof(coap_conn_handler_t)); 00101 nsdynmemlib_stub.returnCounter = 1; 00102 coap_message_handler_stub.coap_ptr = NULL; 00103 00104 if( 1 != coap_service_initialize(1, 2, 0, NULL, NULL )) 00105 return false; 00106 00107 thread_conn_handler_stub.int_value = 5; 00108 if( 5 != coap_service_virtual_socket_recv(1, &buf, 10, NULL, 0) ) 00109 return false; 00110 00111 coap_service_delete(1); 00112 00113 free( thread_conn_handler_stub.handler_obj ); 00114 thread_conn_handler_stub.handler_obj = NULL; 00115 00116 thread_conn_handler_stub.int_value = 0; 00117 00118 return true; 00119 } 00120 00121 bool test_coap_service_virtual_socket_set_cb() 00122 { 00123 if( -1 != coap_service_virtual_socket_set_cb(1, NULL) ) 00124 return false; 00125 00126 thread_conn_handler_stub.handler_obj = (coap_conn_handler_t*)malloc(sizeof(coap_conn_handler_t)); 00127 memset(thread_conn_handler_stub.handler_obj, 0, sizeof(coap_conn_handler_t)); 00128 nsdynmemlib_stub.returnCounter = 1; 00129 coap_message_handler_stub.coap_ptr = NULL; 00130 00131 if( 1 != coap_service_initialize(1, 2, 0, NULL, NULL )) 00132 return false; 00133 00134 if( 0 != coap_service_virtual_socket_set_cb(1, NULL) ) 00135 return false; 00136 00137 coap_service_delete(1); 00138 00139 free( thread_conn_handler_stub.handler_obj ); 00140 thread_conn_handler_stub.handler_obj = NULL; 00141 00142 return true; 00143 } 00144 00145 bool test_coap_service_register_uri() 00146 { 00147 if( -1 != coap_service_register_uri(1, "as", 1, &request_recv_cb)) 00148 return false; 00149 00150 thread_conn_handler_stub.handler_obj = (coap_conn_handler_t*)malloc(sizeof(coap_conn_handler_t)); 00151 memset(thread_conn_handler_stub.handler_obj, 0, sizeof(coap_conn_handler_t)); 00152 nsdynmemlib_stub.returnCounter = 1; 00153 coap_message_handler_stub.coap_ptr = NULL; 00154 00155 if( 1 != coap_service_initialize(1, 2, 0, NULL, NULL )) 00156 return false; 00157 00158 if( -2 != coap_service_register_uri(1, "as", 1, &request_recv_cb) ) 00159 return false; 00160 00161 nsdynmemlib_stub.returnCounter = 1; 00162 if( -2 != coap_service_register_uri(1, "as", 1, &request_recv_cb) ) 00163 return false; 00164 00165 nsdynmemlib_stub.returnCounter = 2; 00166 if( 0 != coap_service_register_uri(1, "as", 1, &request_recv_cb) ) 00167 return false; 00168 00169 coap_service_delete(1); 00170 00171 free( thread_conn_handler_stub.handler_obj ); 00172 thread_conn_handler_stub.handler_obj = NULL; 00173 00174 return true; 00175 } 00176 00177 bool test_coap_service_unregister_uri() 00178 { 00179 if( -1 != coap_service_unregister_uri(1, "as")) 00180 return false; 00181 00182 thread_conn_handler_stub.handler_obj = (coap_conn_handler_t*)malloc(sizeof(coap_conn_handler_t)); 00183 memset(thread_conn_handler_stub.handler_obj, 0, sizeof(coap_conn_handler_t)); 00184 nsdynmemlib_stub.returnCounter = 1; 00185 coap_message_handler_stub.coap_ptr = NULL; 00186 thread_conn_handler_stub.int_value = 0; 00187 00188 if( 1 != coap_service_initialize(1, 2, 0, NULL, NULL )) 00189 return false; 00190 00191 nsdynmemlib_stub.returnCounter = 2; 00192 if( 0 != coap_service_register_uri(1, "as", 1, &request_recv_cb) ) 00193 return false; 00194 00195 if( -2 != coap_service_unregister_uri(1, "ts") ) 00196 return false; 00197 00198 if( 0 != coap_service_unregister_uri(1, "as") ) 00199 return false; 00200 00201 coap_service_delete(1); 00202 00203 free( thread_conn_handler_stub.handler_obj ); 00204 thread_conn_handler_stub.handler_obj = NULL; 00205 00206 return true; 00207 } 00208 00209 bool test_coap_service_request_send() 00210 { 00211 uint8_t buf[16]; 00212 coap_message_handler_stub.uint16_value = 6; 00213 if( 6 != coap_service_request_send(0,0,&buf,0,0,0,NULL, 0,NULL,0,NULL)) 00214 return false; 00215 return true; 00216 } 00217 00218 bool test_coap_service_request_delete() 00219 { 00220 if( 0 != coap_service_request_delete(NULL,0)) 00221 return false; 00222 return true; 00223 } 00224 00225 bool test_coap_service_response_send() 00226 { 00227 uint8_t buf[16]; 00228 coap_message_handler_stub.int8_value = 6; 00229 if( 6 != coap_service_response_send(0,0,NULL, 65, 0,NULL, 0)) 00230 return false; 00231 return true; 00232 } 00233 00234 bool test_coap_callbacks() 00235 { 00236 thread_conn_handler_stub.handler_obj = (coap_conn_handler_t*)malloc(sizeof(coap_conn_handler_t)); 00237 memset(thread_conn_handler_stub.handler_obj, 0, sizeof(coap_conn_handler_t)); 00238 nsdynmemlib_stub.returnCounter = 1; 00239 coap_message_handler_stub.coap_ptr = (coap_msg_handler_t *)malloc(sizeof(coap_msg_handler_t)); 00240 memset(coap_message_handler_stub.coap_ptr, 0, sizeof(coap_msg_handler_t)); 00241 00242 if( 1 != coap_service_initialize(1, 2, 0, NULL, NULL )) 00243 return false; 00244 00245 if( 0 != coap_message_handler_stub.coap_ptr->sn_coap_service_malloc(0)) 00246 return false; 00247 00248 nsdynmemlib_stub.returnCounter = 1; 00249 void *handle = coap_message_handler_stub.coap_ptr->sn_coap_service_malloc(5); 00250 if( 0 == handle ) 00251 return false; 00252 00253 coap_message_handler_stub.coap_ptr->sn_coap_service_free(handle); 00254 00255 //coap_tx_function 00256 uint8_t data[14]; 00257 memset(&data, 3, 14); 00258 sn_nsdl_addr_s addr; 00259 addr.addr_len = 2; 00260 addr.port = 4; 00261 addr.addr_ptr = &data; 00262 if( 0 != coap_message_handler_stub.coap_ptr->sn_coap_tx_callback(NULL, 0, &addr, NULL)) 00263 return false; 00264 00265 coap_transaction_t *tr = (coap_transaction_t *)malloc(sizeof(coap_transaction_t)); 00266 memset(tr, 0, sizeof(coap_transaction_t)); 00267 00268 if( 0 != coap_message_handler_stub.coap_ptr->sn_coap_tx_callback(&data, 0, &addr, tr)) 00269 return false; 00270 00271 tr->service_id = 1; 00272 thread_conn_handler_stub.int_value = -2; 00273 if( 0 != coap_message_handler_stub.coap_ptr->sn_coap_tx_callback(&data, 0, &addr, tr)) 00274 return false; 00275 00276 nsdynmemlib_stub.returnCounter = 1; 00277 if( 0 != coap_message_handler_stub.coap_ptr->sn_coap_tx_callback(&data, 2, &addr, tr)) 00278 return false; 00279 00280 free(tr->data_ptr); 00281 free(tr); 00282 00283 coap_service_delete(1); 00284 00285 free( coap_message_handler_stub.coap_ptr ); 00286 coap_message_handler_stub.coap_ptr = NULL; 00287 00288 free( thread_conn_handler_stub.handler_obj ); 00289 thread_conn_handler_stub.handler_obj = NULL; 00290 00291 return true; 00292 } 00293 00294 #define COAP_TICK_TIMER 0xf1 //MUST BE SAME AS IN coap_service_api.c 00295 bool test_eventOS_callbacks() 00296 { 00297 thread_conn_handler_stub.handler_obj = (coap_conn_handler_t*)malloc(sizeof(coap_conn_handler_t)); 00298 memset(thread_conn_handler_stub.handler_obj, 0, sizeof(coap_conn_handler_t)); 00299 nsdynmemlib_stub.returnCounter = 1; 00300 if( 1 != coap_service_initialize(1, 2, 0, NULL, NULL )) 00301 return false; 00302 00303 if( eventOs_event_stub.event_ptr ){ 00304 arm_event_s event; 00305 event.event_type = ARM_LIB_TASKLET_INIT_EVENT; 00306 eventOs_event_stub.event_ptr(&event); 00307 00308 event.event_type = ARM_LIB_SYSTEM_TIMER_EVENT; 00309 event.event_id = COAP_TICK_TIMER; 00310 eventOs_event_stub.event_ptr(&event); 00311 } 00312 00313 coap_service_delete(1); 00314 free( thread_conn_handler_stub.handler_obj ); 00315 thread_conn_handler_stub.handler_obj = NULL; 00316 return true; 00317 } 00318 00319 bool test_conn_handler_callbacks() 00320 { 00321 uint8_t buf[16]; 00322 thread_conn_handler_stub.handler_obj = (coap_conn_handler_t*)malloc(sizeof(coap_conn_handler_t)); 00323 memset(thread_conn_handler_stub.handler_obj, 0, sizeof(coap_conn_handler_t)); 00324 nsdynmemlib_stub.returnCounter = 1; 00325 if( 1 != coap_service_initialize(1, 2, COAP_SERVICE_OPTIONS_SECURE_BYPASS, &sec_start_cb, &sec_done_cb )) 00326 return false; 00327 00328 if( thread_conn_handler_stub.send_to_sock_cb ){ 00329 thread_conn_handler_stub.bool_value = true; 00330 coap_service_virtual_socket_set_cb(1, &virtual_sock_send_cb); 00331 if( 2 != thread_conn_handler_stub.send_to_sock_cb(1, buf, 12, NULL, 0)) 00332 return false; 00333 thread_conn_handler_stub.bool_value = false; 00334 if( -1 != thread_conn_handler_stub.send_to_sock_cb(1, buf, 12, NULL, 0)) 00335 return false; 00336 } 00337 00338 if( thread_conn_handler_stub.receive_from_sock_cb ){ 00339 coap_message_handler_stub.int16_value = 2; 00340 if( -1 != thread_conn_handler_stub.receive_from_sock_cb(1, buf, 12, NULL, NULL, 0)) 00341 return false; 00342 00343 nsdynmemlib_stub.returnCounter = 1; 00344 uint8_t * ptr = ns_dyn_mem_alloc(5); 00345 memset(ptr, 3, 5); 00346 nsdynmemlib_stub.returnCounter = 1; 00347 if( 2 != thread_conn_handler_stub.receive_from_sock_cb(1, buf, 12, NULL, ptr, 5)) 00348 return false; 00349 ns_dyn_mem_free(ptr); 00350 coap_message_handler_stub.int16_value = 0; 00351 00352 //This could be moved to own test function, 00353 //but thread_conn_handler_stub.receive_from_sock_cb must be called successfully 00354 if( coap_message_handler_stub.cb ){ 00355 if( -1 != coap_message_handler_stub.cb(1, NULL, NULL) ) 00356 return false; 00357 00358 sn_coap_hdr_s * coap = (sn_coap_hdr_s *)malloc(sizeof(sn_coap_hdr_s)); 00359 memset(coap, 0, sizeof(sn_coap_hdr_s)); 00360 00361 uint8_t uri[2] = "as"; 00362 coap->uri_path_ptr = &uri; 00363 coap->uri_path_len=2; 00364 00365 if( -1 != coap_message_handler_stub.cb(1, coap, NULL) ) 00366 return false; 00367 00368 thread_conn_handler_stub.bool_value = true; 00369 nsdynmemlib_stub.returnCounter = 2; 00370 if( 0 != coap_service_register_uri(1, "as", 1, &request_recv_cb) ) 00371 return false; 00372 00373 if( -1 != coap_message_handler_stub.cb(1, coap, NULL) ) 00374 return false; 00375 00376 coap_transaction_t *tr = (coap_transaction_t *)malloc(sizeof(coap_transaction_t)); 00377 memset(tr, 0, sizeof(coap_transaction_t)); 00378 00379 if( 2 != coap_message_handler_stub.cb(1, coap, tr) ) 00380 return false; 00381 00382 free(tr); 00383 tr = NULL; 00384 00385 thread_conn_handler_stub.bool_value = false; 00386 free(coap); 00387 coap = NULL; 00388 } 00389 } 00390 00391 if(thread_conn_handler_stub.get_passwd_cb){ 00392 thread_conn_handler_stub.bool_value = true; 00393 if( 2 != thread_conn_handler_stub.get_passwd_cb(1, buf, 12, NULL, 0)) 00394 return false; 00395 thread_conn_handler_stub.bool_value = false; 00396 if( -1 != thread_conn_handler_stub.get_passwd_cb(1, buf, 12, NULL, 0)) 00397 return false; 00398 } 00399 00400 if(thread_conn_handler_stub.sec_done_cb){ 00401 uint8_t block[40]; 00402 thread_conn_handler_stub.bool_value = true; 00403 00404 coap_transaction_t *tr = (coap_transaction_t *)malloc(sizeof(coap_transaction_t)); 00405 memset(tr, 0, sizeof(coap_transaction_t)); 00406 nsdynmemlib_stub.returnCounter = 1; 00407 tr->data_ptr = ns_dyn_mem_alloc(1); 00408 tr->data_len = 1; 00409 coap_message_handler_stub.coap_tx_ptr = tr; 00410 00411 thread_conn_handler_stub.sec_done_cb(1, buf, 12, block); 00412 00413 free(tr); 00414 coap_message_handler_stub.coap_tx_ptr = NULL; 00415 00416 thread_conn_handler_stub.bool_value = false; 00417 00418 } 00419 00420 coap_service_delete(1); 00421 free( thread_conn_handler_stub.handler_obj ); 00422 thread_conn_handler_stub.handler_obj = NULL; 00423 00424 return true; 00425 }
Generated on Sun Jul 17 2022 08:25:32 by
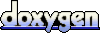