
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
test_coap_security_handler.c
00001 /* 00002 * Copyright (c) 2015-2017 ARM Limited. All Rights Reserved. 00003 */ 00004 #include "test_coap_security_handler.h" 00005 #include "coap_security_handler.h" 00006 #include <string.h> 00007 #include "nsdynmemLIB_stub.h" 00008 #include "mbedtls_stub.h" 00009 #include "mbedtls/ssl.h" 00010 00011 static int send_to_socket(int8_t socket_id, void *handle, const unsigned char *buf, size_t len) 00012 { 00013 00014 } 00015 00016 static int receive_from_socket(int8_t socket_id, unsigned char *buf, size_t len) 00017 { 00018 00019 } 00020 00021 static void start_timer_callback(int8_t timer_id, uint32_t int_ms, uint32_t fin_ms) 00022 { 00023 00024 } 00025 00026 static int timer_status_callback(int8_t timer_id) 00027 { 00028 00029 } 00030 00031 bool test_thread_security_create() 00032 { 00033 if( NULL != coap_security_create(1,2,NULL,ECJPAKE,&send_to_socket, &receive_from_socket, &start_timer_callback, NULL) ) 00034 return false; 00035 00036 if( NULL != coap_security_create(1,2,NULL,ECJPAKE,&send_to_socket, &receive_from_socket, &start_timer_callback, &timer_status_callback) ) 00037 return false; 00038 00039 nsdynmemlib_stub.returnCounter = 1; 00040 mbedtls_stub.expected_int = -1; 00041 if( NULL != coap_security_create(1,2,NULL,ECJPAKE,&send_to_socket, &receive_from_socket, &start_timer_callback, &timer_status_callback) ) 00042 return false; 00043 00044 mbedtls_stub.expected_int = 0; 00045 nsdynmemlib_stub.returnCounter = 2; 00046 mbedtls_stub.crt_expected_int = -1; 00047 if( NULL != coap_security_create(1,2,NULL,ECJPAKE,&send_to_socket, &receive_from_socket, &start_timer_callback, &timer_status_callback) ) 00048 return false; 00049 00050 nsdynmemlib_stub.returnCounter = 2; 00051 mbedtls_stub.crt_expected_int = 0; 00052 coap_security_t *handle = coap_security_create(1,2,NULL,ECJPAKE,&send_to_socket, &receive_from_socket, &start_timer_callback, &timer_status_callback); 00053 if( NULL == handle ) 00054 return false; 00055 00056 coap_security_destroy(handle); 00057 00058 return true; 00059 } 00060 00061 bool test_thread_security_destroy() 00062 { 00063 nsdynmemlib_stub.returnCounter = 2; 00064 mbedtls_stub.crt_expected_int = 0; 00065 coap_security_t *handle = coap_security_create(1,2,NULL,ECJPAKE,&send_to_socket, &receive_from_socket, &start_timer_callback, &timer_status_callback); 00066 if( NULL == handle ) 00067 return false; 00068 00069 coap_security_destroy(handle); 00070 return true; 00071 } 00072 00073 bool test_coap_security_handler_connect() 00074 { 00075 nsdynmemlib_stub.returnCounter = 2; 00076 mbedtls_stub.crt_expected_int = 0; 00077 coap_security_t *handle = coap_security_create(1,2,NULL,ECJPAKE,&send_to_socket, &receive_from_socket, &start_timer_callback, &timer_status_callback); 00078 if( NULL == handle ) 00079 return false; 00080 00081 unsigned char pw = "pwd"; 00082 coap_security_keys_t keys; 00083 keys._priv = &pw; 00084 keys._priv_len = 3; 00085 if( -1 != coap_security_handler_connect_non_blocking(NULL, true, DTLS, keys, 0, 1) ) 00086 return false; 00087 mbedtls_stub.useCounter = true; 00088 mbedtls_stub.counter = 0; 00089 mbedtls_stub.retArray[0] = -1; 00090 mbedtls_stub.retArray[1] = -1; 00091 mbedtls_stub.retArray[2] = -1; 00092 mbedtls_stub.retArray[3] = -1; 00093 mbedtls_stub.retArray[4] = -1; 00094 mbedtls_stub.retArray[5] = MBEDTLS_ERR_SSL_HELLO_VERIFY_REQUIRED; 00095 mbedtls_stub.retArray[6] = -1; 00096 mbedtls_stub.retArray[7] = -1; 00097 00098 if( -1 != coap_security_handler_connect_non_blocking(handle, true, DTLS, keys, 0, 1) ) 00099 return false; 00100 00101 mbedtls_stub.counter = 0; 00102 mbedtls_stub.retArray[0] = 0; 00103 if( -1 != coap_security_handler_connect_non_blocking(handle, true, DTLS, keys, 0, 1) ) 00104 return false; 00105 00106 mbedtls_stub.counter = 0; 00107 // mbedtls_stub.retArray[0] = 0; 00108 mbedtls_stub.retArray[1] = 0; 00109 if( -1 != coap_security_handler_connect_non_blocking(handle, true, DTLS, keys, 0, 1) ) 00110 return false; 00111 00112 simple_cookie_t c; 00113 memset(&c, 0, sizeof(simple_cookie_t)); 00114 mbedtls_stub.cookie_obj = &c; 00115 memset(&mbedtls_stub.cookie_value, 1, 8); 00116 mbedtls_stub.cookie_len = 2; 00117 mbedtls_stub.counter = 0; 00118 // mbedtls_stub.retArray[0] = 0; 00119 // mbedtls_stub.retArray[1] = 0; 00120 mbedtls_stub.retArray[2] = 0; 00121 if( -1 != coap_security_handler_connect_non_blocking(handle, true, DTLS, keys, 0, 1) ) 00122 return false; 00123 00124 c.len = 8; 00125 memset(&c.value, 1, 8); 00126 mbedtls_stub.cookie_obj = &c; 00127 memset(&mbedtls_stub.cookie_value, 1, 8); 00128 00129 mbedtls_stub.cookie_len = 8; 00130 mbedtls_stub.counter = 0; 00131 // mbedtls_stub.retArray[0] = 0; 00132 // mbedtls_stub.retArray[1] = 0; 00133 // mbedtls_stub.retArray[2] = 0; 00134 mbedtls_stub.retArray[3] = 0; 00135 if( -1 != coap_security_handler_connect_non_blocking(handle, true, DTLS, keys, 0, 1) ) 00136 return false; 00137 00138 mbedtls_stub.counter = 0; 00139 // mbedtls_stub.retArray[0] = 0; 00140 // mbedtls_stub.retArray[1] = 0; 00141 // mbedtls_stub.retArray[2] = 0; 00142 // mbedtls_stub.retArray[3] = 0; 00143 mbedtls_stub.retArray[4] = 0; 00144 if( -1 != coap_security_handler_connect_non_blocking(handle, true, DTLS, keys, 0, 1) ) 00145 return false; 00146 00147 mbedtls_stub.counter = 0; 00148 // mbedtls_stub.retArray[0] = 0; 00149 // mbedtls_stub.retArray[1] = 0; 00150 // mbedtls_stub.retArray[2] = 0; 00151 // mbedtls_stub.retArray[3] = 0; 00152 // mbedtls_stub.retArray[4] = 0; 00153 mbedtls_stub.retArray[6] = 0; 00154 mbedtls_stub.retArray[7] = 0; 00155 if( 1 != coap_security_handler_connect_non_blocking(handle, true, DTLS, keys, 0, 1) ) 00156 return false; 00157 00158 mbedtls_stub.counter = 0; 00159 mbedtls_stub.retArray[5] = MBEDTLS_ERR_SSL_BAD_HS_FINISHED; 00160 00161 if( -1 != coap_security_handler_connect_non_blocking(handle, true, DTLS, keys, 0, 1) ) 00162 return false; 00163 00164 mbedtls_stub.counter = 0; 00165 mbedtls_stub.retArray[5] = HANDSHAKE_FINISHED_VALUE; 00166 00167 if( 1 != coap_security_handler_connect_non_blocking(handle, true, DTLS, keys, 0, 1) ) 00168 return false; 00169 00170 coap_security_destroy(handle); 00171 return true; 00172 } 00173 00174 bool test_coap_security_handler_continue_connecting() 00175 { 00176 nsdynmemlib_stub.returnCounter = 2; 00177 mbedtls_stub.crt_expected_int = 0; 00178 coap_security_t *handle = coap_security_create(1,2,NULL,ECJPAKE,&send_to_socket, &receive_from_socket, &start_timer_callback, &timer_status_callback); 00179 if( NULL == handle ) 00180 return false; 00181 00182 mbedtls_stub.useCounter = true; 00183 mbedtls_stub.counter = 0; 00184 mbedtls_stub.retArray[0] = MBEDTLS_ERR_SSL_HELLO_VERIFY_REQUIRED; 00185 mbedtls_stub.retArray[1] = -1; 00186 mbedtls_stub.retArray[2] = -1; 00187 00188 if( -1 != coap_security_handler_continue_connecting(handle) ) 00189 return false; 00190 00191 mbedtls_stub.counter = 0; 00192 mbedtls_stub.retArray[0] = MBEDTLS_ERR_SSL_HELLO_VERIFY_REQUIRED; 00193 mbedtls_stub.retArray[1] = 0; 00194 mbedtls_stub.retArray[2] = 0; 00195 00196 if( 1 != coap_security_handler_continue_connecting(handle) ) 00197 return false; 00198 00199 mbedtls_stub.counter = 0; 00200 mbedtls_stub.retArray[0] = MBEDTLS_ERR_SSL_BAD_HS_FINISHED; 00201 00202 if( MBEDTLS_ERR_SSL_BAD_HS_FINISHED != coap_security_handler_continue_connecting(handle) ) 00203 return false; 00204 00205 mbedtls_stub.counter = 0; 00206 mbedtls_stub.retArray[0] = MBEDTLS_ERR_SSL_WANT_READ; 00207 00208 if( 1 != coap_security_handler_continue_connecting(handle) ) 00209 return false; 00210 00211 mbedtls_stub.counter = 0; 00212 mbedtls_stub.retArray[0] = HANDSHAKE_FINISHED_VALUE_RETURN_ZERO; 00213 00214 if( 0 != coap_security_handler_continue_connecting(handle) ) 00215 return false; 00216 00217 coap_security_destroy(handle); 00218 return true; 00219 } 00220 00221 bool test_coap_security_handler_send_message() 00222 { 00223 nsdynmemlib_stub.returnCounter = 2; 00224 mbedtls_stub.crt_expected_int = 0; 00225 coap_security_t *handle = coap_security_create(1,2,NULL,ECJPAKE,&send_to_socket, &receive_from_socket, &start_timer_callback, &timer_status_callback); 00226 if( NULL == handle ) 00227 return false; 00228 00229 if( -1 != coap_security_handler_send_message(NULL, NULL, 0)) 00230 return false; 00231 00232 mbedtls_stub.expected_int = 6; 00233 unsigned char cbuf[6]; 00234 if( 6 != coap_security_handler_send_message(handle, &cbuf, 6)) 00235 return false; 00236 00237 coap_security_destroy(handle); 00238 return true; 00239 } 00240 00241 bool test_thread_security_send_close_alert() 00242 { 00243 nsdynmemlib_stub.returnCounter = 2; 00244 mbedtls_stub.crt_expected_int = 0; 00245 coap_security_t *handle = coap_security_create(1,2,NULL,ECJPAKE,&send_to_socket, &receive_from_socket, &start_timer_callback, &timer_status_callback); 00246 if( NULL == handle ) 00247 return false; 00248 00249 if( -1 != coap_security_send_close_alert(NULL)) 00250 return false; 00251 00252 mbedtls_stub.expected_int = 0; 00253 if( 0 != coap_security_send_close_alert(handle)) 00254 return false; 00255 00256 coap_security_destroy(handle); 00257 return true; 00258 } 00259 00260 bool test_coap_security_handler_read() 00261 { 00262 nsdynmemlib_stub.returnCounter = 2; 00263 mbedtls_stub.crt_expected_int = 0; 00264 coap_security_t *handle = coap_security_create(1,2,NULL,ECJPAKE,&send_to_socket, &receive_from_socket, &start_timer_callback, &timer_status_callback); 00265 if( NULL == handle ) 00266 return false; 00267 00268 if( -1 != coap_security_handler_read(NULL, NULL, 0)) 00269 return false; 00270 00271 mbedtls_stub.expected_int = 6; 00272 unsigned char cbuf[6]; 00273 if( 6 != coap_security_handler_read(handle, &cbuf, 6)) 00274 return false; 00275 00276 coap_security_destroy(handle); 00277 return true; 00278 } 00279
Generated on Sun Jul 17 2022 08:25:32 by
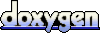