
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
test_c.c
00001 #include "mbed_assert.h" 00002 #define THE_ANSWER 42 00003 00004 // Tests for static asserts in different contexts 00005 // multiple asserts are used to garuntee no conflicts occur in generated labels 00006 00007 // Test for static asserts in global context 00008 MBED_STATIC_ASSERT(sizeof(int) >= sizeof(char), 00009 "An int must be larger than char"); 00010 MBED_STATIC_ASSERT(2 + 2 == 4, 00011 "Hopefully the universe is mathematically consistent"); 00012 MBED_STATIC_ASSERT(THE_ANSWER == 42, 00013 "Said Deep Thought, with infinite majesty and calm"); 00014 00015 struct test { 00016 int dummy; 00017 00018 // Test for static asserts in struct context 00019 MBED_STRUCT_STATIC_ASSERT(sizeof(int) >= sizeof(char), 00020 "An int must be larger than char"); 00021 MBED_STRUCT_STATIC_ASSERT(2 + 2 == 4, 00022 "Hopefully the universe is mathematically consistent"); 00023 MBED_STRUCT_STATIC_ASSERT(THE_ANSWER == 42, 00024 "Said Deep Thought, with infinite majesty and calm"); 00025 }; 00026 00027 MBED_STATIC_ASSERT(sizeof(struct test) == sizeof(int), 00028 "Static assertions should not change the size of a struct"); 00029 00030 void doit_c(void) { 00031 // Test for static asserts in function context 00032 MBED_STATIC_ASSERT(sizeof(int) >= sizeof(char), 00033 "An int must be larger than char"); 00034 MBED_STATIC_ASSERT(2 + 2 == 4, 00035 "Hopefully the universe is mathematically consistent"); 00036 MBED_STATIC_ASSERT(THE_ANSWER == 42, 00037 "Said Deep Thought, with infinite majesty and calm"); 00038 } 00039
Generated on Sun Jul 17 2022 08:25:32 by
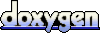