
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
test_api_test.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2016 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 00018 import pytest 00019 from mock import patch 00020 from tools.targets import set_targets_json_location 00021 from tools.test_api import find_tests, build_tests 00022 00023 """ 00024 Tests for test_api.py 00025 """ 00026 00027 def setUp (self): 00028 """ 00029 Called before each test case 00030 00031 :return: 00032 """ 00033 self.base_dir = 'base_dir' 00034 self.target = "K64F" 00035 self.toolchain_name = "ARM" 00036 00037 @pytest.mark.parametrize("base_dir", ["base_dir"]) 00038 @pytest.mark.parametrize("target", ["K64F"]) 00039 @pytest.mark.parametrize("toolchain_name", ["ARM"]) 00040 @pytest.mark.parametrize("app_config", ["app_config", None]) 00041 def test_find_tests_app_config (base_dir, target, toolchain_name, app_config): 00042 """ 00043 Test find_tests for correct use of app_config 00044 00045 :param base_dir: dummy value for the test base directory 00046 :param target: the target to "test" for 00047 :param toolchain_name: the toolchain to use for "testing" 00048 :param app_config: Application configuration parameter to find tests 00049 """ 00050 set_targets_json_location() 00051 with patch('tools.test_api.scan_resources') as mock_scan_resources,\ 00052 patch('tools.test_api.prepare_toolchain') as mock_prepare_toolchain: 00053 mock_scan_resources().inc_dirs.return_value = [] 00054 00055 find_tests(base_dir, target, toolchain_name, app_config=app_config) 00056 00057 args = mock_prepare_toolchain.call_args 00058 assert 'app_config' in args[1],\ 00059 "prepare_toolchain was not called with app_config" 00060 assert args[1]['app_config'] == app_config,\ 00061 "prepare_toolchain was called with an incorrect app_config" 00062 00063 00064 @pytest.mark.parametrize("build_path", ["build_path"]) 00065 @pytest.mark.parametrize("target", ["K64F"]) 00066 @pytest.mark.parametrize("toolchain_name", ["ARM"]) 00067 @pytest.mark.parametrize("app_config", ["app_config", None]) 00068 def test_find_tests_app_config (build_path, target, toolchain_name, app_config): 00069 """ 00070 Test find_tests for correct use of app_config 00071 00072 :param base_dir: dummy value for the test base directory 00073 :param target: the target to "test" for 00074 :param toolchain_name: the toolchain to use for "testing" 00075 :param app_config: Application configuration parameter to find tests 00076 """ 00077 tests = {'test1': 'test1_path','test2': 'test2_path'} 00078 src_paths = ['.'] 00079 set_targets_json_location() 00080 with patch('tools.test_api.scan_resources') as mock_scan_resources,\ 00081 patch('tools.test_api.build_project') as mock_build_project: 00082 mock_build_project.return_value = "build_project" 00083 mock_scan_resources().inc_dirs.return_value = [] 00084 00085 build_tests(tests, src_paths, build_path, target, toolchain_name, 00086 app_config=app_config) 00087 00088 arg_list = mock_build_project.call_args_list 00089 for args in arg_list: 00090 assert 'app_config' in args[1],\ 00091 "build_tests was not called with app_config" 00092 assert args[1]['app_config'] == app_config,\ 00093 "build_tests was called with an incorrect app_config"
Generated on Sun Jul 17 2022 08:25:32 by
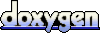