
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
target_test.py
00001 #!/usr/bin/env python 00002 """ 00003 mbed 00004 Copyright (c) 2017-2017 ARM Limited 00005 00006 Licensed under the Apache License, Version 2.0 (the "License"); 00007 you may not use this file except in compliance with the License. 00008 You may obtain a copy of the License at 00009 00010 http://www.apache.org/licenses/LICENSE-2.0 00011 00012 Unless required by applicable law or agreed to in writing, software 00013 distributed under the License is distributed on an "AS IS" BASIS, 00014 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 See the License for the specific language governing permissions and 00016 limitations under the License. 00017 """ 00018 import os 00019 import sys 00020 import shutil 00021 import tempfile 00022 from os.path import join, abspath, dirname 00023 from contextlib import contextmanager 00024 import pytest 00025 00026 from tools.targets import TARGETS, TARGET_MAP, Target, update_target_data 00027 from tools.arm_pack_manager import Cache 00028 00029 00030 def test_device_name(): 00031 """Assert device name is in a pack""" 00032 cache = Cache(True, True) 00033 named_targets = (target for target in TARGETS if 00034 hasattr(target, "device_name")) 00035 for target in named_targets: 00036 assert target.device_name in cache.index,\ 00037 ("Target %s contains invalid device_name %s" % 00038 (target.name, target.device_name)) 00039 00040 @contextmanager 00041 def temp_target_file(extra_target, json_filename='custom_targets.json'): 00042 """Create an extra targets temp file in a context manager 00043 00044 :param extra_target: the contents of the extra targets temp file 00045 """ 00046 tempdir = tempfile.mkdtemp() 00047 try: 00048 targetfile = os.path.join(tempdir, json_filename) 00049 with open(targetfile, 'w') as f: 00050 f.write(extra_target) 00051 yield tempdir 00052 finally: 00053 # Reset extra targets 00054 Target.set_targets_json_location() 00055 # Delete temp files 00056 shutil.rmtree(tempdir) 00057 00058 def test_add_extra_targets(): 00059 """Search for extra targets json in a source folder""" 00060 test_target_json = """ 00061 { 00062 "Test_Target": { 00063 "inherits": ["Target"] 00064 } 00065 } 00066 """ 00067 with temp_target_file(test_target_json) as source_dir: 00068 Target.add_extra_targets(source_dir=source_dir) 00069 update_target_data() 00070 00071 assert 'Test_Target' in TARGET_MAP 00072 assert TARGET_MAP['Test_Target'].core is None, \ 00073 "attributes should be inherited from Target" 00074 00075 def test_modify_existing_target(): 00076 """Set default targets file, then override base Target definition""" 00077 initial_target_json = """ 00078 { 00079 "Target": { 00080 "core": null, 00081 "default_toolchain": "ARM", 00082 "supported_toolchains": null, 00083 "extra_labels": [], 00084 "is_disk_virtual": false, 00085 "macros": [], 00086 "device_has": [], 00087 "features": [], 00088 "detect_code": [], 00089 "public": false, 00090 "default_lib": "std", 00091 "bootloader_supported": false 00092 }, 00093 "Test_Target": { 00094 "inherits": ["Target"], 00095 "core": "Cortex-M4", 00096 "supported_toolchains": ["ARM"] 00097 } 00098 }""" 00099 00100 test_target_json = """ 00101 { 00102 "Target": { 00103 "core": "Cortex-M0", 00104 "default_toolchain": "GCC_ARM", 00105 "supported_toolchains": null, 00106 "extra_labels": [], 00107 "is_disk_virtual": false, 00108 "macros": [], 00109 "device_has": [], 00110 "features": [], 00111 "detect_code": [], 00112 "public": false, 00113 "default_lib": "std", 00114 "bootloader_supported": true 00115 } 00116 } 00117 """ 00118 00119 with temp_target_file(initial_target_json, json_filename="targets.json") as targets_dir: 00120 Target.set_targets_json_location(os.path.join(targets_dir, "targets.json")) 00121 update_target_data() 00122 assert TARGET_MAP["Test_Target"].core == "Cortex-M4" 00123 assert TARGET_MAP["Test_Target"].default_toolchain == 'ARM' 00124 assert TARGET_MAP["Test_Target"].bootloader_supported == False 00125 00126 with temp_target_file(test_target_json) as source_dir: 00127 Target.add_extra_targets(source_dir=source_dir) 00128 update_target_data() 00129 00130 assert TARGET_MAP["Test_Target"].core == "Cortex-M4" 00131 # The existing target should not be modified by custom targets 00132 assert TARGET_MAP["Test_Target"].default_toolchain != 'GCC_ARM' 00133 assert TARGET_MAP["Test_Target"].bootloader_supported != True
Generated on Sun Jul 17 2022 08:25:31 by
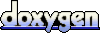