
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
sys_arch.h
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 #ifndef __ARCH_SYS_ARCH_H__ 00019 #define __ARCH_SYS_ARCH_H__ 00020 00021 #include "lwip/opt.h" 00022 #include "mbed_rtos_storage.h" 00023 00024 extern u8_t lwip_ram_heap[]; 00025 00026 #if NO_SYS == 0 00027 #include "cmsis_os2.h" 00028 00029 // === SEMAPHORE === 00030 typedef struct { 00031 osSemaphoreId_t id; 00032 osSemaphoreAttr_t attr; 00033 mbed_rtos_storage_semaphore_t data; 00034 } sys_sem_t; 00035 00036 #define sys_sem_valid(x) (((*x).id == NULL) ? 0 : 1) 00037 #define sys_sem_set_invalid(x) ( (*x).id = NULL) 00038 00039 // === MUTEX === 00040 typedef struct { 00041 osMutexId_t id; 00042 osMutexAttr_t attr; 00043 mbed_rtos_storage_mutex_t data; 00044 } sys_mutex_t; 00045 00046 // === MAIL BOX === 00047 #define MB_SIZE 8 00048 00049 typedef struct { 00050 osEventFlagsId_t id; 00051 osEventFlagsAttr_t attr; 00052 mbed_rtos_storage_event_flags_t data; 00053 00054 uint8_t post_idx; 00055 uint8_t fetch_idx; 00056 void* queue[MB_SIZE]; 00057 } sys_mbox_t; 00058 00059 #define SYS_MBOX_FETCH_EVENT 0x1 00060 #define SYS_MBOX_POST_EVENT 0x2 00061 00062 #define SYS_MBOX_NULL ((uint32_t) NULL) 00063 #define sys_mbox_valid(x) (((*x).id == NULL) ? 0 : 1) 00064 #define sys_mbox_set_invalid(x) ( (*x).id = NULL) 00065 00066 #if ((DEFAULT_RAW_RECVMBOX_SIZE) > (MB_SIZE)) || \ 00067 ((DEFAULT_UDP_RECVMBOX_SIZE) > (MB_SIZE)) || \ 00068 ((DEFAULT_TCP_RECVMBOX_SIZE) > (MB_SIZE)) || \ 00069 ((DEFAULT_ACCEPTMBOX_SIZE) > (MB_SIZE)) || \ 00070 ((TCPIP_MBOX_SIZE) > (MB_SIZE)) 00071 # error Mailbox size not supported 00072 #endif 00073 00074 // === THREAD === 00075 typedef struct { 00076 osThreadId_t id; 00077 osThreadAttr_t attr; 00078 mbed_rtos_storage_thread_t data; 00079 } sys_thread_data_t; 00080 typedef sys_thread_data_t* sys_thread_t; 00081 00082 #define SYS_THREAD_POOL_N 6 00083 #define SYS_DEFAULT_THREAD_STACK_DEPTH OS_STACK_SIZE 00084 00085 // === PROTECTION === 00086 typedef int sys_prot_t; 00087 00088 #else 00089 #ifdef __cplusplus 00090 extern "C" { 00091 #endif 00092 00093 /** \brief Init systick to 1ms rate 00094 * 00095 * This init the systick to 1ms rate. This function is only used in standalone 00096 * systems. 00097 */ 00098 void SysTick_Init(void); 00099 00100 00101 /** \brief Get the current systick time in milliSeconds 00102 * 00103 * Returns the current systick time in milliSeconds. This function is only 00104 * used in standalone systems. 00105 * 00106 * /returns current systick time in milliSeconds 00107 */ 00108 uint32_t SysTick_GetMS(void); 00109 00110 /** \brief Delay for the specified number of milliSeconds 00111 * 00112 * For standalone systems. This function will block for the specified 00113 * number of milliSconds. For RTOS based systems, this function will delay 00114 * the task by the specified number of milliSeconds. 00115 * 00116 * \param[in] ms Time in milliSeconds to delay 00117 */ 00118 void osDelay(uint32_t ms); 00119 00120 #ifdef __cplusplus 00121 } 00122 #endif 00123 #endif 00124 00125 #endif /* __ARCH_SYS_ARCH_H__ */
Generated on Sun Jul 17 2022 08:25:31 by
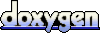