
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
syms.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2013 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 00017 00018 Utility to find which libraries could define a given symbol 00019 """ 00020 from argparse import ArgumentParser 00021 from os.path import join, splitext 00022 from os import walk 00023 from subprocess import Popen, PIPE 00024 00025 00026 OBJ_EXT = ['.o', '.a', '.ar'] 00027 00028 00029 def find_sym_in_lib(sym, obj_path): 00030 contain_symbol = False 00031 00032 out = Popen(["nm", "-C", obj_path], stdout=PIPE, stderr=PIPE).communicate()[0] 00033 for line in out.splitlines(): 00034 tokens = line.split() 00035 n = len(tokens) 00036 if n == 2: 00037 sym_type = tokens[0] 00038 sym_name = tokens[1] 00039 elif n == 3: 00040 sym_type = tokens[1] 00041 sym_name = tokens[2] 00042 else: 00043 continue 00044 00045 if sym_type == "U": 00046 # This object is using this symbol, not defining it 00047 continue 00048 00049 if sym_name == sym: 00050 contain_symbol = True 00051 00052 return contain_symbol 00053 00054 00055 def find_sym_in_path(sym, dir_path): 00056 for root, _, files in walk(dir_path): 00057 for file in files: 00058 00059 _, ext = splitext(file) 00060 if ext not in OBJ_EXT: continue 00061 00062 path = join(root, file) 00063 if find_sym_in_lib(sym, path): 00064 print path 00065 00066 00067 if __name__ == '__main__': 00068 parser = ArgumentParser(description='Find Symbol') 00069 parser.add_argument('-s', '--sym', required=True, 00070 help='The symbol to be searched') 00071 parser.add_argument('-p', '--path', required=True, 00072 help='The path where to search') 00073 args = parser.parse_args() 00074 00075 find_sym_in_path(args.sym, args.path)
Generated on Sun Jul 17 2022 08:25:31 by
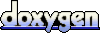