
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ssl_cli.c
00001 /* 00002 * SSLv3/TLSv1 client-side functions 00003 * 00004 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00005 * SPDX-License-Identifier: Apache-2.0 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00008 * not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00015 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 * 00019 * This file is part of mbed TLS (https://tls.mbed.org) 00020 */ 00021 00022 #if !defined(MBEDTLS_CONFIG_FILE) 00023 #include "mbedtls/config.h" 00024 #else 00025 #include MBEDTLS_CONFIG_FILE 00026 #endif 00027 00028 #if defined(MBEDTLS_SSL_CLI_C) 00029 00030 #if defined(MBEDTLS_PLATFORM_C) 00031 #include "mbedtls/platform.h" 00032 #else 00033 #include <stdlib.h> 00034 #define mbedtls_calloc calloc 00035 #define mbedtls_free free 00036 #endif 00037 00038 #include "mbedtls/debug.h" 00039 #include "mbedtls/ssl.h" 00040 #include "mbedtls/ssl_internal.h" 00041 00042 #include <string.h> 00043 00044 #include <stdint.h> 00045 00046 #if defined(MBEDTLS_HAVE_TIME) 00047 #include "mbedtls/platform_time.h" 00048 #endif 00049 00050 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 00051 /* Implementation that should never be optimized out by the compiler */ 00052 static void mbedtls_zeroize( void *v, size_t n ) { 00053 volatile unsigned char *p = v; while( n-- ) *p++ = 0; 00054 } 00055 #endif 00056 00057 #if defined(MBEDTLS_SSL_SERVER_NAME_INDICATION) 00058 static void ssl_write_hostname_ext( mbedtls_ssl_context *ssl, 00059 unsigned char *buf, 00060 size_t *olen ) 00061 { 00062 unsigned char *p = buf; 00063 const unsigned char *end = ssl->out_msg + MBEDTLS_SSL_MAX_CONTENT_LEN; 00064 size_t hostname_len; 00065 00066 *olen = 0; 00067 00068 if( ssl->hostname == NULL ) 00069 return; 00070 00071 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, adding server name extension: %s", 00072 ssl->hostname ) ); 00073 00074 hostname_len = strlen( ssl->hostname ); 00075 00076 if( end < p || (size_t)( end - p ) < hostname_len + 9 ) 00077 { 00078 MBEDTLS_SSL_DEBUG_MSG( 1, ( "buffer too small" ) ); 00079 return; 00080 } 00081 00082 /* 00083 * struct { 00084 * NameType name_type; 00085 * select (name_type) { 00086 * case host_name: HostName; 00087 * } name; 00088 * } ServerName; 00089 * 00090 * enum { 00091 * host_name(0), (255) 00092 * } NameType; 00093 * 00094 * opaque HostName<1..2^16-1>; 00095 * 00096 * struct { 00097 * ServerName server_name_list<1..2^16-1> 00098 * } ServerNameList; 00099 */ 00100 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_SERVERNAME >> 8 ) & 0xFF ); 00101 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_SERVERNAME ) & 0xFF ); 00102 00103 *p++ = (unsigned char)( ( (hostname_len + 5) >> 8 ) & 0xFF ); 00104 *p++ = (unsigned char)( ( (hostname_len + 5) ) & 0xFF ); 00105 00106 *p++ = (unsigned char)( ( (hostname_len + 3) >> 8 ) & 0xFF ); 00107 *p++ = (unsigned char)( ( (hostname_len + 3) ) & 0xFF ); 00108 00109 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_SERVERNAME_HOSTNAME ) & 0xFF ); 00110 *p++ = (unsigned char)( ( hostname_len >> 8 ) & 0xFF ); 00111 *p++ = (unsigned char)( ( hostname_len ) & 0xFF ); 00112 00113 memcpy( p, ssl->hostname, hostname_len ); 00114 00115 *olen = hostname_len + 9; 00116 } 00117 #endif /* MBEDTLS_SSL_SERVER_NAME_INDICATION */ 00118 00119 #if defined(MBEDTLS_SSL_RENEGOTIATION) 00120 static void ssl_write_renegotiation_ext( mbedtls_ssl_context *ssl, 00121 unsigned char *buf, 00122 size_t *olen ) 00123 { 00124 unsigned char *p = buf; 00125 const unsigned char *end = ssl->out_msg + MBEDTLS_SSL_MAX_CONTENT_LEN; 00126 00127 *olen = 0; 00128 00129 if( ssl->renego_status != MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS ) 00130 return; 00131 00132 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, adding renegotiation extension" ) ); 00133 00134 if( end < p || (size_t)( end - p ) < 5 + ssl->verify_data_len ) 00135 { 00136 MBEDTLS_SSL_DEBUG_MSG( 1, ( "buffer too small" ) ); 00137 return; 00138 } 00139 00140 /* 00141 * Secure renegotiation 00142 */ 00143 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_RENEGOTIATION_INFO >> 8 ) & 0xFF ); 00144 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_RENEGOTIATION_INFO ) & 0xFF ); 00145 00146 *p++ = 0x00; 00147 *p++ = ( ssl->verify_data_len + 1 ) & 0xFF; 00148 *p++ = ssl->verify_data_len & 0xFF; 00149 00150 memcpy( p, ssl->own_verify_data, ssl->verify_data_len ); 00151 00152 *olen = 5 + ssl->verify_data_len; 00153 } 00154 #endif /* MBEDTLS_SSL_RENEGOTIATION */ 00155 00156 /* 00157 * Only if we handle at least one key exchange that needs signatures. 00158 */ 00159 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) && \ 00160 defined(MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED) 00161 static void ssl_write_signature_algorithms_ext( mbedtls_ssl_context *ssl, 00162 unsigned char *buf, 00163 size_t *olen ) 00164 { 00165 unsigned char *p = buf; 00166 const unsigned char *end = ssl->out_msg + MBEDTLS_SSL_MAX_CONTENT_LEN; 00167 size_t sig_alg_len = 0; 00168 const int *md; 00169 #if defined(MBEDTLS_RSA_C) || defined(MBEDTLS_ECDSA_C) 00170 unsigned char *sig_alg_list = buf + 6; 00171 #endif 00172 00173 *olen = 0; 00174 00175 if( ssl->conf->max_minor_ver != MBEDTLS_SSL_MINOR_VERSION_3 ) 00176 return; 00177 00178 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, adding signature_algorithms extension" ) ); 00179 00180 for( md = ssl->conf->sig_hashes; *md != MBEDTLS_MD_NONE; md++ ) 00181 { 00182 #if defined(MBEDTLS_ECDSA_C) 00183 sig_alg_len += 2; 00184 #endif 00185 #if defined(MBEDTLS_RSA_C) 00186 sig_alg_len += 2; 00187 #endif 00188 } 00189 00190 if( end < p || (size_t)( end - p ) < sig_alg_len + 6 ) 00191 { 00192 MBEDTLS_SSL_DEBUG_MSG( 1, ( "buffer too small" ) ); 00193 return; 00194 } 00195 00196 /* 00197 * Prepare signature_algorithms extension (TLS 1.2) 00198 */ 00199 sig_alg_len = 0; 00200 00201 for( md = ssl->conf->sig_hashes; *md != MBEDTLS_MD_NONE; md++ ) 00202 { 00203 #if defined(MBEDTLS_ECDSA_C) 00204 sig_alg_list[sig_alg_len++] = mbedtls_ssl_hash_from_md_alg( *md ); 00205 sig_alg_list[sig_alg_len++] = MBEDTLS_SSL_SIG_ECDSA; 00206 #endif 00207 #if defined(MBEDTLS_RSA_C) 00208 sig_alg_list[sig_alg_len++] = mbedtls_ssl_hash_from_md_alg( *md ); 00209 sig_alg_list[sig_alg_len++] = MBEDTLS_SSL_SIG_RSA; 00210 #endif 00211 } 00212 00213 /* 00214 * enum { 00215 * none(0), md5(1), sha1(2), sha224(3), sha256(4), sha384(5), 00216 * sha512(6), (255) 00217 * } HashAlgorithm; 00218 * 00219 * enum { anonymous(0), rsa(1), dsa(2), ecdsa(3), (255) } 00220 * SignatureAlgorithm; 00221 * 00222 * struct { 00223 * HashAlgorithm hash; 00224 * SignatureAlgorithm signature; 00225 * } SignatureAndHashAlgorithm; 00226 * 00227 * SignatureAndHashAlgorithm 00228 * supported_signature_algorithms<2..2^16-2>; 00229 */ 00230 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_SIG_ALG >> 8 ) & 0xFF ); 00231 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_SIG_ALG ) & 0xFF ); 00232 00233 *p++ = (unsigned char)( ( ( sig_alg_len + 2 ) >> 8 ) & 0xFF ); 00234 *p++ = (unsigned char)( ( ( sig_alg_len + 2 ) ) & 0xFF ); 00235 00236 *p++ = (unsigned char)( ( sig_alg_len >> 8 ) & 0xFF ); 00237 *p++ = (unsigned char)( ( sig_alg_len ) & 0xFF ); 00238 00239 *olen = 6 + sig_alg_len; 00240 } 00241 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 && 00242 MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED */ 00243 00244 #if defined(MBEDTLS_ECDH_C) || defined(MBEDTLS_ECDSA_C) || \ 00245 defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 00246 static void ssl_write_supported_elliptic_curves_ext( mbedtls_ssl_context *ssl, 00247 unsigned char *buf, 00248 size_t *olen ) 00249 { 00250 unsigned char *p = buf; 00251 const unsigned char *end = ssl->out_msg + MBEDTLS_SSL_MAX_CONTENT_LEN; 00252 unsigned char *elliptic_curve_list = p + 6; 00253 size_t elliptic_curve_len = 0; 00254 const mbedtls_ecp_curve_info *info; 00255 #if defined(MBEDTLS_ECP_C) 00256 const mbedtls_ecp_group_id *grp_id; 00257 #else 00258 ((void) ssl); 00259 #endif 00260 00261 *olen = 0; 00262 00263 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, adding supported_elliptic_curves extension" ) ); 00264 00265 #if defined(MBEDTLS_ECP_C) 00266 for( grp_id = ssl->conf->curve_list; *grp_id != MBEDTLS_ECP_DP_NONE; grp_id++ ) 00267 #else 00268 for( info = mbedtls_ecp_curve_list(); info->grp_id != MBEDTLS_ECP_DP_NONE; info++ ) 00269 #endif 00270 { 00271 #if defined(MBEDTLS_ECP_C) 00272 info = mbedtls_ecp_curve_info_from_grp_id( *grp_id ); 00273 #endif 00274 if( info == NULL ) 00275 { 00276 MBEDTLS_SSL_DEBUG_MSG( 1, ( "invalid curve in ssl configuration" ) ); 00277 return; 00278 } 00279 00280 elliptic_curve_len += 2; 00281 } 00282 00283 if( end < p || (size_t)( end - p ) < 6 + elliptic_curve_len ) 00284 { 00285 MBEDTLS_SSL_DEBUG_MSG( 1, ( "buffer too small" ) ); 00286 return; 00287 } 00288 00289 elliptic_curve_len = 0; 00290 00291 #if defined(MBEDTLS_ECP_C) 00292 for( grp_id = ssl->conf->curve_list; *grp_id != MBEDTLS_ECP_DP_NONE; grp_id++ ) 00293 #else 00294 for( info = mbedtls_ecp_curve_list(); info->grp_id != MBEDTLS_ECP_DP_NONE; info++ ) 00295 #endif 00296 { 00297 #if defined(MBEDTLS_ECP_C) 00298 info = mbedtls_ecp_curve_info_from_grp_id( *grp_id ); 00299 #endif 00300 elliptic_curve_list[elliptic_curve_len++] = info->tls_id >> 8; 00301 elliptic_curve_list[elliptic_curve_len++] = info->tls_id & 0xFF; 00302 } 00303 00304 if( elliptic_curve_len == 0 ) 00305 return; 00306 00307 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_SUPPORTED_ELLIPTIC_CURVES >> 8 ) & 0xFF ); 00308 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_SUPPORTED_ELLIPTIC_CURVES ) & 0xFF ); 00309 00310 *p++ = (unsigned char)( ( ( elliptic_curve_len + 2 ) >> 8 ) & 0xFF ); 00311 *p++ = (unsigned char)( ( ( elliptic_curve_len + 2 ) ) & 0xFF ); 00312 00313 *p++ = (unsigned char)( ( ( elliptic_curve_len ) >> 8 ) & 0xFF ); 00314 *p++ = (unsigned char)( ( ( elliptic_curve_len ) ) & 0xFF ); 00315 00316 *olen = 6 + elliptic_curve_len; 00317 } 00318 00319 static void ssl_write_supported_point_formats_ext( mbedtls_ssl_context *ssl, 00320 unsigned char *buf, 00321 size_t *olen ) 00322 { 00323 unsigned char *p = buf; 00324 const unsigned char *end = ssl->out_msg + MBEDTLS_SSL_MAX_CONTENT_LEN; 00325 00326 *olen = 0; 00327 00328 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, adding supported_point_formats extension" ) ); 00329 00330 if( end < p || (size_t)( end - p ) < 6 ) 00331 { 00332 MBEDTLS_SSL_DEBUG_MSG( 1, ( "buffer too small" ) ); 00333 return; 00334 } 00335 00336 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_SUPPORTED_POINT_FORMATS >> 8 ) & 0xFF ); 00337 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_SUPPORTED_POINT_FORMATS ) & 0xFF ); 00338 00339 *p++ = 0x00; 00340 *p++ = 2; 00341 00342 *p++ = 1; 00343 *p++ = MBEDTLS_ECP_PF_UNCOMPRESSED; 00344 00345 *olen = 6; 00346 } 00347 #endif /* MBEDTLS_ECDH_C || MBEDTLS_ECDSA_C || 00348 MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 00349 00350 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 00351 static void ssl_write_ecjpake_kkpp_ext( mbedtls_ssl_context *ssl, 00352 unsigned char *buf, 00353 size_t *olen ) 00354 { 00355 int ret; 00356 unsigned char *p = buf; 00357 const unsigned char *end = ssl->out_msg + MBEDTLS_SSL_MAX_CONTENT_LEN; 00358 size_t kkpp_len; 00359 00360 *olen = 0; 00361 00362 /* Skip costly extension if we can't use EC J-PAKE anyway */ 00363 if( mbedtls_ecjpake_check( &ssl->handshake->ecjpake_ctx ) != 0 ) 00364 return; 00365 00366 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, adding ecjpake_kkpp extension" ) ); 00367 00368 if( end - p < 4 ) 00369 { 00370 MBEDTLS_SSL_DEBUG_MSG( 1, ( "buffer too small" ) ); 00371 return; 00372 } 00373 00374 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_ECJPAKE_KKPP >> 8 ) & 0xFF ); 00375 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_ECJPAKE_KKPP ) & 0xFF ); 00376 00377 /* 00378 * We may need to send ClientHello multiple times for Hello verification. 00379 * We don't want to compute fresh values every time (both for performance 00380 * and consistency reasons), so cache the extension content. 00381 */ 00382 if( ssl->handshake->ecjpake_cache == NULL || 00383 ssl->handshake->ecjpake_cache_len == 0 ) 00384 { 00385 MBEDTLS_SSL_DEBUG_MSG( 3, ( "generating new ecjpake parameters" ) ); 00386 00387 ret = mbedtls_ecjpake_write_round_one( &ssl->handshake->ecjpake_ctx, 00388 p + 2, end - p - 2, &kkpp_len, 00389 ssl->conf->f_rng, ssl->conf->p_rng ); 00390 if( ret != 0 ) 00391 { 00392 MBEDTLS_SSL_DEBUG_RET( 1 , "mbedtls_ecjpake_write_round_one", ret ); 00393 return; 00394 } 00395 00396 ssl->handshake->ecjpake_cache = mbedtls_calloc( 1, kkpp_len ); 00397 if( ssl->handshake->ecjpake_cache == NULL ) 00398 { 00399 MBEDTLS_SSL_DEBUG_MSG( 1, ( "allocation failed" ) ); 00400 return; 00401 } 00402 00403 memcpy( ssl->handshake->ecjpake_cache, p + 2, kkpp_len ); 00404 ssl->handshake->ecjpake_cache_len = kkpp_len; 00405 } 00406 else 00407 { 00408 MBEDTLS_SSL_DEBUG_MSG( 3, ( "re-using cached ecjpake parameters" ) ); 00409 00410 kkpp_len = ssl->handshake->ecjpake_cache_len; 00411 00412 if( (size_t)( end - p - 2 ) < kkpp_len ) 00413 { 00414 MBEDTLS_SSL_DEBUG_MSG( 1, ( "buffer too small" ) ); 00415 return; 00416 } 00417 00418 memcpy( p + 2, ssl->handshake->ecjpake_cache, kkpp_len ); 00419 } 00420 00421 *p++ = (unsigned char)( ( kkpp_len >> 8 ) & 0xFF ); 00422 *p++ = (unsigned char)( ( kkpp_len ) & 0xFF ); 00423 00424 *olen = kkpp_len + 4; 00425 } 00426 #endif /* MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 00427 00428 #if defined(MBEDTLS_SSL_MAX_FRAGMENT_LENGTH) 00429 static void ssl_write_max_fragment_length_ext( mbedtls_ssl_context *ssl, 00430 unsigned char *buf, 00431 size_t *olen ) 00432 { 00433 unsigned char *p = buf; 00434 const unsigned char *end = ssl->out_msg + MBEDTLS_SSL_MAX_CONTENT_LEN; 00435 00436 *olen = 0; 00437 00438 if( ssl->conf->mfl_code == MBEDTLS_SSL_MAX_FRAG_LEN_NONE ) { 00439 return; 00440 } 00441 00442 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, adding max_fragment_length extension" ) ); 00443 00444 if( end < p || (size_t)( end - p ) < 5 ) 00445 { 00446 MBEDTLS_SSL_DEBUG_MSG( 1, ( "buffer too small" ) ); 00447 return; 00448 } 00449 00450 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_MAX_FRAGMENT_LENGTH >> 8 ) & 0xFF ); 00451 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_MAX_FRAGMENT_LENGTH ) & 0xFF ); 00452 00453 *p++ = 0x00; 00454 *p++ = 1; 00455 00456 *p++ = ssl->conf->mfl_code; 00457 00458 *olen = 5; 00459 } 00460 #endif /* MBEDTLS_SSL_MAX_FRAGMENT_LENGTH */ 00461 00462 #if defined(MBEDTLS_SSL_TRUNCATED_HMAC) 00463 static void ssl_write_truncated_hmac_ext( mbedtls_ssl_context *ssl, 00464 unsigned char *buf, size_t *olen ) 00465 { 00466 unsigned char *p = buf; 00467 const unsigned char *end = ssl->out_msg + MBEDTLS_SSL_MAX_CONTENT_LEN; 00468 00469 *olen = 0; 00470 00471 if( ssl->conf->trunc_hmac == MBEDTLS_SSL_TRUNC_HMAC_DISABLED ) 00472 { 00473 return; 00474 } 00475 00476 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, adding truncated_hmac extension" ) ); 00477 00478 if( end < p || (size_t)( end - p ) < 4 ) 00479 { 00480 MBEDTLS_SSL_DEBUG_MSG( 1, ( "buffer too small" ) ); 00481 return; 00482 } 00483 00484 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_TRUNCATED_HMAC >> 8 ) & 0xFF ); 00485 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_TRUNCATED_HMAC ) & 0xFF ); 00486 00487 *p++ = 0x00; 00488 *p++ = 0x00; 00489 00490 *olen = 4; 00491 } 00492 #endif /* MBEDTLS_SSL_TRUNCATED_HMAC */ 00493 00494 #if defined(MBEDTLS_SSL_ENCRYPT_THEN_MAC) 00495 static void ssl_write_encrypt_then_mac_ext( mbedtls_ssl_context *ssl, 00496 unsigned char *buf, size_t *olen ) 00497 { 00498 unsigned char *p = buf; 00499 const unsigned char *end = ssl->out_msg + MBEDTLS_SSL_MAX_CONTENT_LEN; 00500 00501 *olen = 0; 00502 00503 if( ssl->conf->encrypt_then_mac == MBEDTLS_SSL_ETM_DISABLED || 00504 ssl->conf->max_minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 ) 00505 { 00506 return; 00507 } 00508 00509 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, adding encrypt_then_mac " 00510 "extension" ) ); 00511 00512 if( end < p || (size_t)( end - p ) < 4 ) 00513 { 00514 MBEDTLS_SSL_DEBUG_MSG( 1, ( "buffer too small" ) ); 00515 return; 00516 } 00517 00518 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_ENCRYPT_THEN_MAC >> 8 ) & 0xFF ); 00519 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_ENCRYPT_THEN_MAC ) & 0xFF ); 00520 00521 *p++ = 0x00; 00522 *p++ = 0x00; 00523 00524 *olen = 4; 00525 } 00526 #endif /* MBEDTLS_SSL_ENCRYPT_THEN_MAC */ 00527 00528 #if defined(MBEDTLS_SSL_EXTENDED_MASTER_SECRET) 00529 static void ssl_write_extended_ms_ext( mbedtls_ssl_context *ssl, 00530 unsigned char *buf, size_t *olen ) 00531 { 00532 unsigned char *p = buf; 00533 const unsigned char *end = ssl->out_msg + MBEDTLS_SSL_MAX_CONTENT_LEN; 00534 00535 *olen = 0; 00536 00537 if( ssl->conf->extended_ms == MBEDTLS_SSL_EXTENDED_MS_DISABLED || 00538 ssl->conf->max_minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 ) 00539 { 00540 return; 00541 } 00542 00543 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, adding extended_master_secret " 00544 "extension" ) ); 00545 00546 if( end < p || (size_t)( end - p ) < 4 ) 00547 { 00548 MBEDTLS_SSL_DEBUG_MSG( 1, ( "buffer too small" ) ); 00549 return; 00550 } 00551 00552 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_EXTENDED_MASTER_SECRET >> 8 ) & 0xFF ); 00553 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_EXTENDED_MASTER_SECRET ) & 0xFF ); 00554 00555 *p++ = 0x00; 00556 *p++ = 0x00; 00557 00558 *olen = 4; 00559 } 00560 #endif /* MBEDTLS_SSL_EXTENDED_MASTER_SECRET */ 00561 00562 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 00563 static void ssl_write_session_ticket_ext( mbedtls_ssl_context *ssl, 00564 unsigned char *buf, size_t *olen ) 00565 { 00566 unsigned char *p = buf; 00567 const unsigned char *end = ssl->out_msg + MBEDTLS_SSL_MAX_CONTENT_LEN; 00568 size_t tlen = ssl->session_negotiate->ticket_len; 00569 00570 *olen = 0; 00571 00572 if( ssl->conf->session_tickets == MBEDTLS_SSL_SESSION_TICKETS_DISABLED ) 00573 { 00574 return; 00575 } 00576 00577 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, adding session ticket extension" ) ); 00578 00579 if( end < p || (size_t)( end - p ) < 4 + tlen ) 00580 { 00581 MBEDTLS_SSL_DEBUG_MSG( 1, ( "buffer too small" ) ); 00582 return; 00583 } 00584 00585 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_SESSION_TICKET >> 8 ) & 0xFF ); 00586 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_SESSION_TICKET ) & 0xFF ); 00587 00588 *p++ = (unsigned char)( ( tlen >> 8 ) & 0xFF ); 00589 *p++ = (unsigned char)( ( tlen ) & 0xFF ); 00590 00591 *olen = 4; 00592 00593 if( ssl->session_negotiate->ticket == NULL || tlen == 0 ) 00594 { 00595 return; 00596 } 00597 00598 MBEDTLS_SSL_DEBUG_MSG( 3, ( "sending session ticket of length %d", tlen ) ); 00599 00600 memcpy( p, ssl->session_negotiate->ticket, tlen ); 00601 00602 *olen += tlen; 00603 } 00604 #endif /* MBEDTLS_SSL_SESSION_TICKETS */ 00605 00606 #if defined(MBEDTLS_SSL_ALPN) 00607 static void ssl_write_alpn_ext( mbedtls_ssl_context *ssl, 00608 unsigned char *buf, size_t *olen ) 00609 { 00610 unsigned char *p = buf; 00611 const unsigned char *end = ssl->out_msg + MBEDTLS_SSL_MAX_CONTENT_LEN; 00612 size_t alpnlen = 0; 00613 const char **cur; 00614 00615 *olen = 0; 00616 00617 if( ssl->conf->alpn_list == NULL ) 00618 { 00619 return; 00620 } 00621 00622 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, adding alpn extension" ) ); 00623 00624 for( cur = ssl->conf->alpn_list; *cur != NULL; cur++ ) 00625 alpnlen += (unsigned char)( strlen( *cur ) & 0xFF ) + 1; 00626 00627 if( end < p || (size_t)( end - p ) < 6 + alpnlen ) 00628 { 00629 MBEDTLS_SSL_DEBUG_MSG( 1, ( "buffer too small" ) ); 00630 return; 00631 } 00632 00633 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_ALPN >> 8 ) & 0xFF ); 00634 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_ALPN ) & 0xFF ); 00635 00636 /* 00637 * opaque ProtocolName<1..2^8-1>; 00638 * 00639 * struct { 00640 * ProtocolName protocol_name_list<2..2^16-1> 00641 * } ProtocolNameList; 00642 */ 00643 00644 /* Skip writing extension and list length for now */ 00645 p += 4; 00646 00647 for( cur = ssl->conf->alpn_list; *cur != NULL; cur++ ) 00648 { 00649 *p = (unsigned char)( strlen( *cur ) & 0xFF ); 00650 memcpy( p + 1, *cur, *p ); 00651 p += 1 + *p; 00652 } 00653 00654 *olen = p - buf; 00655 00656 /* List length = olen - 2 (ext_type) - 2 (ext_len) - 2 (list_len) */ 00657 buf[4] = (unsigned char)( ( ( *olen - 6 ) >> 8 ) & 0xFF ); 00658 buf[5] = (unsigned char)( ( ( *olen - 6 ) ) & 0xFF ); 00659 00660 /* Extension length = olen - 2 (ext_type) - 2 (ext_len) */ 00661 buf[2] = (unsigned char)( ( ( *olen - 4 ) >> 8 ) & 0xFF ); 00662 buf[3] = (unsigned char)( ( ( *olen - 4 ) ) & 0xFF ); 00663 } 00664 #endif /* MBEDTLS_SSL_ALPN */ 00665 00666 /* 00667 * Generate random bytes for ClientHello 00668 */ 00669 static int ssl_generate_random( mbedtls_ssl_context *ssl ) 00670 { 00671 int ret; 00672 unsigned char *p = ssl->handshake->randbytes; 00673 #if defined(MBEDTLS_HAVE_TIME) 00674 mbedtls_time_t t; 00675 #endif 00676 00677 /* 00678 * When responding to a verify request, MUST reuse random (RFC 6347 4.2.1) 00679 */ 00680 #if defined(MBEDTLS_SSL_PROTO_DTLS) 00681 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM && 00682 ssl->handshake->verify_cookie != NULL ) 00683 { 00684 return( 0 ); 00685 } 00686 #endif 00687 00688 #if defined(MBEDTLS_HAVE_TIME) 00689 t = mbedtls_time( NULL ); 00690 *p++ = (unsigned char)( t >> 24 ); 00691 *p++ = (unsigned char)( t >> 16 ); 00692 *p++ = (unsigned char)( t >> 8 ); 00693 *p++ = (unsigned char)( t ); 00694 00695 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, current time: %lu", t ) ); 00696 #else 00697 if( ( ret = ssl->conf->f_rng( ssl->conf->p_rng, p, 4 ) ) != 0 ) 00698 return( ret ); 00699 00700 p += 4; 00701 #endif /* MBEDTLS_HAVE_TIME */ 00702 00703 if( ( ret = ssl->conf->f_rng( ssl->conf->p_rng, p, 28 ) ) != 0 ) 00704 return( ret ); 00705 00706 return( 0 ); 00707 } 00708 00709 static int ssl_write_client_hello( mbedtls_ssl_context *ssl ) 00710 { 00711 int ret; 00712 size_t i, n, olen, ext_len = 0; 00713 unsigned char *buf; 00714 unsigned char *p, *q; 00715 unsigned char offer_compress; 00716 const int *ciphersuites; 00717 const mbedtls_ssl_ciphersuite_t *ciphersuite_info; 00718 00719 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write client hello" ) ); 00720 00721 if( ssl->conf->f_rng == NULL ) 00722 { 00723 MBEDTLS_SSL_DEBUG_MSG( 1, ( "no RNG provided") ); 00724 return( MBEDTLS_ERR_SSL_NO_RNG ); 00725 } 00726 00727 #if defined(MBEDTLS_SSL_RENEGOTIATION) 00728 if( ssl->renego_status == MBEDTLS_SSL_INITIAL_HANDSHAKE ) 00729 #endif 00730 { 00731 ssl->major_ver = ssl->conf->min_major_ver; 00732 ssl->minor_ver = ssl->conf->min_minor_ver; 00733 } 00734 00735 if( ssl->conf->max_major_ver == 0 ) 00736 { 00737 MBEDTLS_SSL_DEBUG_MSG( 1, ( "configured max major version is invalid, " 00738 "consider using mbedtls_ssl_config_defaults()" ) ); 00739 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 00740 } 00741 00742 /* 00743 * 0 . 0 handshake type 00744 * 1 . 3 handshake length 00745 * 4 . 5 highest version supported 00746 * 6 . 9 current UNIX time 00747 * 10 . 37 random bytes 00748 */ 00749 buf = ssl->out_msg; 00750 p = buf + 4; 00751 00752 mbedtls_ssl_write_version( ssl->conf->max_major_ver, ssl->conf->max_minor_ver, 00753 ssl->conf->transport, p ); 00754 p += 2; 00755 00756 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, max version: [%d:%d]", 00757 buf[4], buf[5] ) ); 00758 00759 if( ( ret = ssl_generate_random( ssl ) ) != 0 ) 00760 { 00761 MBEDTLS_SSL_DEBUG_RET( 1, "ssl_generate_random", ret ); 00762 return( ret ); 00763 } 00764 00765 memcpy( p, ssl->handshake->randbytes, 32 ); 00766 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, random bytes", p, 32 ); 00767 p += 32; 00768 00769 /* 00770 * 38 . 38 session id length 00771 * 39 . 39+n session id 00772 * 39+n . 39+n DTLS only: cookie length (1 byte) 00773 * 40+n . .. DTSL only: cookie 00774 * .. . .. ciphersuitelist length (2 bytes) 00775 * .. . .. ciphersuitelist 00776 * .. . .. compression methods length (1 byte) 00777 * .. . .. compression methods 00778 * .. . .. extensions length (2 bytes) 00779 * .. . .. extensions 00780 */ 00781 n = ssl->session_negotiate->id_len; 00782 00783 if( n < 16 || n > 32 || 00784 #if defined(MBEDTLS_SSL_RENEGOTIATION) 00785 ssl->renego_status != MBEDTLS_SSL_INITIAL_HANDSHAKE || 00786 #endif 00787 ssl->handshake->resume == 0 ) 00788 { 00789 n = 0; 00790 } 00791 00792 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 00793 /* 00794 * RFC 5077 section 3.4: "When presenting a ticket, the client MAY 00795 * generate and include a Session ID in the TLS ClientHello." 00796 */ 00797 #if defined(MBEDTLS_SSL_RENEGOTIATION) 00798 if( ssl->renego_status == MBEDTLS_SSL_INITIAL_HANDSHAKE ) 00799 #endif 00800 { 00801 if( ssl->session_negotiate->ticket != NULL && 00802 ssl->session_negotiate->ticket_len != 0 ) 00803 { 00804 ret = ssl->conf->f_rng( ssl->conf->p_rng, ssl->session_negotiate->id, 32 ); 00805 00806 if( ret != 0 ) 00807 return( ret ); 00808 00809 ssl->session_negotiate->id_len = n = 32; 00810 } 00811 } 00812 #endif /* MBEDTLS_SSL_SESSION_TICKETS */ 00813 00814 *p++ = (unsigned char) n; 00815 00816 for( i = 0; i < n; i++ ) 00817 *p++ = ssl->session_negotiate->id[i]; 00818 00819 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, session id len.: %d", n ) ); 00820 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, session id", buf + 39, n ); 00821 00822 /* 00823 * DTLS cookie 00824 */ 00825 #if defined(MBEDTLS_SSL_PROTO_DTLS) 00826 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 00827 { 00828 if( ssl->handshake->verify_cookie == NULL ) 00829 { 00830 MBEDTLS_SSL_DEBUG_MSG( 3, ( "no verify cookie to send" ) ); 00831 *p++ = 0; 00832 } 00833 else 00834 { 00835 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, cookie", 00836 ssl->handshake->verify_cookie, 00837 ssl->handshake->verify_cookie_len ); 00838 00839 *p++ = ssl->handshake->verify_cookie_len; 00840 memcpy( p, ssl->handshake->verify_cookie, 00841 ssl->handshake->verify_cookie_len ); 00842 p += ssl->handshake->verify_cookie_len; 00843 } 00844 } 00845 #endif 00846 00847 /* 00848 * Ciphersuite list 00849 */ 00850 ciphersuites = ssl->conf->ciphersuite_list[ssl->minor_ver]; 00851 00852 /* Skip writing ciphersuite length for now */ 00853 n = 0; 00854 q = p; 00855 p += 2; 00856 00857 for( i = 0; ciphersuites[i] != 0; i++ ) 00858 { 00859 ciphersuite_info = mbedtls_ssl_ciphersuite_from_id( ciphersuites[i] ); 00860 00861 if( ciphersuite_info == NULL ) 00862 continue; 00863 00864 if( ciphersuite_info->min_minor_ver > ssl->conf->max_minor_ver || 00865 ciphersuite_info->max_minor_ver < ssl->conf->min_minor_ver ) 00866 continue; 00867 00868 #if defined(MBEDTLS_SSL_PROTO_DTLS) 00869 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM && 00870 ( ciphersuite_info->flags & MBEDTLS_CIPHERSUITE_NODTLS ) ) 00871 continue; 00872 #endif 00873 00874 #if defined(MBEDTLS_ARC4_C) 00875 if( ssl->conf->arc4_disabled == MBEDTLS_SSL_ARC4_DISABLED && 00876 ciphersuite_info->cipher == MBEDTLS_CIPHER_ARC4_128 ) 00877 continue; 00878 #endif 00879 00880 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 00881 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE && 00882 mbedtls_ecjpake_check( &ssl->handshake->ecjpake_ctx ) != 0 ) 00883 continue; 00884 #endif 00885 00886 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, add ciphersuite: %04x", 00887 ciphersuites[i] ) ); 00888 00889 n++; 00890 *p++ = (unsigned char)( ciphersuites[i] >> 8 ); 00891 *p++ = (unsigned char)( ciphersuites[i] ); 00892 } 00893 00894 /* 00895 * Add TLS_EMPTY_RENEGOTIATION_INFO_SCSV 00896 */ 00897 #if defined(MBEDTLS_SSL_RENEGOTIATION) 00898 if( ssl->renego_status == MBEDTLS_SSL_INITIAL_HANDSHAKE ) 00899 #endif 00900 { 00901 *p++ = (unsigned char)( MBEDTLS_SSL_EMPTY_RENEGOTIATION_INFO >> 8 ); 00902 *p++ = (unsigned char)( MBEDTLS_SSL_EMPTY_RENEGOTIATION_INFO ); 00903 n++; 00904 } 00905 00906 /* Some versions of OpenSSL don't handle it correctly if not at end */ 00907 #if defined(MBEDTLS_SSL_FALLBACK_SCSV) 00908 if( ssl->conf->fallback == MBEDTLS_SSL_IS_FALLBACK ) 00909 { 00910 MBEDTLS_SSL_DEBUG_MSG( 3, ( "adding FALLBACK_SCSV" ) ); 00911 *p++ = (unsigned char)( MBEDTLS_SSL_FALLBACK_SCSV_VALUE >> 8 ); 00912 *p++ = (unsigned char)( MBEDTLS_SSL_FALLBACK_SCSV_VALUE ); 00913 n++; 00914 } 00915 #endif 00916 00917 *q++ = (unsigned char)( n >> 7 ); 00918 *q++ = (unsigned char)( n << 1 ); 00919 00920 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, got %d ciphersuites", n ) ); 00921 00922 #if defined(MBEDTLS_ZLIB_SUPPORT) 00923 offer_compress = 1; 00924 #else 00925 offer_compress = 0; 00926 #endif 00927 00928 /* 00929 * We don't support compression with DTLS right now: is many records come 00930 * in the same datagram, uncompressing one could overwrite the next one. 00931 * We don't want to add complexity for handling that case unless there is 00932 * an actual need for it. 00933 */ 00934 #if defined(MBEDTLS_SSL_PROTO_DTLS) 00935 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 00936 offer_compress = 0; 00937 #endif 00938 00939 if( offer_compress ) 00940 { 00941 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, compress len.: %d", 2 ) ); 00942 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, compress alg.: %d %d", 00943 MBEDTLS_SSL_COMPRESS_DEFLATE, MBEDTLS_SSL_COMPRESS_NULL ) ); 00944 00945 *p++ = 2; 00946 *p++ = MBEDTLS_SSL_COMPRESS_DEFLATE; 00947 *p++ = MBEDTLS_SSL_COMPRESS_NULL; 00948 } 00949 else 00950 { 00951 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, compress len.: %d", 1 ) ); 00952 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, compress alg.: %d", 00953 MBEDTLS_SSL_COMPRESS_NULL ) ); 00954 00955 *p++ = 1; 00956 *p++ = MBEDTLS_SSL_COMPRESS_NULL; 00957 } 00958 00959 // First write extensions, then the total length 00960 // 00961 #if defined(MBEDTLS_SSL_SERVER_NAME_INDICATION) 00962 ssl_write_hostname_ext( ssl, p + 2 + ext_len, &olen ); 00963 ext_len += olen; 00964 #endif 00965 00966 #if defined(MBEDTLS_SSL_RENEGOTIATION) 00967 ssl_write_renegotiation_ext( ssl, p + 2 + ext_len, &olen ); 00968 ext_len += olen; 00969 #endif 00970 00971 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) && \ 00972 defined(MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED) 00973 ssl_write_signature_algorithms_ext( ssl, p + 2 + ext_len, &olen ); 00974 ext_len += olen; 00975 #endif 00976 00977 #if defined(MBEDTLS_ECDH_C) || defined(MBEDTLS_ECDSA_C) || \ 00978 defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 00979 ssl_write_supported_elliptic_curves_ext( ssl, p + 2 + ext_len, &olen ); 00980 ext_len += olen; 00981 00982 ssl_write_supported_point_formats_ext( ssl, p + 2 + ext_len, &olen ); 00983 ext_len += olen; 00984 #endif 00985 00986 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 00987 ssl_write_ecjpake_kkpp_ext( ssl, p + 2 + ext_len, &olen ); 00988 ext_len += olen; 00989 #endif 00990 00991 #if defined(MBEDTLS_SSL_MAX_FRAGMENT_LENGTH) 00992 ssl_write_max_fragment_length_ext( ssl, p + 2 + ext_len, &olen ); 00993 ext_len += olen; 00994 #endif 00995 00996 #if defined(MBEDTLS_SSL_TRUNCATED_HMAC) 00997 ssl_write_truncated_hmac_ext( ssl, p + 2 + ext_len, &olen ); 00998 ext_len += olen; 00999 #endif 01000 01001 #if defined(MBEDTLS_SSL_ENCRYPT_THEN_MAC) 01002 ssl_write_encrypt_then_mac_ext( ssl, p + 2 + ext_len, &olen ); 01003 ext_len += olen; 01004 #endif 01005 01006 #if defined(MBEDTLS_SSL_EXTENDED_MASTER_SECRET) 01007 ssl_write_extended_ms_ext( ssl, p + 2 + ext_len, &olen ); 01008 ext_len += olen; 01009 #endif 01010 01011 #if defined(MBEDTLS_SSL_ALPN) 01012 ssl_write_alpn_ext( ssl, p + 2 + ext_len, &olen ); 01013 ext_len += olen; 01014 #endif 01015 01016 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 01017 ssl_write_session_ticket_ext( ssl, p + 2 + ext_len, &olen ); 01018 ext_len += olen; 01019 #endif 01020 01021 /* olen unused if all extensions are disabled */ 01022 ((void) olen); 01023 01024 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello, total extension length: %d", 01025 ext_len ) ); 01026 01027 if( ext_len > 0 ) 01028 { 01029 *p++ = (unsigned char)( ( ext_len >> 8 ) & 0xFF ); 01030 *p++ = (unsigned char)( ( ext_len ) & 0xFF ); 01031 p += ext_len; 01032 } 01033 01034 ssl->out_msglen = p - buf; 01035 ssl->out_msgtype = MBEDTLS_SSL_MSG_HANDSHAKE; 01036 ssl->out_msg[0] = MBEDTLS_SSL_HS_CLIENT_HELLO; 01037 01038 ssl->state++; 01039 01040 #if defined(MBEDTLS_SSL_PROTO_DTLS) 01041 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 01042 mbedtls_ssl_send_flight_completed( ssl ); 01043 #endif 01044 01045 if( ( ret = mbedtls_ssl_write_record( ssl ) ) != 0 ) 01046 { 01047 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_write_record", ret ); 01048 return( ret ); 01049 } 01050 01051 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write client hello" ) ); 01052 01053 return( 0 ); 01054 } 01055 01056 static int ssl_parse_renegotiation_info( mbedtls_ssl_context *ssl, 01057 const unsigned char *buf, 01058 size_t len ) 01059 { 01060 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01061 if( ssl->renego_status != MBEDTLS_SSL_INITIAL_HANDSHAKE ) 01062 { 01063 /* Check verify-data in constant-time. The length OTOH is no secret */ 01064 if( len != 1 + ssl->verify_data_len * 2 || 01065 buf[0] != ssl->verify_data_len * 2 || 01066 mbedtls_ssl_safer_memcmp( buf + 1, 01067 ssl->own_verify_data, ssl->verify_data_len ) != 0 || 01068 mbedtls_ssl_safer_memcmp( buf + 1 + ssl->verify_data_len, 01069 ssl->peer_verify_data, ssl->verify_data_len ) != 0 ) 01070 { 01071 MBEDTLS_SSL_DEBUG_MSG( 1, ( "non-matching renegotiation info" ) ); 01072 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01073 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 01074 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01075 } 01076 } 01077 else 01078 #endif /* MBEDTLS_SSL_RENEGOTIATION */ 01079 { 01080 if( len != 1 || buf[0] != 0x00 ) 01081 { 01082 MBEDTLS_SSL_DEBUG_MSG( 1, ( "non-zero length renegotiation info" ) ); 01083 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01084 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 01085 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01086 } 01087 01088 ssl->secure_renegotiation = MBEDTLS_SSL_SECURE_RENEGOTIATION; 01089 } 01090 01091 return( 0 ); 01092 } 01093 01094 #if defined(MBEDTLS_SSL_MAX_FRAGMENT_LENGTH) 01095 static int ssl_parse_max_fragment_length_ext( mbedtls_ssl_context *ssl, 01096 const unsigned char *buf, 01097 size_t len ) 01098 { 01099 /* 01100 * server should use the extension only if we did, 01101 * and if so the server's value should match ours (and len is always 1) 01102 */ 01103 if( ssl->conf->mfl_code == MBEDTLS_SSL_MAX_FRAG_LEN_NONE || 01104 len != 1 || 01105 buf[0] != ssl->conf->mfl_code ) 01106 { 01107 MBEDTLS_SSL_DEBUG_MSG( 1, ( "non-matching max fragment length extension" ) ); 01108 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01109 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 01110 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01111 } 01112 01113 return( 0 ); 01114 } 01115 #endif /* MBEDTLS_SSL_MAX_FRAGMENT_LENGTH */ 01116 01117 #if defined(MBEDTLS_SSL_TRUNCATED_HMAC) 01118 static int ssl_parse_truncated_hmac_ext( mbedtls_ssl_context *ssl, 01119 const unsigned char *buf, 01120 size_t len ) 01121 { 01122 if( ssl->conf->trunc_hmac == MBEDTLS_SSL_TRUNC_HMAC_DISABLED || 01123 len != 0 ) 01124 { 01125 MBEDTLS_SSL_DEBUG_MSG( 1, ( "non-matching truncated HMAC extension" ) ); 01126 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01127 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 01128 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01129 } 01130 01131 ((void) buf); 01132 01133 ssl->session_negotiate->trunc_hmac = MBEDTLS_SSL_TRUNC_HMAC_ENABLED; 01134 01135 return( 0 ); 01136 } 01137 #endif /* MBEDTLS_SSL_TRUNCATED_HMAC */ 01138 01139 #if defined(MBEDTLS_SSL_ENCRYPT_THEN_MAC) 01140 static int ssl_parse_encrypt_then_mac_ext( mbedtls_ssl_context *ssl, 01141 const unsigned char *buf, 01142 size_t len ) 01143 { 01144 if( ssl->conf->encrypt_then_mac == MBEDTLS_SSL_ETM_DISABLED || 01145 ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 || 01146 len != 0 ) 01147 { 01148 MBEDTLS_SSL_DEBUG_MSG( 1, ( "non-matching encrypt-then-MAC extension" ) ); 01149 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01150 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 01151 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01152 } 01153 01154 ((void) buf); 01155 01156 ssl->session_negotiate->encrypt_then_mac = MBEDTLS_SSL_ETM_ENABLED; 01157 01158 return( 0 ); 01159 } 01160 #endif /* MBEDTLS_SSL_ENCRYPT_THEN_MAC */ 01161 01162 #if defined(MBEDTLS_SSL_EXTENDED_MASTER_SECRET) 01163 static int ssl_parse_extended_ms_ext( mbedtls_ssl_context *ssl, 01164 const unsigned char *buf, 01165 size_t len ) 01166 { 01167 if( ssl->conf->extended_ms == MBEDTLS_SSL_EXTENDED_MS_DISABLED || 01168 ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 || 01169 len != 0 ) 01170 { 01171 MBEDTLS_SSL_DEBUG_MSG( 1, ( "non-matching extended master secret extension" ) ); 01172 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01173 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 01174 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01175 } 01176 01177 ((void) buf); 01178 01179 ssl->handshake->extended_ms = MBEDTLS_SSL_EXTENDED_MS_ENABLED; 01180 01181 return( 0 ); 01182 } 01183 #endif /* MBEDTLS_SSL_EXTENDED_MASTER_SECRET */ 01184 01185 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 01186 static int ssl_parse_session_ticket_ext( mbedtls_ssl_context *ssl, 01187 const unsigned char *buf, 01188 size_t len ) 01189 { 01190 if( ssl->conf->session_tickets == MBEDTLS_SSL_SESSION_TICKETS_DISABLED || 01191 len != 0 ) 01192 { 01193 MBEDTLS_SSL_DEBUG_MSG( 1, ( "non-matching session ticket extension" ) ); 01194 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01195 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 01196 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01197 } 01198 01199 ((void) buf); 01200 01201 ssl->handshake->new_session_ticket = 1; 01202 01203 return( 0 ); 01204 } 01205 #endif /* MBEDTLS_SSL_SESSION_TICKETS */ 01206 01207 #if defined(MBEDTLS_ECDH_C) || defined(MBEDTLS_ECDSA_C) || \ 01208 defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 01209 static int ssl_parse_supported_point_formats_ext( mbedtls_ssl_context *ssl, 01210 const unsigned char *buf, 01211 size_t len ) 01212 { 01213 size_t list_size; 01214 const unsigned char *p; 01215 01216 list_size = buf[0]; 01217 if( list_size + 1 != len ) 01218 { 01219 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server hello message" ) ); 01220 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01221 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 01222 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01223 } 01224 01225 p = buf + 1; 01226 while( list_size > 0 ) 01227 { 01228 if( p[0] == MBEDTLS_ECP_PF_UNCOMPRESSED || 01229 p[0] == MBEDTLS_ECP_PF_COMPRESSED ) 01230 { 01231 #if defined(MBEDTLS_ECDH_C) || defined(MBEDTLS_ECDSA_C) 01232 ssl->handshake->ecdh_ctx.point_format = p[0]; 01233 #endif 01234 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 01235 ssl->handshake->ecjpake_ctx.point_format = p[0]; 01236 #endif 01237 MBEDTLS_SSL_DEBUG_MSG( 4, ( "point format selected: %d", p[0] ) ); 01238 return( 0 ); 01239 } 01240 01241 list_size--; 01242 p++; 01243 } 01244 01245 MBEDTLS_SSL_DEBUG_MSG( 1, ( "no point format in common" ) ); 01246 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01247 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 01248 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01249 } 01250 #endif /* MBEDTLS_ECDH_C || MBEDTLS_ECDSA_C || 01251 MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 01252 01253 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 01254 static int ssl_parse_ecjpake_kkpp( mbedtls_ssl_context *ssl, 01255 const unsigned char *buf, 01256 size_t len ) 01257 { 01258 int ret; 01259 01260 if( ssl->transform_negotiate->ciphersuite_info->key_exchange != 01261 MBEDTLS_KEY_EXCHANGE_ECJPAKE ) 01262 { 01263 MBEDTLS_SSL_DEBUG_MSG( 3, ( "skip ecjpake kkpp extension" ) ); 01264 return( 0 ); 01265 } 01266 01267 /* If we got here, we no longer need our cached extension */ 01268 mbedtls_free( ssl->handshake->ecjpake_cache ); 01269 ssl->handshake->ecjpake_cache = NULL; 01270 ssl->handshake->ecjpake_cache_len = 0; 01271 01272 if( ( ret = mbedtls_ecjpake_read_round_one( &ssl->handshake->ecjpake_ctx, 01273 buf, len ) ) != 0 ) 01274 { 01275 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecjpake_read_round_one", ret ); 01276 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01277 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 01278 return( ret ); 01279 } 01280 01281 return( 0 ); 01282 } 01283 #endif /* MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 01284 01285 #if defined(MBEDTLS_SSL_ALPN) 01286 static int ssl_parse_alpn_ext( mbedtls_ssl_context *ssl, 01287 const unsigned char *buf, size_t len ) 01288 { 01289 size_t list_len, name_len; 01290 const char **p; 01291 01292 /* If we didn't send it, the server shouldn't send it */ 01293 if( ssl->conf->alpn_list == NULL ) 01294 { 01295 MBEDTLS_SSL_DEBUG_MSG( 1, ( "non-matching ALPN extension" ) ); 01296 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01297 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 01298 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01299 } 01300 01301 /* 01302 * opaque ProtocolName<1..2^8-1>; 01303 * 01304 * struct { 01305 * ProtocolName protocol_name_list<2..2^16-1> 01306 * } ProtocolNameList; 01307 * 01308 * the "ProtocolNameList" MUST contain exactly one "ProtocolName" 01309 */ 01310 01311 /* Min length is 2 (list_len) + 1 (name_len) + 1 (name) */ 01312 if( len < 4 ) 01313 { 01314 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01315 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 01316 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01317 } 01318 01319 list_len = ( buf[0] << 8 ) | buf[1]; 01320 if( list_len != len - 2 ) 01321 { 01322 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01323 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 01324 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01325 } 01326 01327 name_len = buf[2]; 01328 if( name_len != list_len - 1 ) 01329 { 01330 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01331 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 01332 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01333 } 01334 01335 /* Check that the server chosen protocol was in our list and save it */ 01336 for( p = ssl->conf->alpn_list; *p != NULL; p++ ) 01337 { 01338 if( name_len == strlen( *p ) && 01339 memcmp( buf + 3, *p, name_len ) == 0 ) 01340 { 01341 ssl->alpn_chosen = *p; 01342 return( 0 ); 01343 } 01344 } 01345 01346 MBEDTLS_SSL_DEBUG_MSG( 1, ( "ALPN extension: no matching protocol" ) ); 01347 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01348 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 01349 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01350 } 01351 #endif /* MBEDTLS_SSL_ALPN */ 01352 01353 /* 01354 * Parse HelloVerifyRequest. Only called after verifying the HS type. 01355 */ 01356 #if defined(MBEDTLS_SSL_PROTO_DTLS) 01357 static int ssl_parse_hello_verify_request( mbedtls_ssl_context *ssl ) 01358 { 01359 const unsigned char *p = ssl->in_msg + mbedtls_ssl_hs_hdr_len( ssl ); 01360 int major_ver, minor_ver; 01361 unsigned char cookie_len; 01362 01363 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse hello verify request" ) ); 01364 01365 /* 01366 * struct { 01367 * ProtocolVersion server_version; 01368 * opaque cookie<0..2^8-1>; 01369 * } HelloVerifyRequest; 01370 */ 01371 MBEDTLS_SSL_DEBUG_BUF( 3, "server version", p, 2 ); 01372 mbedtls_ssl_read_version( &major_ver, &minor_ver, ssl->conf->transport, p ); 01373 p += 2; 01374 01375 /* 01376 * Since the RFC is not clear on this point, accept DTLS 1.0 (TLS 1.1) 01377 * even is lower than our min version. 01378 */ 01379 if( major_ver < MBEDTLS_SSL_MAJOR_VERSION_3 || 01380 minor_ver < MBEDTLS_SSL_MINOR_VERSION_2 || 01381 major_ver > ssl->conf->max_major_ver || 01382 minor_ver > ssl->conf->max_minor_ver ) 01383 { 01384 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server version" ) ); 01385 01386 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01387 MBEDTLS_SSL_ALERT_MSG_PROTOCOL_VERSION ); 01388 01389 return( MBEDTLS_ERR_SSL_BAD_HS_PROTOCOL_VERSION ); 01390 } 01391 01392 cookie_len = *p++; 01393 MBEDTLS_SSL_DEBUG_BUF( 3, "cookie", p, cookie_len ); 01394 01395 if( ( ssl->in_msg + ssl->in_msglen ) - p < cookie_len ) 01396 { 01397 MBEDTLS_SSL_DEBUG_MSG( 1, 01398 ( "cookie length does not match incoming message size" ) ); 01399 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01400 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 01401 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01402 } 01403 01404 mbedtls_free( ssl->handshake->verify_cookie ); 01405 01406 ssl->handshake->verify_cookie = mbedtls_calloc( 1, cookie_len ); 01407 if( ssl->handshake->verify_cookie == NULL ) 01408 { 01409 MBEDTLS_SSL_DEBUG_MSG( 1, ( "alloc failed (%d bytes)", cookie_len ) ); 01410 return( MBEDTLS_ERR_SSL_ALLOC_FAILED ); 01411 } 01412 01413 memcpy( ssl->handshake->verify_cookie, p, cookie_len ); 01414 ssl->handshake->verify_cookie_len = cookie_len; 01415 01416 /* Start over at ClientHello */ 01417 ssl->state = MBEDTLS_SSL_CLIENT_HELLO; 01418 mbedtls_ssl_reset_checksum( ssl ); 01419 01420 mbedtls_ssl_recv_flight_completed( ssl ); 01421 01422 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= parse hello verify request" ) ); 01423 01424 return( 0 ); 01425 } 01426 #endif /* MBEDTLS_SSL_PROTO_DTLS */ 01427 01428 static int ssl_parse_server_hello( mbedtls_ssl_context *ssl ) 01429 { 01430 int ret, i; 01431 size_t n; 01432 size_t ext_len; 01433 unsigned char *buf, *ext; 01434 unsigned char comp; 01435 #if defined(MBEDTLS_ZLIB_SUPPORT) 01436 int accept_comp; 01437 #endif 01438 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01439 int renegotiation_info_seen = 0; 01440 #endif 01441 int handshake_failure = 0; 01442 const mbedtls_ssl_ciphersuite_t *suite_info; 01443 #if defined(MBEDTLS_DEBUG_C) 01444 uint32_t t; 01445 #endif 01446 01447 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse server hello" ) ); 01448 01449 buf = ssl->in_msg; 01450 01451 if( ( ret = mbedtls_ssl_read_record( ssl ) ) != 0 ) 01452 { 01453 /* No alert on a read error. */ 01454 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_read_record", ret ); 01455 return( ret ); 01456 } 01457 01458 if( ssl->in_msgtype != MBEDTLS_SSL_MSG_HANDSHAKE ) 01459 { 01460 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01461 if( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS ) 01462 { 01463 ssl->renego_records_seen++; 01464 01465 if( ssl->conf->renego_max_records >= 0 && 01466 ssl->renego_records_seen > ssl->conf->renego_max_records ) 01467 { 01468 MBEDTLS_SSL_DEBUG_MSG( 1, ( "renegotiation requested, " 01469 "but not honored by server" ) ); 01470 return( MBEDTLS_ERR_SSL_UNEXPECTED_MESSAGE ); 01471 } 01472 01473 MBEDTLS_SSL_DEBUG_MSG( 1, ( "non-handshake message during renego" ) ); 01474 01475 ssl->keep_current_message = 1; 01476 return( MBEDTLS_ERR_SSL_WAITING_SERVER_HELLO_RENEGO ); 01477 } 01478 #endif /* MBEDTLS_SSL_RENEGOTIATION */ 01479 01480 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server hello message" ) ); 01481 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01482 MBEDTLS_SSL_ALERT_MSG_UNEXPECTED_MESSAGE ); 01483 return( MBEDTLS_ERR_SSL_UNEXPECTED_MESSAGE ); 01484 } 01485 01486 #if defined(MBEDTLS_SSL_PROTO_DTLS) 01487 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 01488 { 01489 if( buf[0] == MBEDTLS_SSL_HS_HELLO_VERIFY_REQUEST ) 01490 { 01491 MBEDTLS_SSL_DEBUG_MSG( 2, ( "received hello verify request" ) ); 01492 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= parse server hello" ) ); 01493 return( ssl_parse_hello_verify_request( ssl ) ); 01494 } 01495 else 01496 { 01497 /* We made it through the verification process */ 01498 mbedtls_free( ssl->handshake->verify_cookie ); 01499 ssl->handshake->verify_cookie = NULL; 01500 ssl->handshake->verify_cookie_len = 0; 01501 } 01502 } 01503 #endif /* MBEDTLS_SSL_PROTO_DTLS */ 01504 01505 if( ssl->in_hslen < 38 + mbedtls_ssl_hs_hdr_len( ssl ) || 01506 buf[0] != MBEDTLS_SSL_HS_SERVER_HELLO ) 01507 { 01508 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server hello message" ) ); 01509 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01510 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 01511 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01512 } 01513 01514 /* 01515 * 0 . 1 server_version 01516 * 2 . 33 random (maybe including 4 bytes of Unix time) 01517 * 34 . 34 session_id length = n 01518 * 35 . 34+n session_id 01519 * 35+n . 36+n cipher_suite 01520 * 37+n . 37+n compression_method 01521 * 01522 * 38+n . 39+n extensions length (optional) 01523 * 40+n . .. extensions 01524 */ 01525 buf += mbedtls_ssl_hs_hdr_len( ssl ); 01526 01527 MBEDTLS_SSL_DEBUG_BUF( 3, "server hello, version", buf + 0, 2 ); 01528 mbedtls_ssl_read_version( &ssl->major_ver, &ssl->minor_ver, 01529 ssl->conf->transport, buf + 0 ); 01530 01531 if( ssl->major_ver < ssl->conf->min_major_ver || 01532 ssl->minor_ver < ssl->conf->min_minor_ver || 01533 ssl->major_ver > ssl->conf->max_major_ver || 01534 ssl->minor_ver > ssl->conf->max_minor_ver ) 01535 { 01536 MBEDTLS_SSL_DEBUG_MSG( 1, ( "server version out of bounds - " 01537 " min: [%d:%d], server: [%d:%d], max: [%d:%d]", 01538 ssl->conf->min_major_ver, ssl->conf->min_minor_ver, 01539 ssl->major_ver, ssl->minor_ver, 01540 ssl->conf->max_major_ver, ssl->conf->max_minor_ver ) ); 01541 01542 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01543 MBEDTLS_SSL_ALERT_MSG_PROTOCOL_VERSION ); 01544 01545 return( MBEDTLS_ERR_SSL_BAD_HS_PROTOCOL_VERSION ); 01546 } 01547 01548 #if defined(MBEDTLS_DEBUG_C) 01549 t = ( (uint32_t) buf[2] << 24 ) 01550 | ( (uint32_t) buf[3] << 16 ) 01551 | ( (uint32_t) buf[4] << 8 ) 01552 | ( (uint32_t) buf[5] ); 01553 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, current time: %lu", t ) ); 01554 #endif 01555 01556 memcpy( ssl->handshake->randbytes + 32, buf + 2, 32 ); 01557 01558 n = buf[34]; 01559 01560 MBEDTLS_SSL_DEBUG_BUF( 3, "server hello, random bytes", buf + 2, 32 ); 01561 01562 if( n > 32 ) 01563 { 01564 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server hello message" ) ); 01565 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01566 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 01567 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01568 } 01569 01570 if( ssl->in_hslen > mbedtls_ssl_hs_hdr_len( ssl ) + 39 + n ) 01571 { 01572 ext_len = ( ( buf[38 + n] << 8 ) 01573 | ( buf[39 + n] ) ); 01574 01575 if( ( ext_len > 0 && ext_len < 4 ) || 01576 ssl->in_hslen != mbedtls_ssl_hs_hdr_len( ssl ) + 40 + n + ext_len ) 01577 { 01578 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server hello message" ) ); 01579 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01580 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 01581 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01582 } 01583 } 01584 else if( ssl->in_hslen == mbedtls_ssl_hs_hdr_len( ssl ) + 38 + n ) 01585 { 01586 ext_len = 0; 01587 } 01588 else 01589 { 01590 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server hello message" ) ); 01591 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01592 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 01593 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01594 } 01595 01596 /* ciphersuite (used later) */ 01597 i = ( buf[35 + n] << 8 ) | buf[36 + n]; 01598 01599 /* 01600 * Read and check compression 01601 */ 01602 comp = buf[37 + n]; 01603 01604 #if defined(MBEDTLS_ZLIB_SUPPORT) 01605 /* See comments in ssl_write_client_hello() */ 01606 #if defined(MBEDTLS_SSL_PROTO_DTLS) 01607 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 01608 accept_comp = 0; 01609 else 01610 #endif 01611 accept_comp = 1; 01612 01613 if( comp != MBEDTLS_SSL_COMPRESS_NULL && 01614 ( comp != MBEDTLS_SSL_COMPRESS_DEFLATE || accept_comp == 0 ) ) 01615 #else /* MBEDTLS_ZLIB_SUPPORT */ 01616 if( comp != MBEDTLS_SSL_COMPRESS_NULL ) 01617 #endif/* MBEDTLS_ZLIB_SUPPORT */ 01618 { 01619 MBEDTLS_SSL_DEBUG_MSG( 1, ( "server hello, bad compression: %d", comp ) ); 01620 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01621 MBEDTLS_SSL_ALERT_MSG_ILLEGAL_PARAMETER ); 01622 return( MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE ); 01623 } 01624 01625 /* 01626 * Initialize update checksum functions 01627 */ 01628 ssl->transform_negotiate->ciphersuite_info = mbedtls_ssl_ciphersuite_from_id( i ); 01629 01630 if( ssl->transform_negotiate->ciphersuite_info == NULL ) 01631 { 01632 MBEDTLS_SSL_DEBUG_MSG( 1, ( "ciphersuite info for %04x not found", i ) ); 01633 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01634 MBEDTLS_SSL_ALERT_MSG_INTERNAL_ERROR ); 01635 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 01636 } 01637 01638 mbedtls_ssl_optimize_checksum( ssl, ssl->transform_negotiate->ciphersuite_info ); 01639 01640 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, session id len.: %d", n ) ); 01641 MBEDTLS_SSL_DEBUG_BUF( 3, "server hello, session id", buf + 35, n ); 01642 01643 /* 01644 * Check if the session can be resumed 01645 */ 01646 if( ssl->handshake->resume == 0 || n == 0 || 01647 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01648 ssl->renego_status != MBEDTLS_SSL_INITIAL_HANDSHAKE || 01649 #endif 01650 ssl->session_negotiate->ciphersuite != i || 01651 ssl->session_negotiate->compression != comp || 01652 ssl->session_negotiate->id_len != n || 01653 memcmp( ssl->session_negotiate->id, buf + 35, n ) != 0 ) 01654 { 01655 ssl->state++; 01656 ssl->handshake->resume = 0; 01657 #if defined(MBEDTLS_HAVE_TIME) 01658 ssl->session_negotiate->start = mbedtls_time( NULL ); 01659 #endif 01660 ssl->session_negotiate->ciphersuite = i; 01661 ssl->session_negotiate->compression = comp; 01662 ssl->session_negotiate->id_len = n; 01663 memcpy( ssl->session_negotiate->id, buf + 35, n ); 01664 } 01665 else 01666 { 01667 ssl->state = MBEDTLS_SSL_SERVER_CHANGE_CIPHER_SPEC; 01668 01669 if( ( ret = mbedtls_ssl_derive_keys( ssl ) ) != 0 ) 01670 { 01671 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_derive_keys", ret ); 01672 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01673 MBEDTLS_SSL_ALERT_MSG_INTERNAL_ERROR ); 01674 return( ret ); 01675 } 01676 } 01677 01678 MBEDTLS_SSL_DEBUG_MSG( 3, ( "%s session has been resumed", 01679 ssl->handshake->resume ? "a" : "no" ) ); 01680 01681 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, chosen ciphersuite: %04x", i ) ); 01682 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, compress alg.: %d", buf[37 + n] ) ); 01683 01684 suite_info = mbedtls_ssl_ciphersuite_from_id( ssl->session_negotiate->ciphersuite ); 01685 if( suite_info == NULL 01686 #if defined(MBEDTLS_ARC4_C) 01687 || ( ssl->conf->arc4_disabled && 01688 suite_info->cipher == MBEDTLS_CIPHER_ARC4_128 ) 01689 #endif 01690 ) 01691 { 01692 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server hello message" ) ); 01693 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01694 MBEDTLS_SSL_ALERT_MSG_ILLEGAL_PARAMETER ); 01695 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01696 } 01697 01698 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, chosen ciphersuite: %s", suite_info->name ) ); 01699 01700 i = 0; 01701 while( 1 ) 01702 { 01703 if( ssl->conf->ciphersuite_list[ssl->minor_ver][i] == 0 ) 01704 { 01705 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server hello message" ) ); 01706 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01707 MBEDTLS_SSL_ALERT_MSG_ILLEGAL_PARAMETER ); 01708 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01709 } 01710 01711 if( ssl->conf->ciphersuite_list[ssl->minor_ver][i++] == 01712 ssl->session_negotiate->ciphersuite ) 01713 { 01714 break; 01715 } 01716 } 01717 01718 if( comp != MBEDTLS_SSL_COMPRESS_NULL 01719 #if defined(MBEDTLS_ZLIB_SUPPORT) 01720 && comp != MBEDTLS_SSL_COMPRESS_DEFLATE 01721 #endif 01722 ) 01723 { 01724 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server hello message" ) ); 01725 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01726 MBEDTLS_SSL_ALERT_MSG_ILLEGAL_PARAMETER ); 01727 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01728 } 01729 ssl->session_negotiate->compression = comp; 01730 01731 ext = buf + 40 + n; 01732 01733 MBEDTLS_SSL_DEBUG_MSG( 2, ( "server hello, total extension length: %d", ext_len ) ); 01734 01735 while( ext_len ) 01736 { 01737 unsigned int ext_id = ( ( ext[0] << 8 ) 01738 | ( ext[1] ) ); 01739 unsigned int ext_size = ( ( ext[2] << 8 ) 01740 | ( ext[3] ) ); 01741 01742 if( ext_size + 4 > ext_len ) 01743 { 01744 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server hello message" ) ); 01745 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01746 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 01747 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01748 } 01749 01750 switch( ext_id ) 01751 { 01752 case MBEDTLS_TLS_EXT_RENEGOTIATION_INFO: 01753 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found renegotiation extension" ) ); 01754 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01755 renegotiation_info_seen = 1; 01756 #endif 01757 01758 if( ( ret = ssl_parse_renegotiation_info( ssl, ext + 4, 01759 ext_size ) ) != 0 ) 01760 return( ret ); 01761 01762 break; 01763 01764 #if defined(MBEDTLS_SSL_MAX_FRAGMENT_LENGTH) 01765 case MBEDTLS_TLS_EXT_MAX_FRAGMENT_LENGTH: 01766 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found max_fragment_length extension" ) ); 01767 01768 if( ( ret = ssl_parse_max_fragment_length_ext( ssl, 01769 ext + 4, ext_size ) ) != 0 ) 01770 { 01771 return( ret ); 01772 } 01773 01774 break; 01775 #endif /* MBEDTLS_SSL_MAX_FRAGMENT_LENGTH */ 01776 01777 #if defined(MBEDTLS_SSL_TRUNCATED_HMAC) 01778 case MBEDTLS_TLS_EXT_TRUNCATED_HMAC: 01779 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found truncated_hmac extension" ) ); 01780 01781 if( ( ret = ssl_parse_truncated_hmac_ext( ssl, 01782 ext + 4, ext_size ) ) != 0 ) 01783 { 01784 return( ret ); 01785 } 01786 01787 break; 01788 #endif /* MBEDTLS_SSL_TRUNCATED_HMAC */ 01789 01790 #if defined(MBEDTLS_SSL_ENCRYPT_THEN_MAC) 01791 case MBEDTLS_TLS_EXT_ENCRYPT_THEN_MAC: 01792 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found encrypt_then_mac extension" ) ); 01793 01794 if( ( ret = ssl_parse_encrypt_then_mac_ext( ssl, 01795 ext + 4, ext_size ) ) != 0 ) 01796 { 01797 return( ret ); 01798 } 01799 01800 break; 01801 #endif /* MBEDTLS_SSL_ENCRYPT_THEN_MAC */ 01802 01803 #if defined(MBEDTLS_SSL_EXTENDED_MASTER_SECRET) 01804 case MBEDTLS_TLS_EXT_EXTENDED_MASTER_SECRET: 01805 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found extended_master_secret extension" ) ); 01806 01807 if( ( ret = ssl_parse_extended_ms_ext( ssl, 01808 ext + 4, ext_size ) ) != 0 ) 01809 { 01810 return( ret ); 01811 } 01812 01813 break; 01814 #endif /* MBEDTLS_SSL_EXTENDED_MASTER_SECRET */ 01815 01816 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 01817 case MBEDTLS_TLS_EXT_SESSION_TICKET: 01818 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found session_ticket extension" ) ); 01819 01820 if( ( ret = ssl_parse_session_ticket_ext( ssl, 01821 ext + 4, ext_size ) ) != 0 ) 01822 { 01823 return( ret ); 01824 } 01825 01826 break; 01827 #endif /* MBEDTLS_SSL_SESSION_TICKETS */ 01828 01829 #if defined(MBEDTLS_ECDH_C) || defined(MBEDTLS_ECDSA_C) || \ 01830 defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 01831 case MBEDTLS_TLS_EXT_SUPPORTED_POINT_FORMATS: 01832 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found supported_point_formats extension" ) ); 01833 01834 if( ( ret = ssl_parse_supported_point_formats_ext( ssl, 01835 ext + 4, ext_size ) ) != 0 ) 01836 { 01837 return( ret ); 01838 } 01839 01840 break; 01841 #endif /* MBEDTLS_ECDH_C || MBEDTLS_ECDSA_C || 01842 MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 01843 01844 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 01845 case MBEDTLS_TLS_EXT_ECJPAKE_KKPP: 01846 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found ecjpake_kkpp extension" ) ); 01847 01848 if( ( ret = ssl_parse_ecjpake_kkpp( ssl, 01849 ext + 4, ext_size ) ) != 0 ) 01850 { 01851 return( ret ); 01852 } 01853 01854 break; 01855 #endif /* MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 01856 01857 #if defined(MBEDTLS_SSL_ALPN) 01858 case MBEDTLS_TLS_EXT_ALPN: 01859 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found alpn extension" ) ); 01860 01861 if( ( ret = ssl_parse_alpn_ext( ssl, ext + 4, ext_size ) ) != 0 ) 01862 return( ret ); 01863 01864 break; 01865 #endif /* MBEDTLS_SSL_ALPN */ 01866 01867 default: 01868 MBEDTLS_SSL_DEBUG_MSG( 3, ( "unknown extension found: %d (ignoring)", 01869 ext_id ) ); 01870 } 01871 01872 ext_len -= 4 + ext_size; 01873 ext += 4 + ext_size; 01874 01875 if( ext_len > 0 && ext_len < 4 ) 01876 { 01877 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server hello message" ) ); 01878 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01879 } 01880 } 01881 01882 /* 01883 * Renegotiation security checks 01884 */ 01885 if( ssl->secure_renegotiation == MBEDTLS_SSL_LEGACY_RENEGOTIATION && 01886 ssl->conf->allow_legacy_renegotiation == MBEDTLS_SSL_LEGACY_BREAK_HANDSHAKE ) 01887 { 01888 MBEDTLS_SSL_DEBUG_MSG( 1, ( "legacy renegotiation, breaking off handshake" ) ); 01889 handshake_failure = 1; 01890 } 01891 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01892 else if( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS && 01893 ssl->secure_renegotiation == MBEDTLS_SSL_SECURE_RENEGOTIATION && 01894 renegotiation_info_seen == 0 ) 01895 { 01896 MBEDTLS_SSL_DEBUG_MSG( 1, ( "renegotiation_info extension missing (secure)" ) ); 01897 handshake_failure = 1; 01898 } 01899 else if( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS && 01900 ssl->secure_renegotiation == MBEDTLS_SSL_LEGACY_RENEGOTIATION && 01901 ssl->conf->allow_legacy_renegotiation == MBEDTLS_SSL_LEGACY_NO_RENEGOTIATION ) 01902 { 01903 MBEDTLS_SSL_DEBUG_MSG( 1, ( "legacy renegotiation not allowed" ) ); 01904 handshake_failure = 1; 01905 } 01906 else if( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS && 01907 ssl->secure_renegotiation == MBEDTLS_SSL_LEGACY_RENEGOTIATION && 01908 renegotiation_info_seen == 1 ) 01909 { 01910 MBEDTLS_SSL_DEBUG_MSG( 1, ( "renegotiation_info extension present (legacy)" ) ); 01911 handshake_failure = 1; 01912 } 01913 #endif /* MBEDTLS_SSL_RENEGOTIATION */ 01914 01915 if( handshake_failure == 1 ) 01916 { 01917 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01918 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 01919 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO ); 01920 } 01921 01922 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= parse server hello" ) ); 01923 01924 return( 0 ); 01925 } 01926 01927 #if defined(MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED) || \ 01928 defined(MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED) 01929 static int ssl_parse_server_dh_params( mbedtls_ssl_context *ssl, unsigned char **p, 01930 unsigned char *end ) 01931 { 01932 int ret = MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE; 01933 01934 /* 01935 * Ephemeral DH parameters: 01936 * 01937 * struct { 01938 * opaque dh_p<1..2^16-1>; 01939 * opaque dh_g<1..2^16-1>; 01940 * opaque dh_Ys<1..2^16-1>; 01941 * } ServerDHParams; 01942 */ 01943 if( ( ret = mbedtls_dhm_read_params( &ssl->handshake->dhm_ctx, p, end ) ) != 0 ) 01944 { 01945 MBEDTLS_SSL_DEBUG_RET( 2, ( "mbedtls_dhm_read_params" ), ret ); 01946 return( ret ); 01947 } 01948 01949 if( ssl->handshake->dhm_ctx.len * 8 < ssl->conf->dhm_min_bitlen ) 01950 { 01951 MBEDTLS_SSL_DEBUG_MSG( 1, ( "DHM prime too short: %d < %d", 01952 ssl->handshake->dhm_ctx.len * 8, 01953 ssl->conf->dhm_min_bitlen ) ); 01954 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_KEY_EXCHANGE ); 01955 } 01956 01957 MBEDTLS_SSL_DEBUG_MPI( 3, "DHM: P ", &ssl->handshake->dhm_ctx.P ); 01958 MBEDTLS_SSL_DEBUG_MPI( 3, "DHM: G ", &ssl->handshake->dhm_ctx.G ); 01959 MBEDTLS_SSL_DEBUG_MPI( 3, "DHM: GY", &ssl->handshake->dhm_ctx.GY ); 01960 01961 return( ret ); 01962 } 01963 #endif /* MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED || 01964 MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED */ 01965 01966 #if defined(MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED) || \ 01967 defined(MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED) || \ 01968 defined(MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED) || \ 01969 defined(MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED) || \ 01970 defined(MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED) 01971 static int ssl_check_server_ecdh_params( const mbedtls_ssl_context *ssl ) 01972 { 01973 const mbedtls_ecp_curve_info *curve_info; 01974 01975 curve_info = mbedtls_ecp_curve_info_from_grp_id( ssl->handshake->ecdh_ctx.grp.id ); 01976 if( curve_info == NULL ) 01977 { 01978 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 01979 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 01980 } 01981 01982 MBEDTLS_SSL_DEBUG_MSG( 2, ( "ECDH curve: %s", curve_info->name ) ); 01983 01984 #if defined(MBEDTLS_ECP_C) 01985 if( mbedtls_ssl_check_curve( ssl, ssl->handshake->ecdh_ctx.grp.id ) != 0 ) 01986 #else 01987 if( ssl->handshake->ecdh_ctx.grp.nbits < 163 || 01988 ssl->handshake->ecdh_ctx.grp.nbits > 521 ) 01989 #endif 01990 return( -1 ); 01991 01992 MBEDTLS_SSL_DEBUG_ECP( 3, "ECDH: Qp", &ssl->handshake->ecdh_ctx.Qp ); 01993 01994 return( 0 ); 01995 } 01996 #endif /* MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED || 01997 MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED || 01998 MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED || 01999 MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED || 02000 MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED */ 02001 02002 #if defined(MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED) || \ 02003 defined(MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED) || \ 02004 defined(MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED) 02005 static int ssl_parse_server_ecdh_params( mbedtls_ssl_context *ssl, 02006 unsigned char **p, 02007 unsigned char *end ) 02008 { 02009 int ret = MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE; 02010 02011 /* 02012 * Ephemeral ECDH parameters: 02013 * 02014 * struct { 02015 * ECParameters curve_params; 02016 * ECPoint public; 02017 * } ServerECDHParams; 02018 */ 02019 if( ( ret = mbedtls_ecdh_read_params( &ssl->handshake->ecdh_ctx, 02020 (const unsigned char **) p, end ) ) != 0 ) 02021 { 02022 MBEDTLS_SSL_DEBUG_RET( 1, ( "mbedtls_ecdh_read_params" ), ret ); 02023 return( ret ); 02024 } 02025 02026 if( ssl_check_server_ecdh_params( ssl ) != 0 ) 02027 { 02028 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server key exchange message (ECDHE curve)" ) ); 02029 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_KEY_EXCHANGE ); 02030 } 02031 02032 return( ret ); 02033 } 02034 #endif /* MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED || 02035 MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED || 02036 MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED */ 02037 02038 #if defined(MBEDTLS_KEY_EXCHANGE__SOME__PSK_ENABLED) 02039 static int ssl_parse_server_psk_hint( mbedtls_ssl_context *ssl, 02040 unsigned char **p, 02041 unsigned char *end ) 02042 { 02043 int ret = MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE; 02044 size_t len; 02045 ((void) ssl); 02046 02047 /* 02048 * PSK parameters: 02049 * 02050 * opaque psk_identity_hint<0..2^16-1>; 02051 */ 02052 len = (*p)[0] << 8 | (*p)[1]; 02053 *p += 2; 02054 02055 if( (*p) + len > end ) 02056 { 02057 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server key exchange message " 02058 "(psk_identity_hint length)" ) ); 02059 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_KEY_EXCHANGE ); 02060 } 02061 02062 /* 02063 * Note: we currently ignore the PKS identity hint, as we only allow one 02064 * PSK to be provisionned on the client. This could be changed later if 02065 * someone needs that feature. 02066 */ 02067 *p += len; 02068 ret = 0; 02069 02070 return( ret ); 02071 } 02072 #endif /* MBEDTLS_KEY_EXCHANGE__SOME__PSK_ENABLED */ 02073 02074 #if defined(MBEDTLS_KEY_EXCHANGE_RSA_ENABLED) || \ 02075 defined(MBEDTLS_KEY_EXCHANGE_RSA_PSK_ENABLED) 02076 /* 02077 * Generate a pre-master secret and encrypt it with the server's RSA key 02078 */ 02079 static int ssl_write_encrypted_pms( mbedtls_ssl_context *ssl, 02080 size_t offset, size_t *olen, 02081 size_t pms_offset ) 02082 { 02083 int ret; 02084 size_t len_bytes = ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 ? 0 : 2; 02085 unsigned char *p = ssl->handshake->premaster + pms_offset; 02086 02087 if( offset + len_bytes > MBEDTLS_SSL_MAX_CONTENT_LEN ) 02088 { 02089 MBEDTLS_SSL_DEBUG_MSG( 1, ( "buffer too small for encrypted pms" ) ); 02090 return( MBEDTLS_ERR_SSL_BUFFER_TOO_SMALL ); 02091 } 02092 02093 /* 02094 * Generate (part of) the pre-master as 02095 * struct { 02096 * ProtocolVersion client_version; 02097 * opaque random[46]; 02098 * } PreMasterSecret; 02099 */ 02100 mbedtls_ssl_write_version( ssl->conf->max_major_ver, ssl->conf->max_minor_ver, 02101 ssl->conf->transport, p ); 02102 02103 if( ( ret = ssl->conf->f_rng( ssl->conf->p_rng, p + 2, 46 ) ) != 0 ) 02104 { 02105 MBEDTLS_SSL_DEBUG_RET( 1, "f_rng", ret ); 02106 return( ret ); 02107 } 02108 02109 ssl->handshake->pmslen = 48; 02110 02111 if( ssl->session_negotiate->peer_cert == NULL ) 02112 { 02113 MBEDTLS_SSL_DEBUG_MSG( 2, ( "certificate required" ) ); 02114 return( MBEDTLS_ERR_SSL_UNEXPECTED_MESSAGE ); 02115 } 02116 02117 /* 02118 * Now write it out, encrypted 02119 */ 02120 if( ! mbedtls_pk_can_do( &ssl->session_negotiate->peer_cert->pk, 02121 MBEDTLS_PK_RSA ) ) 02122 { 02123 MBEDTLS_SSL_DEBUG_MSG( 1, ( "certificate key type mismatch" ) ); 02124 return( MBEDTLS_ERR_SSL_PK_TYPE_MISMATCH ); 02125 } 02126 02127 if( ( ret = mbedtls_pk_encrypt( &ssl->session_negotiate->peer_cert->pk, 02128 p, ssl->handshake->pmslen, 02129 ssl->out_msg + offset + len_bytes, olen, 02130 MBEDTLS_SSL_MAX_CONTENT_LEN - offset - len_bytes, 02131 ssl->conf->f_rng, ssl->conf->p_rng ) ) != 0 ) 02132 { 02133 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_rsa_pkcs1_encrypt", ret ); 02134 return( ret ); 02135 } 02136 02137 #if defined(MBEDTLS_SSL_PROTO_TLS1) || defined(MBEDTLS_SSL_PROTO_TLS1_1) || \ 02138 defined(MBEDTLS_SSL_PROTO_TLS1_2) 02139 if( len_bytes == 2 ) 02140 { 02141 ssl->out_msg[offset+0] = (unsigned char)( *olen >> 8 ); 02142 ssl->out_msg[offset+1] = (unsigned char)( *olen ); 02143 *olen += 2; 02144 } 02145 #endif 02146 02147 return( 0 ); 02148 } 02149 #endif /* MBEDTLS_KEY_EXCHANGE_RSA_ENABLED || 02150 MBEDTLS_KEY_EXCHANGE_RSA_PSK_ENABLED */ 02151 02152 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 02153 #if defined(MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED) || \ 02154 defined(MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED) || \ 02155 defined(MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED) 02156 static int ssl_parse_signature_algorithm( mbedtls_ssl_context *ssl, 02157 unsigned char **p, 02158 unsigned char *end, 02159 mbedtls_md_type_t *md_alg, 02160 mbedtls_pk_type_t *pk_alg ) 02161 { 02162 ((void) ssl); 02163 *md_alg = MBEDTLS_MD_NONE; 02164 *pk_alg = MBEDTLS_PK_NONE; 02165 02166 /* Only in TLS 1.2 */ 02167 if( ssl->minor_ver != MBEDTLS_SSL_MINOR_VERSION_3 ) 02168 { 02169 return( 0 ); 02170 } 02171 02172 if( (*p) + 2 > end ) 02173 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_KEY_EXCHANGE ); 02174 02175 /* 02176 * Get hash algorithm 02177 */ 02178 if( ( *md_alg = mbedtls_ssl_md_alg_from_hash( (*p)[0] ) ) == MBEDTLS_MD_NONE ) 02179 { 02180 MBEDTLS_SSL_DEBUG_MSG( 1, ( "Server used unsupported " 02181 "HashAlgorithm %d", *(p)[0] ) ); 02182 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_KEY_EXCHANGE ); 02183 } 02184 02185 /* 02186 * Get signature algorithm 02187 */ 02188 if( ( *pk_alg = mbedtls_ssl_pk_alg_from_sig( (*p)[1] ) ) == MBEDTLS_PK_NONE ) 02189 { 02190 MBEDTLS_SSL_DEBUG_MSG( 1, ( "server used unsupported " 02191 "SignatureAlgorithm %d", (*p)[1] ) ); 02192 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_KEY_EXCHANGE ); 02193 } 02194 02195 /* 02196 * Check if the hash is acceptable 02197 */ 02198 if( mbedtls_ssl_check_sig_hash( ssl, *md_alg ) != 0 ) 02199 { 02200 MBEDTLS_SSL_DEBUG_MSG( 1, ( "server used HashAlgorithm %d that was not offered", 02201 *(p)[0] ) ); 02202 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_KEY_EXCHANGE ); 02203 } 02204 02205 MBEDTLS_SSL_DEBUG_MSG( 2, ( "Server used SignatureAlgorithm %d", (*p)[1] ) ); 02206 MBEDTLS_SSL_DEBUG_MSG( 2, ( "Server used HashAlgorithm %d", (*p)[0] ) ); 02207 *p += 2; 02208 02209 return( 0 ); 02210 } 02211 #endif /* MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED || 02212 MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED || 02213 MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED */ 02214 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 02215 02216 #if defined(MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED) || \ 02217 defined(MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED) 02218 static int ssl_get_ecdh_params_from_cert( mbedtls_ssl_context *ssl ) 02219 { 02220 int ret; 02221 const mbedtls_ecp_keypair *peer_key; 02222 02223 if( ssl->session_negotiate->peer_cert == NULL ) 02224 { 02225 MBEDTLS_SSL_DEBUG_MSG( 2, ( "certificate required" ) ); 02226 return( MBEDTLS_ERR_SSL_UNEXPECTED_MESSAGE ); 02227 } 02228 02229 if( ! mbedtls_pk_can_do( &ssl->session_negotiate->peer_cert->pk, 02230 MBEDTLS_PK_ECKEY ) ) 02231 { 02232 MBEDTLS_SSL_DEBUG_MSG( 1, ( "server key not ECDH capable" ) ); 02233 return( MBEDTLS_ERR_SSL_PK_TYPE_MISMATCH ); 02234 } 02235 02236 peer_key = mbedtls_pk_ec( ssl->session_negotiate->peer_cert->pk ); 02237 02238 if( ( ret = mbedtls_ecdh_get_params( &ssl->handshake->ecdh_ctx, peer_key, 02239 MBEDTLS_ECDH_THEIRS ) ) != 0 ) 02240 { 02241 MBEDTLS_SSL_DEBUG_RET( 1, ( "mbedtls_ecdh_get_params" ), ret ); 02242 return( ret ); 02243 } 02244 02245 if( ssl_check_server_ecdh_params( ssl ) != 0 ) 02246 { 02247 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server certificate (ECDH curve)" ) ); 02248 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE ); 02249 } 02250 02251 return( ret ); 02252 } 02253 #endif /* MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED) || 02254 MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED */ 02255 02256 static int ssl_parse_server_key_exchange( mbedtls_ssl_context *ssl ) 02257 { 02258 int ret; 02259 const mbedtls_ssl_ciphersuite_t *ciphersuite_info = 02260 ssl->transform_negotiate->ciphersuite_info; 02261 unsigned char *p, *end; 02262 02263 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse server key exchange" ) ); 02264 02265 #if defined(MBEDTLS_KEY_EXCHANGE_RSA_ENABLED) 02266 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA ) 02267 { 02268 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip parse server key exchange" ) ); 02269 ssl->state++; 02270 return( 0 ); 02271 } 02272 ((void) p); 02273 ((void) end); 02274 #endif 02275 02276 #if defined(MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED) || \ 02277 defined(MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED) 02278 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDH_RSA || 02279 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA ) 02280 { 02281 if( ( ret = ssl_get_ecdh_params_from_cert( ssl ) ) != 0 ) 02282 { 02283 MBEDTLS_SSL_DEBUG_RET( 1, "ssl_get_ecdh_params_from_cert", ret ); 02284 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 02285 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 02286 return( ret ); 02287 } 02288 02289 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip parse server key exchange" ) ); 02290 ssl->state++; 02291 return( 0 ); 02292 } 02293 ((void) p); 02294 ((void) end); 02295 #endif /* MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED || 02296 MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED */ 02297 02298 if( ( ret = mbedtls_ssl_read_record( ssl ) ) != 0 ) 02299 { 02300 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_read_record", ret ); 02301 return( ret ); 02302 } 02303 02304 if( ssl->in_msgtype != MBEDTLS_SSL_MSG_HANDSHAKE ) 02305 { 02306 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server key exchange message" ) ); 02307 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 02308 MBEDTLS_SSL_ALERT_MSG_UNEXPECTED_MESSAGE ); 02309 return( MBEDTLS_ERR_SSL_UNEXPECTED_MESSAGE ); 02310 } 02311 02312 /* 02313 * ServerKeyExchange may be skipped with PSK and RSA-PSK when the server 02314 * doesn't use a psk_identity_hint 02315 */ 02316 if( ssl->in_msg[0] != MBEDTLS_SSL_HS_SERVER_KEY_EXCHANGE ) 02317 { 02318 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK || 02319 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA_PSK ) 02320 { 02321 /* Current message is probably either 02322 * CertificateRequest or ServerHelloDone */ 02323 ssl->keep_current_message = 1; 02324 goto exit; 02325 } 02326 02327 MBEDTLS_SSL_DEBUG_MSG( 1, ( "server key exchange message must " 02328 "not be skipped" ) ); 02329 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 02330 MBEDTLS_SSL_ALERT_MSG_UNEXPECTED_MESSAGE ); 02331 02332 return( MBEDTLS_ERR_SSL_UNEXPECTED_MESSAGE ); 02333 } 02334 02335 p = ssl->in_msg + mbedtls_ssl_hs_hdr_len( ssl ); 02336 end = ssl->in_msg + ssl->in_hslen; 02337 MBEDTLS_SSL_DEBUG_BUF( 3, "server key exchange", p, end - p ); 02338 02339 #if defined(MBEDTLS_KEY_EXCHANGE__SOME__PSK_ENABLED) 02340 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK || 02341 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA_PSK || 02342 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK || 02343 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK ) 02344 { 02345 if( ssl_parse_server_psk_hint( ssl, &p, end ) != 0 ) 02346 { 02347 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server key exchange message" ) ); 02348 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 02349 MBEDTLS_SSL_ALERT_MSG_ILLEGAL_PARAMETER ); 02350 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_KEY_EXCHANGE ); 02351 } 02352 } /* FALLTROUGH */ 02353 #endif /* MBEDTLS_KEY_EXCHANGE__SOME__PSK_ENABLED */ 02354 02355 #if defined(MBEDTLS_KEY_EXCHANGE_PSK_ENABLED) || \ 02356 defined(MBEDTLS_KEY_EXCHANGE_RSA_PSK_ENABLED) 02357 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK || 02358 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA_PSK ) 02359 ; /* nothing more to do */ 02360 else 02361 #endif /* MBEDTLS_KEY_EXCHANGE_PSK_ENABLED || 02362 MBEDTLS_KEY_EXCHANGE_RSA_PSK_ENABLED */ 02363 #if defined(MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED) || \ 02364 defined(MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED) 02365 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_RSA || 02366 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK ) 02367 { 02368 if( ssl_parse_server_dh_params( ssl, &p, end ) != 0 ) 02369 { 02370 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server key exchange message" ) ); 02371 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 02372 MBEDTLS_SSL_ALERT_MSG_ILLEGAL_PARAMETER ); 02373 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_KEY_EXCHANGE ); 02374 } 02375 } 02376 else 02377 #endif /* MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED || 02378 MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED */ 02379 #if defined(MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED) || \ 02380 defined(MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED) || \ 02381 defined(MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED) 02382 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_RSA || 02383 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK || 02384 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA ) 02385 { 02386 if( ssl_parse_server_ecdh_params( ssl, &p, end ) != 0 ) 02387 { 02388 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server key exchange message" ) ); 02389 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 02390 MBEDTLS_SSL_ALERT_MSG_ILLEGAL_PARAMETER ); 02391 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_KEY_EXCHANGE ); 02392 } 02393 } 02394 else 02395 #endif /* MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED || 02396 MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED || 02397 MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED */ 02398 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 02399 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE ) 02400 { 02401 ret = mbedtls_ecjpake_read_round_two( &ssl->handshake->ecjpake_ctx, 02402 p, end - p ); 02403 if( ret != 0 ) 02404 { 02405 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecjpake_read_round_two", ret ); 02406 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 02407 MBEDTLS_SSL_ALERT_MSG_ILLEGAL_PARAMETER ); 02408 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_KEY_EXCHANGE ); 02409 } 02410 } 02411 else 02412 #endif /* MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 02413 { 02414 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 02415 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 02416 } 02417 02418 #if defined(MBEDTLS_KEY_EXCHANGE__WITH_SERVER_SIGNATURE__ENABLED) 02419 if( mbedtls_ssl_ciphersuite_uses_server_signature( ciphersuite_info ) ) 02420 { 02421 size_t sig_len, hashlen; 02422 unsigned char hash[64]; 02423 mbedtls_md_type_t md_alg = MBEDTLS_MD_NONE; 02424 mbedtls_pk_type_t pk_alg = MBEDTLS_PK_NONE; 02425 unsigned char *params = ssl->in_msg + mbedtls_ssl_hs_hdr_len( ssl ); 02426 size_t params_len = p - params; 02427 02428 /* 02429 * Handle the digitally-signed structure 02430 */ 02431 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 02432 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_3 ) 02433 { 02434 if( ssl_parse_signature_algorithm( ssl, &p, end, 02435 &md_alg, &pk_alg ) != 0 ) 02436 { 02437 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server key exchange message" ) ); 02438 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 02439 MBEDTLS_SSL_ALERT_MSG_ILLEGAL_PARAMETER ); 02440 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_KEY_EXCHANGE ); 02441 } 02442 02443 if( pk_alg != mbedtls_ssl_get_ciphersuite_sig_pk_alg( ciphersuite_info ) ) 02444 { 02445 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server key exchange message" ) ); 02446 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 02447 MBEDTLS_SSL_ALERT_MSG_ILLEGAL_PARAMETER ); 02448 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_KEY_EXCHANGE ); 02449 } 02450 } 02451 else 02452 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 02453 #if defined(MBEDTLS_SSL_PROTO_SSL3) || defined(MBEDTLS_SSL_PROTO_TLS1) || \ 02454 defined(MBEDTLS_SSL_PROTO_TLS1_1) 02455 if( ssl->minor_ver < MBEDTLS_SSL_MINOR_VERSION_3 ) 02456 { 02457 pk_alg = mbedtls_ssl_get_ciphersuite_sig_pk_alg( ciphersuite_info ); 02458 02459 /* Default hash for ECDSA is SHA-1 */ 02460 if( pk_alg == MBEDTLS_PK_ECDSA && md_alg == MBEDTLS_MD_NONE ) 02461 md_alg = MBEDTLS_MD_SHA1; 02462 } 02463 else 02464 #endif 02465 { 02466 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 02467 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 02468 } 02469 02470 /* 02471 * Read signature 02472 */ 02473 sig_len = ( p[0] << 8 ) | p[1]; 02474 p += 2; 02475 02476 if( end != p + sig_len ) 02477 { 02478 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server key exchange message" ) ); 02479 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 02480 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 02481 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_KEY_EXCHANGE ); 02482 } 02483 02484 MBEDTLS_SSL_DEBUG_BUF( 3, "signature", p, sig_len ); 02485 02486 /* 02487 * Compute the hash that has been signed 02488 */ 02489 #if defined(MBEDTLS_SSL_PROTO_SSL3) || defined(MBEDTLS_SSL_PROTO_TLS1) || \ 02490 defined(MBEDTLS_SSL_PROTO_TLS1_1) 02491 if( md_alg == MBEDTLS_MD_NONE ) 02492 { 02493 mbedtls_md5_context mbedtls_md5; 02494 mbedtls_sha1_context mbedtls_sha1; 02495 02496 mbedtls_md5_init( &mbedtls_md5 ); 02497 mbedtls_sha1_init( &mbedtls_sha1 ); 02498 02499 hashlen = 36; 02500 02501 /* 02502 * digitally-signed struct { 02503 * opaque md5_hash[16]; 02504 * opaque sha_hash[20]; 02505 * }; 02506 * 02507 * md5_hash 02508 * MD5(ClientHello.random + ServerHello.random 02509 * + ServerParams); 02510 * sha_hash 02511 * SHA(ClientHello.random + ServerHello.random 02512 * + ServerParams); 02513 */ 02514 mbedtls_md5_starts( &mbedtls_md5 ); 02515 mbedtls_md5_update( &mbedtls_md5, ssl->handshake->randbytes, 64 ); 02516 mbedtls_md5_update( &mbedtls_md5, params, params_len ); 02517 mbedtls_md5_finish( &mbedtls_md5, hash ); 02518 02519 mbedtls_sha1_starts( &mbedtls_sha1 ); 02520 mbedtls_sha1_update( &mbedtls_sha1, ssl->handshake->randbytes, 64 ); 02521 mbedtls_sha1_update( &mbedtls_sha1, params, params_len ); 02522 mbedtls_sha1_finish( &mbedtls_sha1, hash + 16 ); 02523 02524 mbedtls_md5_free( &mbedtls_md5 ); 02525 mbedtls_sha1_free( &mbedtls_sha1 ); 02526 } 02527 else 02528 #endif /* MBEDTLS_SSL_PROTO_SSL3 || MBEDTLS_SSL_PROTO_TLS1 || \ 02529 MBEDTLS_SSL_PROTO_TLS1_1 */ 02530 #if defined(MBEDTLS_SSL_PROTO_TLS1) || defined(MBEDTLS_SSL_PROTO_TLS1_1) || \ 02531 defined(MBEDTLS_SSL_PROTO_TLS1_2) 02532 if( md_alg != MBEDTLS_MD_NONE ) 02533 { 02534 mbedtls_md_context_t ctx; 02535 02536 mbedtls_md_init( &ctx ); 02537 02538 /* Info from md_alg will be used instead */ 02539 hashlen = 0; 02540 02541 /* 02542 * digitally-signed struct { 02543 * opaque client_random[32]; 02544 * opaque server_random[32]; 02545 * ServerDHParams params; 02546 * }; 02547 */ 02548 if( ( ret = mbedtls_md_setup( &ctx, 02549 mbedtls_md_info_from_type( md_alg ), 0 ) ) != 0 ) 02550 { 02551 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_md_setup", ret ); 02552 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 02553 MBEDTLS_SSL_ALERT_MSG_INTERNAL_ERROR ); 02554 return( ret ); 02555 } 02556 02557 mbedtls_md_starts( &ctx ); 02558 mbedtls_md_update( &ctx, ssl->handshake->randbytes, 64 ); 02559 mbedtls_md_update( &ctx, params, params_len ); 02560 mbedtls_md_finish( &ctx, hash ); 02561 mbedtls_md_free( &ctx ); 02562 } 02563 else 02564 #endif /* MBEDTLS_SSL_PROTO_TLS1 || MBEDTLS_SSL_PROTO_TLS1_1 || \ 02565 MBEDTLS_SSL_PROTO_TLS1_2 */ 02566 { 02567 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 02568 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 02569 } 02570 02571 MBEDTLS_SSL_DEBUG_BUF( 3, "parameters hash", hash, hashlen != 0 ? hashlen : 02572 (unsigned int) ( mbedtls_md_get_size( mbedtls_md_info_from_type( md_alg ) ) ) ); 02573 02574 if( ssl->session_negotiate->peer_cert == NULL ) 02575 { 02576 MBEDTLS_SSL_DEBUG_MSG( 2, ( "certificate required" ) ); 02577 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 02578 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 02579 return( MBEDTLS_ERR_SSL_UNEXPECTED_MESSAGE ); 02580 } 02581 02582 /* 02583 * Verify signature 02584 */ 02585 if( ! mbedtls_pk_can_do( &ssl->session_negotiate->peer_cert->pk, pk_alg ) ) 02586 { 02587 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server key exchange message" ) ); 02588 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 02589 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 02590 return( MBEDTLS_ERR_SSL_PK_TYPE_MISMATCH ); 02591 } 02592 02593 if( ( ret = mbedtls_pk_verify( &ssl->session_negotiate->peer_cert->pk, 02594 md_alg, hash, hashlen, p, sig_len ) ) != 0 ) 02595 { 02596 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 02597 MBEDTLS_SSL_ALERT_MSG_DECRYPT_ERROR ); 02598 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_pk_verify", ret ); 02599 return( ret ); 02600 } 02601 } 02602 #endif /* MBEDTLS_KEY_EXCHANGE__WITH_SERVER_SIGNATURE__ENABLED */ 02603 02604 exit: 02605 ssl->state++; 02606 02607 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= parse server key exchange" ) ); 02608 02609 return( 0 ); 02610 } 02611 02612 #if ! defined(MBEDTLS_KEY_EXCHANGE__CERT_REQ_ALLOWED__ENABLED) 02613 static int ssl_parse_certificate_request( mbedtls_ssl_context *ssl ) 02614 { 02615 const mbedtls_ssl_ciphersuite_t *ciphersuite_info = 02616 ssl->transform_negotiate->ciphersuite_info; 02617 02618 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse certificate request" ) ); 02619 02620 if( ! mbedtls_ssl_ciphersuite_cert_req_allowed( ciphersuite_info ) ) 02621 { 02622 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip parse certificate request" ) ); 02623 ssl->state++; 02624 return( 0 ); 02625 } 02626 02627 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 02628 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 02629 } 02630 #else /* MBEDTLS_KEY_EXCHANGE__CERT_REQ_ALLOWED__ENABLED */ 02631 static int ssl_parse_certificate_request( mbedtls_ssl_context *ssl ) 02632 { 02633 int ret; 02634 unsigned char *buf; 02635 size_t n = 0; 02636 size_t cert_type_len = 0, dn_len = 0; 02637 const mbedtls_ssl_ciphersuite_t *ciphersuite_info = 02638 ssl->transform_negotiate->ciphersuite_info; 02639 02640 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse certificate request" ) ); 02641 02642 if( ! mbedtls_ssl_ciphersuite_cert_req_allowed( ciphersuite_info ) ) 02643 { 02644 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip parse certificate request" ) ); 02645 ssl->state++; 02646 return( 0 ); 02647 } 02648 02649 if( ( ret = mbedtls_ssl_read_record( ssl ) ) != 0 ) 02650 { 02651 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_read_record", ret ); 02652 return( ret ); 02653 } 02654 02655 if( ssl->in_msgtype != MBEDTLS_SSL_MSG_HANDSHAKE ) 02656 { 02657 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad certificate request message" ) ); 02658 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 02659 MBEDTLS_SSL_ALERT_MSG_UNEXPECTED_MESSAGE ); 02660 return( MBEDTLS_ERR_SSL_UNEXPECTED_MESSAGE ); 02661 } 02662 02663 ssl->state++; 02664 ssl->client_auth = ( ssl->in_msg[0] == MBEDTLS_SSL_HS_CERTIFICATE_REQUEST ); 02665 02666 MBEDTLS_SSL_DEBUG_MSG( 3, ( "got %s certificate request", 02667 ssl->client_auth ? "a" : "no" ) ); 02668 02669 if( ssl->client_auth == 0 ) 02670 { 02671 /* Current message is probably the ServerHelloDone */ 02672 ssl->keep_current_message = 1; 02673 goto exit; 02674 } 02675 02676 /* 02677 * struct { 02678 * ClientCertificateType certificate_types<1..2^8-1>; 02679 * SignatureAndHashAlgorithm 02680 * supported_signature_algorithms<2^16-1>; -- TLS 1.2 only 02681 * DistinguishedName certificate_authorities<0..2^16-1>; 02682 * } CertificateRequest; 02683 * 02684 * Since we only support a single certificate on clients, let's just 02685 * ignore all the information that's supposed to help us pick a 02686 * certificate. 02687 * 02688 * We could check that our certificate matches the request, and bail out 02689 * if it doesn't, but it's simpler to just send the certificate anyway, 02690 * and give the server the opportunity to decide if it should terminate 02691 * the connection when it doesn't like our certificate. 02692 * 02693 * Same goes for the hash in TLS 1.2's signature_algorithms: at this 02694 * point we only have one hash available (see comments in 02695 * write_certificate_verify), so let's just use what we have. 02696 * 02697 * However, we still minimally parse the message to check it is at least 02698 * superficially sane. 02699 */ 02700 buf = ssl->in_msg; 02701 02702 /* certificate_types */ 02703 cert_type_len = buf[mbedtls_ssl_hs_hdr_len( ssl )]; 02704 n = cert_type_len; 02705 02706 if( ssl->in_hslen < mbedtls_ssl_hs_hdr_len( ssl ) + 2 + n ) 02707 { 02708 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad certificate request message" ) ); 02709 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 02710 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 02711 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE_REQUEST ); 02712 } 02713 02714 /* supported_signature_algorithms */ 02715 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 02716 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_3 ) 02717 { 02718 size_t sig_alg_len = ( ( buf[mbedtls_ssl_hs_hdr_len( ssl ) + 1 + n] << 8 ) 02719 | ( buf[mbedtls_ssl_hs_hdr_len( ssl ) + 2 + n] ) ); 02720 #if defined(MBEDTLS_DEBUG_C) 02721 unsigned char* sig_alg = buf + mbedtls_ssl_hs_hdr_len( ssl ) + 3 + n; 02722 size_t i; 02723 02724 for( i = 0; i < sig_alg_len; i += 2 ) 02725 { 02726 MBEDTLS_SSL_DEBUG_MSG( 3, ( "Supported Signature Algorithm found: %d" 02727 ",%d", sig_alg[i], sig_alg[i + 1] ) ); 02728 } 02729 #endif 02730 02731 n += 2 + sig_alg_len; 02732 02733 if( ssl->in_hslen < mbedtls_ssl_hs_hdr_len( ssl ) + 2 + n ) 02734 { 02735 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad certificate request message" ) ); 02736 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 02737 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 02738 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE_REQUEST ); 02739 } 02740 } 02741 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 02742 02743 /* certificate_authorities */ 02744 dn_len = ( ( buf[mbedtls_ssl_hs_hdr_len( ssl ) + 1 + n] << 8 ) 02745 | ( buf[mbedtls_ssl_hs_hdr_len( ssl ) + 2 + n] ) ); 02746 02747 n += dn_len; 02748 if( ssl->in_hslen != mbedtls_ssl_hs_hdr_len( ssl ) + 3 + n ) 02749 { 02750 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad certificate request message" ) ); 02751 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 02752 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 02753 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE_REQUEST ); 02754 } 02755 02756 exit: 02757 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= parse certificate request" ) ); 02758 02759 return( 0 ); 02760 } 02761 #endif /* MBEDTLS_KEY_EXCHANGE__CERT_REQ_ALLOWED__ENABLED */ 02762 02763 static int ssl_parse_server_hello_done( mbedtls_ssl_context *ssl ) 02764 { 02765 int ret; 02766 02767 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse server hello done" ) ); 02768 02769 if( ( ret = mbedtls_ssl_read_record( ssl ) ) != 0 ) 02770 { 02771 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_read_record", ret ); 02772 return( ret ); 02773 } 02774 02775 if( ssl->in_msgtype != MBEDTLS_SSL_MSG_HANDSHAKE ) 02776 { 02777 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server hello done message" ) ); 02778 return( MBEDTLS_ERR_SSL_UNEXPECTED_MESSAGE ); 02779 } 02780 02781 if( ssl->in_hslen != mbedtls_ssl_hs_hdr_len( ssl ) || 02782 ssl->in_msg[0] != MBEDTLS_SSL_HS_SERVER_HELLO_DONE ) 02783 { 02784 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad server hello done message" ) ); 02785 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 02786 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 02787 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_HELLO_DONE ); 02788 } 02789 02790 ssl->state++; 02791 02792 #if defined(MBEDTLS_SSL_PROTO_DTLS) 02793 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 02794 mbedtls_ssl_recv_flight_completed( ssl ); 02795 #endif 02796 02797 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= parse server hello done" ) ); 02798 02799 return( 0 ); 02800 } 02801 02802 static int ssl_write_client_key_exchange( mbedtls_ssl_context *ssl ) 02803 { 02804 int ret; 02805 size_t i, n; 02806 const mbedtls_ssl_ciphersuite_t *ciphersuite_info = 02807 ssl->transform_negotiate->ciphersuite_info; 02808 02809 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write client key exchange" ) ); 02810 02811 #if defined(MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED) 02812 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_RSA ) 02813 { 02814 /* 02815 * DHM key exchange -- send G^X mod P 02816 */ 02817 n = ssl->handshake->dhm_ctx.len; 02818 02819 ssl->out_msg[4] = (unsigned char)( n >> 8 ); 02820 ssl->out_msg[5] = (unsigned char)( n ); 02821 i = 6; 02822 02823 ret = mbedtls_dhm_make_public( &ssl->handshake->dhm_ctx, 02824 (int) mbedtls_mpi_size( &ssl->handshake->dhm_ctx.P ), 02825 &ssl->out_msg[i], n, 02826 ssl->conf->f_rng, ssl->conf->p_rng ); 02827 if( ret != 0 ) 02828 { 02829 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_dhm_make_public", ret ); 02830 return( ret ); 02831 } 02832 02833 MBEDTLS_SSL_DEBUG_MPI( 3, "DHM: X ", &ssl->handshake->dhm_ctx.X ); 02834 MBEDTLS_SSL_DEBUG_MPI( 3, "DHM: GX", &ssl->handshake->dhm_ctx.GX ); 02835 02836 if( ( ret = mbedtls_dhm_calc_secret( &ssl->handshake->dhm_ctx, 02837 ssl->handshake->premaster, 02838 MBEDTLS_PREMASTER_SIZE, 02839 &ssl->handshake->pmslen, 02840 ssl->conf->f_rng, ssl->conf->p_rng ) ) != 0 ) 02841 { 02842 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_dhm_calc_secret", ret ); 02843 return( ret ); 02844 } 02845 02846 MBEDTLS_SSL_DEBUG_MPI( 3, "DHM: K ", &ssl->handshake->dhm_ctx.K ); 02847 } 02848 else 02849 #endif /* MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED */ 02850 #if defined(MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED) || \ 02851 defined(MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED) || \ 02852 defined(MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED) || \ 02853 defined(MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED) 02854 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_RSA || 02855 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA || 02856 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDH_RSA || 02857 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA ) 02858 { 02859 /* 02860 * ECDH key exchange -- send client public value 02861 */ 02862 i = 4; 02863 02864 ret = mbedtls_ecdh_make_public( &ssl->handshake->ecdh_ctx, 02865 &n, 02866 &ssl->out_msg[i], 1000, 02867 ssl->conf->f_rng, ssl->conf->p_rng ); 02868 if( ret != 0 ) 02869 { 02870 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecdh_make_public", ret ); 02871 return( ret ); 02872 } 02873 02874 MBEDTLS_SSL_DEBUG_ECP( 3, "ECDH: Q", &ssl->handshake->ecdh_ctx.Q ); 02875 02876 if( ( ret = mbedtls_ecdh_calc_secret( &ssl->handshake->ecdh_ctx, 02877 &ssl->handshake->pmslen, 02878 ssl->handshake->premaster, 02879 MBEDTLS_MPI_MAX_SIZE, 02880 ssl->conf->f_rng, ssl->conf->p_rng ) ) != 0 ) 02881 { 02882 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecdh_calc_secret", ret ); 02883 return( ret ); 02884 } 02885 02886 MBEDTLS_SSL_DEBUG_MPI( 3, "ECDH: z", &ssl->handshake->ecdh_ctx.z ); 02887 } 02888 else 02889 #endif /* MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED || 02890 MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED || 02891 MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED || 02892 MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED */ 02893 #if defined(MBEDTLS_KEY_EXCHANGE__SOME__PSK_ENABLED) 02894 if( mbedtls_ssl_ciphersuite_uses_psk( ciphersuite_info ) ) 02895 { 02896 /* 02897 * opaque psk_identity<0..2^16-1>; 02898 */ 02899 if( ssl->conf->psk == NULL || ssl->conf->psk_identity == NULL ) 02900 { 02901 MBEDTLS_SSL_DEBUG_MSG( 1, ( "got no private key for PSK" ) ); 02902 return( MBEDTLS_ERR_SSL_PRIVATE_KEY_REQUIRED ); 02903 } 02904 02905 i = 4; 02906 n = ssl->conf->psk_identity_len; 02907 02908 if( i + 2 + n > MBEDTLS_SSL_MAX_CONTENT_LEN ) 02909 { 02910 MBEDTLS_SSL_DEBUG_MSG( 1, ( "psk identity too long or " 02911 "SSL buffer too short" ) ); 02912 return( MBEDTLS_ERR_SSL_BUFFER_TOO_SMALL ); 02913 } 02914 02915 ssl->out_msg[i++] = (unsigned char)( n >> 8 ); 02916 ssl->out_msg[i++] = (unsigned char)( n ); 02917 02918 memcpy( ssl->out_msg + i, ssl->conf->psk_identity, ssl->conf->psk_identity_len ); 02919 i += ssl->conf->psk_identity_len; 02920 02921 #if defined(MBEDTLS_KEY_EXCHANGE_PSK_ENABLED) 02922 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK ) 02923 { 02924 n = 0; 02925 } 02926 else 02927 #endif 02928 #if defined(MBEDTLS_KEY_EXCHANGE_RSA_PSK_ENABLED) 02929 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA_PSK ) 02930 { 02931 if( ( ret = ssl_write_encrypted_pms( ssl, i, &n, 2 ) ) != 0 ) 02932 return( ret ); 02933 } 02934 else 02935 #endif 02936 #if defined(MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED) 02937 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK ) 02938 { 02939 /* 02940 * ClientDiffieHellmanPublic public (DHM send G^X mod P) 02941 */ 02942 n = ssl->handshake->dhm_ctx.len; 02943 02944 if( i + 2 + n > MBEDTLS_SSL_MAX_CONTENT_LEN ) 02945 { 02946 MBEDTLS_SSL_DEBUG_MSG( 1, ( "psk identity or DHM size too long" 02947 " or SSL buffer too short" ) ); 02948 return( MBEDTLS_ERR_SSL_BUFFER_TOO_SMALL ); 02949 } 02950 02951 ssl->out_msg[i++] = (unsigned char)( n >> 8 ); 02952 ssl->out_msg[i++] = (unsigned char)( n ); 02953 02954 ret = mbedtls_dhm_make_public( &ssl->handshake->dhm_ctx, 02955 (int) mbedtls_mpi_size( &ssl->handshake->dhm_ctx.P ), 02956 &ssl->out_msg[i], n, 02957 ssl->conf->f_rng, ssl->conf->p_rng ); 02958 if( ret != 0 ) 02959 { 02960 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_dhm_make_public", ret ); 02961 return( ret ); 02962 } 02963 } 02964 else 02965 #endif /* MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED */ 02966 #if defined(MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED) 02967 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK ) 02968 { 02969 /* 02970 * ClientECDiffieHellmanPublic public; 02971 */ 02972 ret = mbedtls_ecdh_make_public( &ssl->handshake->ecdh_ctx, &n, 02973 &ssl->out_msg[i], MBEDTLS_SSL_MAX_CONTENT_LEN - i, 02974 ssl->conf->f_rng, ssl->conf->p_rng ); 02975 if( ret != 0 ) 02976 { 02977 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecdh_make_public", ret ); 02978 return( ret ); 02979 } 02980 02981 MBEDTLS_SSL_DEBUG_ECP( 3, "ECDH: Q", &ssl->handshake->ecdh_ctx.Q ); 02982 } 02983 else 02984 #endif /* MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED */ 02985 { 02986 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 02987 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 02988 } 02989 02990 if( ( ret = mbedtls_ssl_psk_derive_premaster( ssl, 02991 ciphersuite_info->key_exchange ) ) != 0 ) 02992 { 02993 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_psk_derive_premaster", ret ); 02994 return( ret ); 02995 } 02996 } 02997 else 02998 #endif /* MBEDTLS_KEY_EXCHANGE__SOME__PSK_ENABLED */ 02999 #if defined(MBEDTLS_KEY_EXCHANGE_RSA_ENABLED) 03000 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA ) 03001 { 03002 i = 4; 03003 if( ( ret = ssl_write_encrypted_pms( ssl, i, &n, 0 ) ) != 0 ) 03004 return( ret ); 03005 } 03006 else 03007 #endif /* MBEDTLS_KEY_EXCHANGE_RSA_ENABLED */ 03008 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 03009 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE ) 03010 { 03011 i = 4; 03012 03013 ret = mbedtls_ecjpake_write_round_two( &ssl->handshake->ecjpake_ctx, 03014 ssl->out_msg + i, MBEDTLS_SSL_MAX_CONTENT_LEN - i, &n, 03015 ssl->conf->f_rng, ssl->conf->p_rng ); 03016 if( ret != 0 ) 03017 { 03018 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecjpake_write_round_two", ret ); 03019 return( ret ); 03020 } 03021 03022 ret = mbedtls_ecjpake_derive_secret( &ssl->handshake->ecjpake_ctx, 03023 ssl->handshake->premaster, 32, &ssl->handshake->pmslen, 03024 ssl->conf->f_rng, ssl->conf->p_rng ); 03025 if( ret != 0 ) 03026 { 03027 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecjpake_derive_secret", ret ); 03028 return( ret ); 03029 } 03030 } 03031 else 03032 #endif /* MBEDTLS_KEY_EXCHANGE_RSA_ENABLED */ 03033 { 03034 ((void) ciphersuite_info); 03035 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 03036 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 03037 } 03038 03039 ssl->out_msglen = i + n; 03040 ssl->out_msgtype = MBEDTLS_SSL_MSG_HANDSHAKE; 03041 ssl->out_msg[0] = MBEDTLS_SSL_HS_CLIENT_KEY_EXCHANGE; 03042 03043 ssl->state++; 03044 03045 if( ( ret = mbedtls_ssl_write_record( ssl ) ) != 0 ) 03046 { 03047 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_write_record", ret ); 03048 return( ret ); 03049 } 03050 03051 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write client key exchange" ) ); 03052 03053 return( 0 ); 03054 } 03055 03056 #if !defined(MBEDTLS_KEY_EXCHANGE_RSA_ENABLED) && \ 03057 !defined(MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED) && \ 03058 !defined(MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED) && \ 03059 !defined(MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED) && \ 03060 !defined(MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED)&& \ 03061 !defined(MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED) 03062 static int ssl_write_certificate_verify( mbedtls_ssl_context *ssl ) 03063 { 03064 const mbedtls_ssl_ciphersuite_t *ciphersuite_info = 03065 ssl->transform_negotiate->ciphersuite_info; 03066 int ret; 03067 03068 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write certificate verify" ) ); 03069 03070 if( ( ret = mbedtls_ssl_derive_keys( ssl ) ) != 0 ) 03071 { 03072 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_derive_keys", ret ); 03073 return( ret ); 03074 } 03075 03076 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK || 03077 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA_PSK || 03078 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK || 03079 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK || 03080 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE ) 03081 { 03082 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip write certificate verify" ) ); 03083 ssl->state++; 03084 return( 0 ); 03085 } 03086 03087 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 03088 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 03089 } 03090 #else 03091 static int ssl_write_certificate_verify( mbedtls_ssl_context *ssl ) 03092 { 03093 int ret = MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE; 03094 const mbedtls_ssl_ciphersuite_t *ciphersuite_info = 03095 ssl->transform_negotiate->ciphersuite_info; 03096 size_t n = 0, offset = 0; 03097 unsigned char hash[48]; 03098 unsigned char *hash_start = hash; 03099 mbedtls_md_type_t md_alg = MBEDTLS_MD_NONE; 03100 unsigned int hashlen; 03101 03102 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write certificate verify" ) ); 03103 03104 if( ( ret = mbedtls_ssl_derive_keys( ssl ) ) != 0 ) 03105 { 03106 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_derive_keys", ret ); 03107 return( ret ); 03108 } 03109 03110 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK || 03111 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA_PSK || 03112 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK || 03113 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK || 03114 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE ) 03115 { 03116 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip write certificate verify" ) ); 03117 ssl->state++; 03118 return( 0 ); 03119 } 03120 03121 if( ssl->client_auth == 0 || mbedtls_ssl_own_cert( ssl ) == NULL ) 03122 { 03123 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip write certificate verify" ) ); 03124 ssl->state++; 03125 return( 0 ); 03126 } 03127 03128 if( mbedtls_ssl_own_key( ssl ) == NULL ) 03129 { 03130 MBEDTLS_SSL_DEBUG_MSG( 1, ( "got no private key for certificate" ) ); 03131 return( MBEDTLS_ERR_SSL_PRIVATE_KEY_REQUIRED ); 03132 } 03133 03134 /* 03135 * Make an RSA signature of the handshake digests 03136 */ 03137 ssl->handshake->calc_verify( ssl, hash ); 03138 03139 #if defined(MBEDTLS_SSL_PROTO_SSL3) || defined(MBEDTLS_SSL_PROTO_TLS1) || \ 03140 defined(MBEDTLS_SSL_PROTO_TLS1_1) 03141 if( ssl->minor_ver != MBEDTLS_SSL_MINOR_VERSION_3 ) 03142 { 03143 /* 03144 * digitally-signed struct { 03145 * opaque md5_hash[16]; 03146 * opaque sha_hash[20]; 03147 * }; 03148 * 03149 * md5_hash 03150 * MD5(handshake_messages); 03151 * 03152 * sha_hash 03153 * SHA(handshake_messages); 03154 */ 03155 hashlen = 36; 03156 md_alg = MBEDTLS_MD_NONE; 03157 03158 /* 03159 * For ECDSA, default hash is SHA-1 only 03160 */ 03161 if( mbedtls_pk_can_do( mbedtls_ssl_own_key( ssl ), MBEDTLS_PK_ECDSA ) ) 03162 { 03163 hash_start += 16; 03164 hashlen -= 16; 03165 md_alg = MBEDTLS_MD_SHA1; 03166 } 03167 } 03168 else 03169 #endif /* MBEDTLS_SSL_PROTO_SSL3 || MBEDTLS_SSL_PROTO_TLS1 || \ 03170 MBEDTLS_SSL_PROTO_TLS1_1 */ 03171 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 03172 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_3 ) 03173 { 03174 /* 03175 * digitally-signed struct { 03176 * opaque handshake_messages[handshake_messages_length]; 03177 * }; 03178 * 03179 * Taking shortcut here. We assume that the server always allows the 03180 * PRF Hash function and has sent it in the allowed signature 03181 * algorithms list received in the Certificate Request message. 03182 * 03183 * Until we encounter a server that does not, we will take this 03184 * shortcut. 03185 * 03186 * Reason: Otherwise we should have running hashes for SHA512 and SHA224 03187 * in order to satisfy 'weird' needs from the server side. 03188 */ 03189 if( ssl->transform_negotiate->ciphersuite_info->mac == 03190 MBEDTLS_MD_SHA384 ) 03191 { 03192 md_alg = MBEDTLS_MD_SHA384; 03193 ssl->out_msg[4] = MBEDTLS_SSL_HASH_SHA384; 03194 } 03195 else 03196 { 03197 md_alg = MBEDTLS_MD_SHA256; 03198 ssl->out_msg[4] = MBEDTLS_SSL_HASH_SHA256; 03199 } 03200 ssl->out_msg[5] = mbedtls_ssl_sig_from_pk( mbedtls_ssl_own_key( ssl ) ); 03201 03202 /* Info from md_alg will be used instead */ 03203 hashlen = 0; 03204 offset = 2; 03205 } 03206 else 03207 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 03208 { 03209 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 03210 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 03211 } 03212 03213 if( ( ret = mbedtls_pk_sign( mbedtls_ssl_own_key( ssl ), md_alg, hash_start, hashlen, 03214 ssl->out_msg + 6 + offset, &n, 03215 ssl->conf->f_rng, ssl->conf->p_rng ) ) != 0 ) 03216 { 03217 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_pk_sign", ret ); 03218 return( ret ); 03219 } 03220 03221 ssl->out_msg[4 + offset] = (unsigned char)( n >> 8 ); 03222 ssl->out_msg[5 + offset] = (unsigned char)( n ); 03223 03224 ssl->out_msglen = 6 + n + offset; 03225 ssl->out_msgtype = MBEDTLS_SSL_MSG_HANDSHAKE; 03226 ssl->out_msg[0] = MBEDTLS_SSL_HS_CERTIFICATE_VERIFY; 03227 03228 ssl->state++; 03229 03230 if( ( ret = mbedtls_ssl_write_record( ssl ) ) != 0 ) 03231 { 03232 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_write_record", ret ); 03233 return( ret ); 03234 } 03235 03236 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write certificate verify" ) ); 03237 03238 return( ret ); 03239 } 03240 #endif /* !MBEDTLS_KEY_EXCHANGE_RSA_ENABLED && 03241 !MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED && 03242 !MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED && 03243 !MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED && 03244 !MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED && 03245 !MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED */ 03246 03247 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 03248 static int ssl_parse_new_session_ticket( mbedtls_ssl_context *ssl ) 03249 { 03250 int ret; 03251 uint32_t lifetime; 03252 size_t ticket_len; 03253 unsigned char *ticket; 03254 const unsigned char *msg; 03255 03256 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse new session ticket" ) ); 03257 03258 if( ( ret = mbedtls_ssl_read_record( ssl ) ) != 0 ) 03259 { 03260 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_read_record", ret ); 03261 return( ret ); 03262 } 03263 03264 if( ssl->in_msgtype != MBEDTLS_SSL_MSG_HANDSHAKE ) 03265 { 03266 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad new session ticket message" ) ); 03267 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 03268 MBEDTLS_SSL_ALERT_MSG_UNEXPECTED_MESSAGE ); 03269 return( MBEDTLS_ERR_SSL_UNEXPECTED_MESSAGE ); 03270 } 03271 03272 /* 03273 * struct { 03274 * uint32 ticket_lifetime_hint; 03275 * opaque ticket<0..2^16-1>; 03276 * } NewSessionTicket; 03277 * 03278 * 0 . 3 ticket_lifetime_hint 03279 * 4 . 5 ticket_len (n) 03280 * 6 . 5+n ticket content 03281 */ 03282 if( ssl->in_msg[0] != MBEDTLS_SSL_HS_NEW_SESSION_TICKET || 03283 ssl->in_hslen < 6 + mbedtls_ssl_hs_hdr_len( ssl ) ) 03284 { 03285 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad new session ticket message" ) ); 03286 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 03287 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 03288 return( MBEDTLS_ERR_SSL_BAD_HS_NEW_SESSION_TICKET ); 03289 } 03290 03291 msg = ssl->in_msg + mbedtls_ssl_hs_hdr_len( ssl ); 03292 03293 lifetime = ( msg[0] << 24 ) | ( msg[1] << 16 ) | 03294 ( msg[2] << 8 ) | ( msg[3] ); 03295 03296 ticket_len = ( msg[4] << 8 ) | ( msg[5] ); 03297 03298 if( ticket_len + 6 + mbedtls_ssl_hs_hdr_len( ssl ) != ssl->in_hslen ) 03299 { 03300 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad new session ticket message" ) ); 03301 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 03302 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 03303 return( MBEDTLS_ERR_SSL_BAD_HS_NEW_SESSION_TICKET ); 03304 } 03305 03306 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ticket length: %d", ticket_len ) ); 03307 03308 /* We're not waiting for a NewSessionTicket message any more */ 03309 ssl->handshake->new_session_ticket = 0; 03310 ssl->state = MBEDTLS_SSL_SERVER_CHANGE_CIPHER_SPEC; 03311 03312 /* 03313 * Zero-length ticket means the server changed his mind and doesn't want 03314 * to send a ticket after all, so just forget it 03315 */ 03316 if( ticket_len == 0 ) 03317 return( 0 ); 03318 03319 mbedtls_zeroize( ssl->session_negotiate->ticket, 03320 ssl->session_negotiate->ticket_len ); 03321 mbedtls_free( ssl->session_negotiate->ticket ); 03322 ssl->session_negotiate->ticket = NULL; 03323 ssl->session_negotiate->ticket_len = 0; 03324 03325 if( ( ticket = mbedtls_calloc( 1, ticket_len ) ) == NULL ) 03326 { 03327 MBEDTLS_SSL_DEBUG_MSG( 1, ( "ticket alloc failed" ) ); 03328 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 03329 MBEDTLS_SSL_ALERT_MSG_INTERNAL_ERROR ); 03330 return( MBEDTLS_ERR_SSL_ALLOC_FAILED ); 03331 } 03332 03333 memcpy( ticket, msg + 6, ticket_len ); 03334 03335 ssl->session_negotiate->ticket = ticket; 03336 ssl->session_negotiate->ticket_len = ticket_len; 03337 ssl->session_negotiate->ticket_lifetime = lifetime; 03338 03339 /* 03340 * RFC 5077 section 3.4: 03341 * "If the client receives a session ticket from the server, then it 03342 * discards any Session ID that was sent in the ServerHello." 03343 */ 03344 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ticket in use, discarding session id" ) ); 03345 ssl->session_negotiate->id_len = 0; 03346 03347 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= parse new session ticket" ) ); 03348 03349 return( 0 ); 03350 } 03351 #endif /* MBEDTLS_SSL_SESSION_TICKETS */ 03352 03353 /* 03354 * SSL handshake -- client side -- single step 03355 */ 03356 int mbedtls_ssl_handshake_client_step( mbedtls_ssl_context *ssl ) 03357 { 03358 int ret = 0; 03359 03360 if( ssl->state == MBEDTLS_SSL_HANDSHAKE_OVER || ssl->handshake == NULL ) 03361 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 03362 03363 MBEDTLS_SSL_DEBUG_MSG( 2, ( "client state: %d", ssl->state ) ); 03364 03365 if( ( ret = mbedtls_ssl_flush_output( ssl ) ) != 0 ) 03366 return( ret ); 03367 03368 #if defined(MBEDTLS_SSL_PROTO_DTLS) 03369 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM && 03370 ssl->handshake->retransmit_state == MBEDTLS_SSL_RETRANS_SENDING ) 03371 { 03372 if( ( ret = mbedtls_ssl_resend( ssl ) ) != 0 ) 03373 return( ret ); 03374 } 03375 #endif 03376 03377 /* Change state now, so that it is right in mbedtls_ssl_read_record(), used 03378 * by DTLS for dropping out-of-sequence ChangeCipherSpec records */ 03379 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 03380 if( ssl->state == MBEDTLS_SSL_SERVER_CHANGE_CIPHER_SPEC && 03381 ssl->handshake->new_session_ticket != 0 ) 03382 { 03383 ssl->state = MBEDTLS_SSL_SERVER_NEW_SESSION_TICKET; 03384 } 03385 #endif 03386 03387 switch( ssl->state ) 03388 { 03389 case MBEDTLS_SSL_HELLO_REQUEST: 03390 ssl->state = MBEDTLS_SSL_CLIENT_HELLO; 03391 break; 03392 03393 /* 03394 * ==> ClientHello 03395 */ 03396 case MBEDTLS_SSL_CLIENT_HELLO: 03397 ret = ssl_write_client_hello( ssl ); 03398 break; 03399 03400 /* 03401 * <== ServerHello 03402 * Certificate 03403 * ( ServerKeyExchange ) 03404 * ( CertificateRequest ) 03405 * ServerHelloDone 03406 */ 03407 case MBEDTLS_SSL_SERVER_HELLO: 03408 ret = ssl_parse_server_hello( ssl ); 03409 break; 03410 03411 case MBEDTLS_SSL_SERVER_CERTIFICATE: 03412 ret = mbedtls_ssl_parse_certificate( ssl ); 03413 break; 03414 03415 case MBEDTLS_SSL_SERVER_KEY_EXCHANGE: 03416 ret = ssl_parse_server_key_exchange( ssl ); 03417 break; 03418 03419 case MBEDTLS_SSL_CERTIFICATE_REQUEST: 03420 ret = ssl_parse_certificate_request( ssl ); 03421 break; 03422 03423 case MBEDTLS_SSL_SERVER_HELLO_DONE: 03424 ret = ssl_parse_server_hello_done( ssl ); 03425 break; 03426 03427 /* 03428 * ==> ( Certificate/Alert ) 03429 * ClientKeyExchange 03430 * ( CertificateVerify ) 03431 * ChangeCipherSpec 03432 * Finished 03433 */ 03434 case MBEDTLS_SSL_CLIENT_CERTIFICATE: 03435 ret = mbedtls_ssl_write_certificate( ssl ); 03436 break; 03437 03438 case MBEDTLS_SSL_CLIENT_KEY_EXCHANGE: 03439 ret = ssl_write_client_key_exchange( ssl ); 03440 break; 03441 03442 case MBEDTLS_SSL_CERTIFICATE_VERIFY: 03443 ret = ssl_write_certificate_verify( ssl ); 03444 break; 03445 03446 case MBEDTLS_SSL_CLIENT_CHANGE_CIPHER_SPEC: 03447 ret = mbedtls_ssl_write_change_cipher_spec( ssl ); 03448 break; 03449 03450 case MBEDTLS_SSL_CLIENT_FINISHED: 03451 ret = mbedtls_ssl_write_finished( ssl ); 03452 break; 03453 03454 /* 03455 * <== ( NewSessionTicket ) 03456 * ChangeCipherSpec 03457 * Finished 03458 */ 03459 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 03460 case MBEDTLS_SSL_SERVER_NEW_SESSION_TICKET: 03461 ret = ssl_parse_new_session_ticket( ssl ); 03462 break; 03463 #endif 03464 03465 case MBEDTLS_SSL_SERVER_CHANGE_CIPHER_SPEC: 03466 ret = mbedtls_ssl_parse_change_cipher_spec( ssl ); 03467 break; 03468 03469 case MBEDTLS_SSL_SERVER_FINISHED: 03470 ret = mbedtls_ssl_parse_finished( ssl ); 03471 break; 03472 03473 case MBEDTLS_SSL_FLUSH_BUFFERS: 03474 MBEDTLS_SSL_DEBUG_MSG( 2, ( "handshake: done" ) ); 03475 ssl->state = MBEDTLS_SSL_HANDSHAKE_WRAPUP; 03476 break; 03477 03478 case MBEDTLS_SSL_HANDSHAKE_WRAPUP: 03479 mbedtls_ssl_handshake_wrapup( ssl ); 03480 break; 03481 03482 default: 03483 MBEDTLS_SSL_DEBUG_MSG( 1, ( "invalid state %d", ssl->state ) ); 03484 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 03485 } 03486 03487 return( ret ); 03488 } 03489 #endif /* MBEDTLS_SSL_CLI_C */
Generated on Sun Jul 17 2022 08:25:31 by
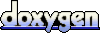