
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
sn_coap_parser_stub.c
00001 /* 00002 * Copyright (c) 2011-2017 ARM Limited. All Rights Reserved. 00003 */ 00004 00005 /** 00006 *\file sn_coap_parser.c 00007 * 00008 * \brief CoAP Header parser 00009 * 00010 * Functionality: Parses CoAP Header 00011 * 00012 */ 00013 00014 #include "ns_types.h" 00015 #include "sn_coap_protocol.h" 00016 #include "sn_coap_parser_stub.h" 00017 00018 sn_coap_parser_def sn_coap_parser_stub; 00019 00020 sn_coap_hdr_s *sn_coap_parser(struct coap_s *handle, uint16_t packet_data_len, uint8_t *packet_data_ptr, coap_version_e *coap_version_ptr) 00021 { 00022 return sn_coap_parser_stub.expectedHeader; 00023 } 00024 00025 void sn_coap_parser_release_allocated_coap_msg_mem(struct coap_s *handle, sn_coap_hdr_s *freed_coap_msg_ptr) 00026 { 00027 if (freed_coap_msg_ptr != NULL) { 00028 if (freed_coap_msg_ptr->uri_path_ptr != NULL) { 00029 free(freed_coap_msg_ptr->uri_path_ptr); 00030 } 00031 00032 if (freed_coap_msg_ptr->token_ptr != NULL) { 00033 free(freed_coap_msg_ptr->token_ptr); 00034 } 00035 00036 if (freed_coap_msg_ptr->options_list_ptr != NULL) { 00037 if (freed_coap_msg_ptr->options_list_ptr->proxy_uri_ptr != NULL) { 00038 free(freed_coap_msg_ptr->options_list_ptr->proxy_uri_ptr); 00039 } 00040 00041 if (freed_coap_msg_ptr->options_list_ptr->etag_ptr != NULL) { 00042 free(freed_coap_msg_ptr->options_list_ptr->etag_ptr); 00043 } 00044 00045 if (freed_coap_msg_ptr->options_list_ptr->uri_host_ptr != NULL) { 00046 free(freed_coap_msg_ptr->options_list_ptr->uri_host_ptr); 00047 } 00048 00049 if (freed_coap_msg_ptr->options_list_ptr->location_path_ptr != NULL) { 00050 free(freed_coap_msg_ptr->options_list_ptr->location_path_ptr); 00051 } 00052 00053 if (freed_coap_msg_ptr->options_list_ptr->location_query_ptr != NULL) { 00054 free(freed_coap_msg_ptr->options_list_ptr->location_query_ptr); 00055 } 00056 00057 if (freed_coap_msg_ptr->options_list_ptr->uri_query_ptr != NULL) { 00058 free(freed_coap_msg_ptr->options_list_ptr->uri_query_ptr); 00059 } 00060 00061 free(freed_coap_msg_ptr->options_list_ptr); 00062 } 00063 00064 free(freed_coap_msg_ptr); 00065 freed_coap_msg_ptr = NULL; 00066 } 00067 }
Generated on Sun Jul 17 2022 08:25:30 by
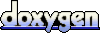