
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
sn_coap_header.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2011-2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 /** 00018 * \file sn_coap_header.h 00019 * 00020 * \brief CoAP C-library User header interface header file 00021 */ 00022 00023 #ifndef SN_COAP_HEADER_H_ 00024 #define SN_COAP_HEADER_H_ 00025 00026 #ifdef __cplusplus 00027 extern "C" { 00028 #endif 00029 00030 /* Handle structure */ 00031 struct coap_s; 00032 00033 /* * * * * * * * * * * * * * */ 00034 /* * * * ENUMERATIONS * * * */ 00035 /* * * * * * * * * * * * * * */ 00036 00037 /** 00038 * \brief Enumeration for CoAP Version 00039 */ 00040 typedef enum coap_version_ { 00041 COAP_VERSION_1 = 0x40, 00042 COAP_VERSION_UNKNOWN = 0xFF 00043 } coap_version_e; 00044 00045 /** 00046 * \brief Enumeration for CoAP Message type, used in CoAP Header 00047 */ 00048 typedef enum sn_coap_msg_type_ { 00049 COAP_MSG_TYPE_CONFIRMABLE = 0x00, /**< Reliable Request messages */ 00050 COAP_MSG_TYPE_NON_CONFIRMABLE = 0x10, /**< Non-reliable Request and Response messages */ 00051 COAP_MSG_TYPE_ACKNOWLEDGEMENT = 0x20, /**< Response to a Confirmable Request */ 00052 COAP_MSG_TYPE_RESET = 0x30 /**< Answer a Bad Request */ 00053 } sn_coap_msg_type_e; 00054 00055 /** 00056 * \brief Enumeration for CoAP Message code, used in CoAP Header 00057 */ 00058 typedef enum sn_coap_msg_code_ { 00059 COAP_MSG_CODE_EMPTY = 0, 00060 COAP_MSG_CODE_REQUEST_GET = 1, 00061 COAP_MSG_CODE_REQUEST_POST = 2, 00062 COAP_MSG_CODE_REQUEST_PUT = 3, 00063 COAP_MSG_CODE_REQUEST_DELETE = 4, 00064 00065 COAP_MSG_CODE_RESPONSE_CREATED = 65, 00066 COAP_MSG_CODE_RESPONSE_DELETED = 66, 00067 COAP_MSG_CODE_RESPONSE_VALID = 67, 00068 COAP_MSG_CODE_RESPONSE_CHANGED = 68, 00069 COAP_MSG_CODE_RESPONSE_CONTENT = 69, 00070 COAP_MSG_CODE_RESPONSE_CONTINUE = 95, 00071 COAP_MSG_CODE_RESPONSE_BAD_REQUEST = 128, 00072 COAP_MSG_CODE_RESPONSE_UNAUTHORIZED = 129, 00073 COAP_MSG_CODE_RESPONSE_BAD_OPTION = 130, 00074 COAP_MSG_CODE_RESPONSE_FORBIDDEN = 131, 00075 COAP_MSG_CODE_RESPONSE_NOT_FOUND = 132, 00076 COAP_MSG_CODE_RESPONSE_METHOD_NOT_ALLOWED = 133, 00077 COAP_MSG_CODE_RESPONSE_NOT_ACCEPTABLE = 134, 00078 COAP_MSG_CODE_RESPONSE_REQUEST_ENTITY_INCOMPLETE = 136, 00079 COAP_MSG_CODE_RESPONSE_PRECONDITION_FAILED = 140, 00080 COAP_MSG_CODE_RESPONSE_REQUEST_ENTITY_TOO_LARGE = 141, 00081 COAP_MSG_CODE_RESPONSE_UNSUPPORTED_CONTENT_FORMAT = 143, 00082 COAP_MSG_CODE_RESPONSE_INTERNAL_SERVER_ERROR = 160, 00083 COAP_MSG_CODE_RESPONSE_NOT_IMPLEMENTED = 161, 00084 COAP_MSG_CODE_RESPONSE_BAD_GATEWAY = 162, 00085 COAP_MSG_CODE_RESPONSE_SERVICE_UNAVAILABLE = 163, 00086 COAP_MSG_CODE_RESPONSE_GATEWAY_TIMEOUT = 164, 00087 COAP_MSG_CODE_RESPONSE_PROXYING_NOT_SUPPORTED = 165 00088 } sn_coap_msg_code_e; 00089 00090 /** 00091 * \brief Enumeration for CoAP Option number, used in CoAP Header 00092 */ 00093 typedef enum sn_coap_option_numbers_ { 00094 COAP_OPTION_IF_MATCH = 1, 00095 COAP_OPTION_URI_HOST = 3, 00096 COAP_OPTION_ETAG = 4, 00097 COAP_OPTION_IF_NONE_MATCH = 5, 00098 COAP_OPTION_OBSERVE = 6, 00099 COAP_OPTION_URI_PORT = 7, 00100 COAP_OPTION_LOCATION_PATH = 8, 00101 COAP_OPTION_URI_PATH = 11, 00102 COAP_OPTION_CONTENT_FORMAT = 12, 00103 COAP_OPTION_MAX_AGE = 14, 00104 COAP_OPTION_URI_QUERY = 15, 00105 COAP_OPTION_ACCEPT = 17, 00106 COAP_OPTION_LOCATION_QUERY = 20, 00107 COAP_OPTION_BLOCK2 = 23, 00108 COAP_OPTION_BLOCK1 = 27, 00109 COAP_OPTION_SIZE2 = 28, 00110 COAP_OPTION_PROXY_URI = 35, 00111 COAP_OPTION_PROXY_SCHEME = 39, 00112 COAP_OPTION_SIZE1 = 60 00113 // 128 = (Reserved) 00114 // 132 = (Reserved) 00115 // 136 = (Reserved) 00116 } sn_coap_option_numbers_e; 00117 00118 /** 00119 * \brief Enumeration for CoAP Content Format codes 00120 */ 00121 typedef enum sn_coap_content_format_ { 00122 COAP_CT_NONE = -1, // internal 00123 COAP_CT_TEXT_PLAIN = 0, 00124 COAP_CT_LINK_FORMAT = 40, 00125 COAP_CT_XML = 41, 00126 COAP_CT_OCTET_STREAM = 42, 00127 COAP_CT_EXI = 47, 00128 COAP_CT_JSON = 50, 00129 COAP_CT__MAX = 0xffff 00130 } sn_coap_content_format_e; 00131 00132 /** 00133 * \brief Enumeration for CoAP Observe option values 00134 * 00135 * draft-ietf-core-observe-16 00136 */ 00137 typedef enum sn_coap_observe_ { 00138 COAP_OBSERVE_NONE = -1, // internal 00139 00140 // Values for GET requests 00141 COAP_OBSERVE_REGISTER = 0, 00142 COAP_OBSERVE_DEREGISTER = 1, 00143 00144 // In responses, value is a 24-bit opaque sequence number 00145 COAP_OBSERVE__MAX = 0xffffff 00146 } sn_coap_observe_e; 00147 00148 /** 00149 * \brief Enumeration for CoAP status, used in CoAP Header 00150 */ 00151 typedef enum sn_coap_status_ { 00152 COAP_STATUS_OK = 0, /**< Default value is OK */ 00153 COAP_STATUS_PARSER_ERROR_IN_HEADER = 1, /**< CoAP will send Reset message to invalid message sender */ 00154 COAP_STATUS_PARSER_DUPLICATED_MSG = 2, /**< CoAP will send Acknowledgement message to duplicated message sender */ 00155 COAP_STATUS_PARSER_BLOCKWISE_MSG_RECEIVING = 3, /**< User will get whole message after all message blocks received. 00156 User must release messages with this status. */ 00157 COAP_STATUS_PARSER_BLOCKWISE_ACK = 4, /**< Acknowledgement for sent Blockwise message received */ 00158 COAP_STATUS_PARSER_BLOCKWISE_MSG_REJECTED = 5, /**< Blockwise message received but not supported by compiling switch */ 00159 COAP_STATUS_PARSER_BLOCKWISE_MSG_RECEIVED = 6, /**< Blockwise message fully received and returned to app. 00160 User must take care of releasing whole payload of the blockwise messages */ 00161 COAP_STATUS_BUILDER_MESSAGE_SENDING_FAILED = 7 /**< When re-transmissions have been done and ACK not received, CoAP library calls 00162 RX callback with this status */ 00163 } sn_coap_status_e; 00164 00165 00166 /* * * * * * * * * * * * * */ 00167 /* * * * STRUCTURES * * * */ 00168 /* * * * * * * * * * * * * */ 00169 00170 /** 00171 * \brief Structure for CoAP Options 00172 */ 00173 typedef struct sn_coap_options_list_ { 00174 uint8_t etag_len; /**< 1-8 bytes. Repeatable */ 00175 unsigned int use_size1:1; 00176 unsigned int use_size2:1; 00177 00178 uint16_t proxy_uri_len; /**< 1-1034 bytes. */ 00179 uint16_t uri_host_len; /**< 1-255 bytes. */ 00180 uint16_t location_path_len; /**< 0-255 bytes. Repeatable */ 00181 uint16_t location_query_len; /**< 0-255 bytes. Repeatable */ 00182 uint16_t uri_query_len; /**< 1-255 bytes. Repeatable */ 00183 00184 sn_coap_content_format_e accept; /**< Value 0-65535. COAP_CT_NONE if not used */ 00185 00186 uint32_t max_age; /**< Value in seconds (default is 60) */ 00187 uint32_t size1; /**< 0-4 bytes. */ 00188 uint32_t size2; /**< 0-4 bytes. */ 00189 int32_t uri_port; /**< Value 0-65535. -1 if not used */ 00190 int32_t observe; /**< Value 0-0xffffff. -1 if not used */ 00191 int32_t block1; /**< Value 0-0xffffff. -1 if not used. Not for user */ 00192 int32_t block2; /**< Value 0-0xffffff. -1 if not used. Not for user */ 00193 00194 uint8_t *proxy_uri_ptr; /**< Must be set to NULL if not used */ 00195 uint8_t *etag_ptr; /**< Must be set to NULL if not used */ 00196 uint8_t *uri_host_ptr; /**< Must be set to NULL if not used */ 00197 uint8_t *location_path_ptr; /**< Must be set to NULL if not used */ 00198 uint8_t *location_query_ptr; /**< Must be set to NULL if not used */ 00199 uint8_t *uri_query_ptr; /**< Must be set to NULL if not used */ 00200 } sn_coap_options_list_s; 00201 00202 /* !!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!! */ 00203 /* !!! Main CoAP message struct !!! */ 00204 /* !!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!! */ 00205 00206 /** 00207 * \brief Main CoAP message struct 00208 */ 00209 typedef struct sn_coap_hdr_ { 00210 uint8_t token_len; /**< 1-8 bytes. */ 00211 00212 sn_coap_status_e coap_status; /**< Used for telling to User special cases when parsing message */ 00213 sn_coap_msg_code_e msg_code; /**< Empty: 0; Requests: 1-31; Responses: 64-191 */ 00214 00215 sn_coap_msg_type_e msg_type; /**< Confirmable, Non-Confirmable, Acknowledgement or Reset */ 00216 sn_coap_content_format_e content_format; /**< Set to COAP_CT_NONE if not used */ 00217 00218 uint16_t msg_id; /**< Message ID. Parser sets parsed message ID, builder sets message ID of built coap message */ 00219 uint16_t uri_path_len; /**< 0-255 bytes. Repeatable. */ 00220 uint16_t payload_len; /**< Must be set to zero if not used */ 00221 00222 uint8_t *token_ptr; /**< Must be set to NULL if not used */ 00223 uint8_t *uri_path_ptr; /**< Must be set to NULL if not used. E.g: temp1/temp2 */ 00224 uint8_t *payload_ptr; /**< Must be set to NULL if not used */ 00225 00226 /* Here are not so often used Options */ 00227 sn_coap_options_list_s *options_list_ptr; /**< Must be set to NULL if not used */ 00228 } sn_coap_hdr_s; 00229 00230 /* * * * * * * * * * * * * * */ 00231 /* * * * ENUMERATIONS * * * */ 00232 /* * * * * * * * * * * * * * */ 00233 00234 00235 /** 00236 * \brief Used protocol 00237 */ 00238 typedef enum sn_nsdl_capab_ { 00239 SN_NSDL_PROTOCOL_HTTP = 0x01, /**< Unsupported */ 00240 SN_NSDL_PROTOCOL_HTTPS = 0x02, /**< Unsupported */ 00241 SN_NSDL_PROTOCOL_COAP = 0x04 /**< Supported */ 00242 } sn_nsdl_capab_e; 00243 00244 /* * * * * * * * * * * * * */ 00245 /* * * * STRUCTURES * * * */ 00246 /* * * * * * * * * * * * * */ 00247 00248 00249 /** 00250 * \brief Used for creating manually registration message with sn_coap_register() 00251 */ 00252 typedef struct registration_info_ { 00253 uint8_t endpoint_len; 00254 uint8_t endpoint_type_len; 00255 uint16_t links_len; 00256 00257 uint8_t *endpoint_ptr; /**< Endpoint name */ 00258 uint8_t *endpoint_type_ptr; /**< Endpoint type */ 00259 uint8_t *links_ptr; /**< Resource registration string */ 00260 } registration_info_t; 00261 00262 00263 /** 00264 * \brief Address type of given address 00265 */ 00266 typedef enum sn_nsdl_addr_type_ { 00267 SN_NSDL_ADDRESS_TYPE_IPV6 = 0x01, /**< Supported */ 00268 SN_NSDL_ADDRESS_TYPE_IPV4 = 0x02, /**< Supported */ 00269 SN_NSDL_ADDRESS_TYPE_HOSTNAME = 0x03, /**< Unsupported */ 00270 SN_NSDL_ADDRESS_TYPE_NONE = 0xFF 00271 } sn_nsdl_addr_type_e; 00272 00273 /** 00274 * \brief Address structure of Packet data 00275 */ 00276 typedef struct sn_nsdl_addr_ { 00277 uint8_t addr_len; 00278 sn_nsdl_addr_type_e type; 00279 uint16_t port; 00280 uint8_t *addr_ptr; 00281 } sn_nsdl_addr_s; 00282 00283 00284 /* * * * * * * * * * * * * * * * * * * * * * */ 00285 /* * * * EXTERNAL FUNCTION PROTOTYPES * * * */ 00286 /* * * * * * * * * * * * * * * * * * * * * * */ 00287 /** 00288 * \fn sn_coap_hdr_s *sn_coap_parser(struct coap_s *handle, uint16_t packet_data_len, uint8_t *packet_data_ptr, coap_version_e *coap_version_ptr) 00289 * 00290 * \brief Parses CoAP message from given Packet data 00291 * 00292 * \param *handle Pointer to CoAP library handle 00293 * 00294 * \param packet_data_len is length of given Packet data to be parsed to CoAP message 00295 * 00296 * \param *packet_data_ptr is source for Packet data to be parsed to CoAP message 00297 * 00298 * \param *coap_version_ptr is destination for parsed CoAP specification version 00299 * 00300 * \return Return value is pointer to parsed CoAP message.\n 00301 * In following failure cases NULL is returned:\n 00302 * -Failure in given pointer (= NULL)\n 00303 * -Failure in memory allocation (malloc() returns NULL) 00304 */ 00305 extern sn_coap_hdr_s *sn_coap_parser(struct coap_s *handle, uint16_t packet_data_len, uint8_t *packet_data_ptr, coap_version_e *coap_version_ptr); 00306 00307 /** 00308 * \fn void sn_coap_parser_release_allocated_coap_msg_mem(struct coap_s *handle, sn_coap_hdr_s *freed_coap_msg_ptr) 00309 * 00310 * \brief Releases memory of given CoAP message 00311 * 00312 * Note!!! Does not release Payload part 00313 * 00314 * \param *handle Pointer to CoAP library handle 00315 * 00316 * \param *freed_coap_msg_ptr is pointer to released CoAP message 00317 */ 00318 extern void sn_coap_parser_release_allocated_coap_msg_mem(struct coap_s *handle, sn_coap_hdr_s *freed_coap_msg_ptr); 00319 00320 /** 00321 * \fn int16_t sn_coap_builder(uint8_t *dst_packet_data_ptr, sn_coap_hdr_s *src_coap_msg_ptr) 00322 * 00323 * \brief Builds an outgoing message buffer from a CoAP header structure. 00324 * 00325 * \param *dst_packet_data_ptr is pointer to allocated destination to built CoAP packet 00326 * 00327 * \param *src_coap_msg_ptr is pointer to source structure for building Packet data 00328 * 00329 * \return Return value is byte count of built Packet data. In failure cases:\n 00330 * -1 = Failure in given CoAP header structure\n 00331 * -2 = Failure in given pointer (= NULL) 00332 */ 00333 extern int16_t sn_coap_builder(uint8_t *dst_packet_data_ptr, sn_coap_hdr_s *src_coap_msg_ptr); 00334 00335 /** 00336 * \fn uint16_t sn_coap_builder_calc_needed_packet_data_size(sn_coap_hdr_s *src_coap_msg_ptr) 00337 * 00338 * \brief Calculates needed Packet data memory size for given CoAP message 00339 * 00340 * \param *src_coap_msg_ptr is pointer to data which needed Packet 00341 * data length is calculated 00342 * 00343 * \return Return value is count of needed memory as bytes for build Packet data 00344 * Null if failed 00345 */ 00346 extern uint16_t sn_coap_builder_calc_needed_packet_data_size(sn_coap_hdr_s *src_coap_msg_ptr); 00347 00348 /** 00349 * \fn int16_t sn_coap_builder_2(uint8_t *dst_packet_data_ptr, sn_coap_hdr_s *src_coap_msg_ptr, uint16_t blockwise_size) 00350 * 00351 * \brief Builds an outgoing message buffer from a CoAP header structure. 00352 * 00353 * \param *dst_packet_data_ptr is pointer to allocated destination to built CoAP packet 00354 * 00355 * \param *src_coap_msg_ptr is pointer to source structure for building Packet data 00356 * 00357 * \param blockwise_payload_size Blockwise message maximum payload size 00358 * 00359 * \return Return value is byte count of built Packet data. In failure cases:\n 00360 * -1 = Failure in given CoAP header structure\n 00361 * -2 = Failure in given pointer (= NULL) 00362 */ 00363 extern int16_t sn_coap_builder_2(uint8_t *dst_packet_data_ptr, sn_coap_hdr_s *src_coap_msg_ptr, uint16_t blockwise_payload_size); 00364 00365 /** 00366 * \fn uint16_t sn_coap_builder_calc_needed_packet_data_size_2(sn_coap_hdr_s *src_coap_msg_ptr, uint16_t blockwise_payload_size) 00367 * 00368 * \brief Calculates needed Packet data memory size for given CoAP message 00369 * 00370 * \param *src_coap_msg_ptr is pointer to data which needed Packet 00371 * data length is calculated 00372 * \param blockwise_payload_size Blockwise message maximum payload size 00373 * 00374 * \return Return value is count of needed memory as bytes for build Packet data 00375 * Null if failed 00376 */ 00377 extern uint16_t sn_coap_builder_calc_needed_packet_data_size_2(sn_coap_hdr_s *src_coap_msg_ptr, uint16_t blockwise_payload_size); 00378 00379 /** 00380 * \fn sn_coap_hdr_s *sn_coap_build_response(struct coap_s *handle, sn_coap_hdr_s *coap_packet_ptr, uint8_t msg_code) 00381 * 00382 * \brief Prepares generic response packet from a request packet. This function allocates memory for the resulting sn_coap_hdr_s 00383 * 00384 * \param *handle Pointer to CoAP library handle 00385 * \param *coap_packet_ptr The request packet pointer 00386 * \param msg_code response messages code 00387 * 00388 * \return *coap_packet_ptr The allocated and pre-filled response packet pointer 00389 * NULL Error in parsing the request 00390 * 00391 */ 00392 extern sn_coap_hdr_s *sn_coap_build_response(struct coap_s *handle, sn_coap_hdr_s *coap_packet_ptr, uint8_t msg_code); 00393 00394 /** 00395 * \brief Initialise a message structure to empty 00396 * 00397 * \param *coap_msg_ptr is pointer to CoAP message to initialise 00398 * 00399 * \return Return value is pointer passed in 00400 */ 00401 extern sn_coap_hdr_s *sn_coap_parser_init_message(sn_coap_hdr_s *coap_msg_ptr); 00402 00403 /** 00404 * \brief Allocate an empty message structure 00405 * 00406 * \param *handle Pointer to CoAP library handle 00407 * 00408 * \return Return value is pointer to an empty CoAP message.\n 00409 * In following failure cases NULL is returned:\n 00410 * -Failure in given pointer (= NULL)\n 00411 * -Failure in memory allocation (malloc() returns NULL) 00412 */ 00413 extern sn_coap_hdr_s *sn_coap_parser_alloc_message(struct coap_s *handle); 00414 00415 /** 00416 * \brief Allocates and initializes options list structure 00417 * 00418 * \param *handle Pointer to CoAP library handle 00419 * \param *coap_msg_ptr is pointer to CoAP message that will contain the options 00420 * 00421 * If the message already has a pointer to an option structure, that pointer 00422 * is returned, rather than a new structure being allocated. 00423 * 00424 * \return Return value is pointer to the CoAP options structure.\n 00425 * In following failure cases NULL is returned:\n 00426 * -Failure in given pointer (= NULL)\n 00427 * -Failure in memory allocation (malloc() returns NULL) 00428 */ 00429 extern sn_coap_options_list_s *sn_coap_parser_alloc_options(struct coap_s *handle, sn_coap_hdr_s *coap_msg_ptr); 00430 00431 #ifdef __cplusplus 00432 } 00433 #endif 00434 00435 #endif /* SN_COAP_HEADER_H_ */
Generated on Sun Jul 17 2022 08:25:30 by
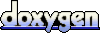