
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
size.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2013 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 import sys 00018 from os.path import join, abspath, dirname, exists, splitext 00019 from subprocess import Popen, PIPE 00020 import csv 00021 from collections import defaultdict 00022 00023 ROOT = abspath(join(dirname(__file__), "..")) 00024 sys.path.insert(0, ROOT) 00025 00026 from tools.paths import BUILD_DIR, TOOLS_DATA 00027 from tools.settings import GCC_ARM_PATH 00028 from tools.tests import TEST_MAP 00029 from tools.build_api import build_mbed_libs, build_project 00030 00031 SIZE = join(GCC_ARM_PATH, 'arm-none-eabi-size') 00032 00033 def get_size(path): 00034 out = Popen([SIZE, path], stdout=PIPE).communicate()[0] 00035 return map(int, out.splitlines()[1].split()[:4]) 00036 00037 def get_percentage(before, after): 00038 if before == 0: 00039 return 0 if after == 0 else 100.0 00040 return float(after - before) / float(before) * 100.0 00041 00042 def human_size(val): 00043 if val>1024: 00044 return "%.0fKb" % (float(val)/1024.0) 00045 return "%d" % val 00046 00047 def print_diff(name, before, after): 00048 print "%s: (%s -> %s) %.2f%%" % (name, human_size(before) , human_size(after) , get_percentage(before , after)) 00049 00050 BENCHMARKS = [ 00051 ("BENCHMARK_1", "CENV"), 00052 ("BENCHMARK_2", "PRINTF"), 00053 ("BENCHMARK_3", "FP"), 00054 ("BENCHMARK_4", "MBED"), 00055 ("BENCHMARK_5", "ALL"), 00056 ] 00057 BENCHMARK_DATA_PATH = join(TOOLS_DATA, 'benchmarks.csv') 00058 00059 00060 def benchmarks(): 00061 # CSV Data 00062 csv_data = csv.writer(open(BENCHMARK_DATA_PATH, 'wb')) 00063 csv_data.writerow(['Toolchain', "Target", "Benchmark", "code", "data", "bss", "flash"]) 00064 00065 # Build 00066 for toolchain in ['ARM', 'uARM', 'GCC_CR', 'GCC_ARM']: 00067 for mcu in ["LPC1768", "LPC11U24"]: 00068 # Build Libraries 00069 build_mbed_libs(mcu, toolchain) 00070 00071 # Build benchmarks 00072 build_dir = join(BUILD_DIR, "benchmarks", mcu, toolchain) 00073 for test_id, title in BENCHMARKS: 00074 # Build Benchmark 00075 try: 00076 test = TEST_MAP[test_id] 00077 path = build_project(test.source_dir, join(build_dir, test_id), 00078 mcu, toolchain, test.dependencies) 00079 base, ext = splitext(path) 00080 # Check Size 00081 code, data, bss, flash = get_size(base+'.elf') 00082 csv_data.writerow([toolchain, mcu, title, code, data, bss, flash]) 00083 except Exception, e: 00084 print "Unable to build %s for toolchain %s targeting %s" % (test_id, toolchain, mcu) 00085 print e 00086 00087 00088 def compare(t1, t2, target): 00089 if not exists(BENCHMARK_DATA_PATH): 00090 benchmarks() 00091 else: 00092 print "Loading: %s" % BENCHMARK_DATA_PATH 00093 00094 data = csv.reader(open(BENCHMARK_DATA_PATH, 'rb')) 00095 00096 benchmarks_data = defaultdict(dict) 00097 for (toolchain, mcu, name, code, data, bss, flash) in data: 00098 if target == mcu: 00099 for t in [t1, t2]: 00100 if toolchain == t: 00101 benchmarks_data[name][t] = map(int, (code, data, bss, flash)) 00102 00103 print "%s vs %s for %s" % (t1, t2, target) 00104 for name, data in benchmarks_data.iteritems(): 00105 try: 00106 # Check Size 00107 code_a, data_a, bss_a, flash_a = data[t1] 00108 code_u, data_u, bss_u, flash_u = data[t2] 00109 00110 print "\n=== %s ===" % name 00111 print_diff("code", code_a , code_u) 00112 print_diff("data", data_a , data_u) 00113 print_diff("bss", bss_a , bss_u) 00114 print_diff("flash", flash_a , flash_u) 00115 except Exception, e: 00116 print "No data for benchmark %s" % (name) 00117 print e 00118 00119 00120 if __name__ == '__main__': 00121 compare("GCC_CR", "LPC1768")
Generated on Sun Jul 17 2022 08:25:30 by
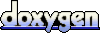