
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
shalib.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2014-2016 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 /** 00015 * \file shalib.h 00016 * \brief SHA256 Library API. 00017 * 00018 * \section hmac256-inctuction HMAC256 process sequence: 00019 * 1. SHALIB_init_HMAC(), Init HMAC IN process by given security signature material 00020 * 2. SHALIB_push_data_HMAC(), Give data sectors(s) one by one 00021 * 3. SHALIB_finish_HMAC(), Finish HMAC and save SHA256 hash to given buffer 00022 * 00023 * \section prf256-inctuction PRF256 process sequence: 00024 * 1. shalib_prf_param_get(), Init PRF and get configure structure 00025 * 2. Set the following parameters to configure structure: 00026 * - HMAC security signature pointer and length 00027 * - Label text 00028 * - Seed data and length 00029 * 3. shalib_prf_calc(), Calc PRF256 HASH 00030 * 00031 */ 00032 00033 #ifndef SHALIB_H_ 00034 #define SHALIB_H_ 00035 00036 #include "ns_types.h" 00037 00038 00039 #ifdef __cplusplus 00040 extern "C" { 00041 #endif 00042 00043 /*! 00044 * \struct prf_sec_param_t 00045 * \brief PRF 256 stucture 00046 * This structure is used to configure PRF calc operation: secret, label, seed and buffer before call shalib_prf_calc(). 00047 */ 00048 typedef struct { 00049 const uint8_t *secret; /**< HMAC security signature pointer. */ 00050 uint8_t sec_len; /**< HMAC security signature length. */ 00051 const char *label; /**< PRF label text. */ 00052 const uint8_t *seed; /**< PRF Seed data. */ 00053 uint8_t seedlen; /**< PRF Seed data length. */ 00054 } prf_sec_param_t; 00055 00056 00057 // Use these for cumulative HMAC 00058 /** 00059 * \brief Init HMAC256 operation by given security material. 00060 * 00061 * \param secret A pointer to security material. 00062 * \param sec_len Length of security material. 00063 */ 00064 void SHALIB_init_HMAC(const uint8_t *secret, uint8_t sec_len); // Call this first... 00065 /** 00066 * \brief Push data for HMAC 00067 * 00068 * \param data A pointer to data. 00069 * \param len Length of data. 00070 */ 00071 void SHALIB_push_data_HMAC(const void *data, uint16_t len); // ... add data ... 00072 /** 00073 * \brief Finish HMAC256 operation and save result in given buffer. 00074 * 00075 * \param buffer A pointer to result buffer. 00076 * \param nwords Length of 32-bit register to save to buffer (8= 256 bit and 4= 128-bit). 00077 */ 00078 void SHALIB_finish_HMAC(void *buffer, uint8_t nwords); // ... get the HMAC digest. 00079 00080 00081 /** PRF API */ 00082 /** 00083 * \brief Init PRF library and SHA registers. 00084 * This function returns configure structure where the user needs to set the following items: 00085 * -Security material and length 00086 * -Label text and length 00087 * -Seed data and length 00088 * 00089 * \return A pointer to PRF configure structure. 00090 00091 */ 00092 prf_sec_param_t *shalib_prf_param_get(void); // GET PRF structure 00093 /* SET secret, label, seed & buffer to 256 PRF */ 00094 /** 00095 * \brief Finish PRF256 operation and save result in given buffer. 00096 */ 00097 void shalib_prf_calc(void *output, uint_fast16_t nwords);// GET 256 PRF 00098 #ifdef __cplusplus 00099 } 00100 #endif 00101 #endif /* SHALIB_H_ */
Generated on Sun Jul 17 2022 08:25:30 by
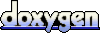