
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
sha512.c
00001 /* 00002 * FIPS-180-2 compliant SHA-384/512 implementation 00003 * 00004 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00005 * SPDX-License-Identifier: Apache-2.0 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00008 * not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00015 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 * 00019 * This file is part of mbed TLS (https://tls.mbed.org) 00020 */ 00021 /* 00022 * The SHA-512 Secure Hash Standard was published by NIST in 2002. 00023 * 00024 * http://csrc.nist.gov/publications/fips/fips180-2/fips180-2.pdf 00025 */ 00026 00027 #if !defined(MBEDTLS_CONFIG_FILE) 00028 #include "mbedtls/config.h" 00029 #else 00030 #include MBEDTLS_CONFIG_FILE 00031 #endif 00032 00033 #if defined(MBEDTLS_SHA512_C) 00034 00035 #include "mbedtls/sha512.h" 00036 00037 #if defined(_MSC_VER) || defined(__WATCOMC__) 00038 #define UL64(x) x##ui64 00039 #else 00040 #define UL64(x) x##ULL 00041 #endif 00042 00043 #include <string.h> 00044 00045 #if defined(MBEDTLS_SELF_TEST) 00046 #if defined(MBEDTLS_PLATFORM_C) 00047 #include "mbedtls/platform.h" 00048 #else 00049 #include <stdio.h> 00050 #include <stdlib.h> 00051 #define mbedtls_printf printf 00052 #define mbedtls_calloc calloc 00053 #define mbedtls_free free 00054 #endif /* MBEDTLS_PLATFORM_C */ 00055 #endif /* MBEDTLS_SELF_TEST */ 00056 00057 #if !defined(MBEDTLS_SHA512_ALT) 00058 00059 /* Implementation that should never be optimized out by the compiler */ 00060 static void mbedtls_zeroize( void *v, size_t n ) { 00061 volatile unsigned char *p = v; while( n-- ) *p++ = 0; 00062 } 00063 00064 /* 00065 * 64-bit integer manipulation macros (big endian) 00066 */ 00067 #ifndef GET_UINT64_BE 00068 #define GET_UINT64_BE(n,b,i) \ 00069 { \ 00070 (n) = ( (uint64_t) (b)[(i) ] << 56 ) \ 00071 | ( (uint64_t) (b)[(i) + 1] << 48 ) \ 00072 | ( (uint64_t) (b)[(i) + 2] << 40 ) \ 00073 | ( (uint64_t) (b)[(i) + 3] << 32 ) \ 00074 | ( (uint64_t) (b)[(i) + 4] << 24 ) \ 00075 | ( (uint64_t) (b)[(i) + 5] << 16 ) \ 00076 | ( (uint64_t) (b)[(i) + 6] << 8 ) \ 00077 | ( (uint64_t) (b)[(i) + 7] ); \ 00078 } 00079 #endif /* GET_UINT64_BE */ 00080 00081 #ifndef PUT_UINT64_BE 00082 #define PUT_UINT64_BE(n,b,i) \ 00083 { \ 00084 (b)[(i) ] = (unsigned char) ( (n) >> 56 ); \ 00085 (b)[(i) + 1] = (unsigned char) ( (n) >> 48 ); \ 00086 (b)[(i) + 2] = (unsigned char) ( (n) >> 40 ); \ 00087 (b)[(i) + 3] = (unsigned char) ( (n) >> 32 ); \ 00088 (b)[(i) + 4] = (unsigned char) ( (n) >> 24 ); \ 00089 (b)[(i) + 5] = (unsigned char) ( (n) >> 16 ); \ 00090 (b)[(i) + 6] = (unsigned char) ( (n) >> 8 ); \ 00091 (b)[(i) + 7] = (unsigned char) ( (n) ); \ 00092 } 00093 #endif /* PUT_UINT64_BE */ 00094 00095 void mbedtls_sha512_init( mbedtls_sha512_context *ctx ) 00096 { 00097 memset( ctx, 0, sizeof( mbedtls_sha512_context ) ); 00098 } 00099 00100 void mbedtls_sha512_free( mbedtls_sha512_context *ctx ) 00101 { 00102 if( ctx == NULL ) 00103 return; 00104 00105 mbedtls_zeroize( ctx, sizeof( mbedtls_sha512_context ) ); 00106 } 00107 00108 void mbedtls_sha512_clone( mbedtls_sha512_context *dst, 00109 const mbedtls_sha512_context *src ) 00110 { 00111 *dst = *src; 00112 } 00113 00114 /* 00115 * SHA-512 context setup 00116 */ 00117 void mbedtls_sha512_starts( mbedtls_sha512_context *ctx, int is384 ) 00118 { 00119 ctx->total [0] = 0; 00120 ctx->total [1] = 0; 00121 00122 if( is384 == 0 ) 00123 { 00124 /* SHA-512 */ 00125 ctx->state [0] = UL64(0x6A09E667F3BCC908); 00126 ctx->state [1] = UL64(0xBB67AE8584CAA73B); 00127 ctx->state [2] = UL64(0x3C6EF372FE94F82B); 00128 ctx->state [3] = UL64(0xA54FF53A5F1D36F1); 00129 ctx->state [4] = UL64(0x510E527FADE682D1); 00130 ctx->state [5] = UL64(0x9B05688C2B3E6C1F); 00131 ctx->state [6] = UL64(0x1F83D9ABFB41BD6B); 00132 ctx->state [7] = UL64(0x5BE0CD19137E2179); 00133 } 00134 else 00135 { 00136 /* SHA-384 */ 00137 ctx->state [0] = UL64(0xCBBB9D5DC1059ED8); 00138 ctx->state [1] = UL64(0x629A292A367CD507); 00139 ctx->state [2] = UL64(0x9159015A3070DD17); 00140 ctx->state [3] = UL64(0x152FECD8F70E5939); 00141 ctx->state [4] = UL64(0x67332667FFC00B31); 00142 ctx->state [5] = UL64(0x8EB44A8768581511); 00143 ctx->state [6] = UL64(0xDB0C2E0D64F98FA7); 00144 ctx->state [7] = UL64(0x47B5481DBEFA4FA4); 00145 } 00146 00147 ctx->is384 = is384; 00148 } 00149 00150 #if !defined(MBEDTLS_SHA512_PROCESS_ALT) 00151 00152 /* 00153 * Round constants 00154 */ 00155 static const uint64_t K[80] = 00156 { 00157 UL64(0x428A2F98D728AE22), UL64(0x7137449123EF65CD), 00158 UL64(0xB5C0FBCFEC4D3B2F), UL64(0xE9B5DBA58189DBBC), 00159 UL64(0x3956C25BF348B538), UL64(0x59F111F1B605D019), 00160 UL64(0x923F82A4AF194F9B), UL64(0xAB1C5ED5DA6D8118), 00161 UL64(0xD807AA98A3030242), UL64(0x12835B0145706FBE), 00162 UL64(0x243185BE4EE4B28C), UL64(0x550C7DC3D5FFB4E2), 00163 UL64(0x72BE5D74F27B896F), UL64(0x80DEB1FE3B1696B1), 00164 UL64(0x9BDC06A725C71235), UL64(0xC19BF174CF692694), 00165 UL64(0xE49B69C19EF14AD2), UL64(0xEFBE4786384F25E3), 00166 UL64(0x0FC19DC68B8CD5B5), UL64(0x240CA1CC77AC9C65), 00167 UL64(0x2DE92C6F592B0275), UL64(0x4A7484AA6EA6E483), 00168 UL64(0x5CB0A9DCBD41FBD4), UL64(0x76F988DA831153B5), 00169 UL64(0x983E5152EE66DFAB), UL64(0xA831C66D2DB43210), 00170 UL64(0xB00327C898FB213F), UL64(0xBF597FC7BEEF0EE4), 00171 UL64(0xC6E00BF33DA88FC2), UL64(0xD5A79147930AA725), 00172 UL64(0x06CA6351E003826F), UL64(0x142929670A0E6E70), 00173 UL64(0x27B70A8546D22FFC), UL64(0x2E1B21385C26C926), 00174 UL64(0x4D2C6DFC5AC42AED), UL64(0x53380D139D95B3DF), 00175 UL64(0x650A73548BAF63DE), UL64(0x766A0ABB3C77B2A8), 00176 UL64(0x81C2C92E47EDAEE6), UL64(0x92722C851482353B), 00177 UL64(0xA2BFE8A14CF10364), UL64(0xA81A664BBC423001), 00178 UL64(0xC24B8B70D0F89791), UL64(0xC76C51A30654BE30), 00179 UL64(0xD192E819D6EF5218), UL64(0xD69906245565A910), 00180 UL64(0xF40E35855771202A), UL64(0x106AA07032BBD1B8), 00181 UL64(0x19A4C116B8D2D0C8), UL64(0x1E376C085141AB53), 00182 UL64(0x2748774CDF8EEB99), UL64(0x34B0BCB5E19B48A8), 00183 UL64(0x391C0CB3C5C95A63), UL64(0x4ED8AA4AE3418ACB), 00184 UL64(0x5B9CCA4F7763E373), UL64(0x682E6FF3D6B2B8A3), 00185 UL64(0x748F82EE5DEFB2FC), UL64(0x78A5636F43172F60), 00186 UL64(0x84C87814A1F0AB72), UL64(0x8CC702081A6439EC), 00187 UL64(0x90BEFFFA23631E28), UL64(0xA4506CEBDE82BDE9), 00188 UL64(0xBEF9A3F7B2C67915), UL64(0xC67178F2E372532B), 00189 UL64(0xCA273ECEEA26619C), UL64(0xD186B8C721C0C207), 00190 UL64(0xEADA7DD6CDE0EB1E), UL64(0xF57D4F7FEE6ED178), 00191 UL64(0x06F067AA72176FBA), UL64(0x0A637DC5A2C898A6), 00192 UL64(0x113F9804BEF90DAE), UL64(0x1B710B35131C471B), 00193 UL64(0x28DB77F523047D84), UL64(0x32CAAB7B40C72493), 00194 UL64(0x3C9EBE0A15C9BEBC), UL64(0x431D67C49C100D4C), 00195 UL64(0x4CC5D4BECB3E42B6), UL64(0x597F299CFC657E2A), 00196 UL64(0x5FCB6FAB3AD6FAEC), UL64(0x6C44198C4A475817) 00197 }; 00198 00199 void mbedtls_sha512_process( mbedtls_sha512_context *ctx, const unsigned char data[128] ) 00200 { 00201 int i; 00202 uint64_t temp1, temp2, W[80]; 00203 uint64_t A, B, C, D, E, F, G, H; 00204 00205 #define SHR(x,n) (x >> n) 00206 #define ROTR(x,n) (SHR(x,n) | (x << (64 - n))) 00207 00208 #define S0(x) (ROTR(x, 1) ^ ROTR(x, 8) ^ SHR(x, 7)) 00209 #define S1(x) (ROTR(x,19) ^ ROTR(x,61) ^ SHR(x, 6)) 00210 00211 #define S2(x) (ROTR(x,28) ^ ROTR(x,34) ^ ROTR(x,39)) 00212 #define S3(x) (ROTR(x,14) ^ ROTR(x,18) ^ ROTR(x,41)) 00213 00214 #define F0(x,y,z) ((x & y) | (z & (x | y))) 00215 #define F1(x,y,z) (z ^ (x & (y ^ z))) 00216 00217 #define P(a,b,c,d,e,f,g,h,x,K) \ 00218 { \ 00219 temp1 = h + S3(e) + F1(e,f,g) + K + x; \ 00220 temp2 = S2(a) + F0(a,b,c); \ 00221 d += temp1; h = temp1 + temp2; \ 00222 } 00223 00224 for( i = 0; i < 16; i++ ) 00225 { 00226 GET_UINT64_BE( W[i], data, i << 3 ); 00227 } 00228 00229 for( ; i < 80; i++ ) 00230 { 00231 W[i] = S1(W[i - 2]) + W[i - 7] + 00232 S0(W[i - 15]) + W[i - 16]; 00233 } 00234 00235 A = ctx->state [0]; 00236 B = ctx->state [1]; 00237 C = ctx->state [2]; 00238 D = ctx->state [3]; 00239 E = ctx->state [4]; 00240 F = ctx->state [5]; 00241 G = ctx->state [6]; 00242 H = ctx->state [7]; 00243 i = 0; 00244 00245 do 00246 { 00247 P( A, B, C, D, E, F, G, H, W[i], K[i] ); i++; 00248 P( H, A, B, C, D, E, F, G, W[i], K[i] ); i++; 00249 P( G, H, A, B, C, D, E, F, W[i], K[i] ); i++; 00250 P( F, G, H, A, B, C, D, E, W[i], K[i] ); i++; 00251 P( E, F, G, H, A, B, C, D, W[i], K[i] ); i++; 00252 P( D, E, F, G, H, A, B, C, W[i], K[i] ); i++; 00253 P( C, D, E, F, G, H, A, B, W[i], K[i] ); i++; 00254 P( B, C, D, E, F, G, H, A, W[i], K[i] ); i++; 00255 } 00256 while( i < 80 ); 00257 00258 ctx->state [0] += A; 00259 ctx->state [1] += B; 00260 ctx->state [2] += C; 00261 ctx->state [3] += D; 00262 ctx->state [4] += E; 00263 ctx->state [5] += F; 00264 ctx->state [6] += G; 00265 ctx->state [7] += H; 00266 } 00267 #endif /* !MBEDTLS_SHA512_PROCESS_ALT */ 00268 00269 /* 00270 * SHA-512 process buffer 00271 */ 00272 void mbedtls_sha512_update( mbedtls_sha512_context *ctx, const unsigned char *input, 00273 size_t ilen ) 00274 { 00275 size_t fill; 00276 unsigned int left; 00277 00278 if( ilen == 0 ) 00279 return; 00280 00281 left = (unsigned int) (ctx->total [0] & 0x7F); 00282 fill = 128 - left; 00283 00284 ctx->total [0] += (uint64_t) ilen; 00285 00286 if( ctx->total [0] < (uint64_t) ilen ) 00287 ctx->total [1]++; 00288 00289 if( left && ilen >= fill ) 00290 { 00291 memcpy( (void *) (ctx->buffer + left), input, fill ); 00292 mbedtls_sha512_process( ctx, ctx->buffer ); 00293 input += fill; 00294 ilen -= fill; 00295 left = 0; 00296 } 00297 00298 while( ilen >= 128 ) 00299 { 00300 mbedtls_sha512_process( ctx, input ); 00301 input += 128; 00302 ilen -= 128; 00303 } 00304 00305 if( ilen > 0 ) 00306 memcpy( (void *) (ctx->buffer + left), input, ilen ); 00307 } 00308 00309 static const unsigned char sha512_padding[128] = 00310 { 00311 0x80, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 00312 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 00313 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 00314 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 00315 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 00316 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 00317 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 00318 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 00319 }; 00320 00321 /* 00322 * SHA-512 final digest 00323 */ 00324 void mbedtls_sha512_finish( mbedtls_sha512_context *ctx, unsigned char output[64] ) 00325 { 00326 size_t last, padn; 00327 uint64_t high, low; 00328 unsigned char msglen[16]; 00329 00330 high = ( ctx->total [0] >> 61 ) 00331 | ( ctx->total [1] << 3 ); 00332 low = ( ctx->total [0] << 3 ); 00333 00334 PUT_UINT64_BE( high, msglen, 0 ); 00335 PUT_UINT64_BE( low, msglen, 8 ); 00336 00337 last = (size_t)( ctx->total [0] & 0x7F ); 00338 padn = ( last < 112 ) ? ( 112 - last ) : ( 240 - last ); 00339 00340 mbedtls_sha512_update( ctx, sha512_padding, padn ); 00341 mbedtls_sha512_update( ctx, msglen, 16 ); 00342 00343 PUT_UINT64_BE( ctx->state [0], output, 0 ); 00344 PUT_UINT64_BE( ctx->state [1], output, 8 ); 00345 PUT_UINT64_BE( ctx->state [2], output, 16 ); 00346 PUT_UINT64_BE( ctx->state [3], output, 24 ); 00347 PUT_UINT64_BE( ctx->state [4], output, 32 ); 00348 PUT_UINT64_BE( ctx->state [5], output, 40 ); 00349 00350 if( ctx->is384 == 0 ) 00351 { 00352 PUT_UINT64_BE( ctx->state [6], output, 48 ); 00353 PUT_UINT64_BE( ctx->state [7], output, 56 ); 00354 } 00355 } 00356 00357 #endif /* !MBEDTLS_SHA512_ALT */ 00358 00359 /* 00360 * output = SHA-512( input buffer ) 00361 */ 00362 void mbedtls_sha512( const unsigned char *input, size_t ilen, 00363 unsigned char output[64], int is384 ) 00364 { 00365 mbedtls_sha512_context ctx; 00366 00367 mbedtls_sha512_init( &ctx ); 00368 mbedtls_sha512_starts( &ctx, is384 ); 00369 mbedtls_sha512_update( &ctx, input, ilen ); 00370 mbedtls_sha512_finish( &ctx, output ); 00371 mbedtls_sha512_free( &ctx ); 00372 } 00373 00374 #if defined(MBEDTLS_SELF_TEST) 00375 00376 /* 00377 * FIPS-180-2 test vectors 00378 */ 00379 static const unsigned char sha512_test_buf[3][113] = 00380 { 00381 { "abc" }, 00382 { "abcdefghbcdefghicdefghijdefghijkefghijklfghijklmghijklmn" 00383 "hijklmnoijklmnopjklmnopqklmnopqrlmnopqrsmnopqrstnopqrstu" }, 00384 { "" } 00385 }; 00386 00387 static const int sha512_test_buflen[3] = 00388 { 00389 3, 112, 1000 00390 }; 00391 00392 static const unsigned char sha512_test_sum[6][64] = 00393 { 00394 /* 00395 * SHA-384 test vectors 00396 */ 00397 { 0xCB, 0x00, 0x75, 0x3F, 0x45, 0xA3, 0x5E, 0x8B, 00398 0xB5, 0xA0, 0x3D, 0x69, 0x9A, 0xC6, 0x50, 0x07, 00399 0x27, 0x2C, 0x32, 0xAB, 0x0E, 0xDE, 0xD1, 0x63, 00400 0x1A, 0x8B, 0x60, 0x5A, 0x43, 0xFF, 0x5B, 0xED, 00401 0x80, 0x86, 0x07, 0x2B, 0xA1, 0xE7, 0xCC, 0x23, 00402 0x58, 0xBA, 0xEC, 0xA1, 0x34, 0xC8, 0x25, 0xA7 }, 00403 { 0x09, 0x33, 0x0C, 0x33, 0xF7, 0x11, 0x47, 0xE8, 00404 0x3D, 0x19, 0x2F, 0xC7, 0x82, 0xCD, 0x1B, 0x47, 00405 0x53, 0x11, 0x1B, 0x17, 0x3B, 0x3B, 0x05, 0xD2, 00406 0x2F, 0xA0, 0x80, 0x86, 0xE3, 0xB0, 0xF7, 0x12, 00407 0xFC, 0xC7, 0xC7, 0x1A, 0x55, 0x7E, 0x2D, 0xB9, 00408 0x66, 0xC3, 0xE9, 0xFA, 0x91, 0x74, 0x60, 0x39 }, 00409 { 0x9D, 0x0E, 0x18, 0x09, 0x71, 0x64, 0x74, 0xCB, 00410 0x08, 0x6E, 0x83, 0x4E, 0x31, 0x0A, 0x4A, 0x1C, 00411 0xED, 0x14, 0x9E, 0x9C, 0x00, 0xF2, 0x48, 0x52, 00412 0x79, 0x72, 0xCE, 0xC5, 0x70, 0x4C, 0x2A, 0x5B, 00413 0x07, 0xB8, 0xB3, 0xDC, 0x38, 0xEC, 0xC4, 0xEB, 00414 0xAE, 0x97, 0xDD, 0xD8, 0x7F, 0x3D, 0x89, 0x85 }, 00415 00416 /* 00417 * SHA-512 test vectors 00418 */ 00419 { 0xDD, 0xAF, 0x35, 0xA1, 0x93, 0x61, 0x7A, 0xBA, 00420 0xCC, 0x41, 0x73, 0x49, 0xAE, 0x20, 0x41, 0x31, 00421 0x12, 0xE6, 0xFA, 0x4E, 0x89, 0xA9, 0x7E, 0xA2, 00422 0x0A, 0x9E, 0xEE, 0xE6, 0x4B, 0x55, 0xD3, 0x9A, 00423 0x21, 0x92, 0x99, 0x2A, 0x27, 0x4F, 0xC1, 0xA8, 00424 0x36, 0xBA, 0x3C, 0x23, 0xA3, 0xFE, 0xEB, 0xBD, 00425 0x45, 0x4D, 0x44, 0x23, 0x64, 0x3C, 0xE8, 0x0E, 00426 0x2A, 0x9A, 0xC9, 0x4F, 0xA5, 0x4C, 0xA4, 0x9F }, 00427 { 0x8E, 0x95, 0x9B, 0x75, 0xDA, 0xE3, 0x13, 0xDA, 00428 0x8C, 0xF4, 0xF7, 0x28, 0x14, 0xFC, 0x14, 0x3F, 00429 0x8F, 0x77, 0x79, 0xC6, 0xEB, 0x9F, 0x7F, 0xA1, 00430 0x72, 0x99, 0xAE, 0xAD, 0xB6, 0x88, 0x90, 0x18, 00431 0x50, 0x1D, 0x28, 0x9E, 0x49, 0x00, 0xF7, 0xE4, 00432 0x33, 0x1B, 0x99, 0xDE, 0xC4, 0xB5, 0x43, 0x3A, 00433 0xC7, 0xD3, 0x29, 0xEE, 0xB6, 0xDD, 0x26, 0x54, 00434 0x5E, 0x96, 0xE5, 0x5B, 0x87, 0x4B, 0xE9, 0x09 }, 00435 { 0xE7, 0x18, 0x48, 0x3D, 0x0C, 0xE7, 0x69, 0x64, 00436 0x4E, 0x2E, 0x42, 0xC7, 0xBC, 0x15, 0xB4, 0x63, 00437 0x8E, 0x1F, 0x98, 0xB1, 0x3B, 0x20, 0x44, 0x28, 00438 0x56, 0x32, 0xA8, 0x03, 0xAF, 0xA9, 0x73, 0xEB, 00439 0xDE, 0x0F, 0xF2, 0x44, 0x87, 0x7E, 0xA6, 0x0A, 00440 0x4C, 0xB0, 0x43, 0x2C, 0xE5, 0x77, 0xC3, 0x1B, 00441 0xEB, 0x00, 0x9C, 0x5C, 0x2C, 0x49, 0xAA, 0x2E, 00442 0x4E, 0xAD, 0xB2, 0x17, 0xAD, 0x8C, 0xC0, 0x9B } 00443 }; 00444 00445 /* 00446 * Checkup routine 00447 */ 00448 int mbedtls_sha512_self_test( int verbose ) 00449 { 00450 int i, j, k, buflen, ret = 0; 00451 unsigned char *buf; 00452 unsigned char sha512sum[64]; 00453 mbedtls_sha512_context ctx; 00454 00455 buf = mbedtls_calloc( 1024, sizeof(unsigned char) ); 00456 if( NULL == buf ) 00457 { 00458 if( verbose != 0 ) 00459 mbedtls_printf( "Buffer allocation failed\n" ); 00460 00461 return( 1 ); 00462 } 00463 00464 mbedtls_sha512_init( &ctx ); 00465 00466 for( i = 0; i < 6; i++ ) 00467 { 00468 j = i % 3; 00469 k = i < 3; 00470 00471 if( verbose != 0 ) 00472 mbedtls_printf( " SHA-%d test #%d: ", 512 - k * 128, j + 1 ); 00473 00474 mbedtls_sha512_starts( &ctx, k ); 00475 00476 if( j == 2 ) 00477 { 00478 memset( buf, 'a', buflen = 1000 ); 00479 00480 for( j = 0; j < 1000; j++ ) 00481 mbedtls_sha512_update( &ctx, buf, buflen ); 00482 } 00483 else 00484 mbedtls_sha512_update( &ctx, sha512_test_buf[j], 00485 sha512_test_buflen[j] ); 00486 00487 mbedtls_sha512_finish( &ctx, sha512sum ); 00488 00489 if( memcmp( sha512sum, sha512_test_sum[i], 64 - k * 16 ) != 0 ) 00490 { 00491 if( verbose != 0 ) 00492 mbedtls_printf( "failed\n" ); 00493 00494 ret = 1; 00495 goto exit; 00496 } 00497 00498 if( verbose != 0 ) 00499 mbedtls_printf( "passed\n" ); 00500 } 00501 00502 if( verbose != 0 ) 00503 mbedtls_printf( "\n" ); 00504 00505 exit: 00506 mbedtls_sha512_free( &ctx ); 00507 mbedtls_free( buf ); 00508 00509 return( ret ); 00510 } 00511 00512 #endif /* MBEDTLS_SELF_TEST */ 00513 00514 #endif /* MBEDTLS_SHA512_C */
Generated on Sun Jul 17 2022 08:25:30 by
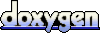