
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
rsa.c
00001 /* 00002 * The RSA public-key cryptosystem 00003 * 00004 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00005 * SPDX-License-Identifier: Apache-2.0 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00008 * not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00015 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 * 00019 * This file is part of mbed TLS (https://tls.mbed.org) 00020 */ 00021 /* 00022 * The following sources were referenced in the design of this implementation 00023 * of the RSA algorithm: 00024 * 00025 * [1] A method for obtaining digital signatures and public-key cryptosystems 00026 * R Rivest, A Shamir, and L Adleman 00027 * http://people.csail.mit.edu/rivest/pubs.html#RSA78 00028 * 00029 * [2] Handbook of Applied Cryptography - 1997, Chapter 8 00030 * Menezes, van Oorschot and Vanstone 00031 * 00032 * [3] Malware Guard Extension: Using SGX to Conceal Cache Attacks 00033 * Michael Schwarz, Samuel Weiser, Daniel Gruss, Clémentine Maurice and 00034 * Stefan Mangard 00035 * https://arxiv.org/abs/1702.08719v2 00036 * 00037 */ 00038 00039 #if !defined(MBEDTLS_CONFIG_FILE) 00040 #include "mbedtls/config.h" 00041 #else 00042 #include MBEDTLS_CONFIG_FILE 00043 #endif 00044 00045 #if defined(MBEDTLS_RSA_C) 00046 00047 #include "mbedtls/rsa.h" 00048 #include "mbedtls/oid.h" 00049 00050 #include <string.h> 00051 00052 #if defined(MBEDTLS_PKCS1_V21) 00053 #include "mbedtls/md.h" 00054 #endif 00055 00056 #if defined(MBEDTLS_PKCS1_V15) && !defined(__OpenBSD__) 00057 #include <stdlib.h> 00058 #endif 00059 00060 #if defined(MBEDTLS_PLATFORM_C) 00061 #include "mbedtls/platform.h" 00062 #else 00063 #include <stdio.h> 00064 #define mbedtls_printf printf 00065 #define mbedtls_calloc calloc 00066 #define mbedtls_free free 00067 #endif 00068 00069 /* Implementation that should never be optimized out by the compiler */ 00070 static void mbedtls_zeroize( void *v, size_t n ) { 00071 volatile unsigned char *p = (unsigned char*)v; while( n-- ) *p++ = 0; 00072 } 00073 00074 /* 00075 * Initialize an RSA context 00076 */ 00077 void mbedtls_rsa_init( mbedtls_rsa_context *ctx, 00078 int padding, 00079 int hash_id ) 00080 { 00081 memset( ctx, 0, sizeof( mbedtls_rsa_context ) ); 00082 00083 mbedtls_rsa_set_padding( ctx, padding, hash_id ); 00084 00085 #if defined(MBEDTLS_THREADING_C) 00086 mbedtls_mutex_init( &ctx->mutex ); 00087 #endif 00088 } 00089 00090 /* 00091 * Set padding for an existing RSA context 00092 */ 00093 void mbedtls_rsa_set_padding( mbedtls_rsa_context *ctx, int padding, int hash_id ) 00094 { 00095 ctx->padding = padding; 00096 ctx->hash_id = hash_id; 00097 } 00098 00099 #if defined(MBEDTLS_GENPRIME) 00100 00101 /* 00102 * Generate an RSA keypair 00103 */ 00104 int mbedtls_rsa_gen_key( mbedtls_rsa_context *ctx, 00105 int (*f_rng)(void *, unsigned char *, size_t), 00106 void *p_rng, 00107 unsigned int nbits, int exponent ) 00108 { 00109 int ret; 00110 mbedtls_mpi P1, Q1, H, G; 00111 00112 if( f_rng == NULL || nbits < 128 || exponent < 3 ) 00113 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00114 00115 if( nbits % 2 ) 00116 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00117 00118 mbedtls_mpi_init( &P1 ); mbedtls_mpi_init( &Q1 ); 00119 mbedtls_mpi_init( &H ); mbedtls_mpi_init( &G ); 00120 00121 /* 00122 * find primes P and Q with Q < P so that: 00123 * GCD( E, (P-1)*(Q-1) ) == 1 00124 */ 00125 MBEDTLS_MPI_CHK( mbedtls_mpi_lset( &ctx->E , exponent ) ); 00126 00127 do 00128 { 00129 MBEDTLS_MPI_CHK( mbedtls_mpi_gen_prime( &ctx->P , nbits >> 1, 0, 00130 f_rng, p_rng ) ); 00131 00132 MBEDTLS_MPI_CHK( mbedtls_mpi_gen_prime( &ctx->Q , nbits >> 1, 0, 00133 f_rng, p_rng ) ); 00134 00135 if( mbedtls_mpi_cmp_mpi( &ctx->P , &ctx->Q ) == 0 ) 00136 continue; 00137 00138 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &ctx->N , &ctx->P , &ctx->Q ) ); 00139 if( mbedtls_mpi_bitlen( &ctx->N ) != nbits ) 00140 continue; 00141 00142 if( mbedtls_mpi_cmp_mpi( &ctx->P , &ctx->Q ) < 0 ) 00143 mbedtls_mpi_swap( &ctx->P , &ctx->Q ); 00144 00145 MBEDTLS_MPI_CHK( mbedtls_mpi_sub_int( &P1, &ctx->P , 1 ) ); 00146 MBEDTLS_MPI_CHK( mbedtls_mpi_sub_int( &Q1, &ctx->Q , 1 ) ); 00147 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &H, &P1, &Q1 ) ); 00148 MBEDTLS_MPI_CHK( mbedtls_mpi_gcd( &G, &ctx->E , &H ) ); 00149 } 00150 while( mbedtls_mpi_cmp_int( &G, 1 ) != 0 ); 00151 00152 /* 00153 * D = E^-1 mod ((P-1)*(Q-1)) 00154 * DP = D mod (P - 1) 00155 * DQ = D mod (Q - 1) 00156 * QP = Q^-1 mod P 00157 */ 00158 MBEDTLS_MPI_CHK( mbedtls_mpi_inv_mod( &ctx->D , &ctx->E , &H ) ); 00159 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &ctx->DP , &ctx->D , &P1 ) ); 00160 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &ctx->DQ , &ctx->D , &Q1 ) ); 00161 MBEDTLS_MPI_CHK( mbedtls_mpi_inv_mod( &ctx->QP , &ctx->Q , &ctx->P ) ); 00162 00163 ctx->len = ( mbedtls_mpi_bitlen( &ctx->N ) + 7 ) >> 3; 00164 00165 cleanup: 00166 00167 mbedtls_mpi_free( &P1 ); mbedtls_mpi_free( &Q1 ); mbedtls_mpi_free( &H ); mbedtls_mpi_free( &G ); 00168 00169 if( ret != 0 ) 00170 { 00171 mbedtls_rsa_free( ctx ); 00172 return( MBEDTLS_ERR_RSA_KEY_GEN_FAILED + ret ); 00173 } 00174 00175 return( 0 ); 00176 } 00177 00178 #endif /* MBEDTLS_GENPRIME */ 00179 00180 /* 00181 * Check a public RSA key 00182 */ 00183 int mbedtls_rsa_check_pubkey( const mbedtls_rsa_context *ctx ) 00184 { 00185 if( !ctx->N .p || !ctx->E .p ) 00186 return( MBEDTLS_ERR_RSA_KEY_CHECK_FAILED ); 00187 00188 if( ( ctx->N .p [0] & 1 ) == 0 || 00189 ( ctx->E .p [0] & 1 ) == 0 ) 00190 return( MBEDTLS_ERR_RSA_KEY_CHECK_FAILED ); 00191 00192 if( mbedtls_mpi_bitlen( &ctx->N ) < 128 || 00193 mbedtls_mpi_bitlen( &ctx->N ) > MBEDTLS_MPI_MAX_BITS ) 00194 return( MBEDTLS_ERR_RSA_KEY_CHECK_FAILED ); 00195 00196 if( mbedtls_mpi_bitlen( &ctx->E ) < 2 || 00197 mbedtls_mpi_cmp_mpi( &ctx->E , &ctx->N ) >= 0 ) 00198 return( MBEDTLS_ERR_RSA_KEY_CHECK_FAILED ); 00199 00200 return( 0 ); 00201 } 00202 00203 /* 00204 * Check a private RSA key 00205 */ 00206 int mbedtls_rsa_check_privkey( const mbedtls_rsa_context *ctx ) 00207 { 00208 int ret; 00209 mbedtls_mpi PQ, DE, P1, Q1, H, I, G, G2, L1, L2, DP, DQ, QP; 00210 00211 if( ( ret = mbedtls_rsa_check_pubkey( ctx ) ) != 0 ) 00212 return( ret ); 00213 00214 if( !ctx->P .p || !ctx->Q .p || !ctx->D .p ) 00215 return( MBEDTLS_ERR_RSA_KEY_CHECK_FAILED ); 00216 00217 mbedtls_mpi_init( &PQ ); mbedtls_mpi_init( &DE ); mbedtls_mpi_init( &P1 ); mbedtls_mpi_init( &Q1 ); 00218 mbedtls_mpi_init( &H ); mbedtls_mpi_init( &I ); mbedtls_mpi_init( &G ); mbedtls_mpi_init( &G2 ); 00219 mbedtls_mpi_init( &L1 ); mbedtls_mpi_init( &L2 ); mbedtls_mpi_init( &DP ); mbedtls_mpi_init( &DQ ); 00220 mbedtls_mpi_init( &QP ); 00221 00222 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &PQ, &ctx->P , &ctx->Q ) ); 00223 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &DE, &ctx->D , &ctx->E ) ); 00224 MBEDTLS_MPI_CHK( mbedtls_mpi_sub_int( &P1, &ctx->P , 1 ) ); 00225 MBEDTLS_MPI_CHK( mbedtls_mpi_sub_int( &Q1, &ctx->Q , 1 ) ); 00226 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &H, &P1, &Q1 ) ); 00227 MBEDTLS_MPI_CHK( mbedtls_mpi_gcd( &G, &ctx->E , &H ) ); 00228 00229 MBEDTLS_MPI_CHK( mbedtls_mpi_gcd( &G2, &P1, &Q1 ) ); 00230 MBEDTLS_MPI_CHK( mbedtls_mpi_div_mpi( &L1, &L2, &H, &G2 ) ); 00231 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &I, &DE, &L1 ) ); 00232 00233 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &DP, &ctx->D , &P1 ) ); 00234 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &DQ, &ctx->D , &Q1 ) ); 00235 MBEDTLS_MPI_CHK( mbedtls_mpi_inv_mod( &QP, &ctx->Q , &ctx->P ) ); 00236 /* 00237 * Check for a valid PKCS1v2 private key 00238 */ 00239 if( mbedtls_mpi_cmp_mpi( &PQ, &ctx->N ) != 0 || 00240 mbedtls_mpi_cmp_mpi( &DP, &ctx->DP ) != 0 || 00241 mbedtls_mpi_cmp_mpi( &DQ, &ctx->DQ ) != 0 || 00242 mbedtls_mpi_cmp_mpi( &QP, &ctx->QP ) != 0 || 00243 mbedtls_mpi_cmp_int( &L2, 0 ) != 0 || 00244 mbedtls_mpi_cmp_int( &I, 1 ) != 0 || 00245 mbedtls_mpi_cmp_int( &G, 1 ) != 0 ) 00246 { 00247 ret = MBEDTLS_ERR_RSA_KEY_CHECK_FAILED; 00248 } 00249 00250 cleanup: 00251 mbedtls_mpi_free( &PQ ); mbedtls_mpi_free( &DE ); mbedtls_mpi_free( &P1 ); mbedtls_mpi_free( &Q1 ); 00252 mbedtls_mpi_free( &H ); mbedtls_mpi_free( &I ); mbedtls_mpi_free( &G ); mbedtls_mpi_free( &G2 ); 00253 mbedtls_mpi_free( &L1 ); mbedtls_mpi_free( &L2 ); mbedtls_mpi_free( &DP ); mbedtls_mpi_free( &DQ ); 00254 mbedtls_mpi_free( &QP ); 00255 00256 if( ret == MBEDTLS_ERR_RSA_KEY_CHECK_FAILED ) 00257 return( ret ); 00258 00259 if( ret != 0 ) 00260 return( MBEDTLS_ERR_RSA_KEY_CHECK_FAILED + ret ); 00261 00262 return( 0 ); 00263 } 00264 00265 /* 00266 * Check if contexts holding a public and private key match 00267 */ 00268 int mbedtls_rsa_check_pub_priv( const mbedtls_rsa_context *pub, const mbedtls_rsa_context *prv ) 00269 { 00270 if( mbedtls_rsa_check_pubkey( pub ) != 0 || 00271 mbedtls_rsa_check_privkey( prv ) != 0 ) 00272 { 00273 return( MBEDTLS_ERR_RSA_KEY_CHECK_FAILED ); 00274 } 00275 00276 if( mbedtls_mpi_cmp_mpi( &pub->N , &prv->N ) != 0 || 00277 mbedtls_mpi_cmp_mpi( &pub->E , &prv->E ) != 0 ) 00278 { 00279 return( MBEDTLS_ERR_RSA_KEY_CHECK_FAILED ); 00280 } 00281 00282 return( 0 ); 00283 } 00284 00285 /* 00286 * Do an RSA public key operation 00287 */ 00288 int mbedtls_rsa_public( mbedtls_rsa_context *ctx, 00289 const unsigned char *input, 00290 unsigned char *output ) 00291 { 00292 int ret; 00293 size_t olen; 00294 mbedtls_mpi T; 00295 00296 mbedtls_mpi_init( &T ); 00297 00298 #if defined(MBEDTLS_THREADING_C) 00299 if( ( ret = mbedtls_mutex_lock( &ctx->mutex ) ) != 0 ) 00300 return( ret ); 00301 #endif 00302 00303 MBEDTLS_MPI_CHK( mbedtls_mpi_read_binary( &T, input, ctx->len ) ); 00304 00305 if( mbedtls_mpi_cmp_mpi( &T, &ctx->N ) >= 0 ) 00306 { 00307 ret = MBEDTLS_ERR_MPI_BAD_INPUT_DATA; 00308 goto cleanup; 00309 } 00310 00311 olen = ctx->len ; 00312 MBEDTLS_MPI_CHK( mbedtls_mpi_exp_mod( &T, &T, &ctx->E , &ctx->N , &ctx->RN ) ); 00313 MBEDTLS_MPI_CHK( mbedtls_mpi_write_binary( &T, output, olen ) ); 00314 00315 cleanup: 00316 #if defined(MBEDTLS_THREADING_C) 00317 if( mbedtls_mutex_unlock( &ctx->mutex ) != 0 ) 00318 return( MBEDTLS_ERR_THREADING_MUTEX_ERROR ); 00319 #endif 00320 00321 mbedtls_mpi_free( &T ); 00322 00323 if( ret != 0 ) 00324 return( MBEDTLS_ERR_RSA_PUBLIC_FAILED + ret ); 00325 00326 return( 0 ); 00327 } 00328 00329 /* 00330 * Generate or update blinding values, see section 10 of: 00331 * KOCHER, Paul C. Timing attacks on implementations of Diffie-Hellman, RSA, 00332 * DSS, and other systems. In : Advances in Cryptology-CRYPTO'96. Springer 00333 * Berlin Heidelberg, 1996. p. 104-113. 00334 */ 00335 static int rsa_prepare_blinding( mbedtls_rsa_context *ctx, 00336 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ) 00337 { 00338 int ret, count = 0; 00339 00340 if( ctx->Vf .p != NULL ) 00341 { 00342 /* We already have blinding values, just update them by squaring */ 00343 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &ctx->Vi , &ctx->Vi , &ctx->Vi ) ); 00344 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &ctx->Vi , &ctx->Vi , &ctx->N ) ); 00345 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &ctx->Vf , &ctx->Vf , &ctx->Vf ) ); 00346 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &ctx->Vf , &ctx->Vf , &ctx->N ) ); 00347 00348 goto cleanup; 00349 } 00350 00351 /* Unblinding value: Vf = random number, invertible mod N */ 00352 do { 00353 if( count++ > 10 ) 00354 return( MBEDTLS_ERR_RSA_RNG_FAILED ); 00355 00356 MBEDTLS_MPI_CHK( mbedtls_mpi_fill_random( &ctx->Vf , ctx->len - 1, f_rng, p_rng ) ); 00357 MBEDTLS_MPI_CHK( mbedtls_mpi_gcd( &ctx->Vi , &ctx->Vf , &ctx->N ) ); 00358 } while( mbedtls_mpi_cmp_int( &ctx->Vi , 1 ) != 0 ); 00359 00360 /* Blinding value: Vi = Vf^(-e) mod N */ 00361 MBEDTLS_MPI_CHK( mbedtls_mpi_inv_mod( &ctx->Vi , &ctx->Vf , &ctx->N ) ); 00362 MBEDTLS_MPI_CHK( mbedtls_mpi_exp_mod( &ctx->Vi , &ctx->Vi , &ctx->E , &ctx->N , &ctx->RN ) ); 00363 00364 00365 cleanup: 00366 return( ret ); 00367 } 00368 00369 /* 00370 * Exponent blinding supposed to prevent side-channel attacks using multiple 00371 * traces of measurements to recover the RSA key. The more collisions are there, 00372 * the more bits of the key can be recovered. See [3]. 00373 * 00374 * Collecting n collisions with m bit long blinding value requires 2^(m-m/n) 00375 * observations on avarage. 00376 * 00377 * For example with 28 byte blinding to achieve 2 collisions the adversary has 00378 * to make 2^112 observations on avarage. 00379 * 00380 * (With the currently (as of 2017 April) known best algorithms breaking 2048 00381 * bit RSA requires approximately as much time as trying out 2^112 random keys. 00382 * Thus in this sense with 28 byte blinding the security is not reduced by 00383 * side-channel attacks like the one in [3]) 00384 * 00385 * This countermeasure does not help if the key recovery is possible with a 00386 * single trace. 00387 */ 00388 #define RSA_EXPONENT_BLINDING 28 00389 00390 /* 00391 * Do an RSA private key operation 00392 */ 00393 int mbedtls_rsa_private( mbedtls_rsa_context *ctx, 00394 int (*f_rng)(void *, unsigned char *, size_t), 00395 void *p_rng, 00396 const unsigned char *input, 00397 unsigned char *output ) 00398 { 00399 int ret; 00400 size_t olen; 00401 mbedtls_mpi T, T1, T2; 00402 mbedtls_mpi P1, Q1, R; 00403 #if defined(MBEDTLS_RSA_NO_CRT) 00404 mbedtls_mpi D_blind; 00405 mbedtls_mpi *D = &ctx->D ; 00406 #else 00407 mbedtls_mpi DP_blind, DQ_blind; 00408 mbedtls_mpi *DP = &ctx->DP ; 00409 mbedtls_mpi *DQ = &ctx->DQ ; 00410 #endif 00411 00412 /* Make sure we have private key info, prevent possible misuse */ 00413 if( ctx->P .p == NULL || ctx->Q .p == NULL || ctx->D .p == NULL ) 00414 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00415 00416 mbedtls_mpi_init( &T ); mbedtls_mpi_init( &T1 ); mbedtls_mpi_init( &T2 ); 00417 mbedtls_mpi_init( &P1 ); mbedtls_mpi_init( &Q1 ); mbedtls_mpi_init( &R ); 00418 00419 00420 if( f_rng != NULL ) 00421 { 00422 #if defined(MBEDTLS_RSA_NO_CRT) 00423 mbedtls_mpi_init( &D_blind ); 00424 #else 00425 mbedtls_mpi_init( &DP_blind ); 00426 mbedtls_mpi_init( &DQ_blind ); 00427 #endif 00428 } 00429 00430 00431 #if defined(MBEDTLS_THREADING_C) 00432 if( ( ret = mbedtls_mutex_lock( &ctx->mutex ) ) != 0 ) 00433 return( ret ); 00434 #endif 00435 00436 MBEDTLS_MPI_CHK( mbedtls_mpi_read_binary( &T, input, ctx->len ) ); 00437 if( mbedtls_mpi_cmp_mpi( &T, &ctx->N ) >= 0 ) 00438 { 00439 ret = MBEDTLS_ERR_MPI_BAD_INPUT_DATA; 00440 goto cleanup; 00441 } 00442 00443 if( f_rng != NULL ) 00444 { 00445 /* 00446 * Blinding 00447 * T = T * Vi mod N 00448 */ 00449 MBEDTLS_MPI_CHK( rsa_prepare_blinding( ctx, f_rng, p_rng ) ); 00450 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &T, &T, &ctx->Vi ) ); 00451 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &T, &T, &ctx->N ) ); 00452 00453 /* 00454 * Exponent blinding 00455 */ 00456 MBEDTLS_MPI_CHK( mbedtls_mpi_sub_int( &P1, &ctx->P , 1 ) ); 00457 MBEDTLS_MPI_CHK( mbedtls_mpi_sub_int( &Q1, &ctx->Q , 1 ) ); 00458 00459 #if defined(MBEDTLS_RSA_NO_CRT) 00460 /* 00461 * D_blind = ( P - 1 ) * ( Q - 1 ) * R + D 00462 */ 00463 MBEDTLS_MPI_CHK( mbedtls_mpi_fill_random( &R, RSA_EXPONENT_BLINDING, 00464 f_rng, p_rng ) ); 00465 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &D_blind, &P1, &Q1 ) ); 00466 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &D_blind, &D_blind, &R ) ); 00467 MBEDTLS_MPI_CHK( mbedtls_mpi_add_mpi( &D_blind, &D_blind, &ctx->D ) ); 00468 00469 D = &D_blind; 00470 #else 00471 /* 00472 * DP_blind = ( P - 1 ) * R + DP 00473 */ 00474 MBEDTLS_MPI_CHK( mbedtls_mpi_fill_random( &R, RSA_EXPONENT_BLINDING, 00475 f_rng, p_rng ) ); 00476 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &DP_blind, &P1, &R ) ); 00477 MBEDTLS_MPI_CHK( mbedtls_mpi_add_mpi( &DP_blind, &DP_blind, 00478 &ctx->DP ) ); 00479 00480 DP = &DP_blind; 00481 00482 /* 00483 * DQ_blind = ( Q - 1 ) * R + DQ 00484 */ 00485 MBEDTLS_MPI_CHK( mbedtls_mpi_fill_random( &R, RSA_EXPONENT_BLINDING, 00486 f_rng, p_rng ) ); 00487 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &DQ_blind, &Q1, &R ) ); 00488 MBEDTLS_MPI_CHK( mbedtls_mpi_add_mpi( &DQ_blind, &DQ_blind, 00489 &ctx->DQ ) ); 00490 00491 DQ = &DQ_blind; 00492 #endif /* MBEDTLS_RSA_NO_CRT */ 00493 } 00494 00495 #if defined(MBEDTLS_RSA_NO_CRT) 00496 MBEDTLS_MPI_CHK( mbedtls_mpi_exp_mod( &T, &T, D, &ctx->N , &ctx->RN ) ); 00497 #else 00498 /* 00499 * Faster decryption using the CRT 00500 * 00501 * T1 = input ^ dP mod P 00502 * T2 = input ^ dQ mod Q 00503 */ 00504 MBEDTLS_MPI_CHK( mbedtls_mpi_exp_mod( &T1, &T, DP, &ctx->P , &ctx->RP ) ); 00505 MBEDTLS_MPI_CHK( mbedtls_mpi_exp_mod( &T2, &T, DQ, &ctx->Q , &ctx->RQ ) ); 00506 00507 /* 00508 * T = (T1 - T2) * (Q^-1 mod P) mod P 00509 */ 00510 MBEDTLS_MPI_CHK( mbedtls_mpi_sub_mpi( &T, &T1, &T2 ) ); 00511 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &T1, &T, &ctx->QP ) ); 00512 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &T, &T1, &ctx->P ) ); 00513 00514 /* 00515 * T = T2 + T * Q 00516 */ 00517 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &T1, &T, &ctx->Q ) ); 00518 MBEDTLS_MPI_CHK( mbedtls_mpi_add_mpi( &T, &T2, &T1 ) ); 00519 #endif /* MBEDTLS_RSA_NO_CRT */ 00520 00521 if( f_rng != NULL ) 00522 { 00523 /* 00524 * Unblind 00525 * T = T * Vf mod N 00526 */ 00527 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &T, &T, &ctx->Vf ) ); 00528 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &T, &T, &ctx->N ) ); 00529 } 00530 00531 olen = ctx->len ; 00532 MBEDTLS_MPI_CHK( mbedtls_mpi_write_binary( &T, output, olen ) ); 00533 00534 cleanup: 00535 #if defined(MBEDTLS_THREADING_C) 00536 if( mbedtls_mutex_unlock( &ctx->mutex ) != 0 ) 00537 return( MBEDTLS_ERR_THREADING_MUTEX_ERROR ); 00538 #endif 00539 00540 mbedtls_mpi_free( &T ); mbedtls_mpi_free( &T1 ); mbedtls_mpi_free( &T2 ); 00541 mbedtls_mpi_free( &P1 ); mbedtls_mpi_free( &Q1 ); mbedtls_mpi_free( &R ); 00542 00543 if( f_rng != NULL ) 00544 { 00545 #if defined(MBEDTLS_RSA_NO_CRT) 00546 mbedtls_mpi_free( &D_blind ); 00547 #else 00548 mbedtls_mpi_free( &DP_blind ); 00549 mbedtls_mpi_free( &DQ_blind ); 00550 #endif 00551 } 00552 00553 if( ret != 0 ) 00554 return( MBEDTLS_ERR_RSA_PRIVATE_FAILED + ret ); 00555 00556 return( 0 ); 00557 } 00558 00559 #if defined(MBEDTLS_PKCS1_V21) 00560 /** 00561 * Generate and apply the MGF1 operation (from PKCS#1 v2.1) to a buffer. 00562 * 00563 * \param dst buffer to mask 00564 * \param dlen length of destination buffer 00565 * \param src source of the mask generation 00566 * \param slen length of the source buffer 00567 * \param md_ctx message digest context to use 00568 */ 00569 static void mgf_mask( unsigned char *dst, size_t dlen, unsigned char *src, 00570 size_t slen, mbedtls_md_context_t *md_ctx ) 00571 { 00572 unsigned char mask[MBEDTLS_MD_MAX_SIZE]; 00573 unsigned char counter[4]; 00574 unsigned char *p; 00575 unsigned int hlen; 00576 size_t i, use_len; 00577 00578 memset( mask, 0, MBEDTLS_MD_MAX_SIZE ); 00579 memset( counter, 0, 4 ); 00580 00581 hlen = mbedtls_md_get_size( md_ctx->md_info ); 00582 00583 /* Generate and apply dbMask */ 00584 p = dst; 00585 00586 while( dlen > 0 ) 00587 { 00588 use_len = hlen; 00589 if( dlen < hlen ) 00590 use_len = dlen; 00591 00592 mbedtls_md_starts( md_ctx ); 00593 mbedtls_md_update( md_ctx, src, slen ); 00594 mbedtls_md_update( md_ctx, counter, 4 ); 00595 mbedtls_md_finish( md_ctx, mask ); 00596 00597 for( i = 0; i < use_len; ++i ) 00598 *p++ ^= mask[i]; 00599 00600 counter[3]++; 00601 00602 dlen -= use_len; 00603 } 00604 00605 mbedtls_zeroize( mask, sizeof( mask ) ); 00606 } 00607 #endif /* MBEDTLS_PKCS1_V21 */ 00608 00609 #if defined(MBEDTLS_PKCS1_V21) 00610 /* 00611 * Implementation of the PKCS#1 v2.1 RSAES-OAEP-ENCRYPT function 00612 */ 00613 int mbedtls_rsa_rsaes_oaep_encrypt( mbedtls_rsa_context *ctx, 00614 int (*f_rng)(void *, unsigned char *, size_t), 00615 void *p_rng, 00616 int mode, 00617 const unsigned char *label, size_t label_len, 00618 size_t ilen, 00619 const unsigned char *input, 00620 unsigned char *output ) 00621 { 00622 size_t olen; 00623 int ret; 00624 unsigned char *p = output; 00625 unsigned int hlen; 00626 const mbedtls_md_info_t *md_info; 00627 mbedtls_md_context_t md_ctx; 00628 00629 if( mode == MBEDTLS_RSA_PRIVATE && ctx->padding != MBEDTLS_RSA_PKCS_V21 ) 00630 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00631 00632 if( f_rng == NULL ) 00633 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00634 00635 md_info = mbedtls_md_info_from_type( (mbedtls_md_type_t) ctx->hash_id ); 00636 if( md_info == NULL ) 00637 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00638 00639 olen = ctx->len ; 00640 hlen = mbedtls_md_get_size( md_info ); 00641 00642 /* first comparison checks for overflow */ 00643 if( ilen + 2 * hlen + 2 < ilen || olen < ilen + 2 * hlen + 2 ) 00644 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00645 00646 memset( output, 0, olen ); 00647 00648 *p++ = 0; 00649 00650 /* Generate a random octet string seed */ 00651 if( ( ret = f_rng( p_rng, p, hlen ) ) != 0 ) 00652 return( MBEDTLS_ERR_RSA_RNG_FAILED + ret ); 00653 00654 p += hlen; 00655 00656 /* Construct DB */ 00657 mbedtls_md( md_info, label, label_len, p ); 00658 p += hlen; 00659 p += olen - 2 * hlen - 2 - ilen; 00660 *p++ = 1; 00661 memcpy( p, input, ilen ); 00662 00663 mbedtls_md_init( &md_ctx ); 00664 if( ( ret = mbedtls_md_setup( &md_ctx, md_info, 0 ) ) != 0 ) 00665 { 00666 mbedtls_md_free( &md_ctx ); 00667 return( ret ); 00668 } 00669 00670 /* maskedDB: Apply dbMask to DB */ 00671 mgf_mask( output + hlen + 1, olen - hlen - 1, output + 1, hlen, 00672 &md_ctx ); 00673 00674 /* maskedSeed: Apply seedMask to seed */ 00675 mgf_mask( output + 1, hlen, output + hlen + 1, olen - hlen - 1, 00676 &md_ctx ); 00677 00678 mbedtls_md_free( &md_ctx ); 00679 00680 return( ( mode == MBEDTLS_RSA_PUBLIC ) 00681 ? mbedtls_rsa_public( ctx, output, output ) 00682 : mbedtls_rsa_private( ctx, f_rng, p_rng, output, output ) ); 00683 } 00684 #endif /* MBEDTLS_PKCS1_V21 */ 00685 00686 #if defined(MBEDTLS_PKCS1_V15) 00687 /* 00688 * Implementation of the PKCS#1 v2.1 RSAES-PKCS1-V1_5-ENCRYPT function 00689 */ 00690 int mbedtls_rsa_rsaes_pkcs1_v15_encrypt( mbedtls_rsa_context *ctx, 00691 int (*f_rng)(void *, unsigned char *, size_t), 00692 void *p_rng, 00693 int mode, size_t ilen, 00694 const unsigned char *input, 00695 unsigned char *output ) 00696 { 00697 size_t nb_pad, olen; 00698 int ret; 00699 unsigned char *p = output; 00700 00701 if( mode == MBEDTLS_RSA_PRIVATE && ctx->padding != MBEDTLS_RSA_PKCS_V15 ) 00702 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00703 00704 // We don't check p_rng because it won't be dereferenced here 00705 if( f_rng == NULL || input == NULL || output == NULL ) 00706 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00707 00708 olen = ctx->len ; 00709 00710 /* first comparison checks for overflow */ 00711 if( ilen + 11 < ilen || olen < ilen + 11 ) 00712 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00713 00714 nb_pad = olen - 3 - ilen; 00715 00716 *p++ = 0; 00717 if( mode == MBEDTLS_RSA_PUBLIC ) 00718 { 00719 *p++ = MBEDTLS_RSA_CRYPT; 00720 00721 while( nb_pad-- > 0 ) 00722 { 00723 int rng_dl = 100; 00724 00725 do { 00726 ret = f_rng( p_rng, p, 1 ); 00727 } while( *p == 0 && --rng_dl && ret == 0 ); 00728 00729 /* Check if RNG failed to generate data */ 00730 if( rng_dl == 0 || ret != 0 ) 00731 return( MBEDTLS_ERR_RSA_RNG_FAILED + ret ); 00732 00733 p++; 00734 } 00735 } 00736 else 00737 { 00738 *p++ = MBEDTLS_RSA_SIGN; 00739 00740 while( nb_pad-- > 0 ) 00741 *p++ = 0xFF; 00742 } 00743 00744 *p++ = 0; 00745 memcpy( p, input, ilen ); 00746 00747 return( ( mode == MBEDTLS_RSA_PUBLIC ) 00748 ? mbedtls_rsa_public( ctx, output, output ) 00749 : mbedtls_rsa_private( ctx, f_rng, p_rng, output, output ) ); 00750 } 00751 #endif /* MBEDTLS_PKCS1_V15 */ 00752 00753 /* 00754 * Add the message padding, then do an RSA operation 00755 */ 00756 int mbedtls_rsa_pkcs1_encrypt( mbedtls_rsa_context *ctx, 00757 int (*f_rng)(void *, unsigned char *, size_t), 00758 void *p_rng, 00759 int mode, size_t ilen, 00760 const unsigned char *input, 00761 unsigned char *output ) 00762 { 00763 switch( ctx->padding ) 00764 { 00765 #if defined(MBEDTLS_PKCS1_V15) 00766 case MBEDTLS_RSA_PKCS_V15: 00767 return mbedtls_rsa_rsaes_pkcs1_v15_encrypt( ctx, f_rng, p_rng, mode, ilen, 00768 input, output ); 00769 #endif 00770 00771 #if defined(MBEDTLS_PKCS1_V21) 00772 case MBEDTLS_RSA_PKCS_V21: 00773 return mbedtls_rsa_rsaes_oaep_encrypt( ctx, f_rng, p_rng, mode, NULL, 0, 00774 ilen, input, output ); 00775 #endif 00776 00777 default: 00778 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 00779 } 00780 } 00781 00782 #if defined(MBEDTLS_PKCS1_V21) 00783 /* 00784 * Implementation of the PKCS#1 v2.1 RSAES-OAEP-DECRYPT function 00785 */ 00786 int mbedtls_rsa_rsaes_oaep_decrypt( mbedtls_rsa_context *ctx, 00787 int (*f_rng)(void *, unsigned char *, size_t), 00788 void *p_rng, 00789 int mode, 00790 const unsigned char *label, size_t label_len, 00791 size_t *olen, 00792 const unsigned char *input, 00793 unsigned char *output, 00794 size_t output_max_len ) 00795 { 00796 int ret; 00797 size_t ilen, i, pad_len; 00798 unsigned char *p, bad, pad_done; 00799 unsigned char buf[MBEDTLS_MPI_MAX_SIZE]; 00800 unsigned char lhash[MBEDTLS_MD_MAX_SIZE]; 00801 unsigned int hlen; 00802 const mbedtls_md_info_t *md_info; 00803 mbedtls_md_context_t md_ctx; 00804 00805 /* 00806 * Parameters sanity checks 00807 */ 00808 if( mode == MBEDTLS_RSA_PRIVATE && ctx->padding != MBEDTLS_RSA_PKCS_V21 ) 00809 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00810 00811 ilen = ctx->len ; 00812 00813 if( ilen < 16 || ilen > sizeof( buf ) ) 00814 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00815 00816 md_info = mbedtls_md_info_from_type( (mbedtls_md_type_t) ctx->hash_id ); 00817 if( md_info == NULL ) 00818 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00819 00820 hlen = mbedtls_md_get_size( md_info ); 00821 00822 // checking for integer underflow 00823 if( 2 * hlen + 2 > ilen ) 00824 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00825 00826 /* 00827 * RSA operation 00828 */ 00829 ret = ( mode == MBEDTLS_RSA_PUBLIC ) 00830 ? mbedtls_rsa_public( ctx, input, buf ) 00831 : mbedtls_rsa_private( ctx, f_rng, p_rng, input, buf ); 00832 00833 if( ret != 0 ) 00834 goto cleanup; 00835 00836 /* 00837 * Unmask data and generate lHash 00838 */ 00839 mbedtls_md_init( &md_ctx ); 00840 if( ( ret = mbedtls_md_setup( &md_ctx, md_info, 0 ) ) != 0 ) 00841 { 00842 mbedtls_md_free( &md_ctx ); 00843 goto cleanup; 00844 } 00845 00846 00847 /* Generate lHash */ 00848 mbedtls_md( md_info, label, label_len, lhash ); 00849 00850 /* seed: Apply seedMask to maskedSeed */ 00851 mgf_mask( buf + 1, hlen, buf + hlen + 1, ilen - hlen - 1, 00852 &md_ctx ); 00853 00854 /* DB: Apply dbMask to maskedDB */ 00855 mgf_mask( buf + hlen + 1, ilen - hlen - 1, buf + 1, hlen, 00856 &md_ctx ); 00857 00858 mbedtls_md_free( &md_ctx ); 00859 00860 /* 00861 * Check contents, in "constant-time" 00862 */ 00863 p = buf; 00864 bad = 0; 00865 00866 bad |= *p++; /* First byte must be 0 */ 00867 00868 p += hlen; /* Skip seed */ 00869 00870 /* Check lHash */ 00871 for( i = 0; i < hlen; i++ ) 00872 bad |= lhash[i] ^ *p++; 00873 00874 /* Get zero-padding len, but always read till end of buffer 00875 * (minus one, for the 01 byte) */ 00876 pad_len = 0; 00877 pad_done = 0; 00878 for( i = 0; i < ilen - 2 * hlen - 2; i++ ) 00879 { 00880 pad_done |= p[i]; 00881 pad_len += ((pad_done | (unsigned char)-pad_done) >> 7) ^ 1; 00882 } 00883 00884 p += pad_len; 00885 bad |= *p++ ^ 0x01; 00886 00887 /* 00888 * The only information "leaked" is whether the padding was correct or not 00889 * (eg, no data is copied if it was not correct). This meets the 00890 * recommendations in PKCS#1 v2.2: an opponent cannot distinguish between 00891 * the different error conditions. 00892 */ 00893 if( bad != 0 ) 00894 { 00895 ret = MBEDTLS_ERR_RSA_INVALID_PADDING; 00896 goto cleanup; 00897 } 00898 00899 if( ilen - ( p - buf ) > output_max_len ) 00900 { 00901 ret = MBEDTLS_ERR_RSA_OUTPUT_TOO_LARGE; 00902 goto cleanup; 00903 } 00904 00905 *olen = ilen - (p - buf); 00906 memcpy( output, p, *olen ); 00907 ret = 0; 00908 00909 cleanup: 00910 mbedtls_zeroize( buf, sizeof( buf ) ); 00911 mbedtls_zeroize( lhash, sizeof( lhash ) ); 00912 00913 return( ret ); 00914 } 00915 #endif /* MBEDTLS_PKCS1_V21 */ 00916 00917 #if defined(MBEDTLS_PKCS1_V15) 00918 /* 00919 * Implementation of the PKCS#1 v2.1 RSAES-PKCS1-V1_5-DECRYPT function 00920 */ 00921 int mbedtls_rsa_rsaes_pkcs1_v15_decrypt( mbedtls_rsa_context *ctx, 00922 int (*f_rng)(void *, unsigned char *, size_t), 00923 void *p_rng, 00924 int mode, size_t *olen, 00925 const unsigned char *input, 00926 unsigned char *output, 00927 size_t output_max_len) 00928 { 00929 int ret; 00930 size_t ilen, pad_count = 0, i; 00931 unsigned char *p, bad, pad_done = 0; 00932 unsigned char buf[MBEDTLS_MPI_MAX_SIZE]; 00933 00934 if( mode == MBEDTLS_RSA_PRIVATE && ctx->padding != MBEDTLS_RSA_PKCS_V15 ) 00935 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00936 00937 ilen = ctx->len ; 00938 00939 if( ilen < 16 || ilen > sizeof( buf ) ) 00940 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00941 00942 ret = ( mode == MBEDTLS_RSA_PUBLIC ) 00943 ? mbedtls_rsa_public( ctx, input, buf ) 00944 : mbedtls_rsa_private( ctx, f_rng, p_rng, input, buf ); 00945 00946 if( ret != 0 ) 00947 goto cleanup; 00948 00949 p = buf; 00950 bad = 0; 00951 00952 /* 00953 * Check and get padding len in "constant-time" 00954 */ 00955 bad |= *p++; /* First byte must be 0 */ 00956 00957 /* This test does not depend on secret data */ 00958 if( mode == MBEDTLS_RSA_PRIVATE ) 00959 { 00960 bad |= *p++ ^ MBEDTLS_RSA_CRYPT; 00961 00962 /* Get padding len, but always read till end of buffer 00963 * (minus one, for the 00 byte) */ 00964 for( i = 0; i < ilen - 3; i++ ) 00965 { 00966 pad_done |= ((p[i] | (unsigned char)-p[i]) >> 7) ^ 1; 00967 pad_count += ((pad_done | (unsigned char)-pad_done) >> 7) ^ 1; 00968 } 00969 00970 p += pad_count; 00971 bad |= *p++; /* Must be zero */ 00972 } 00973 else 00974 { 00975 bad |= *p++ ^ MBEDTLS_RSA_SIGN; 00976 00977 /* Get padding len, but always read till end of buffer 00978 * (minus one, for the 00 byte) */ 00979 for( i = 0; i < ilen - 3; i++ ) 00980 { 00981 pad_done |= ( p[i] != 0xFF ); 00982 pad_count += ( pad_done == 0 ); 00983 } 00984 00985 p += pad_count; 00986 bad |= *p++; /* Must be zero */ 00987 } 00988 00989 bad |= ( pad_count < 8 ); 00990 00991 if( bad ) 00992 { 00993 ret = MBEDTLS_ERR_RSA_INVALID_PADDING; 00994 goto cleanup; 00995 } 00996 00997 if( ilen - ( p - buf ) > output_max_len ) 00998 { 00999 ret = MBEDTLS_ERR_RSA_OUTPUT_TOO_LARGE; 01000 goto cleanup; 01001 } 01002 01003 *olen = ilen - (p - buf); 01004 memcpy( output, p, *olen ); 01005 ret = 0; 01006 01007 cleanup: 01008 mbedtls_zeroize( buf, sizeof( buf ) ); 01009 01010 return( ret ); 01011 } 01012 #endif /* MBEDTLS_PKCS1_V15 */ 01013 01014 /* 01015 * Do an RSA operation, then remove the message padding 01016 */ 01017 int mbedtls_rsa_pkcs1_decrypt( mbedtls_rsa_context *ctx, 01018 int (*f_rng)(void *, unsigned char *, size_t), 01019 void *p_rng, 01020 int mode, size_t *olen, 01021 const unsigned char *input, 01022 unsigned char *output, 01023 size_t output_max_len) 01024 { 01025 switch( ctx->padding ) 01026 { 01027 #if defined(MBEDTLS_PKCS1_V15) 01028 case MBEDTLS_RSA_PKCS_V15: 01029 return mbedtls_rsa_rsaes_pkcs1_v15_decrypt( ctx, f_rng, p_rng, mode, olen, 01030 input, output, output_max_len ); 01031 #endif 01032 01033 #if defined(MBEDTLS_PKCS1_V21) 01034 case MBEDTLS_RSA_PKCS_V21: 01035 return mbedtls_rsa_rsaes_oaep_decrypt( ctx, f_rng, p_rng, mode, NULL, 0, 01036 olen, input, output, 01037 output_max_len ); 01038 #endif 01039 01040 default: 01041 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 01042 } 01043 } 01044 01045 #if defined(MBEDTLS_PKCS1_V21) 01046 /* 01047 * Implementation of the PKCS#1 v2.1 RSASSA-PSS-SIGN function 01048 */ 01049 int mbedtls_rsa_rsassa_pss_sign( mbedtls_rsa_context *ctx, 01050 int (*f_rng)(void *, unsigned char *, size_t), 01051 void *p_rng, 01052 int mode, 01053 mbedtls_md_type_t md_alg, 01054 unsigned int hashlen, 01055 const unsigned char *hash, 01056 unsigned char *sig ) 01057 { 01058 size_t olen; 01059 unsigned char *p = sig; 01060 unsigned char salt[MBEDTLS_MD_MAX_SIZE]; 01061 unsigned int slen, hlen, offset = 0; 01062 int ret; 01063 size_t msb; 01064 const mbedtls_md_info_t *md_info; 01065 mbedtls_md_context_t md_ctx; 01066 01067 if( mode == MBEDTLS_RSA_PRIVATE && ctx->padding != MBEDTLS_RSA_PKCS_V21 ) 01068 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01069 01070 if( f_rng == NULL ) 01071 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01072 01073 olen = ctx->len ; 01074 01075 if( md_alg != MBEDTLS_MD_NONE ) 01076 { 01077 /* Gather length of hash to sign */ 01078 md_info = mbedtls_md_info_from_type( md_alg ); 01079 if( md_info == NULL ) 01080 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01081 01082 hashlen = mbedtls_md_get_size( md_info ); 01083 } 01084 01085 md_info = mbedtls_md_info_from_type( (mbedtls_md_type_t) ctx->hash_id ); 01086 if( md_info == NULL ) 01087 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01088 01089 hlen = mbedtls_md_get_size( md_info ); 01090 slen = hlen; 01091 01092 if( olen < hlen + slen + 2 ) 01093 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01094 01095 memset( sig, 0, olen ); 01096 01097 /* Generate salt of length slen */ 01098 if( ( ret = f_rng( p_rng, salt, slen ) ) != 0 ) 01099 return( MBEDTLS_ERR_RSA_RNG_FAILED + ret ); 01100 01101 /* Note: EMSA-PSS encoding is over the length of N - 1 bits */ 01102 msb = mbedtls_mpi_bitlen( &ctx->N ) - 1; 01103 p += olen - hlen * 2 - 2; 01104 *p++ = 0x01; 01105 memcpy( p, salt, slen ); 01106 p += slen; 01107 01108 mbedtls_md_init( &md_ctx ); 01109 if( ( ret = mbedtls_md_setup( &md_ctx, md_info, 0 ) ) != 0 ) 01110 { 01111 mbedtls_md_free( &md_ctx ); 01112 /* No need to zeroize salt: we didn't use it. */ 01113 return( ret ); 01114 } 01115 01116 /* Generate H = Hash( M' ) */ 01117 mbedtls_md_starts( &md_ctx ); 01118 mbedtls_md_update( &md_ctx, p, 8 ); 01119 mbedtls_md_update( &md_ctx, hash, hashlen ); 01120 mbedtls_md_update( &md_ctx, salt, slen ); 01121 mbedtls_md_finish( &md_ctx, p ); 01122 mbedtls_zeroize( salt, sizeof( salt ) ); 01123 01124 /* Compensate for boundary condition when applying mask */ 01125 if( msb % 8 == 0 ) 01126 offset = 1; 01127 01128 /* maskedDB: Apply dbMask to DB */ 01129 mgf_mask( sig + offset, olen - hlen - 1 - offset, p, hlen, &md_ctx ); 01130 01131 mbedtls_md_free( &md_ctx ); 01132 01133 msb = mbedtls_mpi_bitlen( &ctx->N ) - 1; 01134 sig[0] &= 0xFF >> ( olen * 8 - msb ); 01135 01136 p += hlen; 01137 *p++ = 0xBC; 01138 01139 return( ( mode == MBEDTLS_RSA_PUBLIC ) 01140 ? mbedtls_rsa_public( ctx, sig, sig ) 01141 : mbedtls_rsa_private( ctx, f_rng, p_rng, sig, sig ) ); 01142 } 01143 #endif /* MBEDTLS_PKCS1_V21 */ 01144 01145 #if defined(MBEDTLS_PKCS1_V15) 01146 /* 01147 * Implementation of the PKCS#1 v2.1 RSASSA-PKCS1-V1_5-SIGN function 01148 */ 01149 /* 01150 * Do an RSA operation to sign the message digest 01151 */ 01152 int mbedtls_rsa_rsassa_pkcs1_v15_sign( mbedtls_rsa_context *ctx, 01153 int (*f_rng)(void *, unsigned char *, size_t), 01154 void *p_rng, 01155 int mode, 01156 mbedtls_md_type_t md_alg, 01157 unsigned int hashlen, 01158 const unsigned char *hash, 01159 unsigned char *sig ) 01160 { 01161 size_t nb_pad, olen, oid_size = 0; 01162 unsigned char *p = sig; 01163 const char *oid = NULL; 01164 unsigned char *sig_try = NULL, *verif = NULL; 01165 size_t i; 01166 unsigned char diff; 01167 volatile unsigned char diff_no_optimize; 01168 int ret; 01169 01170 if( mode == MBEDTLS_RSA_PRIVATE && ctx->padding != MBEDTLS_RSA_PKCS_V15 ) 01171 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01172 01173 olen = ctx->len ; 01174 nb_pad = olen - 3; 01175 01176 if( md_alg != MBEDTLS_MD_NONE ) 01177 { 01178 const mbedtls_md_info_t *md_info = mbedtls_md_info_from_type( md_alg ); 01179 if( md_info == NULL ) 01180 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01181 01182 if( mbedtls_oid_get_oid_by_md( md_alg, &oid, &oid_size ) != 0 ) 01183 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01184 01185 nb_pad -= 10 + oid_size; 01186 01187 hashlen = mbedtls_md_get_size( md_info ); 01188 } 01189 01190 nb_pad -= hashlen; 01191 01192 if( ( nb_pad < 8 ) || ( nb_pad > olen ) ) 01193 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01194 01195 *p++ = 0; 01196 *p++ = MBEDTLS_RSA_SIGN; 01197 memset( p, 0xFF, nb_pad ); 01198 p += nb_pad; 01199 *p++ = 0; 01200 01201 if( md_alg == MBEDTLS_MD_NONE ) 01202 { 01203 memcpy( p, hash, hashlen ); 01204 } 01205 else 01206 { 01207 /* 01208 * DigestInfo ::= SEQUENCE { 01209 * digestAlgorithm DigestAlgorithmIdentifier, 01210 * digest Digest } 01211 * 01212 * DigestAlgorithmIdentifier ::= AlgorithmIdentifier 01213 * 01214 * Digest ::= OCTET STRING 01215 */ 01216 *p++ = MBEDTLS_ASN1_SEQUENCE | MBEDTLS_ASN1_CONSTRUCTED; 01217 *p++ = (unsigned char) ( 0x08 + oid_size + hashlen ); 01218 *p++ = MBEDTLS_ASN1_SEQUENCE | MBEDTLS_ASN1_CONSTRUCTED; 01219 *p++ = (unsigned char) ( 0x04 + oid_size ); 01220 *p++ = MBEDTLS_ASN1_OID; 01221 *p++ = oid_size & 0xFF; 01222 memcpy( p, oid, oid_size ); 01223 p += oid_size; 01224 *p++ = MBEDTLS_ASN1_NULL; 01225 *p++ = 0x00; 01226 *p++ = MBEDTLS_ASN1_OCTET_STRING; 01227 *p++ = hashlen; 01228 memcpy( p, hash, hashlen ); 01229 } 01230 01231 if( mode == MBEDTLS_RSA_PUBLIC ) 01232 return( mbedtls_rsa_public( ctx, sig, sig ) ); 01233 01234 /* 01235 * In order to prevent Lenstra's attack, make the signature in a 01236 * temporary buffer and check it before returning it. 01237 */ 01238 sig_try = mbedtls_calloc( 1, ctx->len ); 01239 if( sig_try == NULL ) 01240 return( MBEDTLS_ERR_MPI_ALLOC_FAILED ); 01241 01242 verif = mbedtls_calloc( 1, ctx->len ); 01243 if( verif == NULL ) 01244 { 01245 mbedtls_free( sig_try ); 01246 return( MBEDTLS_ERR_MPI_ALLOC_FAILED ); 01247 } 01248 01249 MBEDTLS_MPI_CHK( mbedtls_rsa_private( ctx, f_rng, p_rng, sig, sig_try ) ); 01250 MBEDTLS_MPI_CHK( mbedtls_rsa_public( ctx, sig_try, verif ) ); 01251 01252 /* Compare in constant time just in case */ 01253 for( diff = 0, i = 0; i < ctx->len ; i++ ) 01254 diff |= verif[i] ^ sig[i]; 01255 diff_no_optimize = diff; 01256 01257 if( diff_no_optimize != 0 ) 01258 { 01259 ret = MBEDTLS_ERR_RSA_PRIVATE_FAILED; 01260 goto cleanup; 01261 } 01262 01263 memcpy( sig, sig_try, ctx->len ); 01264 01265 cleanup: 01266 mbedtls_free( sig_try ); 01267 mbedtls_free( verif ); 01268 01269 return( ret ); 01270 } 01271 #endif /* MBEDTLS_PKCS1_V15 */ 01272 01273 /* 01274 * Do an RSA operation to sign the message digest 01275 */ 01276 int mbedtls_rsa_pkcs1_sign( mbedtls_rsa_context *ctx, 01277 int (*f_rng)(void *, unsigned char *, size_t), 01278 void *p_rng, 01279 int mode, 01280 mbedtls_md_type_t md_alg, 01281 unsigned int hashlen, 01282 const unsigned char *hash, 01283 unsigned char *sig ) 01284 { 01285 switch( ctx->padding ) 01286 { 01287 #if defined(MBEDTLS_PKCS1_V15) 01288 case MBEDTLS_RSA_PKCS_V15: 01289 return mbedtls_rsa_rsassa_pkcs1_v15_sign( ctx, f_rng, p_rng, mode, md_alg, 01290 hashlen, hash, sig ); 01291 #endif 01292 01293 #if defined(MBEDTLS_PKCS1_V21) 01294 case MBEDTLS_RSA_PKCS_V21: 01295 return mbedtls_rsa_rsassa_pss_sign( ctx, f_rng, p_rng, mode, md_alg, 01296 hashlen, hash, sig ); 01297 #endif 01298 01299 default: 01300 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 01301 } 01302 } 01303 01304 #if defined(MBEDTLS_PKCS1_V21) 01305 /* 01306 * Implementation of the PKCS#1 v2.1 RSASSA-PSS-VERIFY function 01307 */ 01308 int mbedtls_rsa_rsassa_pss_verify_ext( mbedtls_rsa_context *ctx, 01309 int (*f_rng)(void *, unsigned char *, size_t), 01310 void *p_rng, 01311 int mode, 01312 mbedtls_md_type_t md_alg, 01313 unsigned int hashlen, 01314 const unsigned char *hash, 01315 mbedtls_md_type_t mgf1_hash_id, 01316 int expected_salt_len, 01317 const unsigned char *sig ) 01318 { 01319 int ret; 01320 size_t siglen; 01321 unsigned char *p; 01322 unsigned char result[MBEDTLS_MD_MAX_SIZE]; 01323 unsigned char zeros[8]; 01324 unsigned int hlen; 01325 size_t slen, msb; 01326 const mbedtls_md_info_t *md_info; 01327 mbedtls_md_context_t md_ctx; 01328 unsigned char buf[MBEDTLS_MPI_MAX_SIZE]; 01329 01330 if( mode == MBEDTLS_RSA_PRIVATE && ctx->padding != MBEDTLS_RSA_PKCS_V21 ) 01331 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01332 01333 siglen = ctx->len ; 01334 01335 if( siglen < 16 || siglen > sizeof( buf ) ) 01336 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01337 01338 ret = ( mode == MBEDTLS_RSA_PUBLIC ) 01339 ? mbedtls_rsa_public( ctx, sig, buf ) 01340 : mbedtls_rsa_private( ctx, f_rng, p_rng, sig, buf ); 01341 01342 if( ret != 0 ) 01343 return( ret ); 01344 01345 p = buf; 01346 01347 if( buf[siglen - 1] != 0xBC ) 01348 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 01349 01350 if( md_alg != MBEDTLS_MD_NONE ) 01351 { 01352 /* Gather length of hash to sign */ 01353 md_info = mbedtls_md_info_from_type( md_alg ); 01354 if( md_info == NULL ) 01355 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01356 01357 hashlen = mbedtls_md_get_size( md_info ); 01358 } 01359 01360 md_info = mbedtls_md_info_from_type( mgf1_hash_id ); 01361 if( md_info == NULL ) 01362 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01363 01364 hlen = mbedtls_md_get_size( md_info ); 01365 slen = siglen - hlen - 1; /* Currently length of salt + padding */ 01366 01367 memset( zeros, 0, 8 ); 01368 01369 /* 01370 * Note: EMSA-PSS verification is over the length of N - 1 bits 01371 */ 01372 msb = mbedtls_mpi_bitlen( &ctx->N ) - 1; 01373 01374 /* Compensate for boundary condition when applying mask */ 01375 if( msb % 8 == 0 ) 01376 { 01377 p++; 01378 siglen -= 1; 01379 } 01380 if( buf[0] >> ( 8 - siglen * 8 + msb ) ) 01381 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01382 01383 mbedtls_md_init( &md_ctx ); 01384 if( ( ret = mbedtls_md_setup( &md_ctx, md_info, 0 ) ) != 0 ) 01385 { 01386 mbedtls_md_free( &md_ctx ); 01387 return( ret ); 01388 } 01389 01390 mgf_mask( p, siglen - hlen - 1, p + siglen - hlen - 1, hlen, &md_ctx ); 01391 01392 buf[0] &= 0xFF >> ( siglen * 8 - msb ); 01393 01394 while( p < buf + siglen && *p == 0 ) 01395 p++; 01396 01397 if( p == buf + siglen || 01398 *p++ != 0x01 ) 01399 { 01400 mbedtls_md_free( &md_ctx ); 01401 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 01402 } 01403 01404 /* Actual salt len */ 01405 slen -= p - buf; 01406 01407 if( expected_salt_len != MBEDTLS_RSA_SALT_LEN_ANY && 01408 slen != (size_t) expected_salt_len ) 01409 { 01410 mbedtls_md_free( &md_ctx ); 01411 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 01412 } 01413 01414 /* 01415 * Generate H = Hash( M' ) 01416 */ 01417 mbedtls_md_starts( &md_ctx ); 01418 mbedtls_md_update( &md_ctx, zeros, 8 ); 01419 mbedtls_md_update( &md_ctx, hash, hashlen ); 01420 mbedtls_md_update( &md_ctx, p, slen ); 01421 mbedtls_md_finish( &md_ctx, result ); 01422 01423 mbedtls_md_free( &md_ctx ); 01424 01425 if( memcmp( p + slen, result, hlen ) == 0 ) 01426 return( 0 ); 01427 else 01428 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01429 } 01430 01431 /* 01432 * Simplified PKCS#1 v2.1 RSASSA-PSS-VERIFY function 01433 */ 01434 int mbedtls_rsa_rsassa_pss_verify( mbedtls_rsa_context *ctx, 01435 int (*f_rng)(void *, unsigned char *, size_t), 01436 void *p_rng, 01437 int mode, 01438 mbedtls_md_type_t md_alg, 01439 unsigned int hashlen, 01440 const unsigned char *hash, 01441 const unsigned char *sig ) 01442 { 01443 mbedtls_md_type_t mgf1_hash_id = ( ctx->hash_id != MBEDTLS_MD_NONE ) 01444 ? (mbedtls_md_type_t) ctx->hash_id 01445 : md_alg; 01446 01447 return( mbedtls_rsa_rsassa_pss_verify_ext( ctx, f_rng, p_rng, mode, 01448 md_alg, hashlen, hash, 01449 mgf1_hash_id, MBEDTLS_RSA_SALT_LEN_ANY, 01450 sig ) ); 01451 01452 } 01453 #endif /* MBEDTLS_PKCS1_V21 */ 01454 01455 #if defined(MBEDTLS_PKCS1_V15) 01456 /* 01457 * Implementation of the PKCS#1 v2.1 RSASSA-PKCS1-v1_5-VERIFY function 01458 */ 01459 int mbedtls_rsa_rsassa_pkcs1_v15_verify( mbedtls_rsa_context *ctx, 01460 int (*f_rng)(void *, unsigned char *, size_t), 01461 void *p_rng, 01462 int mode, 01463 mbedtls_md_type_t md_alg, 01464 unsigned int hashlen, 01465 const unsigned char *hash, 01466 const unsigned char *sig ) 01467 { 01468 int ret; 01469 size_t len, siglen, asn1_len; 01470 unsigned char *p, *p0, *end; 01471 mbedtls_md_type_t msg_md_alg; 01472 const mbedtls_md_info_t *md_info; 01473 mbedtls_asn1_buf oid; 01474 unsigned char buf[MBEDTLS_MPI_MAX_SIZE]; 01475 01476 if( mode == MBEDTLS_RSA_PRIVATE && ctx->padding != MBEDTLS_RSA_PKCS_V15 ) 01477 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01478 01479 siglen = ctx->len ; 01480 01481 if( siglen < 16 || siglen > sizeof( buf ) ) 01482 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01483 01484 ret = ( mode == MBEDTLS_RSA_PUBLIC ) 01485 ? mbedtls_rsa_public( ctx, sig, buf ) 01486 : mbedtls_rsa_private( ctx, f_rng, p_rng, sig, buf ); 01487 01488 if( ret != 0 ) 01489 return( ret ); 01490 01491 p = buf; 01492 01493 if( *p++ != 0 || *p++ != MBEDTLS_RSA_SIGN ) 01494 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 01495 01496 while( *p != 0 ) 01497 { 01498 if( p >= buf + siglen - 1 || *p != 0xFF ) 01499 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 01500 p++; 01501 } 01502 p++; /* skip 00 byte */ 01503 01504 /* We've read: 00 01 PS 00 where PS must be at least 8 bytes */ 01505 if( p - buf < 11 ) 01506 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 01507 01508 len = siglen - ( p - buf ); 01509 01510 if( len == hashlen && md_alg == MBEDTLS_MD_NONE ) 01511 { 01512 if( memcmp( p, hash, hashlen ) == 0 ) 01513 return( 0 ); 01514 else 01515 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01516 } 01517 01518 md_info = mbedtls_md_info_from_type( md_alg ); 01519 if( md_info == NULL ) 01520 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01521 hashlen = mbedtls_md_get_size( md_info ); 01522 01523 end = p + len; 01524 01525 /* 01526 * Parse the ASN.1 structure inside the PKCS#1 v1.5 structure. 01527 * Insist on 2-byte length tags, to protect against variants of 01528 * Bleichenbacher's forgery attack against lax PKCS#1v1.5 verification. 01529 */ 01530 p0 = p; 01531 if( ( ret = mbedtls_asn1_get_tag( &p, end, &asn1_len, 01532 MBEDTLS_ASN1_CONSTRUCTED | MBEDTLS_ASN1_SEQUENCE ) ) != 0 ) 01533 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01534 if( p != p0 + 2 || asn1_len + 2 != len ) 01535 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01536 01537 p0 = p; 01538 if( ( ret = mbedtls_asn1_get_tag( &p, end, &asn1_len, 01539 MBEDTLS_ASN1_CONSTRUCTED | MBEDTLS_ASN1_SEQUENCE ) ) != 0 ) 01540 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01541 if( p != p0 + 2 || asn1_len + 6 + hashlen != len ) 01542 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01543 01544 p0 = p; 01545 if( ( ret = mbedtls_asn1_get_tag( &p, end, &oid.len, MBEDTLS_ASN1_OID ) ) != 0 ) 01546 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01547 if( p != p0 + 2 ) 01548 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01549 01550 oid.p = p; 01551 p += oid.len; 01552 01553 if( mbedtls_oid_get_md_alg( &oid, &msg_md_alg ) != 0 ) 01554 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01555 01556 if( md_alg != msg_md_alg ) 01557 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01558 01559 /* 01560 * assume the algorithm parameters must be NULL 01561 */ 01562 p0 = p; 01563 if( ( ret = mbedtls_asn1_get_tag( &p, end, &asn1_len, MBEDTLS_ASN1_NULL ) ) != 0 ) 01564 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01565 if( p != p0 + 2 ) 01566 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01567 01568 p0 = p; 01569 if( ( ret = mbedtls_asn1_get_tag( &p, end, &asn1_len, MBEDTLS_ASN1_OCTET_STRING ) ) != 0 ) 01570 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01571 if( p != p0 + 2 || asn1_len != hashlen ) 01572 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01573 01574 if( memcmp( p, hash, hashlen ) != 0 ) 01575 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01576 01577 p += hashlen; 01578 01579 if( p != end ) 01580 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01581 01582 return( 0 ); 01583 } 01584 #endif /* MBEDTLS_PKCS1_V15 */ 01585 01586 /* 01587 * Do an RSA operation and check the message digest 01588 */ 01589 int mbedtls_rsa_pkcs1_verify( mbedtls_rsa_context *ctx, 01590 int (*f_rng)(void *, unsigned char *, size_t), 01591 void *p_rng, 01592 int mode, 01593 mbedtls_md_type_t md_alg, 01594 unsigned int hashlen, 01595 const unsigned char *hash, 01596 const unsigned char *sig ) 01597 { 01598 switch( ctx->padding ) 01599 { 01600 #if defined(MBEDTLS_PKCS1_V15) 01601 case MBEDTLS_RSA_PKCS_V15: 01602 return mbedtls_rsa_rsassa_pkcs1_v15_verify( ctx, f_rng, p_rng, mode, md_alg, 01603 hashlen, hash, sig ); 01604 #endif 01605 01606 #if defined(MBEDTLS_PKCS1_V21) 01607 case MBEDTLS_RSA_PKCS_V21: 01608 return mbedtls_rsa_rsassa_pss_verify( ctx, f_rng, p_rng, mode, md_alg, 01609 hashlen, hash, sig ); 01610 #endif 01611 01612 default: 01613 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 01614 } 01615 } 01616 01617 /* 01618 * Copy the components of an RSA key 01619 */ 01620 int mbedtls_rsa_copy( mbedtls_rsa_context *dst, const mbedtls_rsa_context *src ) 01621 { 01622 int ret; 01623 01624 dst->ver = src->ver ; 01625 dst->len = src->len ; 01626 01627 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->N , &src->N ) ); 01628 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->E , &src->E ) ); 01629 01630 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->D , &src->D ) ); 01631 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->P , &src->P ) ); 01632 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->Q , &src->Q ) ); 01633 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->DP , &src->DP ) ); 01634 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->DQ , &src->DQ ) ); 01635 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->QP , &src->QP ) ); 01636 01637 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->RN , &src->RN ) ); 01638 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->RP , &src->RP ) ); 01639 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->RQ , &src->RQ ) ); 01640 01641 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->Vi , &src->Vi ) ); 01642 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->Vf , &src->Vf ) ); 01643 01644 dst->padding = src->padding ; 01645 dst->hash_id = src->hash_id ; 01646 01647 cleanup: 01648 if( ret != 0 ) 01649 mbedtls_rsa_free( dst ); 01650 01651 return( ret ); 01652 } 01653 01654 /* 01655 * Free the components of an RSA key 01656 */ 01657 void mbedtls_rsa_free( mbedtls_rsa_context *ctx ) 01658 { 01659 mbedtls_mpi_free( &ctx->Vi ); mbedtls_mpi_free( &ctx->Vf ); 01660 mbedtls_mpi_free( &ctx->RQ ); mbedtls_mpi_free( &ctx->RP ); mbedtls_mpi_free( &ctx->RN ); 01661 mbedtls_mpi_free( &ctx->QP ); mbedtls_mpi_free( &ctx->DQ ); mbedtls_mpi_free( &ctx->DP ); 01662 mbedtls_mpi_free( &ctx->Q ); mbedtls_mpi_free( &ctx->P ); mbedtls_mpi_free( &ctx->D ); 01663 mbedtls_mpi_free( &ctx->E ); mbedtls_mpi_free( &ctx->N ); 01664 01665 #if defined(MBEDTLS_THREADING_C) 01666 mbedtls_mutex_free( &ctx->mutex ); 01667 #endif 01668 } 01669 01670 #if defined(MBEDTLS_SELF_TEST) 01671 01672 #include "mbedtls/sha1.h" 01673 01674 /* 01675 * Example RSA-1024 keypair, for test purposes 01676 */ 01677 #define KEY_LEN 128 01678 01679 #define RSA_N "9292758453063D803DD603D5E777D788" \ 01680 "8ED1D5BF35786190FA2F23EBC0848AEA" \ 01681 "DDA92CA6C3D80B32C4D109BE0F36D6AE" \ 01682 "7130B9CED7ACDF54CFC7555AC14EEBAB" \ 01683 "93A89813FBF3C4F8066D2D800F7C38A8" \ 01684 "1AE31942917403FF4946B0A83D3D3E05" \ 01685 "EE57C6F5F5606FB5D4BC6CD34EE0801A" \ 01686 "5E94BB77B07507233A0BC7BAC8F90F79" 01687 01688 #define RSA_E "10001" 01689 01690 #define RSA_D "24BF6185468786FDD303083D25E64EFC" \ 01691 "66CA472BC44D253102F8B4A9D3BFA750" \ 01692 "91386C0077937FE33FA3252D28855837" \ 01693 "AE1B484A8A9A45F7EE8C0C634F99E8CD" \ 01694 "DF79C5CE07EE72C7F123142198164234" \ 01695 "CABB724CF78B8173B9F880FC86322407" \ 01696 "AF1FEDFDDE2BEB674CA15F3E81A1521E" \ 01697 "071513A1E85B5DFA031F21ECAE91A34D" 01698 01699 #define RSA_P "C36D0EB7FCD285223CFB5AABA5BDA3D8" \ 01700 "2C01CAD19EA484A87EA4377637E75500" \ 01701 "FCB2005C5C7DD6EC4AC023CDA285D796" \ 01702 "C3D9E75E1EFC42488BB4F1D13AC30A57" 01703 01704 #define RSA_Q "C000DF51A7C77AE8D7C7370C1FF55B69" \ 01705 "E211C2B9E5DB1ED0BF61D0D9899620F4" \ 01706 "910E4168387E3C30AA1E00C339A79508" \ 01707 "8452DD96A9A5EA5D9DCA68DA636032AF" 01708 01709 #define RSA_DP "C1ACF567564274FB07A0BBAD5D26E298" \ 01710 "3C94D22288ACD763FD8E5600ED4A702D" \ 01711 "F84198A5F06C2E72236AE490C93F07F8" \ 01712 "3CC559CD27BC2D1CA488811730BB5725" 01713 01714 #define RSA_DQ "4959CBF6F8FEF750AEE6977C155579C7" \ 01715 "D8AAEA56749EA28623272E4F7D0592AF" \ 01716 "7C1F1313CAC9471B5C523BFE592F517B" \ 01717 "407A1BD76C164B93DA2D32A383E58357" 01718 01719 #define RSA_QP "9AE7FBC99546432DF71896FC239EADAE" \ 01720 "F38D18D2B2F0E2DD275AA977E2BF4411" \ 01721 "F5A3B2A5D33605AEBBCCBA7FEB9F2D2F" \ 01722 "A74206CEC169D74BF5A8C50D6F48EA08" 01723 01724 #define PT_LEN 24 01725 #define RSA_PT "\xAA\xBB\xCC\x03\x02\x01\x00\xFF\xFF\xFF\xFF\xFF" \ 01726 "\x11\x22\x33\x0A\x0B\x0C\xCC\xDD\xDD\xDD\xDD\xDD" 01727 01728 #if defined(MBEDTLS_PKCS1_V15) 01729 static int myrand( void *rng_state, unsigned char *output, size_t len ) 01730 { 01731 #if !defined(__OpenBSD__) 01732 size_t i; 01733 01734 if( rng_state != NULL ) 01735 rng_state = NULL; 01736 01737 for( i = 0; i < len; ++i ) 01738 output[i] = rand(); 01739 #else 01740 if( rng_state != NULL ) 01741 rng_state = NULL; 01742 01743 arc4random_buf( output, len ); 01744 #endif /* !OpenBSD */ 01745 01746 return( 0 ); 01747 } 01748 #endif /* MBEDTLS_PKCS1_V15 */ 01749 01750 /* 01751 * Checkup routine 01752 */ 01753 int mbedtls_rsa_self_test( int verbose ) 01754 { 01755 int ret = 0; 01756 #if defined(MBEDTLS_PKCS1_V15) 01757 size_t len; 01758 mbedtls_rsa_context rsa; 01759 unsigned char rsa_plaintext[PT_LEN]; 01760 unsigned char rsa_decrypted[PT_LEN]; 01761 unsigned char rsa_ciphertext[KEY_LEN]; 01762 #if defined(MBEDTLS_SHA1_C) 01763 unsigned char sha1sum[20]; 01764 #endif 01765 01766 mbedtls_rsa_init( &rsa, MBEDTLS_RSA_PKCS_V15, 0 ); 01767 01768 rsa.len = KEY_LEN; 01769 MBEDTLS_MPI_CHK( mbedtls_mpi_read_string( &rsa.N , 16, RSA_N ) ); 01770 MBEDTLS_MPI_CHK( mbedtls_mpi_read_string( &rsa.E , 16, RSA_E ) ); 01771 MBEDTLS_MPI_CHK( mbedtls_mpi_read_string( &rsa.D , 16, RSA_D ) ); 01772 MBEDTLS_MPI_CHK( mbedtls_mpi_read_string( &rsa.P , 16, RSA_P ) ); 01773 MBEDTLS_MPI_CHK( mbedtls_mpi_read_string( &rsa.Q , 16, RSA_Q ) ); 01774 MBEDTLS_MPI_CHK( mbedtls_mpi_read_string( &rsa.DP , 16, RSA_DP ) ); 01775 MBEDTLS_MPI_CHK( mbedtls_mpi_read_string( &rsa.DQ , 16, RSA_DQ ) ); 01776 MBEDTLS_MPI_CHK( mbedtls_mpi_read_string( &rsa.QP , 16, RSA_QP ) ); 01777 01778 if( verbose != 0 ) 01779 mbedtls_printf( " RSA key validation: " ); 01780 01781 if( mbedtls_rsa_check_pubkey( &rsa ) != 0 || 01782 mbedtls_rsa_check_privkey( &rsa ) != 0 ) 01783 { 01784 if( verbose != 0 ) 01785 mbedtls_printf( "failed\n" ); 01786 01787 return( 1 ); 01788 } 01789 01790 if( verbose != 0 ) 01791 mbedtls_printf( "passed\n PKCS#1 encryption : " ); 01792 01793 memcpy( rsa_plaintext, RSA_PT, PT_LEN ); 01794 01795 if( mbedtls_rsa_pkcs1_encrypt( &rsa, myrand, NULL, MBEDTLS_RSA_PUBLIC, PT_LEN, 01796 rsa_plaintext, rsa_ciphertext ) != 0 ) 01797 { 01798 if( verbose != 0 ) 01799 mbedtls_printf( "failed\n" ); 01800 01801 return( 1 ); 01802 } 01803 01804 if( verbose != 0 ) 01805 mbedtls_printf( "passed\n PKCS#1 decryption : " ); 01806 01807 if( mbedtls_rsa_pkcs1_decrypt( &rsa, myrand, NULL, MBEDTLS_RSA_PRIVATE, &len, 01808 rsa_ciphertext, rsa_decrypted, 01809 sizeof(rsa_decrypted) ) != 0 ) 01810 { 01811 if( verbose != 0 ) 01812 mbedtls_printf( "failed\n" ); 01813 01814 return( 1 ); 01815 } 01816 01817 if( memcmp( rsa_decrypted, rsa_plaintext, len ) != 0 ) 01818 { 01819 if( verbose != 0 ) 01820 mbedtls_printf( "failed\n" ); 01821 01822 return( 1 ); 01823 } 01824 01825 if( verbose != 0 ) 01826 mbedtls_printf( "passed\n" ); 01827 01828 #if defined(MBEDTLS_SHA1_C) 01829 if( verbose != 0 ) 01830 mbedtls_printf( " PKCS#1 data sign : " ); 01831 01832 mbedtls_sha1( rsa_plaintext, PT_LEN, sha1sum ); 01833 01834 if( mbedtls_rsa_pkcs1_sign( &rsa, myrand, NULL, MBEDTLS_RSA_PRIVATE, MBEDTLS_MD_SHA1, 0, 01835 sha1sum, rsa_ciphertext ) != 0 ) 01836 { 01837 if( verbose != 0 ) 01838 mbedtls_printf( "failed\n" ); 01839 01840 return( 1 ); 01841 } 01842 01843 if( verbose != 0 ) 01844 mbedtls_printf( "passed\n PKCS#1 sig. verify: " ); 01845 01846 if( mbedtls_rsa_pkcs1_verify( &rsa, NULL, NULL, MBEDTLS_RSA_PUBLIC, MBEDTLS_MD_SHA1, 0, 01847 sha1sum, rsa_ciphertext ) != 0 ) 01848 { 01849 if( verbose != 0 ) 01850 mbedtls_printf( "failed\n" ); 01851 01852 return( 1 ); 01853 } 01854 01855 if( verbose != 0 ) 01856 mbedtls_printf( "passed\n" ); 01857 #endif /* MBEDTLS_SHA1_C */ 01858 01859 if( verbose != 0 ) 01860 mbedtls_printf( "\n" ); 01861 01862 cleanup: 01863 mbedtls_rsa_free( &rsa ); 01864 #else /* MBEDTLS_PKCS1_V15 */ 01865 ((void) verbose); 01866 #endif /* MBEDTLS_PKCS1_V15 */ 01867 return( ret ); 01868 } 01869 01870 #endif /* MBEDTLS_SELF_TEST */ 01871 01872 #endif /* MBEDTLS_RSA_C */
Generated on Sun Jul 17 2022 08:25:29 by
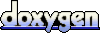