
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
rpc_gateway_exports.h
00001 /* 00002 * Copyright (c) 2016, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #ifndef __UVISOR_API_RPC_GATEWAY_EXPORTS_H__ 00018 #define __UVISOR_API_RPC_GATEWAY_EXPORTS_H__ 00019 00020 #include "api/inc/uvisor_exports.h" 00021 #include "api/inc/magic_exports.h" 00022 #include <stdint.h> 00023 00024 /* ldr pc, [pc, #<label - instr + 4>] 00025 * LDR (immediate) - ARMv7M ARM section A7.7.42 00026 * 1111;1 00 0; 0 10 1; <Rn - 1111>; <Rt - 1111>; <imm12> (Encoding T3) */ 00027 #define LDR_PC_PC_IMM_OPCODE(instr, label) \ 00028 ((uint32_t) (0xF000F8DFUL | ((((uint32_t) (label) - ((uint32_t) (instr) + 4)) & 0xFFFUL) << 16))) 00029 00030 /** RPC gateway structure 00031 * 00032 * This struct is packed because we must ensure that the `ldr_pc` field has no 00033 * padding before itself and will be located at a valid instruction location, 00034 * and that the `caller` and `target` field are at a pre-determined offset from 00035 * the `ldr_pc` field. 00036 */ 00037 typedef struct RPCGateway { 00038 uint32_t ldr_pc; 00039 uint32_t magic; 00040 uint32_t box_ptr; 00041 uint32_t target; 00042 uint32_t caller; /* This is not for use by anything other than the ldr_pc. It's like a pretend literal pool. */ 00043 } UVISOR_PACKED UVISOR_ALIGN(4) TRPCGateway; 00044 00045 #endif /* __UVISOR_API_RPC_GATEWAY_EXPORTS_H__ */
Generated on Sun Jul 17 2022 08:25:29 by
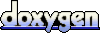