
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
register_gateway_exports.h
00001 /* 00002 * Copyright (c) 2015-2016, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #ifndef __UVISOR_API_REGISTER_GATEWAY_EXPORTS_H__ 00018 #define __UVISOR_API_REGISTER_GATEWAY_EXPORTS_H__ 00019 00020 /** Register gateway magic 00021 * 00022 * The following magic is used to verify a register gateway structure. It has 00023 * been generated starting from the ARM Thumb/Thumb-2 invalid opcode. 00024 * ARM Thumb (with the Thumb-2 extension): 0xF7FxAxxx (32 bits) 00025 * The 'x's can be freely chosen (they have been chosen randomly here). This 00026 * requires the Thumb-2 extensions because otherwise only 16 bits opcodes are 00027 * accepted. 00028 */ 00029 #if defined(__thumb__) && defined(__thumb2__) 00030 #define UVISOR_REGISTER_GATEWAY_MAGIC 0xF7F3A89E 00031 #else 00032 #error "Unsupported instruction set. The ARM Thumb-2 instruction set must be supported." 00033 #endif /* __thumb__ && __thumb2__ */ 00034 00035 /** Register gateway structure 00036 * 00037 * This struct is packed because we must ensure that the `svc_opcode` field has 00038 * no padding before itself, and that the `bxlr` field is at a pre-determined 00039 * offset from the `branch` field. 00040 */ 00041 typedef struct { 00042 uint16_t svc_opcode; 00043 uint16_t branch; 00044 uint32_t magic; 00045 uint32_t box_ptr; 00046 uint32_t address; 00047 uint32_t mask; 00048 uint16_t operation; 00049 uint16_t bxlr; 00050 } UVISOR_PACKED UVISOR_ALIGN(4) TRegisterGateway; 00051 00052 /** Register gateway operation - Masks 00053 * @internal 00054 * These are used to extract the operation fields. 00055 */ 00056 #define __UVISOR_RGW_OP_TYPE_MASK ((uint16_t) 0x00FF) 00057 #define __UVISOR_RGW_OP_TYPE_POS 0 00058 #define __UVISOR_RGW_OP_WIDTH_MASK ((uint16_t) 0x3F00) 00059 #define __UVISOR_RGW_OP_WIDTH_POS 8 00060 #define __UVISOR_RGW_OP_SHARED_MASK ((uint16_t) 0x8000) 00061 #define __UVISOR_RGW_OP_SHARED_POS 15 00062 00063 /** Register gateway operations 00064 * The user can specify the following properties: 00065 * - Access type: read/write, and-, or-, xor-, replac-ed. 00066 * - Access width: 8, 16, 32 bits. 00067 * - Access shared: Whether the gateway can be shared with other boxes. 00068 * These parameters are stored in a 16-bit value as follows: 00069 * 00070 * 15 14 13 12 11 10 9 8 7 6 5 4 3 2 1 0 00071 * | |__________________| |____________________| 00072 * | | | 00073 * shared width type 00074 * 00075 * @note The operation value must be hardcoded. 00076 */ 00077 #define UVISOR_RGW_OP(type, width, shared) \ 00078 ((uint16_t) ((((uint16_t) (type) << __UVISOR_RGW_OP_TYPE_POS) & __UVISOR_RGW_OP_TYPE_MASK) | \ 00079 (((uint16_t) (width) << __UVISOR_RGW_OP_WIDTH_POS) & __UVISOR_RGW_OP_WIDTH_MASK) | \ 00080 (((uint16_t) (shared) << __UVISOR_RGW_OP_SHARED_POS) & __UVISOR_RGW_OP_SHARED_MASK))) 00081 00082 /** Register gateway operation - Shared */ 00083 #define UVISOR_RGW_SHARED 1 00084 #define UVISOR_RGW_EXCLUSIVE 0 00085 00086 /** Register gateway operation - Type */ 00087 #define UVISOR_RGW_OP_READ 0 /**< value = *address */ 00088 #define UVISOR_RGW_OP_READ_AND 1 /**< value = *address & mask */ 00089 #define UVISOR_RGW_OP_WRITE 2 /**< *address = value */ 00090 #define UVISOR_RGW_OP_WRITE_AND 3 /**< *address &= value | mask */ 00091 #define UVISOR_RGW_OP_WRITE_OR 4 /**< *address |= value & ~mask */ 00092 #define UVISOR_RGW_OP_WRITE_XOR 5 /**< *address ^= value & mask */ 00093 #define UVISOR_RGW_OP_WRITE_REPLACE 6 /**< *address = (*address & ~mask) | (value & mask) */ 00094 00095 #endif /* __UVISOR_API_REGISTER_GATEWAY_EXPORTS_H__ */
Generated on Sun Jul 17 2022 08:25:29 by
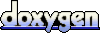