
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
read.cpp
Go to the documentation of this file.
00001 /* 00002 * mbed Microcontroller Library 00003 * Copyright (c) 2006-2016 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 /** @file read.cpp Test cases to read KVs in the CFSTORE using the drv->Read() API call. 00019 * 00020 * Please consult the documentation under the test-case functions for 00021 * a description of the individual test case. 00022 */ 00023 00024 #include "mbed.h" 00025 #include "cfstore_config.h" 00026 #include "cfstore_test.h" 00027 #include "cfstore_debug.h" 00028 #include "Driver_Common.h" 00029 #include "configuration_store.h" 00030 #include "utest/utest.h" 00031 #include "unity/unity.h" 00032 #include "greentea-client/test_env.h" 00033 #include "cfstore_utest.h" 00034 #ifdef YOTTA_CFG_CFSTORE_UVISOR 00035 #include "uvisor-lib/uvisor-lib.h" 00036 #endif /* YOTTA_CFG_CFSTORE_UVISOR */ 00037 00038 #include <stdio.h> 00039 #include <string.h> 00040 #include <inttypes.h> 00041 00042 using namespace utest::v1; 00043 00044 static char cfstore_read_utest_msg_g[CFSTORE_UTEST_MSG_BUF_SIZE]; 00045 00046 /* Configure secure box. */ 00047 #ifdef YOTTA_CFG_CFSTORE_UVISOR 00048 UVISOR_BOX_NAMESPACE("com.arm.mbed.cfstore.test.read.box1"); 00049 UVISOR_BOX_CONFIG(cfstore_read_box1, UVISOR_BOX_STACK_SIZE); 00050 #endif /* YOTTA_CFG_CFSTORE_UVISOR */ 00051 00052 00053 /* KV data for test_01 */ 00054 static cfstore_kv_data_t cfstore_read_test_01_kv_data[] = { 00055 CFSTORE_INIT_1_TABLE_MID_NODE, 00056 { NULL, NULL}, 00057 }; 00058 00059 /* report whether built/configured for flash sync or async mode */ 00060 static control_t cfstore_read_test_00(const size_t call_count) 00061 { 00062 int32_t ret = ARM_DRIVER_ERROR; 00063 00064 (void) call_count; 00065 ret = cfstore_test_startup(); 00066 CFSTORE_TEST_UTEST_MESSAGE(cfstore_read_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to perform test startup (ret=%d).\n", __func__, (int) ret); 00067 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_read_utest_msg_g); 00068 return CaseNext; 00069 } 00070 00071 00072 /* @brief check char at offset is as expected */ 00073 static int32_t cfstore_read_seek_test(ARM_CFSTORE_HANDLE hkey, uint32_t offset, const char expected) 00074 { 00075 ARM_CFSTORE_SIZE len = 1; 00076 char read_buf[1]; 00077 int32_t ret = ARM_DRIVER_ERROR; 00078 ARM_CFSTORE_DRIVER* drv = &cfstore_driver; 00079 00080 ret = drv->Rseek(hkey, offset); 00081 if(ret < ARM_DRIVER_OK){ 00082 CFSTORE_ERRLOG("%s:failed to Rseek() to desired offset (offset=%d) (ret=%d).\n", __func__, (int) offset, (int) ret); 00083 goto out0; 00084 } 00085 ret = drv->Read(hkey, read_buf, &len); 00086 if(ret < ARM_DRIVER_OK){ 00087 CFSTORE_ERRLOG("%s:failed to Read() (offset=%d)(ret=%d).\n", __func__, (int) offset, (int) ret); 00088 goto out0; 00089 } 00090 if(read_buf[0] != expected){ 00091 ret = ARM_DRIVER_ERROR; 00092 goto out0; 00093 } 00094 out0: 00095 return ret; 00096 } 00097 00098 /** @brief 00099 * 00100 * @return on success returns CaseNext to continue to next test case, otherwise will assert on errors. 00101 */ 00102 control_t cfstore_read_test_01_end (const size_t call_count) 00103 { 00104 int32_t ret = ARM_DRIVER_ERROR; 00105 ARM_CFSTORE_SIZE len = 0; 00106 ARM_CFSTORE_DRIVER* drv = &cfstore_driver; 00107 ARM_CFSTORE_KEYDESC kdesc; 00108 ARM_CFSTORE_HANDLE_INIT(hkey); 00109 cfstore_test_rw_data_entry_t *node; 00110 ARM_CFSTORE_FMODE flags; 00111 00112 CFSTORE_DBGLOG("%s:entered\n", __func__); 00113 (void) call_count; 00114 memset(&kdesc, 0, sizeof(kdesc)); 00115 memset(&flags, 0, sizeof(flags)); 00116 00117 /* create a key for reading */ 00118 kdesc.drl = ARM_RETENTION_WHILE_DEVICE_ACTIVE; 00119 len = strlen(cfstore_read_test_01_kv_data[0].value); 00120 ret = cfstore_test_create(cfstore_read_test_01_kv_data[0].key_name, (char*) cfstore_read_test_01_kv_data[0].value, &len, &kdesc); 00121 CFSTORE_TEST_UTEST_MESSAGE(cfstore_read_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to create KV in store (ret=%d).\n", __func__, (int) ret); 00122 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_read_utest_msg_g); 00123 00124 /* now open the newly created key */ 00125 ret = drv->Open(cfstore_read_test_01_kv_data[0].key_name, flags, hkey); 00126 CFSTORE_TEST_UTEST_MESSAGE(cfstore_read_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to open node (key_name=\"%s\", value=\"%s\")(ret=%d)\n", __func__, cfstore_read_test_01_kv_data[0].key_name, cfstore_read_test_01_kv_data[0].value, (int) ret); 00127 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_read_utest_msg_g); 00128 00129 /* seek back and forth in key and check the characters are as expected */ 00130 node = cfstore_test_rw_data_table; 00131 while(node->offset != CFSTORE_TEST_RW_TABLE_SENTINEL) 00132 { 00133 ret = cfstore_read_seek_test(hkey, node->offset, node->rw_char); 00134 CFSTORE_TEST_UTEST_MESSAGE(cfstore_read_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Rseek() to offset (%d) didn't read expected char (\'%c\') (ret=%d)\n", __func__, (int) node->offset, node->rw_char, (int) ret); 00135 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_read_utest_msg_g); 00136 node++; 00137 } 00138 CFSTORE_TEST_UTEST_MESSAGE(cfstore_read_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Close() call failed.\n", __func__); 00139 TEST_ASSERT_MESSAGE(drv->Close(hkey) >= ARM_DRIVER_OK, cfstore_read_utest_msg_g); 00140 00141 ret = drv->Uninitialize(); 00142 CFSTORE_TEST_UTEST_MESSAGE(cfstore_read_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Uninitialize() call failed.\n", __func__); 00143 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_read_utest_msg_g); 00144 return CaseNext; 00145 } 00146 00147 00148 /** @brief 00149 * 00150 * @return on success returns CaseNext to continue to next test case, otherwise will assert on errors. 00151 */ 00152 control_t cfstore_read_test_02_end (const size_t call_count) 00153 { 00154 (void) call_count; 00155 /*todo: implement test */ 00156 CFSTORE_TEST_UTEST_MESSAGE(cfstore_read_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Warn: Not implemented\n", __func__); 00157 CFSTORE_DBGLOG("%s: WARN: requires implementation\n", __func__); 00158 TEST_ASSERT_MESSAGE(true, cfstore_read_utest_msg_g); 00159 return CaseNext; 00160 } 00161 00162 00163 /// @cond CFSTORE_DOXYGEN_DISABLE 00164 utest::v1::status_t greentea_setup(const size_t number_of_cases) 00165 { 00166 GREENTEA_SETUP(200, "default_auto"); 00167 return greentea_test_setup_handler(number_of_cases); 00168 } 00169 00170 Case cases[] = { 00171 /* 1 2 3 4 5 6 7 */ 00172 /* 1234567890123456789012345678901234567890123456789012345678901234567890 */ 00173 Case("READ_test_00", cfstore_read_test_00), 00174 Case("READ_test_01_start", cfstore_utest_default_start), 00175 Case("READ_test_01_end", cfstore_read_test_01_end ), 00176 Case("READ_test_02_start", cfstore_utest_default_start), 00177 Case("READ_test_02_end", cfstore_read_test_02_end ), 00178 }; 00179 00180 00181 /* Declare your test specification with a custom setup handler */ 00182 Specification specification(greentea_setup, cases); 00183 00184 int main() 00185 { 00186 return !Harness::run(specification); 00187 } 00188 /// @endcond
Generated on Sun Jul 17 2022 08:25:29 by
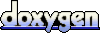