
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
dns.h
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * DNS - host name to IP address resolver. 00004 */ 00005 00006 /* 00007 * Port to lwIP from uIP 00008 * by Jim Pettinato April 2007 00009 * 00010 * security fixes and more by Simon Goldschmidt 00011 * 00012 * uIP version Copyright (c) 2002-2003, Adam Dunkels. 00013 * All rights reserved. 00014 * 00015 * Redistribution and use in source and binary forms, with or without 00016 * modification, are permitted provided that the following conditions 00017 * are met: 00018 * 1. Redistributions of source code must retain the above copyright 00019 * notice, this list of conditions and the following disclaimer. 00020 * 2. Redistributions in binary form must reproduce the above copyright 00021 * notice, this list of conditions and the following disclaimer in the 00022 * documentation and/or other materials provided with the distribution. 00023 * 3. The name of the author may not be used to endorse or promote 00024 * products derived from this software without specific prior 00025 * written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS 00028 * OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00029 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE 00030 * ARE DISCLAIMED. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY 00031 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00032 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE 00033 * GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00034 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 00035 * WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING 00036 * NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00037 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00038 */ 00039 00040 #ifndef LWIP_HDR_PROT_DNS_H 00041 #define LWIP_HDR_PROT_DNS_H 00042 00043 #include "lwip/arch.h" 00044 00045 #ifdef __cplusplus 00046 extern "C" { 00047 #endif 00048 00049 /** DNS server port address */ 00050 #ifndef DNS_SERVER_PORT 00051 #define DNS_SERVER_PORT 53 00052 #endif 00053 00054 /* DNS field TYPE used for "Resource Records" */ 00055 #define DNS_RRTYPE_A 1 /* a host address */ 00056 #define DNS_RRTYPE_NS 2 /* an authoritative name server */ 00057 #define DNS_RRTYPE_MD 3 /* a mail destination (Obsolete - use MX) */ 00058 #define DNS_RRTYPE_MF 4 /* a mail forwarder (Obsolete - use MX) */ 00059 #define DNS_RRTYPE_CNAME 5 /* the canonical name for an alias */ 00060 #define DNS_RRTYPE_SOA 6 /* marks the start of a zone of authority */ 00061 #define DNS_RRTYPE_MB 7 /* a mailbox domain name (EXPERIMENTAL) */ 00062 #define DNS_RRTYPE_MG 8 /* a mail group member (EXPERIMENTAL) */ 00063 #define DNS_RRTYPE_MR 9 /* a mail rename domain name (EXPERIMENTAL) */ 00064 #define DNS_RRTYPE_NULL 10 /* a null RR (EXPERIMENTAL) */ 00065 #define DNS_RRTYPE_WKS 11 /* a well known service description */ 00066 #define DNS_RRTYPE_PTR 12 /* a domain name pointer */ 00067 #define DNS_RRTYPE_HINFO 13 /* host information */ 00068 #define DNS_RRTYPE_MINFO 14 /* mailbox or mail list information */ 00069 #define DNS_RRTYPE_MX 15 /* mail exchange */ 00070 #define DNS_RRTYPE_TXT 16 /* text strings */ 00071 #define DNS_RRTYPE_AAAA 28 /* IPv6 address */ 00072 #define DNS_RRTYPE_SRV 33 /* service location */ 00073 #define DNS_RRTYPE_ANY 255 /* any type */ 00074 00075 /* DNS field CLASS used for "Resource Records" */ 00076 #define DNS_RRCLASS_IN 1 /* the Internet */ 00077 #define DNS_RRCLASS_CS 2 /* the CSNET class (Obsolete - used only for examples in some obsolete RFCs) */ 00078 #define DNS_RRCLASS_CH 3 /* the CHAOS class */ 00079 #define DNS_RRCLASS_HS 4 /* Hesiod [Dyer 87] */ 00080 #define DNS_RRCLASS_ANY 255 /* any class */ 00081 #define DNS_RRCLASS_FLUSH 0x800 /* Flush bit */ 00082 00083 /* DNS protocol flags */ 00084 #define DNS_FLAG1_RESPONSE 0x80 00085 #define DNS_FLAG1_OPCODE_STATUS 0x10 00086 #define DNS_FLAG1_OPCODE_INVERSE 0x08 00087 #define DNS_FLAG1_OPCODE_STANDARD 0x00 00088 #define DNS_FLAG1_AUTHORATIVE 0x04 00089 #define DNS_FLAG1_TRUNC 0x02 00090 #define DNS_FLAG1_RD 0x01 00091 #define DNS_FLAG2_RA 0x80 00092 #define DNS_FLAG2_ERR_MASK 0x0f 00093 #define DNS_FLAG2_ERR_NONE 0x00 00094 #define DNS_FLAG2_ERR_NAME 0x03 00095 00096 #define DNS_HDR_GET_OPCODE(hdr) ((((hdr)->flags1) >> 3) & 0xF) 00097 00098 #ifdef PACK_STRUCT_USE_INCLUDES 00099 # include "arch/bpstruct.h" 00100 #endif 00101 PACK_STRUCT_BEGIN 00102 /** DNS message header */ 00103 struct dns_hdr { 00104 PACK_STRUCT_FIELD(u16_t id); 00105 PACK_STRUCT_FLD_8(u8_t flags1); 00106 PACK_STRUCT_FLD_8(u8_t flags2); 00107 PACK_STRUCT_FIELD(u16_t numquestions); 00108 PACK_STRUCT_FIELD(u16_t numanswers); 00109 PACK_STRUCT_FIELD(u16_t numauthrr); 00110 PACK_STRUCT_FIELD(u16_t numextrarr); 00111 } PACK_STRUCT_STRUCT; 00112 PACK_STRUCT_END 00113 #ifdef PACK_STRUCT_USE_INCLUDES 00114 # include "arch/epstruct.h" 00115 #endif 00116 #define SIZEOF_DNS_HDR 12 00117 00118 00119 /* Multicast DNS definitions */ 00120 00121 /** UDP port for multicast DNS queries */ 00122 #ifndef DNS_MQUERY_PORT 00123 #define DNS_MQUERY_PORT 5353 00124 #endif 00125 00126 /* IPv4 group for multicast DNS queries: 224.0.0.251 */ 00127 #ifndef DNS_MQUERY_IPV4_GROUP_INIT 00128 #define DNS_MQUERY_IPV4_GROUP_INIT IPADDR4_INIT_BYTES(224,0,0,251) 00129 #endif 00130 00131 /* IPv6 group for multicast DNS queries: FF02::FB */ 00132 #ifndef DNS_MQUERY_IPV6_GROUP_INIT 00133 #define DNS_MQUERY_IPV6_GROUP_INIT IPADDR6_INIT_HOST(0xFF020000,0,0,0xFB) 00134 #endif 00135 00136 #ifdef __cplusplus 00137 } 00138 #endif 00139 00140 #endif /* LWIP_HDR_PROT_DNS_H */
Generated on Sun Jul 17 2022 08:25:22 by
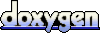