
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ppp_opts.h
00001 /* 00002 * Redistribution and use in source and binary forms, with or without modification, 00003 * are permitted provided that the following conditions are met: 00004 * 00005 * 1. Redistributions of source code must retain the above copyright notice, 00006 * this list of conditions and the following disclaimer. 00007 * 2. Redistributions in binary form must reproduce the above copyright notice, 00008 * this list of conditions and the following disclaimer in the documentation 00009 * and/or other materials provided with the distribution. 00010 * 3. The name of the author may not be used to endorse or promote products 00011 * derived from this software without specific prior written permission. 00012 * 00013 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00014 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00015 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00016 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00017 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00018 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00019 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00020 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00021 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00022 * OF SUCH DAMAGE. 00023 * 00024 * This file is part of the lwIP TCP/IP stack. 00025 * 00026 */ 00027 00028 #ifndef LWIP_PPP_OPTS_H 00029 #define LWIP_PPP_OPTS_H 00030 00031 #include "lwip/opt.h" 00032 00033 /** 00034 * PPP_SUPPORT==1: Enable PPP. 00035 */ 00036 #ifndef PPP_SUPPORT 00037 #define PPP_SUPPORT 0 00038 #endif 00039 00040 /** 00041 * PPPOE_SUPPORT==1: Enable PPP Over Ethernet 00042 */ 00043 #ifndef PPPOE_SUPPORT 00044 #define PPPOE_SUPPORT 0 00045 #endif 00046 00047 /** 00048 * PPPOL2TP_SUPPORT==1: Enable PPP Over L2TP 00049 */ 00050 #ifndef PPPOL2TP_SUPPORT 00051 #define PPPOL2TP_SUPPORT 0 00052 #endif 00053 00054 /** 00055 * PPPOL2TP_AUTH_SUPPORT==1: Enable PPP Over L2TP Auth (enable MD5 support) 00056 */ 00057 #ifndef PPPOL2TP_AUTH_SUPPORT 00058 #define PPPOL2TP_AUTH_SUPPORT PPPOL2TP_SUPPORT 00059 #endif 00060 00061 /** 00062 * PPPOS_SUPPORT==1: Enable PPP Over Serial 00063 */ 00064 #ifndef PPPOS_SUPPORT 00065 #define PPPOS_SUPPORT PPP_SUPPORT 00066 #endif 00067 00068 /** 00069 * LWIP_PPP_API==1: Enable PPP API (in pppapi.c) 00070 */ 00071 #ifndef LWIP_PPP_API 00072 #define LWIP_PPP_API (PPP_SUPPORT && (NO_SYS == 0)) 00073 #endif 00074 00075 /** 00076 * MEMP_NUM_PPP_PCB: the number of simultaneously active PPP 00077 * connections (requires the PPP_SUPPORT option) 00078 */ 00079 #ifndef MEMP_NUM_PPP_PCB 00080 #define MEMP_NUM_PPP_PCB 1 00081 #endif 00082 00083 #if PPP_SUPPORT 00084 00085 /** 00086 * MEMP_NUM_PPPOS_INTERFACES: the number of concurrently active PPPoS 00087 * interfaces (only used with PPPOS_SUPPORT==1) 00088 */ 00089 #ifndef MEMP_NUM_PPPOS_INTERFACES 00090 #define MEMP_NUM_PPPOS_INTERFACES MEMP_NUM_PPP_PCB 00091 #endif 00092 00093 /** 00094 * MEMP_NUM_PPPOE_INTERFACES: the number of concurrently active PPPoE 00095 * interfaces (only used with PPPOE_SUPPORT==1) 00096 */ 00097 #ifndef MEMP_NUM_PPPOE_INTERFACES 00098 #define MEMP_NUM_PPPOE_INTERFACES 1 00099 #endif 00100 00101 /** 00102 * MEMP_NUM_PPPOL2TP_INTERFACES: the number of concurrently active PPPoL2TP 00103 * interfaces (only used with PPPOL2TP_SUPPORT==1) 00104 */ 00105 #ifndef MEMP_NUM_PPPOL2TP_INTERFACES 00106 #define MEMP_NUM_PPPOL2TP_INTERFACES 1 00107 #endif 00108 00109 /** 00110 * MEMP_NUM_PPP_API_MSG: Number of concurrent PPP API messages (in pppapi.c) 00111 */ 00112 #ifndef MEMP_NUM_PPP_API_MSG 00113 #define MEMP_NUM_PPP_API_MSG 5 00114 #endif 00115 00116 /** 00117 * PPP_DEBUG: Enable debugging for PPP. 00118 */ 00119 #ifndef PPP_DEBUG 00120 #define PPP_DEBUG LWIP_DBG_OFF 00121 #endif 00122 00123 /** 00124 * PPP_INPROC_IRQ_SAFE==1 call pppos_input() using tcpip_callback(). 00125 * 00126 * Please read the "PPPoS input path" chapter in the PPP documentation about this option. 00127 */ 00128 #ifndef PPP_INPROC_IRQ_SAFE 00129 #define PPP_INPROC_IRQ_SAFE 0 00130 #endif 00131 00132 /** 00133 * PRINTPKT_SUPPORT==1: Enable PPP print packet support 00134 * 00135 * Mandatory for debugging, it displays exchanged packet content in debug trace. 00136 */ 00137 #ifndef PRINTPKT_SUPPORT 00138 #define PRINTPKT_SUPPORT 0 00139 #endif 00140 00141 /** 00142 * PPP_IPV4_SUPPORT==1: Enable PPP IPv4 support 00143 */ 00144 #ifndef PPP_IPV4_SUPPORT 00145 #define PPP_IPV4_SUPPORT (LWIP_IPV4) 00146 #endif 00147 00148 /** 00149 * PPP_IPV6_SUPPORT==1: Enable PPP IPv6 support 00150 */ 00151 #ifndef PPP_IPV6_SUPPORT 00152 #define PPP_IPV6_SUPPORT (LWIP_IPV6) 00153 #endif 00154 00155 /** 00156 * PPP_NOTIFY_PHASE==1: Support PPP notify phase support 00157 * 00158 * PPP notify phase support allows you to set a callback which is 00159 * called on change of the internal PPP state machine. 00160 * 00161 * This can be used for example to set a LED pattern depending on the 00162 * current phase of the PPP session. 00163 */ 00164 #ifndef PPP_NOTIFY_PHASE 00165 #define PPP_NOTIFY_PHASE 0 00166 #endif 00167 00168 /** 00169 * pbuf_type PPP is using for LCP, PAP, CHAP, EAP, CCP, IPCP and IP6CP packets. 00170 * 00171 * Memory allocated must be single buffered for PPP to works, it requires pbuf 00172 * that are not going to be chained when allocated. This requires setting 00173 * PBUF_POOL_BUFSIZE to at least 512 bytes, which is quite huge for small systems. 00174 * 00175 * Setting PPP_USE_PBUF_RAM to 1 makes PPP use memory from heap where continuous 00176 * buffers are required, allowing you to use a smaller PBUF_POOL_BUFSIZE. 00177 */ 00178 #ifndef PPP_USE_PBUF_RAM 00179 #define PPP_USE_PBUF_RAM 0 00180 #endif 00181 00182 /** 00183 * PPP_FCS_TABLE: Keep a 256*2 byte table to speed up FCS calculation for PPPoS 00184 */ 00185 #ifndef PPP_FCS_TABLE 00186 #define PPP_FCS_TABLE 1 00187 #endif 00188 00189 /** 00190 * PAP_SUPPORT==1: Support PAP. 00191 */ 00192 #ifndef PAP_SUPPORT 00193 #define PAP_SUPPORT 0 00194 #endif 00195 00196 /** 00197 * CHAP_SUPPORT==1: Support CHAP. 00198 */ 00199 #ifndef CHAP_SUPPORT 00200 #define CHAP_SUPPORT 0 00201 #endif 00202 00203 /** 00204 * MSCHAP_SUPPORT==1: Support MSCHAP. 00205 */ 00206 #ifndef MSCHAP_SUPPORT 00207 #define MSCHAP_SUPPORT 0 00208 #endif 00209 #if MSCHAP_SUPPORT 00210 /* MSCHAP requires CHAP support */ 00211 #undef CHAP_SUPPORT 00212 #define CHAP_SUPPORT 1 00213 #endif /* MSCHAP_SUPPORT */ 00214 00215 /** 00216 * EAP_SUPPORT==1: Support EAP. 00217 */ 00218 #ifndef EAP_SUPPORT 00219 #define EAP_SUPPORT 0 00220 #endif 00221 00222 /** 00223 * CCP_SUPPORT==1: Support CCP. 00224 */ 00225 #ifndef CCP_SUPPORT 00226 #define CCP_SUPPORT 0 00227 #endif 00228 00229 /** 00230 * MPPE_SUPPORT==1: Support MPPE. 00231 */ 00232 #ifndef MPPE_SUPPORT 00233 #define MPPE_SUPPORT 0 00234 #endif 00235 #if MPPE_SUPPORT 00236 /* MPPE requires CCP support */ 00237 #undef CCP_SUPPORT 00238 #define CCP_SUPPORT 1 00239 /* MPPE requires MSCHAP support */ 00240 #undef MSCHAP_SUPPORT 00241 #define MSCHAP_SUPPORT 1 00242 /* MSCHAP requires CHAP support */ 00243 #undef CHAP_SUPPORT 00244 #define CHAP_SUPPORT 1 00245 #endif /* MPPE_SUPPORT */ 00246 00247 /** 00248 * CBCP_SUPPORT==1: Support CBCP. CURRENTLY NOT SUPPORTED! DO NOT SET! 00249 */ 00250 #ifndef CBCP_SUPPORT 00251 #define CBCP_SUPPORT 0 00252 #endif 00253 00254 /** 00255 * ECP_SUPPORT==1: Support ECP. CURRENTLY NOT SUPPORTED! DO NOT SET! 00256 */ 00257 #ifndef ECP_SUPPORT 00258 #define ECP_SUPPORT 0 00259 #endif 00260 00261 /** 00262 * DEMAND_SUPPORT==1: Support dial on demand. CURRENTLY NOT SUPPORTED! DO NOT SET! 00263 */ 00264 #ifndef DEMAND_SUPPORT 00265 #define DEMAND_SUPPORT 0 00266 #endif 00267 00268 /** 00269 * LQR_SUPPORT==1: Support Link Quality Report. Do nothing except exchanging some LCP packets. 00270 */ 00271 #ifndef LQR_SUPPORT 00272 #define LQR_SUPPORT 0 00273 #endif 00274 00275 /** 00276 * PPP_SERVER==1: Enable PPP server support (waiting for incoming PPP session). 00277 * 00278 * Currently only supported for PPPoS. 00279 */ 00280 #ifndef PPP_SERVER 00281 #define PPP_SERVER 0 00282 #endif 00283 00284 #if PPP_SERVER 00285 /* 00286 * PPP_OUR_NAME: Our name for authentication purposes 00287 */ 00288 #ifndef PPP_OUR_NAME 00289 #define PPP_OUR_NAME "lwIP" 00290 #endif 00291 #endif /* PPP_SERVER */ 00292 00293 /** 00294 * VJ_SUPPORT==1: Support VJ header compression. 00295 */ 00296 #ifndef VJ_SUPPORT 00297 #define VJ_SUPPORT 1 00298 #endif 00299 /* VJ compression is only supported for TCP over IPv4 over PPPoS. */ 00300 #if !PPPOS_SUPPORT || !PPP_IPV4_SUPPORT || !LWIP_TCP 00301 #undef VJ_SUPPORT 00302 #define VJ_SUPPORT 0 00303 #endif /* !PPPOS_SUPPORT */ 00304 00305 /** 00306 * PPP_MD5_RANDM==1: Use MD5 for better randomness. 00307 * Enabled by default if CHAP, EAP, or L2TP AUTH support is enabled. 00308 */ 00309 #ifndef PPP_MD5_RANDM 00310 #define PPP_MD5_RANDM (CHAP_SUPPORT || EAP_SUPPORT || PPPOL2TP_AUTH_SUPPORT) 00311 #endif 00312 00313 /** 00314 * PolarSSL embedded library 00315 * 00316 * 00317 * lwIP contains some files fetched from the latest BSD release of 00318 * the PolarSSL project (PolarSSL 0.10.1-bsd) for ciphers and encryption 00319 * methods we need for lwIP PPP support. 00320 * 00321 * The PolarSSL files were cleaned to contain only the necessary struct 00322 * fields and functions needed for lwIP. 00323 * 00324 * The PolarSSL API was not changed at all, so if you are already using 00325 * PolarSSL you can choose to skip the compilation of the included PolarSSL 00326 * library into lwIP. 00327 * 00328 * If you are not using the embedded copy you must include external 00329 * libraries into your arch/cc.h port file. 00330 * 00331 * Beware of the stack requirements which can be a lot larger if you are not 00332 * using our cleaned PolarSSL library. 00333 */ 00334 00335 /** 00336 * LWIP_USE_EXTERNAL_POLARSSL: Use external PolarSSL library 00337 */ 00338 #ifndef LWIP_USE_EXTERNAL_POLARSSL 00339 #define LWIP_USE_EXTERNAL_POLARSSL 0 00340 #endif 00341 00342 /** 00343 * LWIP_USE_EXTERNAL_MBEDTLS: Use external mbed TLS library 00344 */ 00345 #ifndef LWIP_USE_EXTERNAL_MBEDTLS 00346 #define LWIP_USE_EXTERNAL_MBEDTLS 0 00347 #endif 00348 00349 /* 00350 * PPP Timeouts 00351 */ 00352 00353 /** 00354 * FSM_DEFTIMEOUT: Timeout time in seconds 00355 */ 00356 #ifndef FSM_DEFTIMEOUT 00357 #define FSM_DEFTIMEOUT 6 00358 #endif 00359 00360 /** 00361 * FSM_DEFMAXTERMREQS: Maximum Terminate-Request transmissions 00362 */ 00363 #ifndef FSM_DEFMAXTERMREQS 00364 #define FSM_DEFMAXTERMREQS 2 00365 #endif 00366 00367 /** 00368 * FSM_DEFMAXCONFREQS: Maximum Configure-Request transmissions 00369 */ 00370 #ifndef FSM_DEFMAXCONFREQS 00371 #define FSM_DEFMAXCONFREQS 10 00372 #endif 00373 00374 /** 00375 * FSM_DEFMAXNAKLOOPS: Maximum number of nak loops 00376 */ 00377 #ifndef FSM_DEFMAXNAKLOOPS 00378 #define FSM_DEFMAXNAKLOOPS 5 00379 #endif 00380 00381 /** 00382 * UPAP_DEFTIMEOUT: Timeout (seconds) for retransmitting req 00383 */ 00384 #ifndef UPAP_DEFTIMEOUT 00385 #define UPAP_DEFTIMEOUT 6 00386 #endif 00387 00388 /** 00389 * UPAP_DEFTRANSMITS: Maximum number of auth-reqs to send 00390 */ 00391 #ifndef UPAP_DEFTRANSMITS 00392 #define UPAP_DEFTRANSMITS 10 00393 #endif 00394 00395 #if PPP_SERVER 00396 /** 00397 * UPAP_DEFREQTIME: Time to wait for auth-req from peer 00398 */ 00399 #ifndef UPAP_DEFREQTIME 00400 #define UPAP_DEFREQTIME 30 00401 #endif 00402 #endif /* PPP_SERVER */ 00403 00404 /** 00405 * CHAP_DEFTIMEOUT: Timeout (seconds) for retransmitting req 00406 */ 00407 #ifndef CHAP_DEFTIMEOUT 00408 #define CHAP_DEFTIMEOUT 6 00409 #endif 00410 00411 /** 00412 * CHAP_DEFTRANSMITS: max # times to send challenge 00413 */ 00414 #ifndef CHAP_DEFTRANSMITS 00415 #define CHAP_DEFTRANSMITS 10 00416 #endif 00417 00418 #if PPP_SERVER 00419 /** 00420 * CHAP_DEFRECHALLENGETIME: If this option is > 0, rechallenge the peer every n seconds 00421 */ 00422 #ifndef CHAP_DEFRECHALLENGETIME 00423 #define CHAP_DEFRECHALLENGETIME 0 00424 #endif 00425 #endif /* PPP_SERVER */ 00426 00427 /** 00428 * EAP_DEFREQTIME: Time to wait for peer request 00429 */ 00430 #ifndef EAP_DEFREQTIME 00431 #define EAP_DEFREQTIME 6 00432 #endif 00433 00434 /** 00435 * EAP_DEFALLOWREQ: max # times to accept requests 00436 */ 00437 #ifndef EAP_DEFALLOWREQ 00438 #define EAP_DEFALLOWREQ 10 00439 #endif 00440 00441 #if PPP_SERVER 00442 /** 00443 * EAP_DEFTIMEOUT: Timeout (seconds) for rexmit 00444 */ 00445 #ifndef EAP_DEFTIMEOUT 00446 #define EAP_DEFTIMEOUT 6 00447 #endif 00448 00449 /** 00450 * EAP_DEFTRANSMITS: max # times to transmit 00451 */ 00452 #ifndef EAP_DEFTRANSMITS 00453 #define EAP_DEFTRANSMITS 10 00454 #endif 00455 #endif /* PPP_SERVER */ 00456 00457 /** 00458 * LCP_DEFLOOPBACKFAIL: Default number of times we receive our magic number from the peer 00459 * before deciding the link is looped-back. 00460 */ 00461 #ifndef LCP_DEFLOOPBACKFAIL 00462 #define LCP_DEFLOOPBACKFAIL 10 00463 #endif 00464 00465 /** 00466 * LCP_ECHOINTERVAL: Interval in seconds between keepalive echo requests, 0 to disable. 00467 */ 00468 #ifndef LCP_ECHOINTERVAL 00469 #define LCP_ECHOINTERVAL 0 00470 #endif 00471 00472 /** 00473 * LCP_MAXECHOFAILS: Number of unanswered echo requests before failure. 00474 */ 00475 #ifndef LCP_MAXECHOFAILS 00476 #define LCP_MAXECHOFAILS 3 00477 #endif 00478 00479 /** 00480 * PPP_MAXIDLEFLAG: Max Xmit idle time (in ms) before resend flag char. 00481 */ 00482 #ifndef PPP_MAXIDLEFLAG 00483 #define PPP_MAXIDLEFLAG 100 00484 #endif 00485 00486 /** 00487 * PPP Packet sizes 00488 */ 00489 00490 /** 00491 * PPP_MRU: Default MRU 00492 */ 00493 #ifndef PPP_MRU 00494 #define PPP_MRU 1500 00495 #endif 00496 00497 /** 00498 * PPP_DEFMRU: Default MRU to try 00499 */ 00500 #ifndef PPP_DEFMRU 00501 #define PPP_DEFMRU 1500 00502 #endif 00503 00504 /** 00505 * PPP_MAXMRU: Normally limit MRU to this (pppd default = 16384) 00506 */ 00507 #ifndef PPP_MAXMRU 00508 #define PPP_MAXMRU 1500 00509 #endif 00510 00511 /** 00512 * PPP_MINMRU: No MRUs below this 00513 */ 00514 #ifndef PPP_MINMRU 00515 #define PPP_MINMRU 128 00516 #endif 00517 00518 /** 00519 * PPPOL2TP_DEFMRU: Default MTU and MRU for L2TP 00520 * Default = 1500 - PPPoE(6) - PPP Protocol(2) - IPv4 header(20) - UDP Header(8) 00521 * - L2TP Header(6) - HDLC Header(2) - PPP Protocol(2) - MPPE Header(2) - PPP Protocol(2) 00522 */ 00523 #if PPPOL2TP_SUPPORT 00524 #ifndef PPPOL2TP_DEFMRU 00525 #define PPPOL2TP_DEFMRU 1450 00526 #endif 00527 #endif /* PPPOL2TP_SUPPORT */ 00528 00529 /** 00530 * MAXNAMELEN: max length of hostname or name for auth 00531 */ 00532 #ifndef MAXNAMELEN 00533 #define MAXNAMELEN 256 00534 #endif 00535 00536 /** 00537 * MAXSECRETLEN: max length of password or secret 00538 */ 00539 #ifndef MAXSECRETLEN 00540 #define MAXSECRETLEN 256 00541 #endif 00542 00543 /* ------------------------------------------------------------------------- */ 00544 00545 /* 00546 * Build triggers for embedded PolarSSL 00547 */ 00548 #if !LWIP_USE_EXTERNAL_POLARSSL && !LWIP_USE_EXTERNAL_MBEDTLS 00549 00550 /* CHAP, EAP, L2TP AUTH and MD5 Random require MD5 support */ 00551 #if CHAP_SUPPORT || EAP_SUPPORT || PPPOL2TP_AUTH_SUPPORT || PPP_MD5_RANDM 00552 #define LWIP_INCLUDED_POLARSSL_MD5 1 00553 #endif /* CHAP_SUPPORT || EAP_SUPPORT || PPPOL2TP_AUTH_SUPPORT || PPP_MD5_RANDM */ 00554 00555 #if MSCHAP_SUPPORT 00556 00557 /* MSCHAP require MD4 support */ 00558 #define LWIP_INCLUDED_POLARSSL_MD4 1 00559 /* MSCHAP require SHA1 support */ 00560 #define LWIP_INCLUDED_POLARSSL_SHA1 1 00561 /* MSCHAP require DES support */ 00562 #define LWIP_INCLUDED_POLARSSL_DES 1 00563 00564 /* MS-CHAP support is required for MPPE */ 00565 #if MPPE_SUPPORT 00566 /* MPPE require ARC4 support */ 00567 #define LWIP_INCLUDED_POLARSSL_ARC4 1 00568 #endif /* MPPE_SUPPORT */ 00569 00570 #endif /* MSCHAP_SUPPORT */ 00571 00572 #endif /* !LWIP_USE_EXTERNAL_POLARSSL && !LWIP_USE_EXTERNAL_MBEDTLS */ 00573 00574 /* Default value if unset */ 00575 #ifndef LWIP_INCLUDED_POLARSSL_MD4 00576 #define LWIP_INCLUDED_POLARSSL_MD4 0 00577 #endif /* LWIP_INCLUDED_POLARSSL_MD4 */ 00578 #ifndef LWIP_INCLUDED_POLARSSL_MD5 00579 #define LWIP_INCLUDED_POLARSSL_MD5 0 00580 #endif /* LWIP_INCLUDED_POLARSSL_MD5 */ 00581 #ifndef LWIP_INCLUDED_POLARSSL_SHA1 00582 #define LWIP_INCLUDED_POLARSSL_SHA1 0 00583 #endif /* LWIP_INCLUDED_POLARSSL_SHA1 */ 00584 #ifndef LWIP_INCLUDED_POLARSSL_DES 00585 #define LWIP_INCLUDED_POLARSSL_DES 0 00586 #endif /* LWIP_INCLUDED_POLARSSL_DES */ 00587 #ifndef LWIP_INCLUDED_POLARSSL_ARC4 00588 #define LWIP_INCLUDED_POLARSSL_ARC4 0 00589 #endif /* LWIP_INCLUDED_POLARSSL_ARC4 */ 00590 00591 #endif /* PPP_SUPPORT */ 00592 00593 #endif /* LWIP_PPP_OPTS_H */
Generated on Sun Jul 17 2022 08:25:29 by
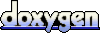