
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ppp_impl.h
00001 /***************************************************************************** 00002 * ppp.h - Network Point to Point Protocol header file. 00003 * 00004 * Copyright (c) 2003 by Marc Boucher, Services Informatiques (MBSI) inc. 00005 * portions Copyright (c) 1997 Global Election Systems Inc. 00006 * 00007 * The authors hereby grant permission to use, copy, modify, distribute, 00008 * and license this software and its documentation for any purpose, provided 00009 * that existing copyright notices are retained in all copies and that this 00010 * notice and the following disclaimer are included verbatim in any 00011 * distributions. No written agreement, license, or royalty fee is required 00012 * for any of the authorized uses. 00013 * 00014 * THIS SOFTWARE IS PROVIDED BY THE CONTRIBUTORS *AS IS* AND ANY EXPRESS OR 00015 * IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES 00016 * OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00017 * IN NO EVENT SHALL THE CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00018 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 00019 * NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00020 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00021 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00022 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF 00023 * THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00024 * 00025 ****************************************************************************** 00026 * REVISION HISTORY 00027 * 00028 * 03-01-01 Marc Boucher <marc@mbsi.ca> 00029 * Ported to lwIP. 00030 * 97-11-05 Guy Lancaster <glanca@gesn.com>, Global Election Systems Inc. 00031 * Original derived from BSD codes. 00032 *****************************************************************************/ 00033 #ifndef LWIP_HDR_PPP_IMPL_H 00034 #define LWIP_HDR_PPP_IMPL_H 00035 00036 #include "netif/ppp/ppp_opts.h" 00037 00038 #if PPP_SUPPORT /* don't build if not configured for use in lwipopts.h */ 00039 00040 #ifdef PPP_INCLUDE_SETTINGS_HEADER 00041 #include "ppp_settings.h" 00042 #endif 00043 00044 #include <stdio.h> /* formats */ 00045 #include <stdarg.h> 00046 #include <string.h> 00047 #include <stdlib.h> /* strtol() */ 00048 00049 #include "lwip/netif.h" 00050 #include "lwip/def.h" 00051 #include "lwip/timeouts.h" 00052 00053 #include "ppp.h" 00054 #include "pppdebug.h" 00055 00056 /* 00057 * Memory used for control packets. 00058 * 00059 * PPP_CTRL_PBUF_MAX_SIZE is the amount of memory we allocate when we 00060 * cannot figure out how much we are going to use before filling the buffer. 00061 */ 00062 #if PPP_USE_PBUF_RAM 00063 #define PPP_CTRL_PBUF_TYPE PBUF_RAM 00064 #define PPP_CTRL_PBUF_MAX_SIZE 512 00065 #else /* PPP_USE_PBUF_RAM */ 00066 #define PPP_CTRL_PBUF_TYPE PBUF_POOL 00067 #define PPP_CTRL_PBUF_MAX_SIZE PBUF_POOL_BUFSIZE 00068 #endif /* PPP_USE_PBUF_RAM */ 00069 00070 /* 00071 * The basic PPP frame. 00072 */ 00073 #define PPP_ADDRESS(p) (((u_char *)(p))[0]) 00074 #define PPP_CONTROL(p) (((u_char *)(p))[1]) 00075 #define PPP_PROTOCOL(p) ((((u_char *)(p))[2] << 8) + ((u_char *)(p))[3]) 00076 00077 /* 00078 * Significant octet values. 00079 */ 00080 #define PPP_ALLSTATIONS 0xff /* All-Stations broadcast address */ 00081 #define PPP_UI 0x03 /* Unnumbered Information */ 00082 #define PPP_FLAG 0x7e /* Flag Sequence */ 00083 #define PPP_ESCAPE 0x7d /* Asynchronous Control Escape */ 00084 #define PPP_TRANS 0x20 /* Asynchronous transparency modifier */ 00085 00086 /* 00087 * Protocol field values. 00088 */ 00089 #define PPP_IP 0x21 /* Internet Protocol */ 00090 #if 0 /* UNUSED */ 00091 #define PPP_AT 0x29 /* AppleTalk Protocol */ 00092 #define PPP_IPX 0x2b /* IPX protocol */ 00093 #endif /* UNUSED */ 00094 #if VJ_SUPPORT 00095 #define PPP_VJC_COMP 0x2d /* VJ compressed TCP */ 00096 #define PPP_VJC_UNCOMP 0x2f /* VJ uncompressed TCP */ 00097 #endif /* VJ_SUPPORT */ 00098 #if PPP_IPV6_SUPPORT 00099 #define PPP_IPV6 0x57 /* Internet Protocol Version 6 */ 00100 #endif /* PPP_IPV6_SUPPORT */ 00101 #if CCP_SUPPORT 00102 #define PPP_COMP 0xfd /* compressed packet */ 00103 #endif /* CCP_SUPPORT */ 00104 #define PPP_IPCP 0x8021 /* IP Control Protocol */ 00105 #if 0 /* UNUSED */ 00106 #define PPP_ATCP 0x8029 /* AppleTalk Control Protocol */ 00107 #define PPP_IPXCP 0x802b /* IPX Control Protocol */ 00108 #endif /* UNUSED */ 00109 #if PPP_IPV6_SUPPORT 00110 #define PPP_IPV6CP 0x8057 /* IPv6 Control Protocol */ 00111 #endif /* PPP_IPV6_SUPPORT */ 00112 #if CCP_SUPPORT 00113 #define PPP_CCP 0x80fd /* Compression Control Protocol */ 00114 #endif /* CCP_SUPPORT */ 00115 #if ECP_SUPPORT 00116 #define PPP_ECP 0x8053 /* Encryption Control Protocol */ 00117 #endif /* ECP_SUPPORT */ 00118 #define PPP_LCP 0xc021 /* Link Control Protocol */ 00119 #if PAP_SUPPORT 00120 #define PPP_PAP 0xc023 /* Password Authentication Protocol */ 00121 #endif /* PAP_SUPPORT */ 00122 #if LQR_SUPPORT 00123 #define PPP_LQR 0xc025 /* Link Quality Report protocol */ 00124 #endif /* LQR_SUPPORT */ 00125 #if CHAP_SUPPORT 00126 #define PPP_CHAP 0xc223 /* Cryptographic Handshake Auth. Protocol */ 00127 #endif /* CHAP_SUPPORT */ 00128 #if CBCP_SUPPORT 00129 #define PPP_CBCP 0xc029 /* Callback Control Protocol */ 00130 #endif /* CBCP_SUPPORT */ 00131 #if EAP_SUPPORT 00132 #define PPP_EAP 0xc227 /* Extensible Authentication Protocol */ 00133 #endif /* EAP_SUPPORT */ 00134 00135 /* 00136 * The following struct gives the addresses of procedures to call 00137 * for a particular lower link level protocol. 00138 */ 00139 struct link_callbacks { 00140 /* Start a connection (e.g. Initiate discovery phase) */ 00141 void (*connect) (ppp_pcb *pcb, void *ctx); 00142 #if PPP_SERVER 00143 /* Listen for an incoming connection (Passive mode) */ 00144 void (*listen) (ppp_pcb *pcb, void *ctx); 00145 #endif /* PPP_SERVER */ 00146 /* End a connection (i.e. initiate disconnect phase) */ 00147 void (*disconnect) (ppp_pcb *pcb, void *ctx); 00148 /* Free lower protocol control block */ 00149 err_t (*free) (ppp_pcb *pcb, void *ctx); 00150 /* Write a pbuf to a ppp link, only used from PPP functions to send PPP packets. */ 00151 err_t (*write)(ppp_pcb *pcb, void *ctx, struct pbuf *p); 00152 /* Send a packet from lwIP core (IPv4 or IPv6) */ 00153 err_t (*netif_output)(ppp_pcb *pcb, void *ctx, struct pbuf *p, u_short protocol); 00154 /* configure the transmit-side characteristics of the PPP interface */ 00155 void (*send_config)(ppp_pcb *pcb, void *ctx, u32_t accm, int pcomp, int accomp); 00156 /* confire the receive-side characteristics of the PPP interface */ 00157 void (*recv_config)(ppp_pcb *pcb, void *ctx, u32_t accm, int pcomp, int accomp); 00158 }; 00159 00160 /* 00161 * What to do with network protocol (NP) packets. 00162 */ 00163 enum NPmode { 00164 NPMODE_PASS, /* pass the packet through */ 00165 NPMODE_DROP, /* silently drop the packet */ 00166 NPMODE_ERROR, /* return an error */ 00167 NPMODE_QUEUE /* save it up for later. */ 00168 }; 00169 00170 /* 00171 * Statistics. 00172 */ 00173 #if PPP_STATS_SUPPORT 00174 struct pppstat { 00175 unsigned int ppp_ibytes; /* bytes received */ 00176 unsigned int ppp_ipackets; /* packets received */ 00177 unsigned int ppp_ierrors; /* receive errors */ 00178 unsigned int ppp_obytes; /* bytes sent */ 00179 unsigned int ppp_opackets; /* packets sent */ 00180 unsigned int ppp_oerrors; /* transmit errors */ 00181 }; 00182 00183 #if VJ_SUPPORT 00184 struct vjstat { 00185 unsigned int vjs_packets; /* outbound packets */ 00186 unsigned int vjs_compressed; /* outbound compressed packets */ 00187 unsigned int vjs_searches; /* searches for connection state */ 00188 unsigned int vjs_misses; /* times couldn't find conn. state */ 00189 unsigned int vjs_uncompressedin; /* inbound uncompressed packets */ 00190 unsigned int vjs_compressedin; /* inbound compressed packets */ 00191 unsigned int vjs_errorin; /* inbound unknown type packets */ 00192 unsigned int vjs_tossed; /* inbound packets tossed because of error */ 00193 }; 00194 #endif /* VJ_SUPPORT */ 00195 00196 struct ppp_stats { 00197 struct pppstat p; /* basic PPP statistics */ 00198 #if VJ_SUPPORT 00199 struct vjstat vj; /* VJ header compression statistics */ 00200 #endif /* VJ_SUPPORT */ 00201 }; 00202 00203 #if CCP_SUPPORT 00204 struct compstat { 00205 unsigned int unc_bytes; /* total uncompressed bytes */ 00206 unsigned int unc_packets; /* total uncompressed packets */ 00207 unsigned int comp_bytes; /* compressed bytes */ 00208 unsigned int comp_packets; /* compressed packets */ 00209 unsigned int inc_bytes; /* incompressible bytes */ 00210 unsigned int inc_packets; /* incompressible packets */ 00211 unsigned int ratio; /* recent compression ratio << 8 */ 00212 }; 00213 00214 struct ppp_comp_stats { 00215 struct compstat c; /* packet compression statistics */ 00216 struct compstat d; /* packet decompression statistics */ 00217 }; 00218 #endif /* CCP_SUPPORT */ 00219 00220 #endif /* PPP_STATS_SUPPORT */ 00221 00222 #if PPP_IDLETIMELIMIT 00223 /* 00224 * The following structure records the time in seconds since 00225 * the last NP packet was sent or received. 00226 */ 00227 struct ppp_idle { 00228 time_t xmit_idle; /* time since last NP packet sent */ 00229 time_t recv_idle; /* time since last NP packet received */ 00230 }; 00231 #endif /* PPP_IDLETIMELIMIT */ 00232 00233 /* values for epdisc.class */ 00234 #define EPD_NULL 0 /* null discriminator, no data */ 00235 #define EPD_LOCAL 1 00236 #define EPD_IP 2 00237 #define EPD_MAC 3 00238 #define EPD_MAGIC 4 00239 #define EPD_PHONENUM 5 00240 00241 /* 00242 * Global variables. 00243 */ 00244 #ifdef HAVE_MULTILINK 00245 extern u8_t multilink; /* enable multilink operation */ 00246 extern u8_t doing_multilink; 00247 extern u8_t multilink_master; 00248 extern u8_t bundle_eof; 00249 extern u8_t bundle_terminating; 00250 #endif 00251 00252 #ifdef MAXOCTETS 00253 extern unsigned int maxoctets; /* Maximum octetes per session (in bytes) */ 00254 extern int maxoctets_dir; /* Direction : 00255 0 - in+out (default) 00256 1 - in 00257 2 - out 00258 3 - max(in,out) */ 00259 extern int maxoctets_timeout; /* Timeout for check of octets limit */ 00260 #define PPP_OCTETS_DIRECTION_SUM 0 00261 #define PPP_OCTETS_DIRECTION_IN 1 00262 #define PPP_OCTETS_DIRECTION_OUT 2 00263 #define PPP_OCTETS_DIRECTION_MAXOVERAL 3 00264 /* same as previos, but little different on RADIUS side */ 00265 #define PPP_OCTETS_DIRECTION_MAXSESSION 4 00266 #endif 00267 00268 /* Data input may be used by CCP and ECP, remove this entry 00269 * from struct protent to save some flash 00270 */ 00271 #define PPP_DATAINPUT 0 00272 00273 /* 00274 * The following struct gives the addresses of procedures to call 00275 * for a particular protocol. 00276 */ 00277 struct protent { 00278 u_short protocol; /* PPP protocol number */ 00279 /* Initialization procedure */ 00280 void (*init) (ppp_pcb *pcb); 00281 /* Process a received packet */ 00282 void (*input) (ppp_pcb *pcb, u_char *pkt, int len); 00283 /* Process a received protocol-reject */ 00284 void (*protrej) (ppp_pcb *pcb); 00285 /* Lower layer has come up */ 00286 void (*lowerup) (ppp_pcb *pcb); 00287 /* Lower layer has gone down */ 00288 void (*lowerdown) (ppp_pcb *pcb); 00289 /* Open the protocol */ 00290 void (*open) (ppp_pcb *pcb); 00291 /* Close the protocol */ 00292 void (*close) (ppp_pcb *pcb, const char *reason); 00293 #if PRINTPKT_SUPPORT 00294 /* Print a packet in readable form */ 00295 int (*printpkt) (const u_char *pkt, int len, 00296 void (*printer) (void *, const char *, ...), 00297 void *arg); 00298 #endif /* PRINTPKT_SUPPORT */ 00299 #if PPP_DATAINPUT 00300 /* Process a received data packet */ 00301 void (*datainput) (ppp_pcb *pcb, u_char *pkt, int len); 00302 #endif /* PPP_DATAINPUT */ 00303 #if PRINTPKT_SUPPORT 00304 const char *name; /* Text name of protocol */ 00305 const char *data_name; /* Text name of corresponding data protocol */ 00306 #endif /* PRINTPKT_SUPPORT */ 00307 #if PPP_OPTIONS 00308 option_t *options; /* List of command-line options */ 00309 /* Check requested options, assign defaults */ 00310 void (*check_options) (void); 00311 #endif /* PPP_OPTIONS */ 00312 #if DEMAND_SUPPORT 00313 /* Configure interface for demand-dial */ 00314 int (*demand_conf) (int unit); 00315 /* Say whether to bring up link for this pkt */ 00316 int (*active_pkt) (u_char *pkt, int len); 00317 #endif /* DEMAND_SUPPORT */ 00318 }; 00319 00320 /* Table of pointers to supported protocols */ 00321 extern const struct protent* const protocols[]; 00322 00323 00324 /* Values for auth_pending, auth_done */ 00325 #if PAP_SUPPORT 00326 #define PAP_WITHPEER 0x1 00327 #define PAP_PEER 0x2 00328 #endif /* PAP_SUPPORT */ 00329 #if CHAP_SUPPORT 00330 #define CHAP_WITHPEER 0x4 00331 #define CHAP_PEER 0x8 00332 #endif /* CHAP_SUPPORT */ 00333 #if EAP_SUPPORT 00334 #define EAP_WITHPEER 0x10 00335 #define EAP_PEER 0x20 00336 #endif /* EAP_SUPPORT */ 00337 00338 /* Values for auth_done only */ 00339 #if CHAP_SUPPORT 00340 #define CHAP_MD5_WITHPEER 0x40 00341 #define CHAP_MD5_PEER 0x80 00342 #if MSCHAP_SUPPORT 00343 #define CHAP_MS_SHIFT 8 /* LSB position for MS auths */ 00344 #define CHAP_MS_WITHPEER 0x100 00345 #define CHAP_MS_PEER 0x200 00346 #define CHAP_MS2_WITHPEER 0x400 00347 #define CHAP_MS2_PEER 0x800 00348 #endif /* MSCHAP_SUPPORT */ 00349 #endif /* CHAP_SUPPORT */ 00350 00351 /* Supported CHAP protocols */ 00352 #if CHAP_SUPPORT 00353 00354 #if MSCHAP_SUPPORT 00355 #define CHAP_MDTYPE_SUPPORTED (MDTYPE_MICROSOFT_V2 | MDTYPE_MICROSOFT | MDTYPE_MD5) 00356 #else /* MSCHAP_SUPPORT */ 00357 #define CHAP_MDTYPE_SUPPORTED (MDTYPE_MD5) 00358 #endif /* MSCHAP_SUPPORT */ 00359 00360 #else /* CHAP_SUPPORT */ 00361 #define CHAP_MDTYPE_SUPPORTED (MDTYPE_NONE) 00362 #endif /* CHAP_SUPPORT */ 00363 00364 #if PPP_STATS_SUPPORT 00365 /* 00366 * PPP statistics structure 00367 */ 00368 struct pppd_stats { 00369 unsigned int bytes_in; 00370 unsigned int bytes_out; 00371 unsigned int pkts_in; 00372 unsigned int pkts_out; 00373 }; 00374 #endif /* PPP_STATS_SUPPORT */ 00375 00376 00377 /* 00378 * PPP private functions 00379 */ 00380 00381 00382 /* 00383 * Functions called from lwIP core. 00384 */ 00385 00386 /* initialize the PPP subsystem */ 00387 int ppp_init(void); 00388 00389 /* 00390 * Functions called from PPP link protocols. 00391 */ 00392 00393 /* Create a new PPP control block */ 00394 ppp_pcb *ppp_new(struct netif *pppif, const struct link_callbacks *callbacks, void *link_ctx_cb, 00395 ppp_link_status_cb_fn link_status_cb, void *ctx_cb); 00396 00397 /* Initiate LCP open request */ 00398 void ppp_start(ppp_pcb *pcb); 00399 00400 /* Called when link failed to setup */ 00401 void ppp_link_failed(ppp_pcb *pcb); 00402 00403 /* Called when link is normally down (i.e. it was asked to end) */ 00404 void ppp_link_end(ppp_pcb *pcb); 00405 00406 /* function called to process input packet */ 00407 void ppp_input(ppp_pcb *pcb, struct pbuf *pb); 00408 00409 /* helper function, merge a pbuf chain into one pbuf */ 00410 struct pbuf *ppp_singlebuf(struct pbuf *p); 00411 00412 00413 /* 00414 * Functions called by PPP protocols. 00415 */ 00416 00417 /* function called by all PPP subsystems to send packets */ 00418 err_t ppp_write(ppp_pcb *pcb, struct pbuf *p); 00419 00420 /* functions called by auth.c link_terminated() */ 00421 void ppp_link_terminated(ppp_pcb *pcb); 00422 00423 void new_phase(ppp_pcb *pcb, int p); 00424 00425 int ppp_send_config(ppp_pcb *pcb, int mtu, u32_t accm, int pcomp, int accomp); 00426 int ppp_recv_config(ppp_pcb *pcb, int mru, u32_t accm, int pcomp, int accomp); 00427 00428 #if PPP_IPV4_SUPPORT 00429 int sifaddr(ppp_pcb *pcb, u32_t our_adr, u32_t his_adr, u32_t netmask); 00430 int cifaddr(ppp_pcb *pcb, u32_t our_adr, u32_t his_adr); 00431 #if 0 /* UNUSED - PROXY ARP */ 00432 int sifproxyarp(ppp_pcb *pcb, u32_t his_adr); 00433 int cifproxyarp(ppp_pcb *pcb, u32_t his_adr); 00434 #endif /* UNUSED - PROXY ARP */ 00435 #if LWIP_DNS 00436 int sdns(ppp_pcb *pcb, u32_t ns1, u32_t ns2); 00437 int cdns(ppp_pcb *pcb, u32_t ns1, u32_t ns2); 00438 #endif /* LWIP_DNS */ 00439 #if VJ_SUPPORT 00440 int sifvjcomp(ppp_pcb *pcb, int vjcomp, int cidcomp, int maxcid); 00441 #endif /* VJ_SUPPORT */ 00442 int sifup(ppp_pcb *pcb); 00443 int sifdown (ppp_pcb *pcb); 00444 u32_t get_mask(u32_t addr); 00445 #endif /* PPP_IPV4_SUPPORT */ 00446 00447 #if PPP_IPV6_SUPPORT 00448 int sif6addr(ppp_pcb *pcb, eui64_t our_eui64, eui64_t his_eui64); 00449 int cif6addr(ppp_pcb *pcb, eui64_t our_eui64, eui64_t his_eui64); 00450 int sif6up(ppp_pcb *pcb); 00451 int sif6down (ppp_pcb *pcb); 00452 #endif /* PPP_IPV6_SUPPORT */ 00453 00454 #if DEMAND_SUPPORT 00455 int sifnpmode(ppp_pcb *pcb, int proto, enum NPmode mode); 00456 #endif /* DEMAND_SUPPORt */ 00457 00458 void netif_set_mtu(ppp_pcb *pcb, int mtu); 00459 int netif_get_mtu(ppp_pcb *pcb); 00460 00461 #if CCP_SUPPORT 00462 #if 0 /* unused */ 00463 int ccp_test(ppp_pcb *pcb, u_char *opt_ptr, int opt_len, int for_transmit); 00464 #endif /* unused */ 00465 void ccp_set(ppp_pcb *pcb, u8_t isopen, u8_t isup, u8_t receive_method, u8_t transmit_method); 00466 void ccp_reset_comp(ppp_pcb *pcb); 00467 void ccp_reset_decomp(ppp_pcb *pcb); 00468 #if 0 /* unused */ 00469 int ccp_fatal_error(ppp_pcb *pcb); 00470 #endif /* unused */ 00471 #endif /* CCP_SUPPORT */ 00472 00473 #if PPP_IDLETIMELIMIT 00474 int get_idle_time(ppp_pcb *pcb, struct ppp_idle *ip); 00475 #endif /* PPP_IDLETIMELIMIT */ 00476 00477 #if DEMAND_SUPPORT 00478 int get_loop_output(void); 00479 #endif /* DEMAND_SUPPORT */ 00480 00481 /* Optional protocol names list, to make our messages a little more informative. */ 00482 #if PPP_PROTOCOLNAME 00483 const char * protocol_name(int proto); 00484 #endif /* PPP_PROTOCOLNAME */ 00485 00486 /* Optional stats support, to get some statistics on the PPP interface */ 00487 #if PPP_STATS_SUPPORT 00488 void print_link_stats(void); /* Print stats, if available */ 00489 void reset_link_stats(int u); /* Reset (init) stats when link goes up */ 00490 void update_link_stats(int u); /* Get stats at link termination */ 00491 #endif /* PPP_STATS_SUPPORT */ 00492 00493 00494 00495 /* 00496 * Inline versions of get/put char/short/long. 00497 * Pointer is advanced; we assume that both arguments 00498 * are lvalues and will already be in registers. 00499 * cp MUST be u_char *. 00500 */ 00501 #define GETCHAR(c, cp) { \ 00502 (c) = *(cp)++; \ 00503 } 00504 #define PUTCHAR(c, cp) { \ 00505 *(cp)++ = (u_char) (c); \ 00506 } 00507 #define GETSHORT(s, cp) { \ 00508 (s) = *(cp)++ << 8; \ 00509 (s) |= *(cp)++; \ 00510 } 00511 #define PUTSHORT(s, cp) { \ 00512 *(cp)++ = (u_char) ((s) >> 8); \ 00513 *(cp)++ = (u_char) (s); \ 00514 } 00515 #define GETLONG(l, cp) { \ 00516 (l) = *(cp)++ << 8; \ 00517 (l) |= *(cp)++; (l) <<= 8; \ 00518 (l) |= *(cp)++; (l) <<= 8; \ 00519 (l) |= *(cp)++; \ 00520 } 00521 #define PUTLONG(l, cp) { \ 00522 *(cp)++ = (u_char) ((l) >> 24); \ 00523 *(cp)++ = (u_char) ((l) >> 16); \ 00524 *(cp)++ = (u_char) ((l) >> 8); \ 00525 *(cp)++ = (u_char) (l); \ 00526 } 00527 00528 #define INCPTR(n, cp) ((cp) += (n)) 00529 #define DECPTR(n, cp) ((cp) -= (n)) 00530 00531 /* 00532 * System dependent definitions for user-level 4.3BSD UNIX implementation. 00533 */ 00534 #define TIMEOUT(f, a, t) do { sys_untimeout((f), (a)); sys_timeout((t)*1000, (f), (a)); } while(0) 00535 #define TIMEOUTMS(f, a, t) do { sys_untimeout((f), (a)); sys_timeout((t), (f), (a)); } while(0) 00536 #define UNTIMEOUT(f, a) sys_untimeout((f), (a)) 00537 00538 #define BZERO(s, n) memset(s, 0, n) 00539 #define BCMP(s1, s2, l) memcmp(s1, s2, l) 00540 00541 #define PRINTMSG(m, l) { ppp_info("Remote message: %0.*v", l, m); } 00542 00543 /* 00544 * MAKEHEADER - Add Header fields to a packet. 00545 */ 00546 #define MAKEHEADER(p, t) { \ 00547 PUTCHAR(PPP_ALLSTATIONS, p); \ 00548 PUTCHAR(PPP_UI, p); \ 00549 PUTSHORT(t, p); } 00550 00551 /* Procedures exported from auth.c */ 00552 void link_required(ppp_pcb *pcb); /* we are starting to use the link */ 00553 void link_terminated(ppp_pcb *pcb); /* we are finished with the link */ 00554 void link_down(ppp_pcb *pcb); /* the LCP layer has left the Opened state */ 00555 void upper_layers_down(ppp_pcb *pcb); /* take all NCPs down */ 00556 void link_established(ppp_pcb *pcb); /* the link is up; authenticate now */ 00557 void start_networks(ppp_pcb *pcb); /* start all the network control protos */ 00558 void continue_networks(ppp_pcb *pcb); /* start network [ip, etc] control protos */ 00559 #if PPP_AUTH_SUPPORT 00560 #if PPP_SERVER 00561 int auth_check_passwd(ppp_pcb *pcb, char *auser, int userlen, char *apasswd, int passwdlen, const char **msg, int *msglen); 00562 /* check the user name and passwd against configuration */ 00563 void auth_peer_fail(ppp_pcb *pcb, int protocol); 00564 /* peer failed to authenticate itself */ 00565 void auth_peer_success(ppp_pcb *pcb, int protocol, int prot_flavor, const char *name, int namelen); 00566 /* peer successfully authenticated itself */ 00567 #endif /* PPP_SERVER */ 00568 void auth_withpeer_fail(ppp_pcb *pcb, int protocol); 00569 /* we failed to authenticate ourselves */ 00570 void auth_withpeer_success(ppp_pcb *pcb, int protocol, int prot_flavor); 00571 /* we successfully authenticated ourselves */ 00572 #endif /* PPP_AUTH_SUPPORT */ 00573 void np_up(ppp_pcb *pcb, int proto); /* a network protocol has come up */ 00574 void np_down(ppp_pcb *pcb, int proto); /* a network protocol has gone down */ 00575 void np_finished(ppp_pcb *pcb, int proto); /* a network protocol no longer needs link */ 00576 #if PPP_AUTH_SUPPORT 00577 int get_secret(ppp_pcb *pcb, const char *client, const char *server, char *secret, int *secret_len, int am_server); 00578 /* get "secret" for chap */ 00579 #endif /* PPP_AUTH_SUPPORT */ 00580 00581 /* Procedures exported from ipcp.c */ 00582 /* int parse_dotted_ip (char *, u32_t *); */ 00583 00584 /* Procedures exported from demand.c */ 00585 #if DEMAND_SUPPORT 00586 void demand_conf (void); /* config interface(s) for demand-dial */ 00587 void demand_block (void); /* set all NPs to queue up packets */ 00588 void demand_unblock (void); /* set all NPs to pass packets */ 00589 void demand_discard (void); /* set all NPs to discard packets */ 00590 void demand_rexmit (int, u32_t); /* retransmit saved frames for an NP*/ 00591 int loop_chars (unsigned char *, int); /* process chars from loopback */ 00592 int loop_frame (unsigned char *, int); /* should we bring link up? */ 00593 #endif /* DEMAND_SUPPORT */ 00594 00595 /* Procedures exported from multilink.c */ 00596 #ifdef HAVE_MULTILINK 00597 void mp_check_options (void); /* Check multilink-related options */ 00598 int mp_join_bundle (void); /* join our link to an appropriate bundle */ 00599 void mp_exit_bundle (void); /* have disconnected our link from bundle */ 00600 void mp_bundle_terminated (void); 00601 char *epdisc_to_str (struct epdisc *); /* string from endpoint discrim. */ 00602 int str_to_epdisc (struct epdisc *, char *); /* endpt disc. from str */ 00603 #else 00604 #define mp_bundle_terminated() /* nothing */ 00605 #define mp_exit_bundle() /* nothing */ 00606 #define doing_multilink 0 00607 #define multilink_master 0 00608 #endif 00609 00610 /* Procedures exported from utils.c. */ 00611 void ppp_print_string(const u_char *p, int len, void (*printer) (void *, const char *, ...), void *arg); /* Format a string for output */ 00612 int ppp_slprintf(char *buf, int buflen, const char *fmt, ...); /* sprintf++ */ 00613 int ppp_vslprintf(char *buf, int buflen, const char *fmt, va_list args); /* vsprintf++ */ 00614 size_t ppp_strlcpy(char *dest, const char *src, size_t len); /* safe strcpy */ 00615 size_t ppp_strlcat(char *dest, const char *src, size_t len); /* safe strncpy */ 00616 void ppp_dbglog(const char *fmt, ...); /* log a debug message */ 00617 void ppp_info(const char *fmt, ...); /* log an informational message */ 00618 void ppp_notice(const char *fmt, ...); /* log a notice-level message */ 00619 void ppp_warn(const char *fmt, ...); /* log a warning message */ 00620 void ppp_error(const char *fmt, ...); /* log an error message */ 00621 void ppp_fatal(const char *fmt, ...); /* log an error message and die(1) */ 00622 #if PRINTPKT_SUPPORT 00623 void ppp_dump_packet(ppp_pcb *pcb, const char *tag, unsigned char *p, int len); 00624 /* dump packet to debug log if interesting */ 00625 #endif /* PRINTPKT_SUPPORT */ 00626 00627 00628 #endif /* PPP_SUPPORT */ 00629 #endif /* LWIP_HDR_PPP_IMPL_H */
Generated on Sun Jul 17 2022 08:25:29 by
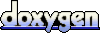