
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
paths.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2013 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 from os.path import join 00018 from os import getenv 00019 00020 # Conventions about the directory structure 00021 from tools.settings import ROOT, BUILD_DIR 00022 00023 # Allow overriding some of the build parameters using environment variables 00024 BUILD_DIR = getenv("MBED_BUILD_DIR") or BUILD_DIR 00025 00026 # Embedded Libraries Sources 00027 LIB_DIR = join(ROOT, "features/unsupported") 00028 00029 TOOLS = join(ROOT, "tools") 00030 TOOLS_DATA = join(TOOLS, "data") 00031 TOOLS_BOOTLOADERS = join(TOOLS, "bootloaders") 00032 00033 # mbed libraries 00034 MBED_HEADER = join(ROOT, "mbed.h") 00035 MBED_DRIVERS = join(ROOT, "drivers") 00036 MBED_PLATFORM = join(ROOT, "platform") 00037 MBED_HAL = join(ROOT, "hal") 00038 00039 MBED_CMSIS_PATH = join(ROOT, "cmsis") 00040 MBED_TARGETS_PATH = join(ROOT, "targets") 00041 00042 MBED_LIBRARIES = join(BUILD_DIR, "mbed") 00043 MBED_LIBRARIES_DRIVERS = join(MBED_LIBRARIES, "drivers") 00044 MBED_LIBRARIES_PLATFORM = join(MBED_LIBRARIES, "platform") 00045 MBED_LIBRARIES_HAL = join(MBED_LIBRARIES, "hal") 00046 00047 MBED_CONFIG_FILE = join(ROOT, "platform/mbed_lib.json") 00048 00049 # Tests 00050 TEST_DIR = join(LIB_DIR, "tests") 00051 HOST_TESTS = join(ROOT, "tools", "host_tests") 00052 00053 # mbed RPC 00054 MBED_RPC = join(LIB_DIR, "rpc") 00055 00056 RPC_LIBRARY = join(BUILD_DIR, "rpc") 00057 00058 # DSP 00059 DSP = join(LIB_DIR, "dsp") 00060 DSP_CMSIS = join(DSP, "cmsis_dsp") 00061 DSP_ABSTRACTION = join(DSP, "dsp") 00062 DSP_LIBRARIES = join(BUILD_DIR, "dsp") 00063 00064 # USB Device 00065 USB = join(LIB_DIR, "USBDevice") 00066 USB_LIBRARIES = join(BUILD_DIR, "usb") 00067 00068 # Export 00069 EXPORT_DIR = join(BUILD_DIR, "export") 00070 EXPORT_WORKSPACE = join(EXPORT_DIR, "workspace") 00071 EXPORT_TMP = join(EXPORT_DIR, ".temp") 00072 00073 # CppUtest library 00074 CPPUTEST_DIR = join(ROOT, "..") 00075 CPPUTEST_SRC = join(CPPUTEST_DIR, "cpputest", "src", "CppUTest") 00076 CPPUTEST_INC = join(CPPUTEST_DIR, "cpputest", "include") 00077 CPPUTEST_INC_EXT = join(CPPUTEST_DIR, "cpputest", "include", "CppUTest") 00078 # Platform dependant code is here (for armcc compiler) 00079 CPPUTEST_PLATFORM_SRC = join(CPPUTEST_DIR, "cpputest", "src", "Platforms", 00080 "armcc") 00081 CPPUTEST_PLATFORM_INC = join(CPPUTEST_DIR, "cpputest", "include", "Platforms", 00082 "armcc") 00083 # Function 'main' used to run all compiled UTs 00084 CPPUTEST_TESTRUNNER_SCR = join(TEST_DIR, "utest", "testrunner") 00085 CPPUTEST_TESTRUNNER_INC = join(TEST_DIR, "utest", "testrunner") 00086 00087 CPPUTEST_LIBRARY = join(BUILD_DIR, "cpputest")
Generated on Sun Jul 17 2022 08:25:29 by
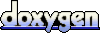