
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
GapEvents.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017-2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef BLE_PAL_GAP_MESSAGE_H_ 00018 #define BLE_PAL_GAP_MESSAGE_H_ 00019 00020 #include "GapTypes.h" 00021 #include "ble/ArrayView.h " 00022 00023 namespace ble { 00024 namespace pal { 00025 00026 /** 00027 * Enumeration of GAP event types 00028 */ 00029 struct GapEventType : SafeEnum<GapEventType, uint8_t> { 00030 enum type { 00031 /** 00032 * Event type used by GapUnexpectedErrorEvent 00033 */ 00034 UNEXPECTED_ERROR, 00035 00036 /** 00037 * Event type used by GapConnectionCompleteEvent 00038 */ 00039 CONNECTION_COMPLETE, 00040 00041 /** 00042 * Event type used by GapAdvertisingReportEvent 00043 */ 00044 ADVERTISING_REPORT, 00045 00046 /** 00047 * Event type used by GapConnectionUpdateEvent 00048 * */ 00049 CONNECTION_UPDATE, 00050 00051 /** 00052 * Event type used by GapRemoteConnectionParameterRequestEvent 00053 */ 00054 REMOTE_CONNECTION_PARAMETER_REQUEST, 00055 00056 /** 00057 * Event type used by GapDisconnectionCompleteEvent 00058 */ 00059 DISCONNECTION_COMPLETE 00060 }; 00061 00062 GapEventType(type event_type) : SafeEnum<GapEventType, uint8_t>(event_type) { } 00063 }; 00064 00065 00066 /** 00067 * Base class of a Gap Event. 00068 * 00069 * Client should use the field type to deduce the actual type of the event. 00070 */ 00071 struct GapEvent { 00072 00073 const GapEventType type; 00074 00075 protected: 00076 GapEvent(GapEventType type) : type(type) { } 00077 00078 // Disable copy construction and copy assignement operations. 00079 GapEvent(const GapEvent&); 00080 GapEvent& operator=(const GapEvent&); 00081 }; 00082 00083 00084 /** 00085 * Model an unexpected error that happen during a gap procedure. 00086 * 00087 * This class is mainly used to notify user code of an unexpected error returned 00088 * in an HCI command complete event. 00089 */ 00090 struct GapUnexpectedErrorEvent : public GapEvent { 00091 GapUnexpectedErrorEvent(uint16_t opcode, uint8_t error_code) : 00092 GapEvent(GapEventType::UNEXPECTED_ERROR), 00093 opcode(opcode), error_code(error_code) { } 00094 00095 /** 00096 * Opcode composed of the OCF and OGF of the command which has returned an 00097 * error. 00098 */ 00099 const uint16_t opcode; 00100 00101 /** 00102 * Error code 00103 */ 00104 const uint8_t error_code; 00105 }; 00106 00107 00108 /** 00109 * Indicate to both ends (slave or master) the end of the connection process. 00110 * 00111 * This structure should be used for Connection Complete Events and Enhanced 00112 * Connection Complete Event. 00113 * 00114 * @note: See Bluetooth 5 Vol 2 PartE: 7.7.65.1 LE Connection Complete Event 00115 * @note: See Bluetooth 5 Vol 2 PartE: 7.7.65.10 LE Enhanced Connection 00116 */ 00117 struct GapConnectionCompleteEvent : public GapEvent { 00118 /** 00119 * Construct a new GapConnectionCompleteEvent. 00120 * 00121 * @param status Status of the operation: 0x00 in case of success otherwise 00122 * the error code associated with the failure. 00123 * 00124 * @param connection_handle handle of the connection created. This handle 00125 * will be used to address the connection in any connection oriented 00126 * operation. 00127 * 00128 * @param role Role of the LE subsystem in the connection. 00129 * 00130 * @param address_type Type of address used by the peer for this connection. 00131 * 00132 * @param address Address of the peer used to establish the connection. 00133 * 00134 * @param connection_interval Connection interval used on this connection. 00135 * It shall be in a range [0x0006 : 0x0C80]. A unit is equal to 1.25ms. 00136 * 00137 * @param connection_latency Number of connection events the slave can 00138 * drop. 00139 * 00140 * @param supervision_timeout Supervision timeout of the connection. It 00141 * shall be in the range [0x000A : 0x0C80] where a unit represent 10ms. 00142 * 00143 * @note: See Bluetooth 5 Vol 2 PartE: 7.7.65.1 LE Connection Complete Event 00144 * @note: See Bluetooth 5 Vol 2 PartE: 7.7.65.10 LE Enhanced Connection 00145 * Complete Event 00146 */ 00147 GapConnectionCompleteEvent( 00148 uint8_t status, 00149 connection_handle_t connection_handle, 00150 connection_role_t role, 00151 advertising_peer_address_type_t peer_address_type, 00152 const address_t& peer_address, 00153 uint16_t connection_interval, 00154 uint16_t connection_latency, 00155 uint16_t supervision_timeout 00156 ) : 00157 GapEvent(GapEventType::CONNECTION_COMPLETE), 00158 status(status), 00159 connection_handle(connection_handle), 00160 role(role), 00161 peer_address_type(peer_address_type), 00162 peer_address(peer_address), 00163 connection_interval(connection_interval), 00164 connection_latency(connection_latency), 00165 supervision_timeout(supervision_timeout) { 00166 } 00167 00168 /* 00169 * @param status Indicate if the connection succesfully completed or not: 00170 * - 0: Connection successfuly completed 00171 * - [0x01 : 0xFF] Connection failed to complete, the value represent 00172 * the code for the error. 00173 */ 00174 const uint8_t status; 00175 00176 /** 00177 * Handle of the connection created, valid if status is equal to 0. 00178 * @important Valid if status is equal to 0. 00179 */ 00180 const connection_handle_t connection_handle; 00181 00182 /** 00183 * Role of the device in the connection 00184 * @important Valid if status is equal to 0. 00185 */ 00186 const connection_role_t role; 00187 00188 /** 00189 * Peer address type. 00190 */ 00191 const advertising_peer_address_type_t peer_address_type; 00192 00193 /** 00194 * Peer address. 00195 */ 00196 const address_t peer_address; 00197 00198 /** 00199 * Connection interval used in this connection. 00200 * It shall be in a range [0x0006 : 0x0C80]. A unit is equal to 1.25ms. 00201 */ 00202 const uint16_t connection_interval; 00203 00204 /** 00205 * Number of connection events the slave can drop. 00206 */ 00207 const uint16_t connection_latency; 00208 00209 /** 00210 * Supervision timeout of the connection 00211 * It shall be in the range [0x000A : 0x0C80] where a unit represent 10ms. 00212 */ 00213 const uint16_t supervision_timeout; 00214 }; 00215 00216 00217 /** 00218 * Report advertising from one or more LE device. 00219 * 00220 * @important This class has to be implemented by the BLE port. 00221 * 00222 * @note: See Bluetooth 5 Vol 2 PartE: 7.7.65.2 LE Advertising Report Event 00223 */ 00224 struct GapAdvertisingReportEvent : public GapEvent { 00225 00226 /** 00227 * POD representing an advertising captured by the LE subsystem. 00228 */ 00229 struct advertising_t { 00230 received_advertising_type_t type; 00231 connection_peer_address_type_t address_type; 00232 const address_t& address; 00233 ArrayView<const uint8_t> data; 00234 int8_t rssi; 00235 }; 00236 00237 GapAdvertisingReportEvent() : GapEvent(GapEventType::ADVERTISING_REPORT) { } 00238 00239 virtual ~GapAdvertisingReportEvent() { } 00240 00241 /** 00242 * Count of advertising in this event. 00243 */ 00244 virtual uint8_t size() const = 0; 00245 00246 /** 00247 * Access the advertising at index i. 00248 */ 00249 virtual advertising_t operator[](uint8_t i) const = 0; 00250 }; 00251 00252 00253 /** 00254 * Indicates the connection update process completion. 00255 * 00256 * If no parameters are updated after a connection update request from the peer 00257 * then this event shall not be emmited. 00258 * 00259 * @note: See Bluetooth 5 Vol 2 PartE: 7.7.65.3 LE Connection Update Complete 00260 * Event. 00261 */ 00262 struct GapConnectionUpdateEvent : public GapEvent { 00263 00264 /** 00265 * Construct a connection update event for a successful process. 00266 * 00267 * @param status Status of the connection update event operation. If equal 00268 * to 0x00 then the process was successful, otherwise the status indicates 00269 * the reason of the faillure. 00270 * 00271 * @param connection_handle Handle of the connection updated. 00272 * 00273 * @param connection_interval New connection interval used by the connection. 00274 * 00275 * @param Connection_latency New connection latency used by the connection. 00276 * 00277 * @param supervision_timeout New connection supervision timeout. 00278 */ 00279 GapConnectionUpdateEvent( 00280 uint8_t status, 00281 connection_handle_t connection_handle, 00282 uint16_t connection_interval, 00283 uint16_t connection_latency, 00284 uint16_t supervision_timeout 00285 ) : 00286 GapEvent(GapEventType::CONNECTION_UPDATE), 00287 status(status), 00288 connection_handle(connection_handle), 00289 connection_interval(connection_interval), 00290 connection_latency(connection_latency), 00291 supervision_timeout(supervision_timeout) { 00292 } 00293 00294 /** 00295 * If equal to 0, the connection update has succesfully completed otherwise 00296 * the process has failled and this field represent the error associated to 00297 * the faillure. 00298 */ 00299 const uint8_t status; 00300 00301 /** 00302 * Handle of the connection which has completed the connection update 00303 * process. 00304 */ 00305 const connection_handle_t connection_handle; 00306 00307 /** 00308 * New connection interval used by the connection. 00309 * It shall be in a range [0x0006 : 0x0C80]. A unit is equal to 1.25ms. 00310 */ 00311 const uint16_t connection_interval; 00312 00313 /* 00314 * New number of connection events the slave can drop. 00315 */ 00316 const uint16_t connection_latency; 00317 00318 /* 00319 * New supervision timeout of the connection. 00320 * It shall be in the range [0x000A : 0x0C80] where a unit represent 10ms. 00321 */ 00322 const uint16_t supervision_timeout; 00323 }; 00324 00325 00326 /** 00327 * indicate a request from the peer to change the connection parameters. 00328 * 00329 * @note: See Bluetooth 5 Vol 2 PartE: 7.7.65.6 LE Remote Connection Parameter 00330 * Request Event. 00331 */ 00332 struct GapRemoteConnectionParameterRequestEvent : public GapEvent { 00333 /** 00334 * Construct a new remote connection parameter request event. 00335 * 00336 * @param connection_handle Handle of the connection with the peer 00337 * requesting the parameter update 00338 * 00339 * @param min_connection_interval Minimum value of the connection interval 00340 * requested by the peer. 00341 * 00342 * @param max_connection_interval Maximum value of the connection interval 00343 * requested by the peer. 00344 * 00345 * @param connection_latency Slave latency requested by the peer. 00346 * 00347 * @param supervision_timeout Supervision timeout requested by the peer. 00348 */ 00349 GapRemoteConnectionParameterRequestEvent( 00350 connection_handle_t connection_handle, 00351 uint16_t min_connection_interval, 00352 uint16_t max_connection_interval, 00353 uint16_t connection_latency, 00354 uint16_t supervision_timeout 00355 ) : GapEvent(GapEventType::REMOTE_CONNECTION_PARAMETER_REQUEST), 00356 connection_handle(connection_handle), 00357 min_connection_interval(min_connection_interval), 00358 max_connection_interval(max_connection_interval), 00359 connection_latency(connection_latency), 00360 supervision_timeout(supervision_timeout) { 00361 } 00362 00363 /** 00364 * Handle of the connection with the peer requesting the parameter update. 00365 */ 00366 const connection_handle_t connection_handle; 00367 00368 /** 00369 * Minimum value of the connection interval requested by the peer. 00370 * It shall be in a range [0x0006 : 0x0C80]. A unit is equal to 1.25ms. 00371 */ 00372 const uint16_t min_connection_interval; 00373 00374 /** 00375 * Maximum value of the connection interval requested by the peer. 00376 * It shall be in a range [0x0006 : 0x0C80]. A unit is equal to 1.25ms. 00377 */ 00378 const uint16_t max_connection_interval; 00379 00380 /* 00381 * Slave latency requested by the peer. 00382 */ 00383 const uint16_t connection_latency; 00384 00385 /* 00386 * Supervision timeout requested by the peer. 00387 * It shall be in the range [0x000A : 0x0C80] where a unit represent 10ms. 00388 */ 00389 const uint16_t supervision_timeout; 00390 }; 00391 00392 00393 /** 00394 * Indicate the end of a disconnection process. 00395 * 00396 * @note: See Bluetooth 5 Vol 2 PartE: 7.7.5 Disconnection Complete Event. 00397 */ 00398 struct GapDisconnectionCompleteEvent : public GapEvent { 00399 /** 00400 * Construct a disconnection complete event. 00401 * 00402 * @param status Status of the procedure. If equal to 0 then the 00403 * disconnection process complete successfully. Otherwise it represents the 00404 * error code associated with the faillure. 00405 * 00406 * @param connection_handle Handle of the connection disconnected. 00407 * 00408 * @param reason Reason of the disconnection 00409 */ 00410 GapDisconnectionCompleteEvent( 00411 uint8_t status, 00412 connection_handle_t connection_handle, 00413 uint8_t reason 00414 ) : GapEvent(GapEventType::DISCONNECTION_COMPLETE), 00415 status(status), 00416 connection_handle(connection_handle), 00417 reason(reason) { 00418 } 00419 00420 /** 00421 * Status of the procedure. If equal to 0 then the procedure was a success 00422 * otherwise this variable contains the error code associated with the 00423 * faillure. 00424 */ 00425 const uint8_t status; 00426 00427 /** 00428 * Handle of the connection used for the procedure. 00429 */ 00430 const connection_handle_t connection_handle; 00431 00432 /** 00433 * Reason for disconnection. 00434 * 00435 * @important ignored in case of faillure. 00436 */ 00437 const uint8_t reason; 00438 }; 00439 00440 } // namespace pal 00441 } // namespace ble 00442 00443 #endif /* BLE_PAL_GAP_MESSAGE_H_ */
Generated on Sun Jul 17 2022 08:25:23 by
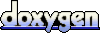