
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
page_allocator_config.h
00001 /* 00002 * Copyright (c) 2016, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 #ifndef __PAGE_ALLOCATOR_CONFIG_H__ 00019 #define __PAGE_ALLOCATOR_CONFIG_H__ 00020 /* This file can be compiled externally to provide the page allocator algorithm 00021 * for devices NOT supported by uVisor. For this purpose this file is copied as 00022 * is into the target build folder and compiled by the target build system. */ 00023 00024 /* We can only protect a small number of pages efficiently, so there should be 00025 * a relatively low limit to the number of pages. 00026 * By default a maximum of 16 pages are allowed. This can only be overwritten 00027 * by the porting engineer for the current platform. */ 00028 #ifndef UVISOR_PAGE_MAX_COUNT 00029 #define UVISOR_PAGE_MAX_COUNT (16UL) 00030 #endif 00031 /* The number of pages is decided by the page size. A small page size leads to 00032 * a lot of pages, however, number of pages is capped for efficiency. 00033 * Furthermore, when allocating large continous memory, a too small page size 00034 * will lead to allocation failures. This can only be overwritten 00035 * by the porting engineer for the current platform. */ 00036 #ifndef UVISOR_PAGE_SIZE_MINIMUM 00037 #define UVISOR_PAGE_SIZE_MINIMUM (1024UL) 00038 #endif 00039 00040 /* Defines the number of uint32_t page owner masks in the owner map. 00041 * +8 is used for ARMv7-M MPUs, where a shift of up to 7-bits may be required 00042 * to align MPU regions. */ 00043 #define UVISOR_PAGE_MAP_COUNT ((UVISOR_PAGE_MAX_COUNT + 31 + 8) / 32) 00044 00045 /* The page box_id is the box id which is 8-bit large. */ 00046 typedef uint8_t page_owner_t; 00047 /* Contains the total number of available pages. */ 00048 extern uint8_t g_page_count_total; 00049 /* Contains the shift of the page owner mask. */ 00050 extern uint8_t g_page_map_shift; 00051 /* Contains the ARMv7-MPU rounded page end. */ 00052 extern uint32_t g_page_head_end_rounded; 00053 00054 /** Sets the page bit in the page map array. 00055 * @param map an array of `uint32_t` containing the page map 00056 * @param page the index of the page to be set 00057 */ 00058 static inline void page_allocator_map_set(uint32_t * const map, uint8_t page) 00059 { 00060 page += g_page_map_shift; 00061 map[page / 32] |= (1UL << (page % 32)); 00062 } 00063 00064 /** Clears the page bit in the page map array. 00065 * @param map an array of `uint32_t` containing the page map 00066 * @param page the index of the page to be set 00067 */ 00068 static inline void page_allocator_map_clear(uint32_t * const map, uint8_t page) 00069 { 00070 page += g_page_map_shift; 00071 map[page / 32] &= ~(1UL << (page % 32)); 00072 } 00073 00074 /** Check if the page bit is set int the page map array. 00075 * @param map an array of `uint32_t` containing the page map 00076 * @param page the index of the page to be set 00077 * @retval 0 if page bit is not set 00078 * @retval 1 if page bit is set 00079 */ 00080 static inline int page_allocator_map_get(const uint32_t * const map, uint8_t page) 00081 { 00082 page += g_page_map_shift; 00083 return (map[page / 32] >> (page % 32)) & 0x1; 00084 } 00085 00086 #endif /* __PAGE_ALLOCATOR_CONFIG_H__ */
Generated on Sun Jul 17 2022 08:25:29 by
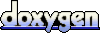