
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
pack_manager.py
00001 import argparse 00002 from os.path import basename 00003 from tools.arm_pack_manager import Cache 00004 from os.path import basename, join, dirname, exists 00005 from os import makedirs 00006 from itertools import takewhile 00007 from fuzzywuzzy import process 00008 from tools.arm_pack_manager import Cache 00009 00010 parser = argparse.ArgumentParser(description='A Handy little utility for keeping your cache of pack files up to date.') 00011 subparsers = parser.add_subparsers(title="Commands") 00012 00013 def subcommand(name, *args, **kwargs): 00014 def subcommand(command): 00015 subparser = subparsers.add_parser(name, **kwargs) 00016 00017 for arg in args: 00018 arg = dict(arg) 00019 opt = arg['name'] 00020 del arg['name'] 00021 00022 if isinstance(opt, basestring): 00023 subparser.add_argument(opt, **arg) 00024 else: 00025 subparser.add_argument(*opt, **arg) 00026 00027 subparser.add_argument("-v", "--verbose", action="store_true", dest="verbose", help="Verbose diagnostic output") 00028 subparser.add_argument("-vv", "--very_verbose", action="store_true", dest="very_verbose", help="Very verbose diagnostic output") 00029 subparser.add_argument("--no-timeouts", action="store_true", help="Remove all timeouts and try to download unconditionally") 00030 subparser.add_argument("--and", action="store_true", dest="intersection", help="combine search terms as if with an and") 00031 subparser.add_argument("--or", action="store_false", dest="intersection", help="combine search terms as if with an or") 00032 subparser.add_argument("--union", action="store_false", dest="intersection", help="combine search terms as if with a set union") 00033 subparser.add_argument("--intersection", action="store_true", dest="intersection", help="combine search terms as if with a set intersection") 00034 00035 def thunk(parsed_args): 00036 cache = Cache(not parsed_args.verbose, parsed_args.no_timeouts) 00037 argv = [arg['dest'] if 'dest' in arg else arg['name'] for arg in args] 00038 argv = [(arg if isinstance(arg, basestring) else arg[-1]).strip('-') 00039 for arg in argv] 00040 argv = {arg: vars(parsed_args)[arg] for arg in argv 00041 if vars(parsed_args)[arg] is not None} 00042 00043 return command(cache, **argv) 00044 00045 subparser.set_defaults(command=thunk) 00046 return command 00047 return subcommand 00048 00049 def user_selection (message, options) : 00050 print(message) 00051 for choice, index in zip(options, range(len(options))) : 00052 print("({}) {}".format(index, choice)) 00053 pick = None 00054 while pick is None : 00055 stdout.write("please select an integer from 0 to {} or \"all\"".format(len(options)-1)) 00056 input = raw_input() 00057 try : 00058 if input == "all" : 00059 pick = options 00060 else : 00061 pick = [options[int(input)]] 00062 except ValueError : 00063 print("I did not understand your input") 00064 return pick 00065 00066 def fuzzy_find(matches, urls) : 00067 choices = {} 00068 for match in matches : 00069 for key, value in process.extract(match, urls, limit=None) : 00070 choices.setdefault(key, 0) 00071 choices[key] += value 00072 choices = sorted([(v, k) for k, v in choices.iteritems()], reverse=True) 00073 if not choices : return [] 00074 elif len(choices) == 1 : return [choices[0][1]] 00075 elif choices[0][0] > choices[1][0] : choices = choices[:1] 00076 else : choices = list(takewhile(lambda t: t[0] == choices[0][0], choices)) 00077 return [v for k,v in choices] 00078 00079 @subcommand('cache', 00080 dict(name='matches', nargs="*", 00081 help="a bunch of things to search for in part names"), 00082 dict(name=['-e','--everything'], action="store_true", 00083 help="download everything possible"), 00084 dict(name=['-d','--descriptors'], action="store_true", 00085 help="download all descriptors"), 00086 dict(name=["-b","--batch"], action="store_true", 00087 help="don't ask for user input and assume download all"), 00088 help="Cache a group of PACK or PDSC files") 00089 def command_cache (cache, matches, everything=False, descriptors=False, batch=False, verbose= False, intersection=True) : 00090 if everything : 00091 cache.cache_everything() 00092 return True 00093 if descriptors : 00094 cache.cache_descriptors() 00095 return True 00096 if not matches : 00097 print("No action specified nothing to do") 00098 else : 00099 urls = cache.get_urls() 00100 if intersection : 00101 choices = fuzzy_find(matches, map(basename, urls)) 00102 else : 00103 choices = sum([fuzzy_find([m], map(basename, urls)) for m in matches], []) 00104 if not batch and len(choices) > 1 : 00105 choices = user_selection("Please select a file to cache", choices) 00106 to_download = [] 00107 for choice in choices : 00108 for url in urls : 00109 if choice in url : 00110 to_download.append(url) 00111 cache.cache_pack_list(to_download) 00112 return True 00113 00114 00115 @subcommand('find-part', 00116 dict(name='matches', nargs="+", help="words to match to processors"), 00117 dict(name=['-l',"--long"], action="store_true", 00118 help="print out part details with part"), 00119 dict(name=['-p', '--parts-only'], action="store_false", dest="print_aliases"), 00120 dict(name=['-a', '--aliases-only'], action="store_false", dest="print_parts"), 00121 help="Find a Part and it's description within the cache") 00122 def command_find_part (cache, matches, long=False, intersection=True, 00123 print_aliases=True, print_parts=True) : 00124 if long : 00125 import pprint 00126 pp = pprint.PrettyPrinter() 00127 parts = cache.index 00128 if intersection : 00129 choices = fuzzy_find(matches, parts.keys()) 00130 aliases = fuzzy_find(matches, cache.aliases.keys()) 00131 else : 00132 choices = sum([fuzzy_find([m], parts.keys()) for m in matches], []) 00133 aliases = sum([fuzzy_find([m], cache.aliases.keys()) for m in matches], []) 00134 if print_parts: 00135 for part in choices : 00136 print part 00137 if long : 00138 pp.pprint(cache.index[part]) 00139 if print_aliases: 00140 for alias in aliases : 00141 print alias 00142 if long : 00143 pp.pprint(cache.index[cache.aliases[alias]]) 00144 00145 @subcommand('dump-parts', 00146 dict(name='out', help='directory to dump to'), 00147 dict(name='parts', nargs='+', help='parts to dump'), 00148 help='Create a directory with an index.json describing the part and all of their associated flashing algorithms.' 00149 ) 00150 def command_dump_parts (cache, out, parts, intersection=False) : 00151 index = {} 00152 if intersection : 00153 for part in fuzzy_find(parts, cache.index): 00154 index.update(cache.index[part]) 00155 else : 00156 for part in parts : 00157 index.update(dict(cache.find_device(part))) 00158 for n, p in index.iteritems() : 00159 try : 00160 if not exists(join(out, dirname(p['algorithm']['file']))) : 00161 makedirs(join(out, dirname(p['algorithm']['file']))) 00162 with open(join(out, p['algorithm']['file']), "wb+") as fd : 00163 fd.write(cache.get_flash_algorthim_binary(n).read()) 00164 except KeyError: 00165 print("[Warning] {} does not have an associated flashing algorithm".format(n)) 00166 with open(join(out, "index.json"), "wb+") as fd : 00167 dump(index,fd) 00168 00169 00170 @subcommand('cache-part', 00171 dict(name='matches', nargs="+", help="words to match to devices"), 00172 help='Cache PACK files associated with the parts matching the provided words') 00173 def command_cache_part (cache, matches, intersection=True) : 00174 index = cache.index 00175 if intersection : 00176 choices = fuzzy_find(matches, index.keys()) 00177 aliases = fuzzy_find(matches, cache.aliases.keys()) 00178 else : 00179 choices = sum([fuzzy_find([m], index.keys()) for m in matches], []) 00180 aliases = sum([fuzzy_find([m], cache.aliases.keys()) for m in matches], []) 00181 urls = set([index[c]['pdsc_file'] for c in choices]) 00182 urls += set([index[cache.aliasse[a]] for a in aliases]) 00183 cache.cache_pack_list(list(urls)) 00184 00185 def get_argparse() : 00186 return parser 00187 00188 def main() : 00189 args = parser.parse_args() 00190 args.command(args) 00191
Generated on Sun Jul 17 2022 08:25:29 by
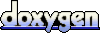