
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
open_stub.c
00001 #include <stdbool.h> 00002 #include <sys/types.h> 00003 #include <sys/stat.h> 00004 #include <fcntl.h> 00005 #include <stdarg.h> 00006 00007 bool allow_open = true; 00008 int __real_open(const char *path, int flags, ...); 00009 int __wrap_open(const char *path, int flags, ...) 00010 { 00011 if (allow_open) { 00012 if (flags & O_CREAT) { 00013 va_list vl; 00014 va_start(vl,flags); 00015 mode_t mode = va_arg(vl, mode_t); 00016 va_end(vl); 00017 return __real_open(path, flags, mode); 00018 } else { 00019 return __real_open(path, flags); 00020 } 00021 } else { 00022 return -1; 00023 } 00024 }
Generated on Sun Jul 17 2022 08:25:29 by
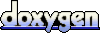