
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
nwk_stats_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2013-2017 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 #ifndef NWK_STATS_API_H 00015 #define NWK_STATS_API_H 00016 /** 00017 * \file nwk_stats_api.h 00018 * \brief 6LowPAN library network stats API 00019 * 00020 * - protocol_stats_start(), Enable stats update. 00021 * - protocol_stats_stop(), Stop stack stats update. 00022 * - protocol_stats_reset(), Reset all stats information to null. 00023 * 00024 * 00025 */ 00026 00027 #include "ns_types.h" 00028 00029 #ifdef __cplusplus 00030 extern "C" { 00031 #endif 00032 00033 /** 00034 * /struct nwk_stats_t 00035 * /brief Struct for network stats buffer structure. 00036 */ 00037 typedef struct nwk_stats_t { 00038 /*mac stats*/ 00039 uint16_t mac_tx_buffer_overflow; /**< MAC TX queue overflow count. */ 00040 uint16_t mac_tx_queue_size; /**< MAC TX queue current size. */ 00041 uint16_t mac_tx_queue_peak; /**< MAC TX queue peak size. */ 00042 uint32_t mac_rx_count; /**< MAC RX packet count. */ 00043 uint32_t mac_tx_count; /**< MAC TX packet count. */ 00044 uint32_t mac_bc_tx_count; /**< MAC broadcast TX packet count. */ 00045 uint32_t mac_rx_drop; /**< MAC RX packet drop count. */ 00046 /* Mac Payload Flow */ 00047 uint32_t mac_tx_bytes; /**< MAC TX bytes count. */ 00048 uint32_t mac_rx_bytes; /**< MAC RX bytes count. */ 00049 uint32_t mac_tx_failed; /**< MAC TX failed count. */ 00050 uint32_t mac_tx_retry; /**< MAC TX retry count. */ 00051 uint32_t mac_tx_cca_cnt; /**< MAC TX CCA count. */ 00052 uint32_t mac_tx_failed_cca; /**< MAC failed CCA count. */ 00053 uint32_t mac_security_drop; /**< MAC security packet drops count. */ 00054 /* 6Lowpan */ 00055 uint32_t ip_rx_count; /**< IP RX packet count. */ 00056 uint32_t ip_tx_count; /**< IP TX packet count. */ 00057 uint32_t ip_rx_drop; /**< IP RX packet drops count. */ 00058 uint32_t ip_cksum_error; /**< IP checksum error count. */ 00059 /* IP Payload Flow */ 00060 uint32_t ip_tx_bytes; /**< IP TX bytes count. */ 00061 uint32_t ip_rx_bytes; /**< IP RX bytes count. */ 00062 uint32_t ip_routed_up; /**< IP routed UP bytes count. */ 00063 uint32_t ip_no_route; /**< IP no route count. */ 00064 /* Fragments */ 00065 uint32_t frag_rx_errors; /**< Fragmentation RX error count. */ 00066 uint32_t frag_tx_errors; /**< Fragmentation TX error count. */ 00067 /*RPL stats*/ 00068 uint32_t rpl_route_routecost_better_change; /**< RPL parent change count. */ 00069 uint32_t ip_routeloop_detect; /**< RPL route loop detection count. */ 00070 uint32_t rpl_memory_overflow; /**< RPL memory overflow count. */ 00071 uint32_t rpl_parent_tx_fail; /**< RPL transmit errors to DODAG parents. */ 00072 uint32_t rpl_unknown_instance; /**< RPL unknown instance ID count. */ 00073 uint32_t rpl_local_repair; /**< RPL local repair count. */ 00074 uint32_t rpl_global_repair; /**< RPL global repair count. */ 00075 uint32_t rpl_malformed_message; /**< RPL malformed message count. */ 00076 uint32_t rpl_time_no_next_hop; /**< RPL seconds without a next hop. */ 00077 uint32_t rpl_total_memory; /**< RPL current memory usage total. */ 00078 /* Buffers */ 00079 uint32_t buf_alloc; /**< Buffer allocation count. */ 00080 uint32_t buf_headroom_realloc; /**< Buffer headroom realloc count. */ 00081 uint32_t buf_headroom_shuffle; /**< Buffer headroom shuffle count. */ 00082 uint32_t buf_headroom_fail; /**< Buffer headroom failure count. */ 00083 /* ETX */ 00084 uint16_t etx_1st_parent; /**< Primary parent ETX. */ 00085 uint16_t etx_2nd_parent; /**< Secondary parent ETX. */ 00086 00087 } nwk_stats_t; 00088 00089 /** 00090 * \brief Enable stats collection. 00091 * 00092 * \param stats_ptr A static pointer to stack update. 00093 * 00094 */ 00095 extern void protocol_stats_start(nwk_stats_t *stats_ptr); 00096 /** 00097 * \brief Disable stats collection. 00098 * 00099 */ 00100 extern void protocol_stats_stop(void); 00101 /** 00102 * \brief Reset stats info fiels. 00103 * 00104 */ 00105 extern void protocol_stats_reset(void); 00106 #ifdef __cplusplus 00107 } 00108 #endif 00109 #endif
Generated on Sun Jul 17 2022 08:25:29 by
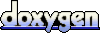