
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
nsapi_types.h
00001 00002 /** \addtogroup netsocket */ 00003 /** @{*/ 00004 /* nsapi.h - The network socket API 00005 * Copyright (c) 2015 ARM Limited 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); 00008 * you may not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, 00015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 */ 00019 00020 #ifndef NSAPI_TYPES_H 00021 #define NSAPI_TYPES_H 00022 00023 #include <stdint.h> 00024 00025 #ifdef __cplusplus 00026 extern "C" { 00027 #endif 00028 00029 00030 /** Enum of standardized error codes 00031 * 00032 * Valid error codes have negative values and may 00033 * be returned by any network operation. 00034 * 00035 * @enum nsapi_error 00036 */ 00037 enum nsapi_error { 00038 NSAPI_ERROR_OK = 0, /*!< no error */ 00039 NSAPI_ERROR_WOULD_BLOCK = -3001, /*!< no data is not available but call is non-blocking */ 00040 NSAPI_ERROR_UNSUPPORTED = -3002, /*!< unsupported functionality */ 00041 NSAPI_ERROR_PARAMETER = -3003, /*!< invalid configuration */ 00042 NSAPI_ERROR_NO_CONNECTION = -3004, /*!< not connected to a network */ 00043 NSAPI_ERROR_NO_SOCKET = -3005, /*!< socket not available for use */ 00044 NSAPI_ERROR_NO_ADDRESS = -3006, /*!< IP address is not known */ 00045 NSAPI_ERROR_NO_MEMORY = -3007, /*!< memory resource not available */ 00046 NSAPI_ERROR_NO_SSID = -3008, /*!< ssid not found */ 00047 NSAPI_ERROR_DNS_FAILURE = -3009, /*!< DNS failed to complete successfully */ 00048 NSAPI_ERROR_DHCP_FAILURE = -3010, /*!< DHCP failed to complete successfully */ 00049 NSAPI_ERROR_AUTH_FAILURE = -3011, /*!< connection to access point failed */ 00050 NSAPI_ERROR_DEVICE_ERROR = -3012, /*!< failure interfacing with the network processor */ 00051 NSAPI_ERROR_IN_PROGRESS = -3013, /*!< operation (eg connect) in progress */ 00052 NSAPI_ERROR_ALREADY = -3014, /*!< operation (eg connect) already in progress */ 00053 NSAPI_ERROR_IS_CONNECTED = -3015, /*!< socket is already connected */ 00054 NSAPI_ERROR_CONNECTION_LOST = -3016, /*!< connection lost */ 00055 NSAPI_ERROR_CONNECTION_TIMEOUT = -3017, /*!< connection timed out */ 00056 NSAPI_ERROR_ADDRESS_IN_USE = -3018, /*!< Address already in use */ 00057 }; 00058 00059 /** Type used to represent error codes 00060 * 00061 * This is a separate type from enum nsapi_error to avoid breaking 00062 * compatibility in type-sensitive overloads 00063 */ 00064 typedef signed int nsapi_error_t; 00065 00066 /** Type used to represent the size of data passed through sockets 00067 */ 00068 typedef unsigned int nsapi_size_t; 00069 00070 /** Type used to represent either a size or error pased through sockets 00071 * 00072 * A valid nsapi_size_or_error_t is either a non-negative size or a 00073 * negative error code from the nsapi_error_t 00074 */ 00075 typedef signed int nsapi_size_or_error_t; 00076 00077 /** Enum of encryption types 00078 * 00079 * The security type specifies a particular security to use when 00080 * connected to a WiFi network 00081 */ 00082 typedef enum nsapi_security { 00083 NSAPI_SECURITY_NONE = 0x0, /*!< open access point */ 00084 NSAPI_SECURITY_WEP = 0x1, /*!< phrase conforms to WEP */ 00085 NSAPI_SECURITY_WPA = 0x2, /*!< phrase conforms to WPA */ 00086 NSAPI_SECURITY_WPA2 = 0x3, /*!< phrase conforms to WPA2 */ 00087 NSAPI_SECURITY_WPA_WPA2 = 0x4, /*!< phrase conforms to WPA/WPA2 */ 00088 NSAPI_SECURITY_PAP = 0x5, /*!< phrase conforms to PPP authentication context */ 00089 NSAPI_SECURITY_CHAP = 0x6, /*!< phrase conforms to PPP authentication context */ 00090 NSAPI_SECURITY_UNKNOWN = 0xFF, /*!< unknown/unsupported security in scan results */ 00091 } nsapi_security_t; 00092 00093 /** Maximum size of IP address representation 00094 */ 00095 #define NSAPI_IP_SIZE NSAPI_IPv6_SIZE 00096 00097 /** Maximum number of bytes for IP address 00098 */ 00099 #define NSAPI_IP_BYTES NSAPI_IPv6_BYTES 00100 00101 /** Maximum size of MAC address representation 00102 */ 00103 #define NSAPI_MAC_SIZE 18 00104 00105 /** Maximum number of bytes for MAC address 00106 */ 00107 #define NSAPI_MAC_BYTES 6 00108 00109 /** Size of IPv4 representation 00110 */ 00111 #define NSAPI_IPv4_SIZE 16 00112 00113 /** Number of bytes in IPv4 address 00114 */ 00115 #define NSAPI_IPv4_BYTES 4 00116 00117 /** Size of IPv6 representation 00118 */ 00119 #define NSAPI_IPv6_SIZE 40 00120 00121 /** Number of bytes in IPv6 address 00122 */ 00123 #define NSAPI_IPv6_BYTES 16 00124 00125 /** Enum of IP address versions 00126 * 00127 * The IP version specifies the type of an IP address. 00128 * 00129 * @enum nsapi_version 00130 */ 00131 typedef enum nsapi_version { 00132 NSAPI_UNSPEC , /*!< Address is unspecified */ 00133 NSAPI_IPv4 , /*!< Address is IPv4 */ 00134 NSAPI_IPv6 , /*!< Address is IPv6 */ 00135 } nsapi_version_t; 00136 00137 /** IP address structure for passing IP addresses by value 00138 */ 00139 typedef struct nsapi_addr { 00140 /** IP version 00141 * - NSAPI_IPv4 00142 * - NSAPI_IPv6 00143 * - NSAPI_UNSPEC 00144 */ 00145 nsapi_version_t version; 00146 00147 /** IP address 00148 * The raw bytes of the IP address stored in big-endian format 00149 */ 00150 uint8_t bytes[NSAPI_IP_BYTES]; 00151 } nsapi_addr_t; 00152 00153 00154 /** Opaque handle for network sockets 00155 */ 00156 typedef void *nsapi_socket_t; 00157 00158 00159 /** Enum of socket protocols 00160 * 00161 * The socket protocol specifies a particular protocol to 00162 * be used with a newly created socket. 00163 * 00164 * @enum nsapi_protocol 00165 */ 00166 typedef enum nsapi_protocol { 00167 NSAPI_TCP , /*!< Socket is of TCP type */ 00168 NSAPI_UDP , /*!< Socket is of UDP type */ 00169 } nsapi_protocol_t; 00170 00171 /** Enum of standardized stack option levels 00172 * for use with NetworkStack::setstackopt and getstackopt. 00173 * 00174 * @enum nsapi_stack_level 00175 */ 00176 typedef enum nsapi_stack_level { 00177 NSAPI_STACK = 5000, /*!< Stack option level - see nsapi_stack_option_t for options */ 00178 } nsapi_stack_level_t; 00179 00180 /** Enum of standardized stack option names for level NSAPI_STACK 00181 * of NetworkStack::setstackopt and getstackopt. 00182 * 00183 * These options may not be supported on all stacks, in which 00184 * case NSAPI_ERROR_UNSUPPORTED may be returned. 00185 * 00186 * @enum nsapi_stack_option 00187 */ 00188 typedef enum nsapi_stack_option { 00189 NSAPI_IPV4_MRU , /*!< Sets/gets size of largest IPv4 fragmented datagram to reassemble */ 00190 NSAPI_IPV6_MRU , /*!< Sets/gets size of largest IPv6 fragmented datagram to reassemble */ 00191 } nsapi_stack_option_t; 00192 00193 /** Enum of standardized socket option levels 00194 * for use with Socket::setsockopt and getsockopt. 00195 * 00196 * @enum nsapi_socket_level 00197 */ 00198 typedef enum nsapi_socket_level { 00199 NSAPI_SOCKET = 7000, /*!< Socket option level - see nsapi_socket_option_t for options */ 00200 } nsapi_socket_level_t; 00201 00202 /** Enum of standardized socket option names for level NSAPI_SOCKET 00203 * of Socket::setsockopt and getsockopt. 00204 * 00205 * These options may not be supported on all stacks, in which 00206 * case NSAPI_ERROR_UNSUPPORTED may be returned. 00207 * 00208 * @enum nsapi_socket_option 00209 */ 00210 typedef enum nsapi_socket_option { 00211 NSAPI_REUSEADDR , /*!< Allow bind to reuse local addresses */ 00212 NSAPI_KEEPALIVE , /*!< Enables sending of keepalive messages */ 00213 NSAPI_KEEPIDLE , /*!< Sets timeout value to initiate keepalive */ 00214 NSAPI_KEEPINTVL , /*!< Sets timeout value for keepalive */ 00215 NSAPI_LINGER , /*!< Keeps close from returning until queues empty */ 00216 NSAPI_SNDBUF , /*!< Sets send buffer size */ 00217 NSAPI_RCVBUF , /*!< Sets recv buffer size */ 00218 NSAPI_ADD_MEMBERSHIP , /*!< Add membership to multicast address */ 00219 NSAPI_DROP_MEMBERSHIP , /*!< Drop membership to multicast address */ 00220 } nsapi_socket_option_t; 00221 00222 /** Supported IP protocol versions of IP stack 00223 * 00224 * @enum nsapi_ip_stack 00225 */ 00226 typedef enum nsapi_ip_stack { 00227 DEFAULT_STACK = 0, 00228 IPV4_STACK, 00229 IPV6_STACK, 00230 IPV4V6_STACK 00231 } nsapi_ip_stack_t; 00232 00233 /* Backwards compatibility - previously didn't distinguish stack and socket options */ 00234 typedef nsapi_socket_level_t nsapi_level_t; 00235 typedef nsapi_socket_option_t nsapi_option_t; 00236 00237 /** nsapi_wifi_ap structure 00238 * 00239 * Structure representing a WiFi Access Point 00240 */ 00241 typedef struct nsapi_wifi_ap { 00242 char ssid[33]; /* 32 is what 802.11 defines as longest possible name; +1 for the \0 */ 00243 uint8_t bssid[6]; 00244 nsapi_security_t security; 00245 int8_t rssi; 00246 uint8_t channel; 00247 } nsapi_wifi_ap_t; 00248 00249 00250 /** nsapi_stack structure 00251 * 00252 * Stack structure representing a specific instance of a stack. 00253 */ 00254 typedef struct nsapi_stack { 00255 /** Network stack operation table 00256 * 00257 * Provides access to the underlying api of the stack. This is not 00258 * flattened into the nsapi_stack to allow allocation in read-only 00259 * memory. 00260 */ 00261 const struct nsapi_stack_api *stack_api; 00262 00263 /** Opaque handle for network stacks 00264 */ 00265 void *stack; 00266 00267 // Internal nsapi buffer 00268 unsigned _stack_buffer[16]; 00269 } nsapi_stack_t; 00270 00271 /** nsapi_ip_mreq structure 00272 */ 00273 typedef struct nsapi_ip_mreq { 00274 nsapi_addr_t imr_multiaddr; /* IP multicast address of group */ 00275 nsapi_addr_t imr_interface; /* local IP address of interface */ 00276 } nsapi_ip_mreq_t; 00277 00278 /** nsapi_stack_api structure 00279 * 00280 * Common api structure for network stack operations. A network stack 00281 * can provide a nsapi_stack_api structure filled out with the 00282 * appropriate implementation. 00283 * 00284 * Unsupported operations can be left as null pointers. 00285 */ 00286 typedef struct nsapi_stack_api 00287 { 00288 /** Get the local IP address 00289 * 00290 * @param stack Stack handle 00291 * @return Local IP Address or null address if not connected 00292 */ 00293 nsapi_addr_t (*get_ip_address)(nsapi_stack_t *stack); 00294 00295 /** Translates a hostname to an IP address 00296 * 00297 * The hostname may be either a domain name or an IP address. If the 00298 * hostname is an IP address, no network transactions will be performed. 00299 * 00300 * If no stack-specific DNS resolution is provided, the hostname 00301 * will be resolve using a UDP socket on the stack. 00302 * 00303 * @param stack Stack handle 00304 * @param addr Destination for the host IP address 00305 * @param host Hostname to resolve 00306 * @param version Address family 00307 * @return 0 on success, negative error code on failure 00308 */ 00309 nsapi_error_t (*gethostbyname)(nsapi_stack_t *stack, const char *host, nsapi_addr_t *addr, nsapi_version_t version); 00310 00311 /** Add a domain name server to list of servers to query 00312 * 00313 * @param addr Destination for the host address 00314 * @return 0 on success, negative error code on failure 00315 */ 00316 nsapi_error_t (*add_dns_server)(nsapi_stack_t *stack, nsapi_addr_t addr); 00317 00318 /* Set stack-specific stack options 00319 * 00320 * The setstackopt allow an application to pass stack-specific hints 00321 * to the underlying stack. For unsupported options, 00322 * NSAPI_ERROR_UNSUPPORTED is returned and the stack is unmodified. 00323 * 00324 * @param stack Stack handle 00325 * @param level Stack-specific protocol level 00326 * @param optname Stack-specific option identifier 00327 * @param optval Option value 00328 * @param optlen Length of the option value 00329 * @return 0 on success, negative error code on failure 00330 */ 00331 nsapi_error_t (*setstackopt)(nsapi_stack_t *stack, int level, 00332 int optname, const void *optval, unsigned optlen); 00333 00334 /* Get stack-specific stack options 00335 * 00336 * The getstackopt allow an application to retrieve stack-specific hints 00337 * from the underlying stack. For unsupported options, 00338 * NSAPI_ERROR_UNSUPPORTED is returned and optval is unmodified. 00339 * 00340 * @param stack Stack handle 00341 * @param level Stack-specific protocol level 00342 * @param optname Stack-specific option identifier 00343 * @param optval Destination for option value 00344 * @param optlen Length of the option value 00345 * @return 0 on success, negative error code on failure 00346 */ 00347 nsapi_error_t (*getstackopt)(nsapi_stack_t *stack, int level, 00348 int optname, void *optval, unsigned *optlen); 00349 00350 /** Opens a socket 00351 * 00352 * Creates a network socket and stores it in the specified handle. 00353 * The handle must be passed to following calls on the socket. 00354 * 00355 * A stack may have a finite number of sockets, in this case 00356 * NSAPI_ERROR_NO_SOCKET is returned if no socket is available. 00357 * 00358 * @param stack Stack context 00359 * @param socket Destination for the handle to a newly created socket 00360 * @param proto Protocol of socket to open, NSAPI_TCP or NSAPI_UDP 00361 * @return 0 on success, negative error code on failure 00362 */ 00363 nsapi_error_t (*socket_open)(nsapi_stack_t *stack, nsapi_socket_t *socket, 00364 nsapi_protocol_t proto); 00365 00366 /** Close the socket 00367 * 00368 * Closes any open connection and deallocates any memory associated 00369 * with the socket. 00370 * 00371 * @param stack Stack handle 00372 * @param socket Socket handle 00373 * @return 0 on success, negative error code on failure 00374 */ 00375 nsapi_error_t (*socket_close)(nsapi_stack_t *stack, nsapi_socket_t socket); 00376 00377 /** Bind a specific address to a socket 00378 * 00379 * Binding a socket specifies the address and port on which to recieve 00380 * data. If the IP address is zeroed, only the port is bound. 00381 * 00382 * @param stack Stack handle 00383 * @param socket Socket handle 00384 * @param addr Local address to bind, may be null 00385 * @param port Local port to bind 00386 * @return 0 on success, negative error code on failure. 00387 */ 00388 nsapi_error_t (*socket_bind)(nsapi_stack_t *stack, nsapi_socket_t socket, 00389 nsapi_addr_t addr, uint16_t port); 00390 00391 /** Listen for connections on a TCP socket 00392 * 00393 * Marks the socket as a passive socket that can be used to accept 00394 * incoming connections. 00395 * 00396 * @param stack Stack handle 00397 * @param socket Socket handle 00398 * @param backlog Number of pending connections that can be queued 00399 * simultaneously 00400 * @return 0 on success, negative error code on failure 00401 */ 00402 nsapi_error_t (*socket_listen)(nsapi_stack_t *stack, nsapi_socket_t socket, int backlog); 00403 00404 /** Connects TCP socket to a remote host 00405 * 00406 * Initiates a connection to a remote server specified by the 00407 * indicated address. 00408 * 00409 * @param stack Stack handle 00410 * @param socket Socket handle 00411 * @param addr The address of the remote host 00412 * @param port The port of the remote host 00413 * @return 0 on success, negative error code on failure 00414 */ 00415 nsapi_error_t (*socket_connect)(nsapi_stack_t *stack, nsapi_socket_t socket, 00416 nsapi_addr_t addr, uint16_t port); 00417 00418 /** Accepts a connection on a TCP socket 00419 * 00420 * The server socket must be bound and set to listen for connections. 00421 * On a new connection, creates a network socket and stores it in the 00422 * specified handle. The handle must be passed to following calls on 00423 * the socket. 00424 * 00425 * A stack may have a finite number of sockets, in this case 00426 * NSAPI_ERROR_NO_SOCKET is returned if no socket is available. 00427 * 00428 * This call is non-blocking. If accept would block, 00429 * NSAPI_ERROR_WOULD_BLOCK is returned immediately. 00430 * 00431 * @param stack Stack handle 00432 * @param server Socket handle to server to accept from 00433 * @param socket Destination for a handle to the newly created socket 00434 * @param addr Destination for the address of the remote host 00435 * @param port Destination for the port of the remote host 00436 * @return 0 on success, negative error code on failure 00437 */ 00438 nsapi_error_t (*socket_accept)(nsapi_stack_t *stack, nsapi_socket_t server, 00439 nsapi_socket_t *socket, nsapi_addr_t *addr, uint16_t *port); 00440 00441 /** Send data over a TCP socket 00442 * 00443 * The socket must be connected to a remote host. Returns the number of 00444 * bytes sent from the buffer. 00445 * 00446 * This call is non-blocking. If send would block, 00447 * NSAPI_ERROR_WOULD_BLOCK is returned immediately. 00448 * 00449 * @param stack Stack handle 00450 * @param socket Socket handle 00451 * @param data Buffer of data to send to the host 00452 * @param size Size of the buffer in bytes 00453 * @return Number of sent bytes on success, negative error 00454 * code on failure 00455 */ 00456 nsapi_size_or_error_t (*socket_send)(nsapi_stack_t *stack, nsapi_socket_t socket, 00457 const void *data, nsapi_size_t size); 00458 00459 /** Receive data over a TCP socket 00460 * 00461 * The socket must be connected to a remote host. Returns the number of 00462 * bytes received into the buffer. 00463 * 00464 * This call is non-blocking. If recv would block, 00465 * NSAPI_ERROR_WOULD_BLOCK is returned immediately. 00466 * 00467 * @param stack Stack handle 00468 * @param socket Socket handle 00469 * @param data Destination buffer for data received from the host 00470 * @param size Size of the buffer in bytes 00471 * @return Number of received bytes on success, negative error 00472 * code on failure 00473 */ 00474 nsapi_size_or_error_t (*socket_recv)(nsapi_stack_t *stack, nsapi_socket_t socket, 00475 void *data, nsapi_size_t size); 00476 00477 /** Send a packet over a UDP socket 00478 * 00479 * Sends data to the specified address. Returns the number of bytes 00480 * sent from the buffer. 00481 * 00482 * This call is non-blocking. If sendto would block, 00483 * NSAPI_ERROR_WOULD_BLOCK is returned immediately. 00484 * 00485 * @param stack Stack handle 00486 * @param socket Socket handle 00487 * @param addr The address of the remote host 00488 * @param port The port of the remote host 00489 * @param data Buffer of data to send to the host 00490 * @param size Size of the buffer in bytes 00491 * @return Number of sent bytes on success, negative error 00492 * code on failure 00493 */ 00494 nsapi_size_or_error_t (*socket_sendto)(nsapi_stack_t *stack, nsapi_socket_t socket, 00495 nsapi_addr_t addr, uint16_t port, const void *data, nsapi_size_t size); 00496 00497 /** Receive a packet over a UDP socket 00498 * 00499 * Receives data and stores the source address in address if address 00500 * is not NULL. Returns the number of bytes received into the buffer. 00501 * 00502 * This call is non-blocking. If recvfrom would block, 00503 * NSAPI_ERROR_WOULD_BLOCK is returned immediately. 00504 * 00505 * @param stack Stack handle 00506 * @param socket Socket handle 00507 * @param addr Destination for the address of the remote host 00508 * @param port Destination for the port of the remote host 00509 * @param data Destination buffer for data received from the host 00510 * @param size Size of the buffer in bytes 00511 * @return Number of received bytes on success, negative error 00512 * code on failure 00513 */ 00514 nsapi_size_or_error_t (*socket_recvfrom)(nsapi_stack_t *stack, nsapi_socket_t socket, 00515 nsapi_addr_t *addr, uint16_t *port, void *buffer, nsapi_size_t size); 00516 00517 /** Register a callback on state change of the socket 00518 * 00519 * The specified callback will be called on state changes such as when 00520 * the socket can recv/send/accept successfully and on when an error 00521 * occurs. The callback may also be called spuriously without reason. 00522 * 00523 * The callback may be called in an interrupt context and should not 00524 * perform expensive operations such as recv/send calls. 00525 * 00526 * @param stack Stack handle 00527 * @param socket Socket handle 00528 * @param callback Function to call on state change 00529 * @param data Argument to pass to callback 00530 */ 00531 void (*socket_attach)(nsapi_stack_t *stack, nsapi_socket_t socket, 00532 void (*callback)(void *), void *data); 00533 00534 /* Set stack-specific socket options 00535 * 00536 * The setsockopt allow an application to pass stack-specific hints 00537 * to the underlying stack. For unsupported options, 00538 * NSAPI_ERROR_UNSUPPORTED is returned and the socket is unmodified. 00539 * 00540 * @param stack Stack handle 00541 * @param socket Socket handle 00542 * @param level Stack-specific protocol level 00543 * @param optname Stack-specific option identifier 00544 * @param optval Option value 00545 * @param optlen Length of the option value 00546 * @return 0 on success, negative error code on failure 00547 */ 00548 nsapi_error_t (*setsockopt)(nsapi_stack_t *stack, nsapi_socket_t socket, int level, 00549 int optname, const void *optval, unsigned optlen); 00550 00551 /* Get stack-specific socket options 00552 * 00553 * The getstackopt allow an application to retrieve stack-specific hints 00554 * from the underlying stack. For unsupported options, 00555 * NSAPI_ERROR_UNSUPPORTED is returned and optval is unmodified. 00556 * 00557 * @param stack Stack handle 00558 * @param socket Socket handle 00559 * @param level Stack-specific protocol level 00560 * @param optname Stack-specific option identifier 00561 * @param optval Destination for option value 00562 * @param optlen Length of the option value 00563 * @return 0 on success, negative error code on failure 00564 */ 00565 nsapi_error_t (*getsockopt)(nsapi_stack_t *stack, nsapi_socket_t socket, int level, 00566 int optname, void *optval, unsigned *optlen); 00567 } nsapi_stack_api_t; 00568 00569 00570 #ifdef __cplusplus 00571 } 00572 #endif 00573 00574 #endif 00575 00576 /** @}*/
Generated on Sun Jul 17 2022 08:25:28 by
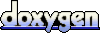