
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
nsapi_ppp.h
00001 /** \addtogroup netsocket */ 00002 /** @{*/ 00003 /* nsapi_ppp.h 00004 * Modified work Copyright (c) 2017 ARM Limited 00005 * 00006 * Licensed under the Apache License, Version 2.0 (the "License"); 00007 * you may not use this file except in compliance with the License. 00008 * You may obtain a copy of the License at 00009 * 00010 * http://www.apache.org/licenses/LICENSE-2.0 00011 * 00012 * Unless required by applicable law or agreed to in writing, software 00013 * distributed under the License is distributed on an "AS IS" BASIS, 00014 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 * See the License for the specific language governing permissions and 00016 * limitations under the License. 00017 */ 00018 #ifndef NSAPI_PPP_H_ 00019 #define NSAPI_PPP_H_ 00020 00021 #include "FileHandle.h" 00022 #include "NetworkStack.h" 00023 00024 namespace mbed { 00025 00026 /** Provide access to the NetworkStack object 00027 * 00028 * @return The underlying NetworkStack object 00029 */ 00030 NetworkStack *nsapi_ppp_get_stack(); 00031 00032 00033 /** Connect to a PPP pipe 00034 * 00035 * @param stream Pointer to a device type file handle (descriptor) 00036 * @param status_cb Optional, user provided callback for connection status 00037 * @param uname Optional, username for the connection 00038 * @param pwd Optional, password for the connection 00039 * @param stack Optional, stack for the connection 00040 * 00041 * @return 0 on success, negative error code on failure 00042 */ 00043 nsapi_error_t nsapi_ppp_connect(FileHandle *stream, Callback<void(nsapi_error_t)> status_cb=0, const char *uname=0, const char *pwd=0, const nsapi_ip_stack_t stack=DEFAULT_STACK); 00044 00045 /** Close a PPP connection 00046 * 00047 * @param stream Pointer to a device type file handle (descriptor) 00048 * 00049 * @return 0 on success, negative error code on failure 00050 */ 00051 nsapi_error_t nsapi_ppp_disconnect(FileHandle *stream); 00052 00053 /** Get IP address 00054 * 00055 * After a successful connection, this API can be used to retrieve assigned IP address. 00056 * 00057 * @param stream Pointer to a device type file handle (descriptor) 00058 * 00059 * @return A string containing IP address or NULL 00060 */ 00061 const char *nsapi_ppp_get_ip_addr(FileHandle *stream); 00062 00063 /** Get network mask 00064 * 00065 * After a successful connection, this API can be used to retrieve network mask 00066 * in case of an IPv4 network. 00067 * 00068 * @param stream Pointer to a device type file handle (descriptor) 00069 * 00070 * @return A string containing network mask or NULL 00071 */ 00072 const char *nsapi_ppp_get_netmask(FileHandle *stream); 00073 00074 /** Get gateway address 00075 * 00076 * After a successful connection, this API can be used to retrieve IP address 00077 * of the default gateway in case of an IPv4 network. 00078 * 00079 * @param stream Pointer to a device type file handle (descriptor) 00080 * 00081 * @return A string containing gateway IP address or NULL 00082 */ 00083 const char *nsapi_ppp_get_gw_addr(FileHandle *stream); 00084 00085 } //namespace mbed 00086 00087 /** @} */ 00088 00089 #endif /* NSAPI_PPP_H_ */
Generated on Sun Jul 17 2022 08:25:28 by
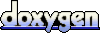