
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
nsapi_dns.h
00001 00002 /** \addtogroup netsocket */ 00003 /** @{*/ 00004 /* nsapi_dns.h 00005 * Original work Copyright (c) 2013 Henry Leinen (henry[dot]leinen [at] online [dot] de) 00006 * Modified work Copyright (c) 2015 ARM Limited 00007 * 00008 * Licensed under the Apache License, Version 2.0 (the "License"); 00009 * you may not use this file except in compliance with the License. 00010 * You may obtain a copy of the License at 00011 * 00012 * http://www.apache.org/licenses/LICENSE-2.0 00013 * 00014 * Unless required by applicable law or agreed to in writing, software 00015 * distributed under the License is distributed on an "AS IS" BASIS, 00016 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00017 * See the License for the specific language governing permissions and 00018 * limitations under the License. 00019 */ 00020 #ifndef NSAPI_DNS_H 00021 #define NSAPI_DNS_H 00022 00023 #include "nsapi_types.h" 00024 #ifdef __cplusplus 00025 #include "netsocket/NetworkStack.h" 00026 #endif 00027 00028 #ifndef __cplusplus 00029 00030 00031 /** Query a domain name server for an IP address of a given hostname 00032 * 00033 * @param stack Network stack as target for DNS query 00034 * @param host Hostname to resolve 00035 * @param addr Destination for the host address 00036 * @param version IP version to resolve 00037 * @return 0 on success, negative error code on failure 00038 * NSAPI_ERROR_DNS_FAILURE indicates the host could not be found 00039 */ 00040 nsapi_error_t nsapi_dns_query(nsapi_stack_t *stack, const char *host, 00041 nsapi_addr_t *addr, nsapi_version_t version); 00042 00043 /** Query a domain name server for multiple IP address of a given hostname 00044 * 00045 * @param stack Network stack as target for DNS query 00046 * @param host Hostname to resolve 00047 * @param addr Array for the host addresses 00048 * @param addr_count Number of addresses allocated in the array 00049 * @param version IP version to resolve 00050 * @return Number of addresses found on success, negative error code on failure 00051 * NSAPI_ERROR_DNS_FAILURE indicates the host could not be found 00052 */ 00053 nsapi_size_or_error_t nsapi_dns_query_multiple(nsapi_stack_t *stack, const char *host, 00054 nsapi_addr_t *addr, nsapi_size_t addr_count, nsapi_version_t version); 00055 00056 /** Add a domain name server to list of servers to query 00057 * 00058 * @param addr Destination for the host address 00059 * @return 0 on success, negative error code on failure 00060 */ 00061 nsapi_error_t nsapi_dns_add_server(nsapi_addr_t addr); 00062 00063 00064 #else 00065 00066 00067 /** Query a domain name server for an IP address of a given hostname 00068 * 00069 * @param stack Network stack as target for DNS query 00070 * @param host Hostname to resolve 00071 * @param addr Destination for the host address 00072 * @param version IP version to resolve (defaults to NSAPI_IPv4) 00073 * @return 0 on success, negative error code on failure 00074 * NSAPI_ERROR_DNS_FAILURE indicates the host could not be found 00075 */ 00076 nsapi_error_t nsapi_dns_query(NetworkStack *stack, const char *host, 00077 SocketAddress *addr, nsapi_version_t version = NSAPI_IPv4 ); 00078 00079 /** Query a domain name server for an IP address of a given hostname 00080 * 00081 * @param stack Network stack as target for DNS query 00082 * @param host Hostname to resolve 00083 * @param addr Destination for the host address 00084 * @param version IP version to resolve (defaults to NSAPI_IPv4) 00085 * @return 0 on success, negative error code on failure 00086 * NSAPI_ERROR_DNS_FAILURE indicates the host could not be found 00087 */ 00088 extern "C" nsapi_error_t nsapi_dns_query(nsapi_stack_t *stack, const char *host, 00089 nsapi_addr_t *addr, nsapi_version_t version = NSAPI_IPv4 ); 00090 00091 /** Query a domain name server for an IP address of a given hostname 00092 * 00093 * @param stack Network stack as target for DNS query 00094 * @param host Hostname to resolve 00095 * @param addr Destination for the host address 00096 * @param version IP version to resolve (defaults to NSAPI_IPv4) 00097 * @return 0 on success, negative error code on failure 00098 * NSAPI_ERROR_DNS_FAILURE indicates the host could not be found 00099 */ 00100 template <typename S> 00101 nsapi_error_t nsapi_dns_query(S *stack, const char *host, 00102 SocketAddress *addr, nsapi_version_t version = NSAPI_IPv4 ) 00103 { 00104 return nsapi_dns_query(nsapi_create_stack(stack), host, addr, version); 00105 } 00106 00107 /** Query a domain name server for multiple IP address of a given hostname 00108 * 00109 * @param stack Network stack as target for DNS query 00110 * @param host Hostname to resolve 00111 * @param addr Array for the host addresses 00112 * @param addr_count Number of addresses allocated in the array 00113 * @param version IP version to resolve (defaults to NSAPI_IPv4) 00114 * @return Number of addresses found on success, negative error code on failure 00115 * NSAPI_ERROR_DNS_FAILURE indicates the host could not be found 00116 */ 00117 nsapi_size_or_error_t nsapi_dns_query_multiple(NetworkStack *stack, const char *host, 00118 SocketAddress *addr, nsapi_size_t addr_count, nsapi_version_t version = NSAPI_IPv4 ); 00119 00120 /** Query a domain name server for multiple IP address of a given hostname 00121 * 00122 * @param stack Network stack as target for DNS query 00123 * @param host Hostname to resolve 00124 * @param addr Array for the host addresses 00125 * @param addr_count Number of addresses allocated in the array 00126 * @param version IP version to resolve (defaults to NSAPI_IPv4) 00127 * @return Number of addresses found on success, negative error code on failure 00128 * NSAPI_ERROR_DNS_FAILURE indicates the host could not be found 00129 */ 00130 extern "C" nsapi_size_or_error_t nsapi_dns_query_multiple(nsapi_stack_t *stack, const char *host, 00131 nsapi_addr_t *addr, nsapi_size_t addr_count, nsapi_version_t version = NSAPI_IPv4 ); 00132 00133 /** Query a domain name server for multiple IP address of a given hostname 00134 * 00135 * @param stack Network stack as target for DNS query 00136 * @param host Hostname to resolve 00137 * @param addr Array for the host addresses 00138 * @param addr_count Number of addresses allocated in the array 00139 * @param version IP version to resolve (defaults to NSAPI_IPv4) 00140 * @return Number of addresses found on success, negative error code on failure 00141 * NSAPI_ERROR_DNS_FAILURE indicates the host could not be found 00142 */ 00143 template <typename S> 00144 nsapi_size_or_error_t nsapi_dns_query_multiple(S *stack, const char *host, 00145 SocketAddress *addr, nsapi_size_t addr_count, nsapi_version_t version = NSAPI_IPv4 ) 00146 { 00147 return nsapi_dns_query_multiple(nsapi_create_stack(stack), 00148 host, addr, addr_count, version); 00149 } 00150 00151 /** Add a domain name server to list of servers to query 00152 * 00153 * @param addr Destination for the host address 00154 * @return 0 on success, negative error code on failure 00155 */ 00156 extern "C" nsapi_error_t nsapi_dns_add_server(nsapi_addr_t addr); 00157 00158 /** Add a domain name server to list of servers to query 00159 * 00160 * @param addr Destination for the host address 00161 * @return 0 on success, negative error code on failure 00162 */ 00163 static inline nsapi_error_t nsapi_dns_add_server(const SocketAddress &address) 00164 { 00165 return nsapi_dns_add_server(address.get_addr()); 00166 } 00167 00168 /** Add a domain name server to list of servers to query 00169 * 00170 * @param addr Destination for the host address 00171 * @return 0 on success, negative error code on failure 00172 */ 00173 static inline nsapi_error_t nsapi_dns_add_server(const char *address) 00174 { 00175 return nsapi_dns_add_server(SocketAddress(address)); 00176 } 00177 00178 00179 #endif 00180 00181 #endif 00182 00183 /** @}*/
Generated on Sun Jul 17 2022 08:25:28 by
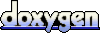