
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ns_trace_stub.c
00001 /* 00002 * Copyright (c) 2014-2016 ARM Limited. All rights reserved. 00003 */ 00004 00005 #include <stdio.h> 00006 #include <string.h> 00007 #include <stdarg.h> 00008 #include <stdlib.h> 00009 00010 #include "ns_trace.h" 00011 #include "ip6string.h" 00012 #include "common_functions.h" 00013 00014 #if defined(_WIN32) || defined(__unix__) || defined(__unix) || defined(unix) 00015 #ifndef MEM_ALLOC 00016 #define MEM_ALLOC malloc 00017 #endif 00018 #ifndef MEM_FREE 00019 #define MEM_FREE free 00020 #endif 00021 #else 00022 #include "nsdynmemLIB.h" 00023 #ifndef MEM_ALLOC 00024 #define MEM_ALLOC ns_dyn_mem_alloc 00025 #endif 00026 #ifndef MEM_FREE 00027 #define MEM_FREE ns_dyn_mem_free 00028 #endif 00029 #endif 00030 00031 #define VT100_COLOR_ERROR "\x1b[31m" 00032 #define VT100_COLOR_WARN "\x1b[33m" 00033 #define VT100_COLOR_INFO "\x1b[39m" 00034 #define VT100_COLOR_DEBUG "\x1b[90m" 00035 00036 /** default max trace line size in bytes */ 00037 #define DEFAULT_TRACE_LINE_LENGTH 1024 00038 /** default max temporary buffer size in bytes, used in 00039 trace_ipv6, trace_array and trace_strn */ 00040 #define DEFAULT_TRACE_TMP_LINE_LEN 128 00041 /** default max filters (include/exclude) length in bytes */ 00042 #define DEFAULT_TRACE_FILTER_LENGTH 24 00043 00044 static void default_print(const char *str); 00045 00046 typedef struct { 00047 /** trace configuration bits */ 00048 uint8_t trace_config; 00049 /** exclude filters list, related group name */ 00050 char *filters_exclude; 00051 /** include filters list, related group name */ 00052 char *filters_include; 00053 /** Filters length */ 00054 int filters_length; 00055 /** trace line */ 00056 char *line; 00057 /** trace line length */ 00058 int line_length; 00059 /** temporary data */ 00060 char *tmp_data; 00061 /** temporary data array length */ 00062 int tmp_data_length; 00063 /** temporary data pointer */ 00064 char *tmp_data_ptr; 00065 00066 /** prefix function, which can be used to put time to the trace line */ 00067 char *(*prefix_f)(size_t); 00068 /** suffix function, which can be used to some string to the end of trace line */ 00069 char *(*suffix_f)(void); 00070 /** print out function. Can be redirect to flash for example. */ 00071 void (*printf)(const char *); 00072 /** print out function for TRACE_LEVEL_CMD */ 00073 void (*cmd_printf)(const char *); 00074 } trace_s; 00075 00076 static trace_s m_trace = { 00077 .filters_exclude = 0, 00078 .filters_include = 0, 00079 .line = 0, 00080 .tmp_data = 0, 00081 .prefix_f = 0, 00082 .suffix_f = 0, 00083 .printf = 0, 00084 .cmd_printf = 0 00085 }; 00086 00087 int trace_init(void) 00088 { 00089 return 0; 00090 } 00091 void trace_free(void) 00092 { 00093 00094 } 00095 00096 void set_trace_config(uint8_t config) 00097 { 00098 00099 } 00100 uint8_t get_trace_config(void) 00101 { 00102 return 0; 00103 } 00104 void set_trace_prefix_function(char *(*pref_f)(size_t)) 00105 { 00106 } 00107 00108 void set_trace_suffix_function(char *(*suffix_f)(void)) 00109 { 00110 } 00111 00112 void set_trace_print_function(void (*printf)(const char *)) 00113 { 00114 } 00115 00116 void set_trace_cmdprint_function(void (*printf)(const char *)) 00117 { 00118 } 00119 00120 void set_trace_exclude_filters(char *filters) 00121 { 00122 } 00123 const char *get_trace_exclude_filters(void) 00124 { 00125 return NULL; 00126 } 00127 00128 const char *get_trace_include_filters(void) 00129 { 00130 return NULL; 00131 } 00132 00133 void set_trace_include_filters(char *filters) 00134 { 00135 } 00136 00137 static int8_t trace_skip(int8_t dlevel, const char *grp) 00138 { 00139 return 0; 00140 } 00141 00142 static void default_print(const char *str) 00143 { 00144 } 00145 00146 void tracef(uint8_t dlevel, const char *grp, const char *fmt, ...) 00147 { 00148 00149 } 00150 const char *trace_last(void) 00151 { 00152 return ""; 00153 } 00154 00155 /* Helping functions */ 00156 #define tmp_data_left() m_trace.tmp_data_length-(m_trace.tmp_data_ptr-m_trace.tmp_data) 00157 char *trace_ipv6(const void *addr_ptr) 00158 { 00159 return ""; 00160 } 00161 00162 char *trace_ipv6_prefix(const uint8_t *prefix, uint8_t prefix_len) 00163 { 00164 return ""; 00165 } 00166 00167 char *trace_array(const uint8_t *buf, uint16_t len) 00168 { 00169 return ""; 00170 } 00171 00172 // rest of debug print functions will be obsolete and will be overridden with new trace interface.. 00173 void debugf(const char *fmt, ...) 00174 { 00175 } 00176 00177 void debug(const char *s) 00178 { 00179 } 00180 00181 void debug_put(char c) 00182 { 00183 } 00184 00185 void debug_hex(uint8_t x) 00186 { 00187 } 00188 00189 void debug_int(int i) 00190 { 00191 } 00192 00193 void printf_array(const void *buf , uint16_t len) 00194 { 00195 } 00196 00197 void printf_ipv6_address(const void *addr_ptr) 00198 { 00199 } 00200 00201 void printf_string(const void *ptr, uint16_t len) 00202 { 00203 } 00204
Generated on Sun Jul 17 2022 08:25:28 by
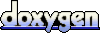